Mastering Java Queues: Common Pitfalls and Solutions
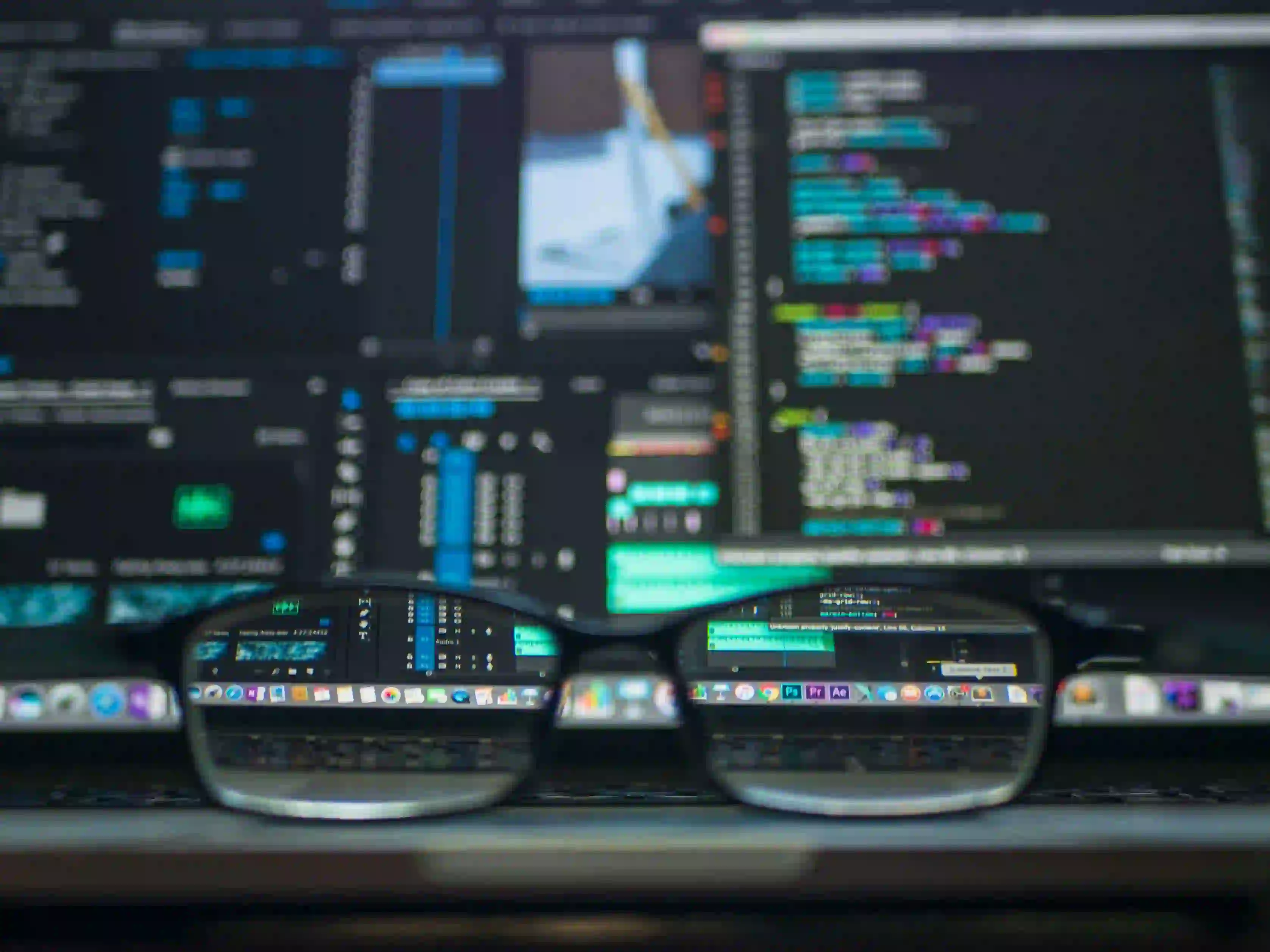
Mastering Java Queues: Common Pitfalls and Solutions
When we talk about data structures in Java, queues hold an important position. They help in managing data in a FIFO (First In, First Out) manner, making them essential for a variety of applications, from task scheduling to message processing. However, mastering Java queues involves understanding not just how to implement them, but also the common pitfalls developers encounter along the way.
In this blog post, we will explore Java queues, identify frequent mistakes, and discuss solutions. Along the way, we will include code snippets and dynamic examples to guide you through the concepts effectively.
Understanding Java Queues
In Java, queues come under the java.util
package and can be implemented using interfaces and classes that support manipulation of the queue structure. The primary interface we interact with is Queue
.
Basic Operations
A queue supports several basic operations, such as:
- Offer: Add an element to the queue.
