Why Poor Cohesion Harms Your Software Design
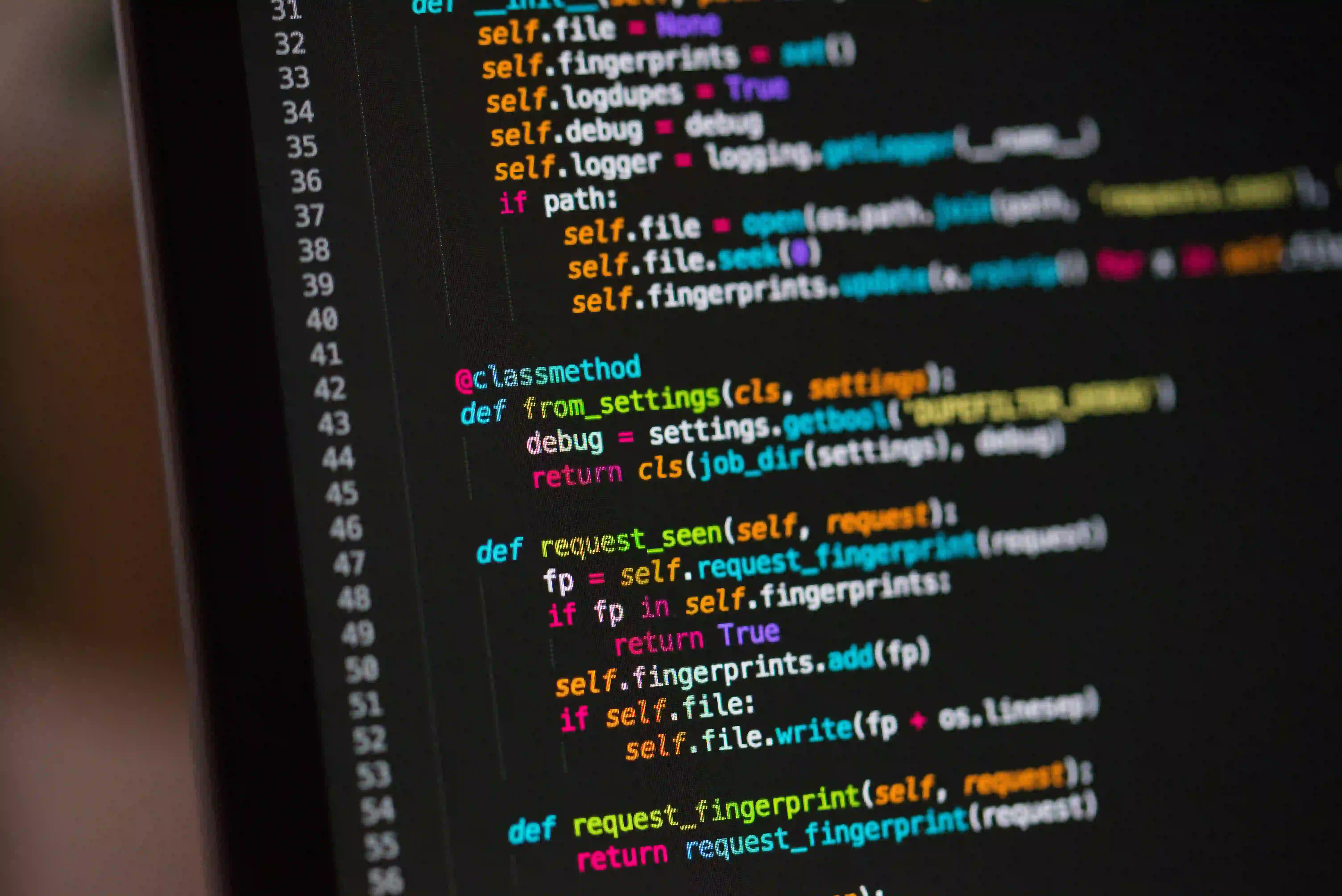
Why Poor Cohesion Harms Your Software Design
In the world of software development, clean architecture and efficient design are crucial for maintainability, scalability, and readability. One of the most vital principles to understand in software design is cohesion. This article will explore what cohesion is, the impact of poor cohesion on your software design, and provide practical coding examples, reinforcing the concept's significance.
What Is Cohesion?
Cohesion refers to how closely related and focused the responsibilities of a single module, class, or component are. Higher cohesion means that the component does one thing well, while lower cohesion indicates that it blends multiple, potentially unrelated responsibilities together.
In an ideal scenario, you want your classes and modules to possess high cohesion. This leads to systems that are easier to manage and understand.
