Common Bootstrap CDI 2.0 Issues in Java SE Development
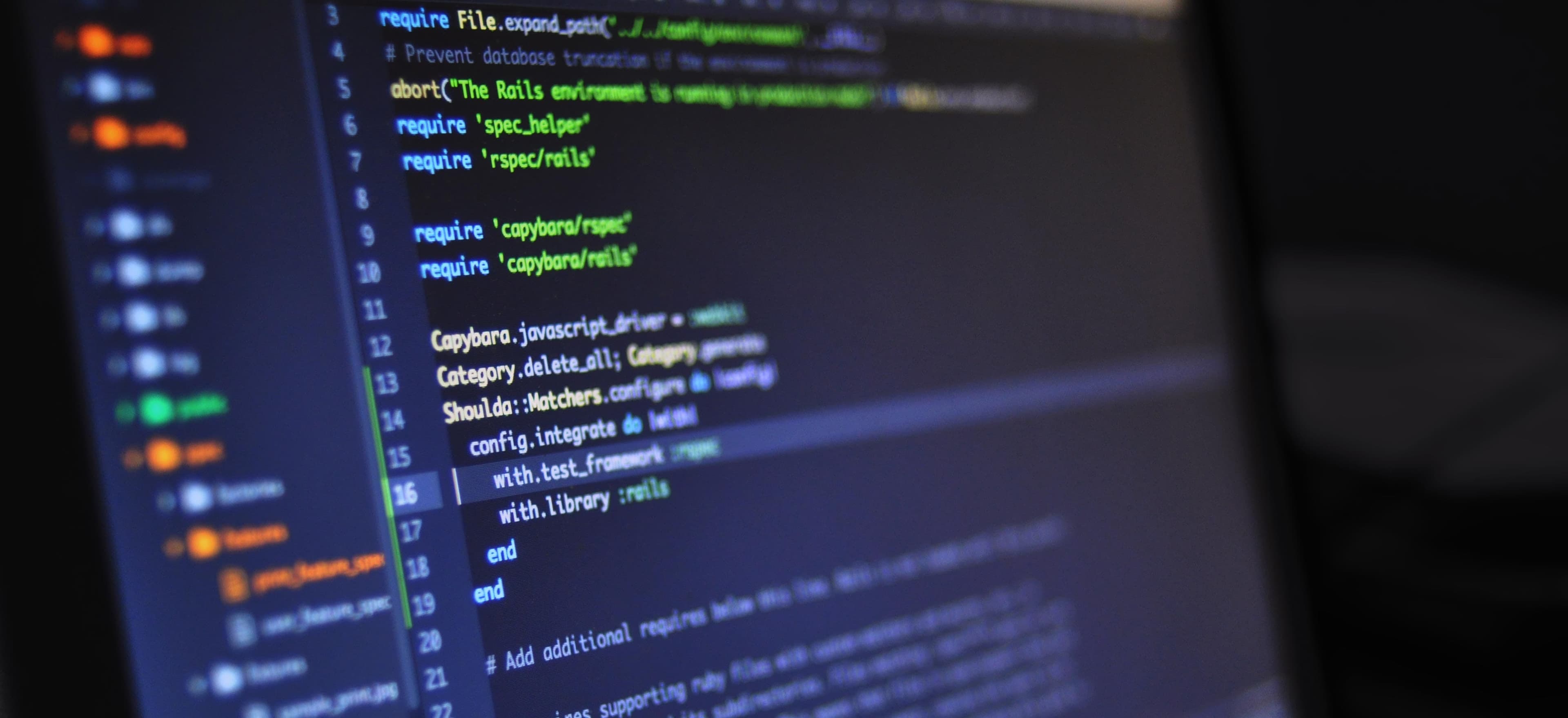
- Published on
Common Bootstrap CDI 2.0 Issues in Java SE Development
In the rapidly evolving landscape of Java development, Context and Dependency Injection (CDI) has carved its niche as a powerful framework that enhances the way components interact. JDBC 2.0, the updated version, now provides even more flexibility and capabilities for managing dependencies in a Java SE environment. However, with this power comes potential pitfalls. In this blog post, we'll explore some common issues developers encounter when bootstrapping CDI 2.0 in Java SE development, accompanied by solutions and best practices to ensure a seamless experience.
Understanding CDI 2.0 and Its Benefits
Before diving into common issues, let’s briefly cover what CDI 2.0 brings to the table:
- Context Management: CDI allows you to manage the lifecycle and visibility of beans, which can simplify business logic.
- Type-safe Dependency Injection: With CDI, you ensure that your components receive the correct implementations without the need for manual instantiation.
- Event Handling: CDI facilitates a robust event system, allowing decoupled components to communicate through events.
Now that we have a grasp of CDI 2.0's significant advantages, let’s delve into potential issues developers face.
