Overcoming Endless Scrolling Issues in Android ListViews
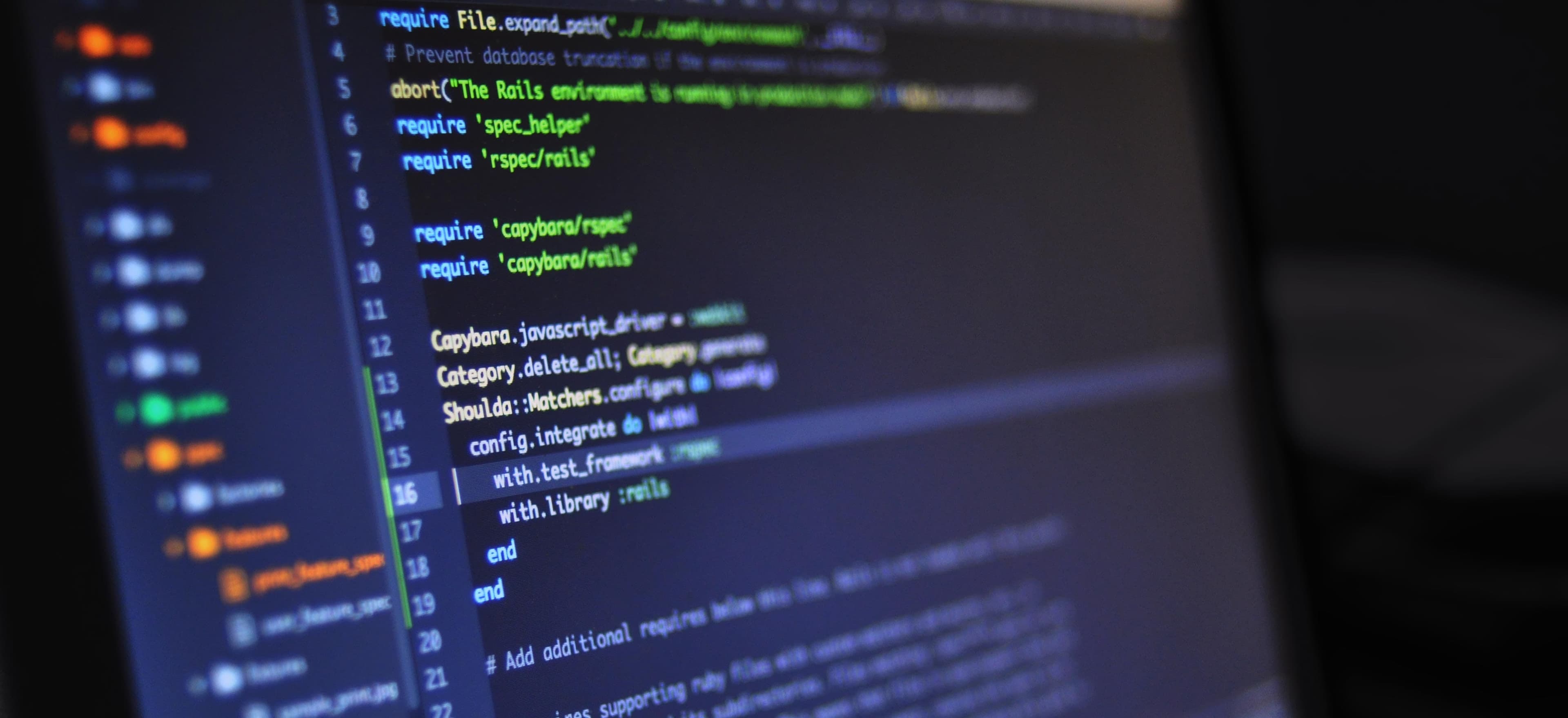
- Published on
Overcoming Endless Scrolling Issues in Android ListViews
When building an Android application, ListView
is a frequently-used component for displaying a list of items. However, developers often encounter issues with endless scrolling, which can result in performance bottlenecks, poor user experience, and unintended behavior. In this blog post, we will explore how to effectively manage endless scrolling in ListViews
, improve their performance, and create a seamless experience for users.
Understanding Endless Scrolling
Endless scrolling, also known as infinite scrolling, is a UI pattern that automatically loads data as the user scrolls down through the list. This approach provides a continuous flow of content without requiring users to navigate manually through pages. Popular applications, such as social media feeds and image galleries, utilize endless scrolling to enhance user engagement.
