Common Misuses of Hamcrest Matchers in Testing
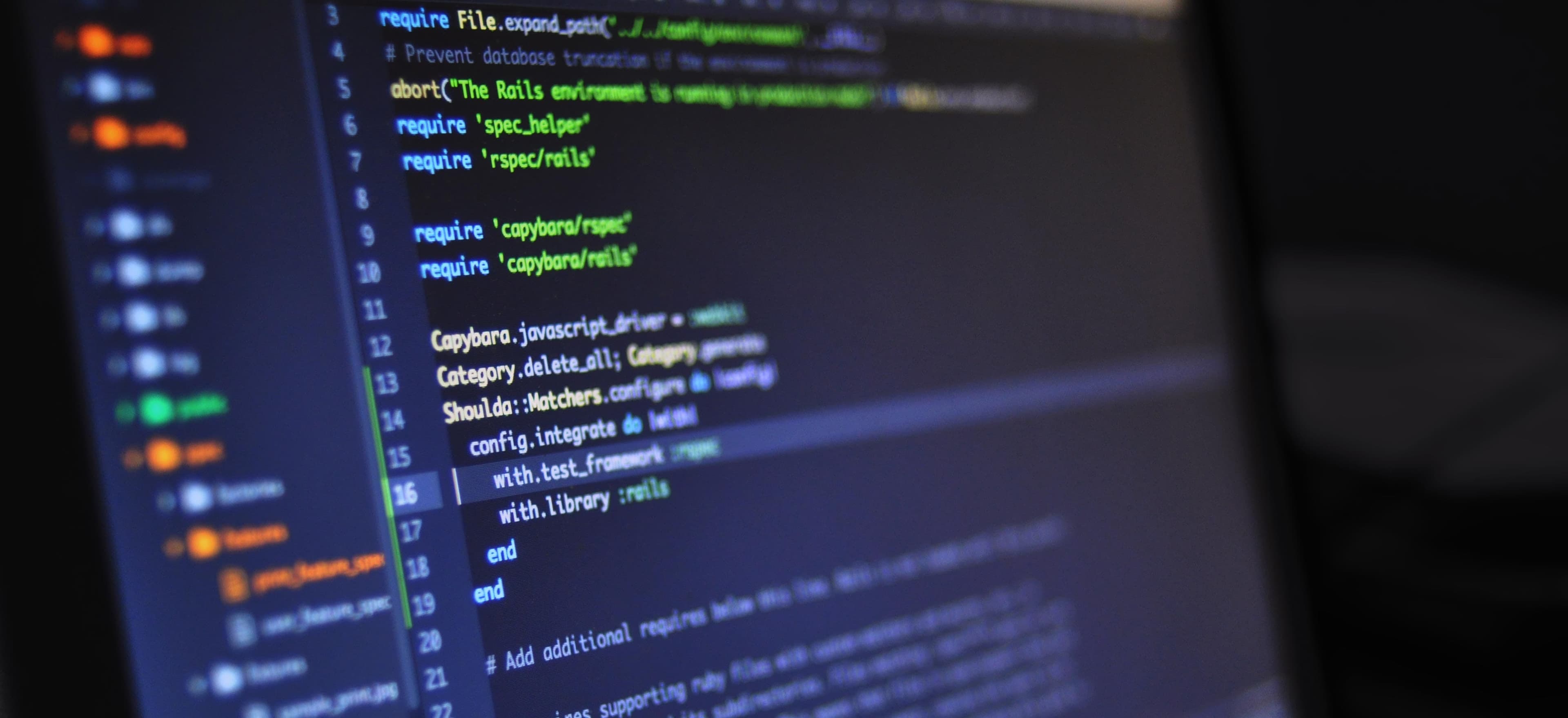
- Published on
Common Misuses of Hamcrest Matchers in Testing
When it comes to testing in Java, Hamcrest Matchers have become an industry favorite due to their expressive syntax. They provide a flexible and readable way to write assertions, allowing for better understanding and maintainability of tests. However, as with any powerful tool, there are potential pitfalls. This blog post will discuss some common misuses of Hamcrest Matchers and how to avoid them.
Diving Into the Subject to Hamcrest Matchers
Before diving into common misuses, let's briefly cover what Hamcrest is. Hamcrest is a framework that provides a set of matchers for making assertions in tests more readable. These matchers can be used with JUnit, TestNG, and other testing frameworks. The syntax allows developers to create human-readable tests, which can be beneficial for both new team members and stakeholders.
