Runtime vs Compile-Time Classpath: Common Pitfalls Explained
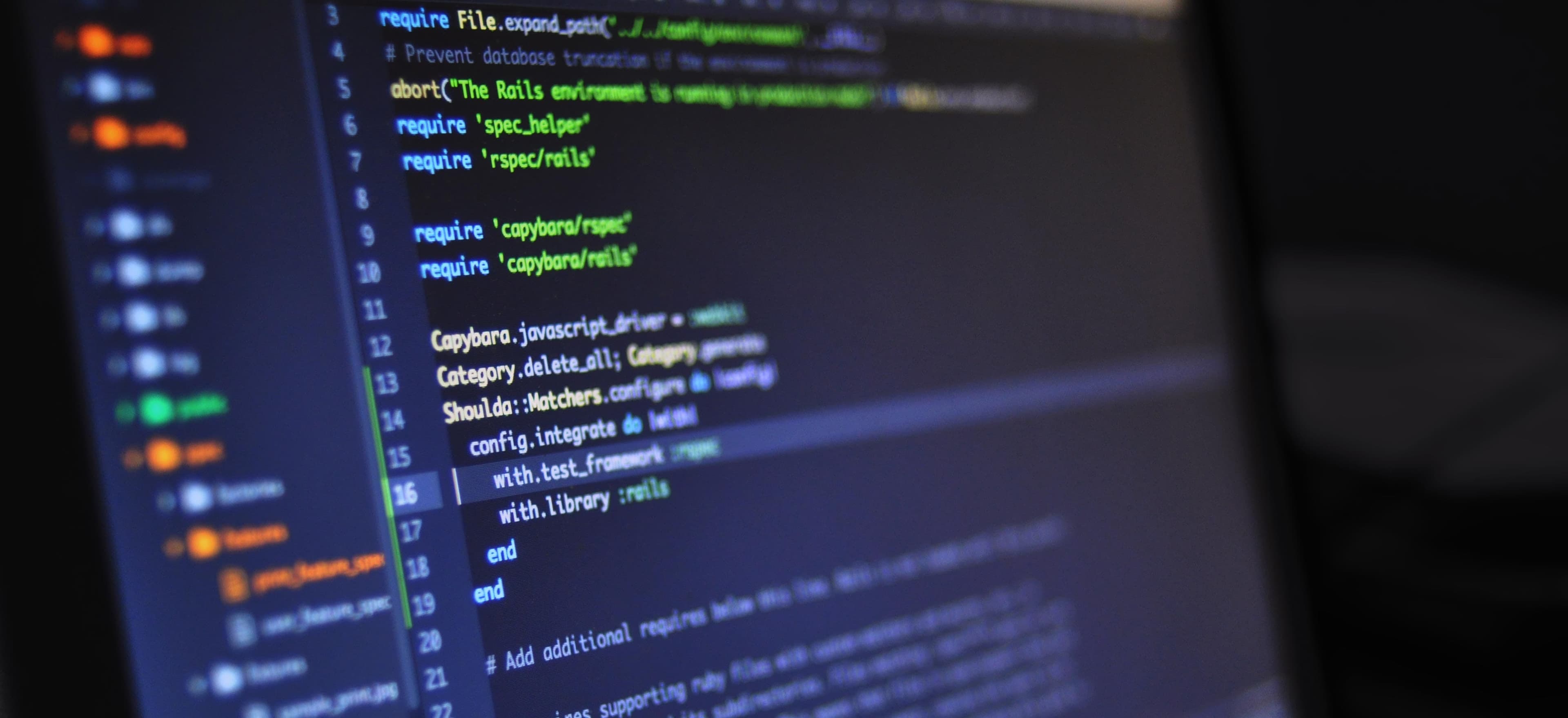
- Published on
Runtime vs Compile-Time Classpath: Common Pitfalls Explained
When developing Java applications, understanding the classpath is crucial for effective project management. The classpath tells the Java Virtual Machine (JVM) and Java compiler where to look for user-defined classes and packages when running a program. However, there are notable differences between the runtime classpath and the compile-time classpath. Below, we explore these two concepts, common pitfalls developers face, and best practices to avoid these issues.
What is Classpath?
The classpath in Java consists of a list of locations, including directories and JAR files, that the JVM uses to locate classes and resources. Essentially, it acts as a bridge between your application and the libraries it depends on.