However, if not handled correctly, endless scrolling can lead to several issues:
- Performance Bottlenecks: Loading too much data at once can overwhelm the device memory, causing lag and stuttering.
- Data Duplication: Fetching data multiple times can result in displaying duplicate items in the list.
- Poor User Experience: Users may be unsure if more items are loading or if they've reached the end of the list.
Setting Up Your ListView
Before we delve deeper into solving the issues associated with endless scrolling, let's start with a basic implementation of a ListView
in an Android application.
// MainActivity.java
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
private ListView listView;
private ArrayAdapter<String> adapter;
private ArrayList<String> itemList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = findViewById(R.id.listView);
itemList = new ArrayList<>();
for (int i = 1; i <= 30; i++) {
itemList.add("Item " + i);
}
adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, itemList);
listView.setAdapter(adapter);
}
}
In the code snippet above, we set up a basic ListView
populated with 30 items. Now we can begin to implement an endless scrolling feature.
Implementing Endless Scrolling
To achieve endless scrolling, we need to set an OnScrollListener
on the ListView
. This listener will monitor the scrolling behavior and trigger data loading when the user reaches the bottom of the list.
Step 1: Add a Scroll Listener
We will create a method to detect when the user reaches the bottom of the ListView
.
listView.setOnScrollListener(new AbsListView.OnScrollListener() {
@Override
public void onScrollStateChanged(AbsListView view, int scrollState) {
// No implementation required
}
@Override
public void onScroll(AbsListView view, int firstVisibleItem, int visibleItemCount, int totalItemCount) {
if (firstVisibleItem + visibleItemCount >= totalItemCount - 1 && totalItemCount > 0) {
// Load more data if we've reached the end of the list
loadMoreData();
}
}
});
Explanation
firstVisibleItem
: The index of the first visible item.visibleItemCount
: The total number of visible items.totalItemCount
: The total number of items in theListView
.
By checking if the sum of firstVisibleItem
and visibleItemCount
exceeds totalItemCount
, we can determine if we have scrolled to the end of the list.
Step 2: Load More Data
Now we need a method to load more data when the user reaches the bottom of the ListView
.
private void loadMoreData() {
// Simulate loading data from a source
new Thread(new Runnable() {
@Override
public void run() {
try {
// Simulating network delay
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Adding more items to the list
runOnUiThread(new Runnable() {
@Override
public void run() {
int start = itemList.size();
for (int i = start + 1; i <= start + 10; i++) {
itemList.add("Item " + i);
}
adapter.notifyDataSetChanged();
}
});
}
}).start();
}
Explanation
- Simulate Loading: We use a separate thread to simulate a loading delay. In a real-world scenario, this could be replaced with a network call to fetch new data.
- Updating the UI: We utilize
runOnUiThread()
to update the UI elements on the main thread. After loading the new data, we notify the adapter that the dataset has changed.
Handling Performance Issues
When implementing endless scrolling, it's crucial to ensure that we don't overload the ListView
with too many items, which can lead to performance degradation. Let’s consider methods to enhance performance:
1. ViewHolder Pattern
By using the ViewHolder pattern, we can significantly reduce the number of calls to findViewById(), improving performance.
public class MyAdapter extends ArrayAdapter<String> {
private final Context context;
private final ArrayList<String> values;
public MyAdapter(Context context, ArrayList<String> values) {
super(context, R.layout.list_item_layout, values);
this.context = context;
this.values = values;
}
@NonNull
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
holder = new ViewHolder();
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.list_item_layout, parent, false);
holder.textView = convertView.findViewById(R.id.textView);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
holder.textView.setText(values.get(position));
return convertView;
}
static class ViewHolder {
TextView textView;
}
}
2. Pagination
Instead of loading all data at once, implement pagination to load data in chunks. This technique ensures that only relevant data is loaded into memory, thus preventing sluggish scrolling.
3. Placeholder & Loading Indicators
To improve user experience, consider implementing loading indicators or placeholders while new data is being fetched. This provides feedback to users that more data is loading and encourages continued interaction.
Closing the Chapter
Implementing endless scrolling in Android ListView
can be a powerful feature when executed correctly. By managing data loading efficiently, employing a ViewHolder pattern, and keeping performance best practices in mind, we can create a smooth and responsive user experience.
For further enhancements and deeper understanding, feel free to explore the Android Developer Guide on ListView and ListView Pagination Methods.
By utilizing the techniques discussed above, you’re well-equipped to tackle endless scrolling in your Android applications. Happy coding!