Resolving AJAX Integration Issues with CKEditor in Spring Boot
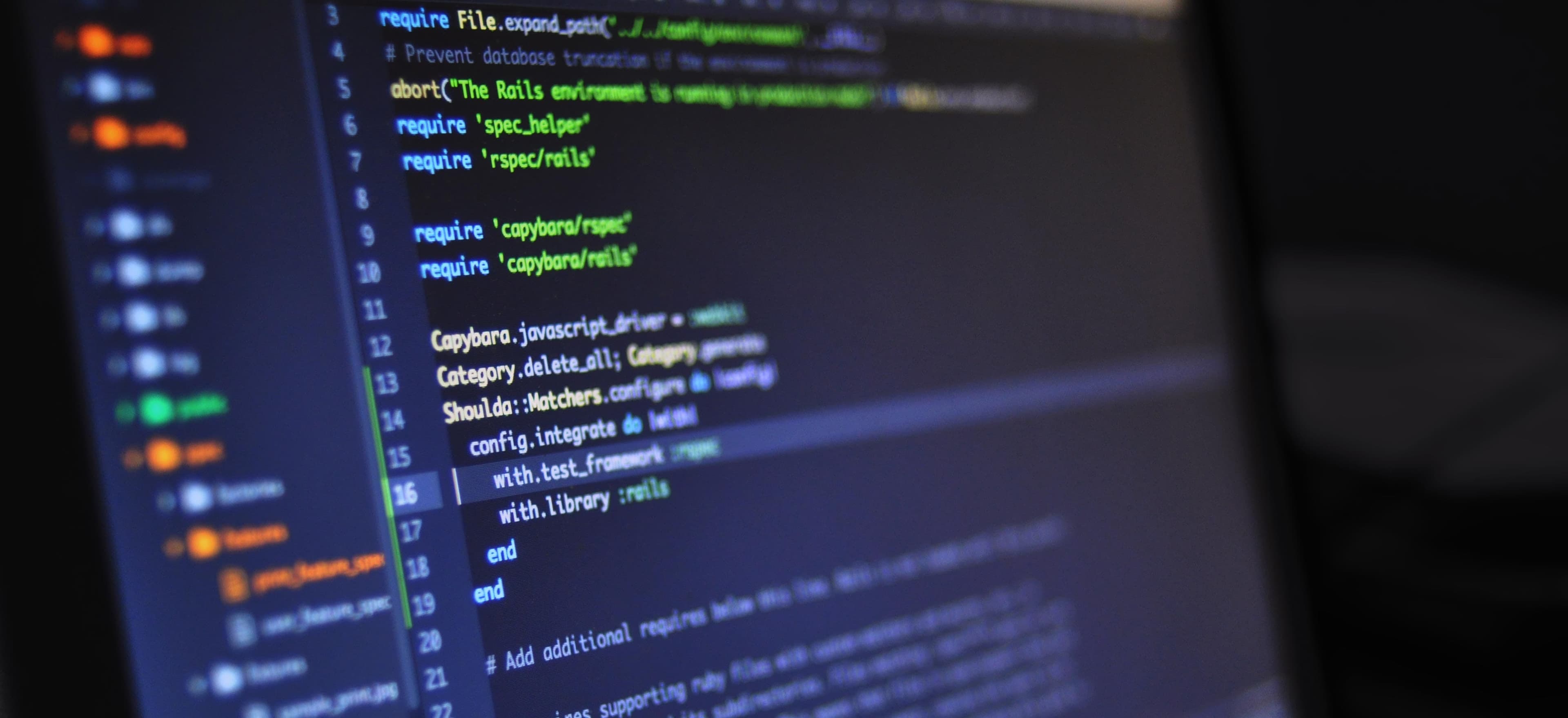
- Published on
Resolving AJAX Integration Issues with CKEditor in Spring Boot
Integrating CKEditor with a Spring Boot application can be an empowering combination, blending a robust web framework with a rich text editor. Developers often encounter AJAX-related integration complexities. This blog aims to dissect these common pitfalls and provide efficient solutions, enhancing the overall development experience.
What is CKEditor?
CKEditor is a popular WYSIWYG (What You See Is What You Get) editor that enables developers to create rich-text editing capabilities effortlessly. It supports various features, including image uploads, video embedding, and real-time collaboration.