Compile-Time Classpath
The compile-time classpath contains all the files and libraries required to compile your Java code. It consists of directories and JAR files that the Java compiler references when translating your source code into bytecode.
In this context, any missing dependencies will result in compilation errors. For instance, if a class that you've imported doesn’t exist in your compile-time classpath, you will see errors such as "cannot find symbol."
Runtime Classpath
The runtime classpath, on the other hand, consists of the libraries and other classes that your application requires while it is running. If the JVM cannot find required classes at runtime, you will encounter runtime exceptions such as ClassNotFoundException
or NoClassDefFoundError
.
The Differences: Key Points
- Purpose: The compile-time classpath is for compilation, while the runtime classpath is for execution.
- Errors: Mismatches in the compile-time classpath will throw compilation errors; mismatches in the runtime classpath will throw exceptions at runtime.
- Configuration: You can configure both paths differently in your project, allowing you to have separate dependencies for compiling and executing.
Common Pitfalls
Understanding the differences between runtime and compile-time classpaths is not sufficient by itself. Developers often encounter several pitfalls related to classpath management. Let's explore some of these common pitfalls and how to avoid them.
1. Mix-up of Classpath Entries
Many developers assume that the classpath is the same at compile-time and runtime, which leads to errors. For example, you may have a library that is present during compilation but is not included when you run your application.
Example Scenario:
You have the following code that uses a library for logging:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class App {
private static final Logger logger = LogManager.getLogger(App.class);
public static void main(String[] args) {
logger.info("Application started.");
}
}
During compilation, you may have the Log4j library in your IDE's libraries. However, if you forget to include it in your JAR or during deployment, it will result in a NoClassDefFoundError
at runtime.
Solution: Always ensure that any libraries you are using at compile-time are also included in the runtime classpath. Check your build configurations carefully (use a tool like Maven or Gradle for dependency management).
2. Version Mismatches
Using different versions of libraries for compile-time and runtime can lead to hard-to-debug issues. For instance, suppose you compile against a new version of a library that has additional methods or modified behavior, but you run the application against an older version.
Example Scenario:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.17.0</version>
</dependency>
If your application is compiled with this version but runs with an older version (e.g., 2.14.0), methods that exist in the newer library may throw NoSuchMethodError
.
Solution: Maintain consistent library versions across both compile-time and runtime classpaths. Use dependency management tools like Maven to enforce version control.
3. Impact of IDE Configurations
Different IDEs handle classpaths in unique ways. Often, developers rely on their IDE's setup but forget that what works locally might not work in production or different development environments.
Example: IntelliJ IDEA builds a separate runtime classpath, which might not be apparent to new developers.
Solution: After setting up dependencies in your IDE, verify that the classpath configurations align with your project's deployment procedures. Configuration files (like pom.xml
for Maven or build.gradle
for Gradle) should dictate your build management.
4. Unused Dependencies
Adding dependencies to the compile-time classpath without verifying their necessity can bloat your project and lead to conflicts between libraries.
Example Scenario:
You may have numerous SQL libraries referenced in pom.xml
:
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.200</version>
</dependency>
<dependency>
<groupId>org.xerial.sqlite-jdbc</groupId>
<artifactId>sqlite-jdbc</artifactId>
<version>3.36.0.3</version>
</dependency>
If you do not use these libraries, it can lead to conflicts during resolving dependencies, impacting file sizes and stability.
Solution: Perform regular code reviews and dependency audits to ensure you are only including what you need.
Best Practices
To avoid classpath-related pitfalls, follow these best practices:
- Use Dependency Management Tools: Tools like Maven or Gradle help automate the management of your classpath, making version control easier.
- Regular Audits: Perform regular audits of your dependencies to avoid redundancies and conflicts.
- Consistent Environment: Use the same environment for development, testing, and production. Containerization tools like Docker can be beneficial.
- Documentation: Clearly document which libraries are needed for compile-time and runtime. This will help onboard new developers.
Bringing It All Together
Navigating the complexities of runtime vs compile-time classpath can significantly improve the reliability and maintainability of your Java applications. By acknowledging the potential pitfalls and implementing best practices, you equip yourself and your team with the tools necessary to produce robust, error-free code.
For further reading on classpath management, you can refer to the Official Java Documentation or explore Maven's Dependency Mechanism.
By staying aware and proactive about your classpath configurations, you can avoid many common pitfalls and ensure that your Java projects run smoothly, both during development and in production. Happy coding!