Common Bootstrapping Issues in CDI 2.0
1. Class Not Found Exception
One of the most common errors during the bootstrap process is the "Class Not Found Exception." This usually occurs because the CDI implementation cannot locate the necessary configuration files or classes.
Solution
Ensure that you include all required dependencies in your project. A beans.xml
file is mandatory for CDI to work correctly. This file typically resides in the META-INF/
directory for JAR files and WEB-INF/
for WAR files.
<!-- beans.xml -->
<beans xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/beans_2_0.xsd"
version="2.0" bean-discovery-mode="all">
</beans>
Why? The beans.xml
file informs the CDI container about the beans it can manage.
2. No Bean Found for Injection Point
Another common issue is when CDI cannot find a suitable bean for injection. This usually results in an exception stating that no beans are available for injection.
Solution
Verify that the bean is annotated correctly and that it matches the type expected by the injection point. Ensure that the @Inject
annotation is placed on the appropriate fields or constructor.
import javax.inject.Inject;
public class OrderService {
@Inject
private PaymentService paymentService; // Ensure PaymentService is a managed bean.
public void processOrder(Order order) {
paymentService.processPayment(order);
}
}
Why? Only beans that are recognized by CDI can be injected, so proper annotations are crucial.
3. Ambiguous Dependencies
Sometimes, you may have multiple beans of the same type, leading to ambiguity. CDI does not know which bean to use, throwing an AmbiguousResolutionException
.
Solution
Use the @Qualifier
annotation to differentiate between the beans. Create custom qualifiers to mark each bean distinctly.
import javax.enterprise.util.AnnotationLiteral;
@Qualifier
@Retention(RUNTIME)
@Target({TYPE, METHOD, FIELD, PARAMETER})
public @interface CreditCardPayment {}
@CreditCardPayment
public class CreditCardPaymentService implements PaymentService {
// Implementation
}
Why? Custom qualifiers help CDI make intentional decisions about which bean to inject, eliminating ambiguity.
4. Lifecycle Issues
Understanding the lifecycle of CDI beans is crucial. If beans are not managed correctly, you could face lifecycle-related issues, such as a bean being collected by the garbage collector prematurely.
Solution
Use appropriate scope annotations such as @ApplicationScoped
, @SessionScoped
, or @RequestScoped
.
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class AppConfig {
// Configuration settings for the application.
}
Why? Properly defining the scope ensures that your beans live as long as necessary and are cleaned up when they're no longer needed.
5. Context Not Activated
Sometimes, you may face issues where the CDI context is not activated, particularly in Java SE environments without a web context.
Solution
Initialize the CDI in your main application using the CDI
class provided by the implementation.
import org.jboss.weld.environment.se.Weld;
import org.jboss.weld.environment.se.WeldContainer;
public class Main {
public static void main(String[] args) {
Weld weld = new Weld();
WeldContainer container = weld.initialize();
MyService myService = container.select(MyService.class).get();
myService.performAction();
weld.shutdown();
}
}
Why? Initializing CDI in a Java SE environment is crucial for making the CDI container aware of your application context.
6. Incompatibility with Older Libraries
When using CDI 2.0, older libraries that were not designed for dependency injection may lead to conflicts or unexpected behavior.
Solution
Keep your libraries up to date and ensure compatibility with CDI 2.0. If encountering issues, consider wrapping third-party classes with CDI managed beans when possible.
public class WrapperService {
private final LegacyService legacyService;
public WrapperService() {
this.legacyService = new LegacyService();
}
public void someOperation() {
legacyService.legacyMethod();
}
}
Why? Wrapping incompatible classes allows you to still use them while benefiting from CDI's capabilities.
Best Practices for Successful Implementation of CDI 2.0
-
Use Proper Annotations: Make sure that you annotate your classes correctly with CDI annotations such as
@Inject
,@Produces
, and@Qualifier
. -
Clear Configuration: Ensure your
beans.xml
file is correctly configured and comprehensively placed in your project structure. -
Define Qualifiers: When dealing with multiple implementations of the same interface, use qualifiers to avoid ambiguity.
-
Scope Your Beans Properly: Carefully select scopes based on how long you need the beans to live throughout their lifecycle.
-
Test Regularly: Run integration tests to ensure that CDI is bootstrapping as expected, and components are interacting correctly.
-
Seek Documentation and Community Resources: Leverage documentation and community forums for additional insights into CDI usage and troubleshooting.
In Conclusion, Here is What Matters
Bootstrapping CDI 2.0 in Java SE development can be a rewarding yet challenging experience. By understanding common issues and their solutions, you can leverage the full potential of CDI's capabilities to build robust and maintainable applications. Remember that practicing good design principles and using the correct annotations will significantly reduce issues and enhance your development workflow.
For further reading, check out the official CDI documentation and related resources. Happy coding!