Setting up CKEditor with Spring Boot
Before diving into AJAX issues, let’s set up a simple Spring Boot application that includes CKEditor.
Step 1: Create a Spring Boot Application
Create a Spring Boot project using Spring Initializr. Select Maven as the project type, and add dependencies such as Spring Web and Thymeleaf.
Step 2: Add CKEditor
Add CKEditor to your HTML file. You can either use a CDN or download its files. Here’s how to load it via CDN:
<script src="https://cdn.ckeditor.com/4.16.0/standard/ckeditor.js"></script>
Step 3: Basic HTML Structure
Below is an example of a basic HTML structure that incorporates CKEditor.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CKEditor with Spring Boot</title>
<script src="https://cdn.ckeditor.com/4.16.0/standard/ckeditor.js"></script>
</head>
<body>
<form id="editorForm">
<textarea name="editor" id="editor" rows="10" cols="80"></textarea>
<button type="submit">Submit</button>
</form>
<script>
CKEDITOR.replace('editor');
</script>
</body>
</html>
Step 4: Spring Boot Controller
Create a simple controller to handle the form submission:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class EditorController {
@PostMapping("/submit")
public String submitForm(@RequestParam("editor") String editorContent) {
// Process the content from CKEditor
System.out.println(editorContent);
return "redirect:/"; // Redirect or render a response as needed
}
}
Integrating AJAX with CKEditor
AJAX (Asynchronous JavaScript and XML) is essential in modern web applications, allowing you to send and retrieve data without refreshing the web page. Integrating it with CKEditor requires an understanding of both the client-side and server-side operations.
Step 1: Update Form Submission
Modify your form submission to use AJAX instead of the traditional POST method.
<script>
document.getElementById('editorForm').addEventListener('submit', function(event) {
event.preventDefault(); // Prevents the default form submission
var editorContent = CKEDITOR.instances.editor.getData(); // Get data from CKEditor
fetch('/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: 'editor=' + encodeURIComponent(editorContent) // Encode content data
})
.then(response => {
if (response.ok) {
alert('Content submitted successfully!');
} else {
alert('Submission failed!');
}
})
.catch(error => console.error('Error:', error));
});
</script>
Step 2: Handle AJAX Request in Spring Boot
In the controller, there's no need for significant changes since we already handle the POST
request. Just ensure that content processing caters to AJAX calls.
Common AJAX Integration Issues
While integrating CKEditor with AJAX in Spring Boot, you might run into several issues. Here are a couple of the most prevalent problems and their solutions:
Issue 1: CORS Policy Restrictions
When sending AJAX requests, the browser’s Same-Origin Policy can restrict cross-origin requests. To resolve this, you can configure CORS in your Spring Boot application.
Solution: Add CORS Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
// Allow cross-origin requests from specific domains
registry.addMapping("/**").allowedOrigins("http://localhost:3000").allowedMethods("GET", "POST");
}
}
Issue 2: Incorrect Content-Type Header
AJAX requests must specify the correct Content-Type
header. Ensure the header matches the expected response on the server side.
Solution: Ensuring Content-Type
This is managed automatically with the fetch
API if you specify the header:
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
Issue 3: Data Not Correctly Formatted
When sending data through AJAX, ensure the data format is correctly handled. In our example, the CKEditor content must be URL-encoded before sending.
Solution: Use encodeURIComponent()
As illustrated in the AJAX submission code:
body: 'editor=' + encodeURIComponent(editorContent)
Testing the AJAX Integration
Make sure to thoroughly test your AJAX integration. Open the browser's developer tools (F12) and check the Network tab to see the AJAX request and response. Ensure that all content is processed as expected.
Expanding the Integration
Once you have successfully integrated CKEditor with AJAX in your Spring Boot application, consider extending its functionality:
- Implement auto-saving of draft content.
- Handle file uploads within CKEditor.
- Add real-time collaboration features using WebSockets.
The Last Word
Combining CKEditor with Spring Boot via AJAX can dramatically enhance the user experience within your application. Although challenges may arise during this process, understanding their sources can streamline integration.
By following the guidelines presented, you can effectively resolve AJAX integration issues. For further insights into Spring Boot and AJAX, consider exploring the Spring Boot Documentation or check out MDN Web Docs for more information on HTTP headers.
Happy coding!
Checkout our other articles