- Poll: Removes and returns the head of the queue, returning null if the queue is empty.
- Peek: Retrieves, but does not remove, the head of the queue, returning null if the queue is empty.
Here's a simple example showcasing how to work with a queue in Java.
import java.util.LinkedList;
import java.util.Queue;
public class QueueExample {
public static void main(String[] args) {
Queue<String> queue = new LinkedList<>();
// Add elements to the queue
queue.offer("First");
queue.offer("Second");
queue.offer("Third");
// Use peek to view the head
System.out.println("Head of the queue: " + queue.peek());
// Poll elements from the queue
while (!queue.isEmpty()) {
System.out.println("Processing: " + queue.poll());
}
// Trying to poll from an empty queue
String emptyPoll = queue.poll();
System.out.println("Polled from empty queue returns: " + emptyPoll);
}
}
Explanation
In the above code:
- We create an instance of
LinkedList
, which implements theQueue
interface. - We add items using the
offer
method. - We use
peek
to look at the first element without removing it and thenpoll
to process elements one by one.
The output illustrates how our queue behaves, even as we attempt polling from an empty queue — which is a common scenario developers usually neglect.
Common Pitfalls When Using Queues
Despite being a straightforward data structure, several common pitfalls can stifle your Java queue usage. Let’s explore these pitfalls and their solutions.
1. Neglecting Concurrency Issues
When a queue is accessed by multiple threads, issues can arise if proper synchronization is not enforced. If two threads try to poll
from the same queue simultaneously, you might run into race conditions.
Solution: Using Thread-Safe Queues
Java provides several thread-safe queues in the java.util.concurrent
package. The ConcurrentLinkedQueue
is a popular choice.
import java.util.concurrent.ConcurrentLinkedQueue;
public class ThreadSafeQueueExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
// Thread to add elements
new Thread(() -> {
for (int i = 0; i < 5; i++) {
queue.offer("Task " + i);
System.out.println("Added: Task " + i);
}
}).start();
// Thread to poll elements
new Thread(() -> {
try {
Thread.sleep(500); // Slight delay to allow for adds
while (true) {
String task = queue.poll();
if (task != null) {
System.out.println("Processed: " + task);
} else {
break; // Break if empty
}
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
}
Explanation
The ConcurrentLinkedQueue
allows multiple threads to access it without running into issues, as it properly handles internal synchronization.
2. Memory Management and Resource Leaks
Improper handling of queues can lead to memory issues, especially in long-running applications where items are continuously added. If you fail to call poll or remove items when no longer needed, your program may use up memory unnecessarily.
Solution: Monitor Queue Size and Clear Old Data
You should keep an eye on the size of your queue and implement a strategy to clear old data.
public class MemoryManagementExample {
public static void main(String[] args) {
Queue<String> queue = new LinkedList<>();
// Simulating adding tasks to the queue
for (int i = 0; i < 10000; i++) {
queue.offer("Task " + i);
}
// Monitor and clear the queue to prevent memory issues
if (queue.size() > 5000) {
while (queue.size() > 1000) {
queue.poll(); // Remove old tasks
}
System.out.println("Cleared old tasks. Queue size is now: " + queue.size());
}
}
}
Explanation
In the above example, we check the size of the queue and clear it as needed to prevent out-of-memory issues.
3. Choosing the Wrong Queue Implementation
Java offers various implementations of the Queue
interface. Each comes with its own characteristics, performance implications, and use cases. Misunderstanding these can lead to inefficiencies.
Solution: Choose the Right Implementation
- LinkedList: Flexible size but slower access.
- ArrayDeque: Faster operations than LinkedList.
- PriorityQueue: For scenarios where elements have priority.
- BlockingQueue: Waits for items to become available (essential for producer-consumer scenarios).
Here's a simple illustration of using a PriorityQueue:
import java.util.PriorityQueue;
public class PriorityQueueExample {
public static void main(String[] args) {
PriorityQueue<Integer> priorityQueue = new PriorityQueue<>();
// Adding elements to PriorityQueue
priorityQueue.offer(5);
priorityQueue.offer(1);
priorityQueue.offer(3);
while (!priorityQueue.isEmpty()) {
System.out.println("Processing: " + priorityQueue.poll());
}
}
}
Explanation
In this case, the PriorityQueue
processes the smallest numbers first, beneficial in scenarios where priority matters over order.
Final Considerations
Queues are an essential structure in Java, providing immense flexibility for developers when managing data in a predictable manner. Understanding common pitfalls like concurrency issues, memory management, and queue choice can significantly enhance your development experience and lead to more efficient applications.
To deepen your understanding of Java collections, you may want to refer to the Java Collections Framework documentation and Learn more about Thread Safety in Java.
Whether you're building applications that require task management, scheduling, or simple data handling, mastering queues will prove indispensable.
Happy coding!