Types of Cohesion
Consider the types of cohesion present when designing software:
- Functional Cohesion: The best type, where all elements contribute to a single, well-defined task.
- Sequential Cohesion: When one part relies on the output of another.
- Communicational Cohesion: Elements that operate on the same data set but do different things.
- Procedural Cohesion: Different actions that are part of a single procedure but may not relate closely.
- Temporal Cohesion: Elements grouped by their timing rather than functional relevance.
- Logical Cohesion: Elements are grouped together because they operate on the same type of input (e.g., several functions that handle different types of data).
- Coincidental Cohesion: The worst form of cohesion, where elements are grouped arbitrarily without any meaningful relationship.
Among these categories, functional cohesion stands out as the most desirable form.
The Detriments of Poor Cohesion
Now that we understand what cohesion is, let’s look at how poor cohesion can harm software design.
1. Difficulty in Understanding the Code
When classes or modules have poor cohesion, understanding the code becomes a challenge. For example, if a module handles user authentication, payment processing, and logging all at once, it is tough to ascertain what the module is meant to do. This can lead to confusion and mistakes when making changes.
Example of Poor Cohesion:
public class Utility {
public void authenticateUser(String username, String password) {
// Authenticate user
}
public void processPayment(String paymentInfo) {
// Process payment
}
public void logUserActivity(String activity) {
// Log user activity
}
}
In this example, the Utility
class is responsible for user authentication, payment processing, and logging activities. This lack of cohesion makes it unclear what the class is supposed to represent or do.
2. Increased Complexity
Poor cohesion often results in complex systems that are difficult to maintain. When modules are tasked with unrelated responsibilities, any change to one feature could inadvertently affect another, leading to bugs and increasing the testing burden.
3. Reduced Reusability
Components designed without clear responsibilities cannot be reused efficiently. If a module has multiple unrelated tasks, developers may be hesitant to use it in other contexts due to the additional features it brings along, which may not be necessary for the intended purpose.
4. Challenges in Testing
High cohesion simplifies testing. You can easily test a class that has a single responsibility. Conversely, testing modules with poor cohesion requires comprehensive integration tests, which can be more time-consuming and error-prone.
Example of Testing Difficulty:
Considering the Utility
class, if you want to test user authentication, you'd have to deal with payment processing and logging functionalities as side effects, complicating test scenarios.
5. Harder Debugging
Debugging systems with multiple responsibilities is a nightmare. If an error occurs, tracing it back to the responsible function can be difficult. Each module becomes an unexplored territory, and developers may find themselves losing precious time trying to understand the root cause of a failure.
Enhancing Cohesion in Software Design
Understanding the drawbacks of poor cohesion allows us to appreciate the importance of designing cohesive modules or classes. Here are some strategies to enhance cohesion within your software design.
1. Single Responsibility Principle (SRP)
Promote the Single Responsibility Principle — every class should have one responsibility. You can then design classes that are highly focused and can be composed as needed.
Refactored Code Example:
public class UserAuthenticator {
public void authenticateUser(String username, String password) {
// Authenticate user
}
}
public class PaymentProcessor {
public void processPayment(String paymentInfo) {
// Process payment
}
}
public class ActivityLogger {
public void logUserActivity(String activity) {
// Log user activity
}
}
In the refactored code, we created separate classes for user authentication, payment processing, and logging activities, respectively. Each class is now focused, making your code easier to test, understand, and maintain.
2. Use Naming Conventions
Choosing expressive names for your classes and methods can also aid clarity and emphasize their responsibilities. Clear names reflect high cohesion and allow developers to intuitively grasp their purpose.
3. Frequent Refactoring
The codebase evolves over time. Monitor for any instances of poor cohesion and refactor where necessary. Regular refactoring helps to piece the components back to their responsible tasks and ensures higher cohesion.
4. Review and Feedback
Engage in code reviews consistently. Having multiple eyes on the code can provide diverse insights, making it easier to spot areas with low cohesion.
The Last Word
Cohesion is a cornerstone of effective software design. Poor cohesion leads to complexity, confusion, and maintenance nightmares. By aiming for high cohesion in your classes and modules, you create systems that are easier to understand, maintain, and scale.
If you are seeking to dive deeper into the principles of software design, consider exploring Martin Fowler’s Refactoring and Domain-Driven Design textbooks, as they offer comprehensive insights into designing better software architectures.
Ultimately, software development is an art steeped in principles that guide developers toward cleaner, more efficient systems. By embracing high cohesion, you're investing in the long-term health of your projects and your productivity. Embrace cohesion, and revolutionize your coding practices today!