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
@Test
public void testStringEquality() {
String actual = "hello";
assertThat(actual, is("hello"));
}
Here, the is()
matcher makes the assertion clear, indicating that the expectation is the string "hello". However, there are common misuses that can muddy test clarity and functionality.
Common Misuses of Hamcrest Matchers
1. Overly Complex Matchers
One of the most frequent misuses is the use of overly complex matchers. While Hamcrest allows for complex expressions, it can lead to confusion. By nesting several matchers together, the readability of tests can suffer.
Example of Overly Complex Matcher:
@Test
public void testUserDetails() {
User user = new User("John", 25);
assertThat(user, allOf(hasProperty("name", is("John")), hasProperty("age", greaterThan(20))));
}
Here, the allOf
matcher is used. While it may be appropriate, excessive nesting can confuse readers about what is being asserted. Instead, consider breaking these assertions into separate tests or simplify them.
Better Approach:
@Test
public void testUserName() {
User user = new User("John", 25);
assertThat(user.getName(), is("John"));
}
@Test
public void testUserAge() {
User user = new User("John", 25);
assertThat(user.getAge(), greaterThan(20));
}
2. Not Leveraging Matchers' Descriptive Nature
Another misuse is failing to take advantage of the descriptive nature of Hamcrest matchers. Assertions should provide clear feedback about what went wrong when they fail. If you rely too heavily on raw JUnit assertions or less descriptive matchers, it can lead to harder-to-debug tests.
Less Descriptive Assertion:
@Test
public void testListSize() {
List<String> list = Arrays.asList("A", "B", "C");
assertEquals(3, list.size());
}
More Descriptive Assertion:
@Test
public void testListSizeUsingHamcrest() {
List<String> list = Arrays.asList("A", "B", "C");
assertThat(list.size(), is(3));
}
When a failure occurs, the latter provides more context, indicating that the size is expected to be 3.
3. Mixing Matchers and Raw Assertions
Mixing Hamcrest matchers with raw assertions can lead to confusion. It's best practice to choose one style and stick with it throughout your tests for consistency.
Mixed Style Example:
@Test
public void testUserListNotNull() {
List<User> users = getUserList();
assertThat(users.size(), is(greaterThan(0)));
assertNotNull(users);
}
In this case, the test combines a Hamcrest matcher with a raw assertion. Choose one:
Consistent Use of Matchers:
@Test
public void testUserListNotNull() {
List<User> users = getUserList();
assertThat(users, is(not(empty())));
}
This approach maintains consistency and leverages the expressive power of Hamcrest.
4. Using Matchers for Non-Matching Scenarios
Another misuse occurs when developers leverage matchers for conditions that are not directly about matching. For example, using a matcher for behavior, rather than state, can lead to inappropriate assertions.
Non-Matching Use of Matchers:
@Test
public void testUserCreation() {
User user = createUser("John");
assertThat(user, is(notNullValue()));
assertThat(user, hasProperty("name"));
}
In these assertions, while they check properties, they don't verify the intended behavior of createUser()
. It is more logical to check the outcome rather than just asserting the state.
Better Behavior-Oriented Assertion:
@Test
public void testUserCreation() {
User user = createUser("John");
assertThat(user.getName(), is("John"));
assertThat(user.getAge(), is(greaterThan(0)));
}
This way, you're confirming that the user was created with the expected values rather than simply verifying the existence of properties.
5. Misusing Logical Matchers
Finally, be cautious with logical matchers such as anyOf
and allOf
. Misuse can lead to tests that pass when they shouldn't or fail silently without providing context.
Example of Misuse:
@Test
public void testEmailValidation() {
String email = "test@example.com";
assertThat(email, anyOf(containsString("@"), containsString(".")));
}
In this test, you might think you're validating an email, but using anyOf
may pass tests for invalid emails like "example.com" or "user@".
Correct Validation:
@Test
public void testEmailValidation() {
String email = "test@example.com";
assertThat(email, allOf(containsString("@"), containsString(".")));
}
Here, you're ensuring the email contains both @
and a .
to mimic a more realistic structure.
Closing the Chapter
Hamcrest Matchers are powerful tools that enhance the clarity and readability of your tests. However, it is crucial to use them thoughtfully to avoid common pitfalls. By understanding and applying best practices when using Hamcrest, you can write more effective unit tests that both enhance code quality and ease collaboration within your team.
To further explore Hamcrest and its usage, consider these official Hamcrest documentation and JUnit testing framework. Always test your assertions thoroughly to ensure they align with the intended behavior and keep demonstrating clarity and expressiveness in your code.
Happy testing!
Checkout our other articles