Mastering SSH Tunneling for Secure MySQL Connections in Java
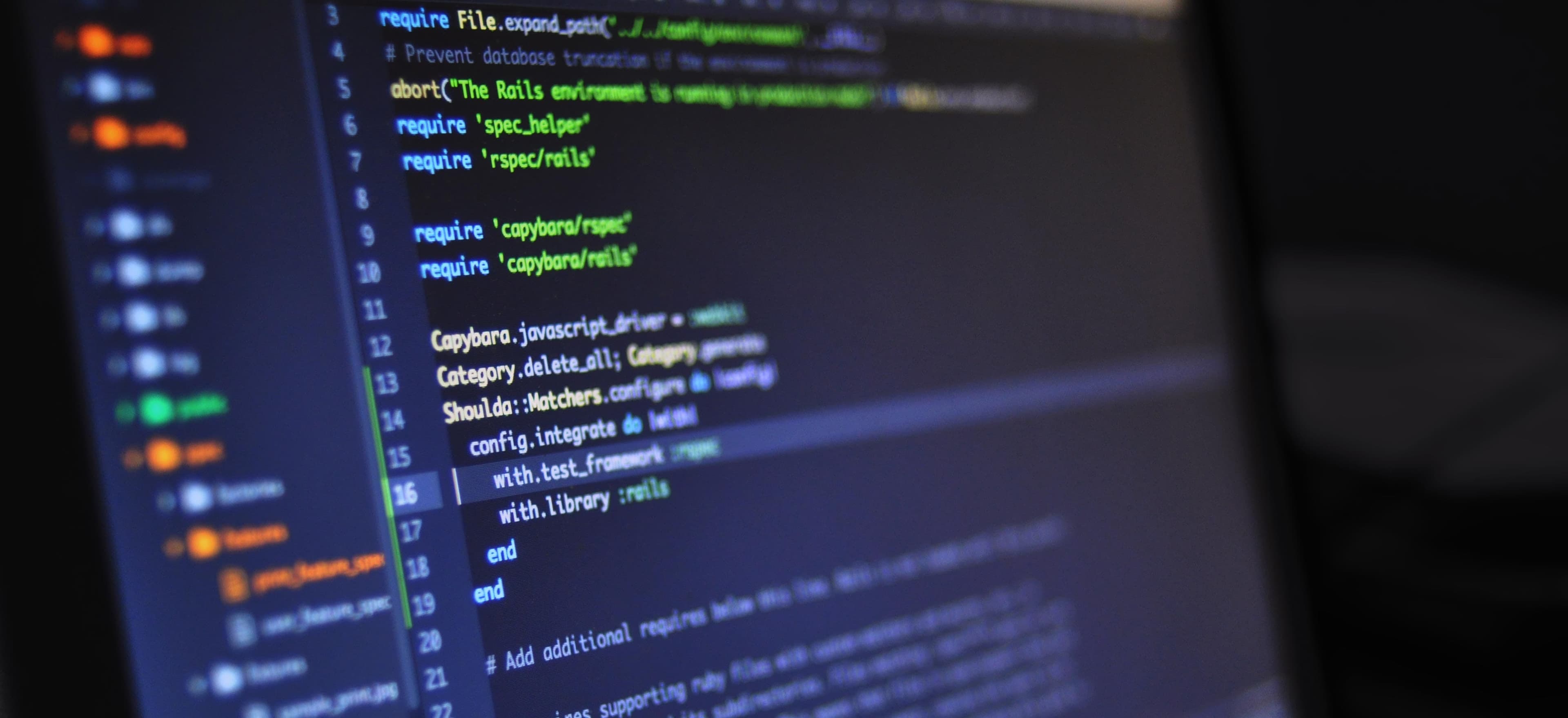
- Published on
Mastering SSH Tunneling for Secure MySQL Connections in Java
In today’s digital landscape, ensuring secure connections to databases is paramount. MySQL, one of the most popular database management systems, often requires secure access layers to protect sensitive information from prying eyes. SSH (Secure Shell) tunneling provides a robust solution, enabling you to secure MySQL connections effectively. In this blog post, we will delve into the mechanics of SSH tunneling, specifically for Java applications, and provide practical examples to help you master this technique.
What is SSH Tunneling?
SSH tunneling is a method of encapsulating network protocols within the Secure Shell (SSH) protocol. It allows users to create a secure connection between a client and a server, routing the database traffic through an encrypted tunnel. This is particularly useful when:
- The MySQL server is hosted in a remote location.
- Direct access to the MySQL server is restricted.
- You want to enhance security through anonymity.
Why Use SSH Tunneling for MySQL?
-
Encryption: SSH automatically encrypts all data exchanged between the client and server, providing a layer of security.
-
Access Control: SSH allows you to define which users can connect to the MySQL server, enhancing control over who accesses sensitive data.
-
Firewall Bypass: With SSH tunneling, you can bypass firewalls that may restrict direct access to MySQL ports.
-
Simplicity: Once configured, the process is relatively straightforward and doesn't require significant changes to the existing infrastructure.
Pre-requisites
- Java Development Kit (JDK): Make sure you have Java installed on your machine.
- MySQL Server: Set up your MySQL server.
- SSH Server: Have an SSH server where the MySQL database is hosted.
- JSch Library: This Java library allows you to communicate via SSH. You can include it in your Java application using Maven:
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
Setting Up SSH Tunneling
Let’s take a step-by-step approach to set up SSH tunneling for your MySQL connections in Java.
Step 1: Creating an SSH Tunnel
Use the JSch library to create an SSH tunnel. The following method establishes the SSH connection to the SSH server and creates a tunnel:
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SSHTunnel {
public static Session createSSHTunnel(String sshHost, int sshPort, String sshUser, String sshPassword,
String remoteHost, int remotePort, int localPort) throws Exception {
JSch jsch = new JSch();
Session session = jsch.getSession(sshUser, sshHost, sshPort);
// Set the SSH password and disable strict host key checking
session.setPassword(sshPassword);
session.setConfig("StrictHostKeyChecking", "no");
// Set up port forwarding
session.setPortForwardingL(localPort, remoteHost, remotePort);
// Connect to the SSH server
session.connect();
return session;
}
}
Why Use the Code This Way?
-
Security Configuration: We disable strict host key checking for development purposes. This should be handled more securely in production.
-
Port Forwarding: This line is crucial as it forwards a specified local port to the MySQL server.
Step 2: Connecting to MySQL Through the Tunnel
Once your SSH tunnel is established, you can connect to MySQL via JDBC. Here’s how:
public class MySQLConnection {
public static Connection getConnection(String localHost, int localPort, String dbUser, String dbPassword, String dbName) throws SQLException {
String url = String.format("jdbc:mysql://%s:%d/%s", localHost, localPort, dbName);
return DriverManager.getConnection(url, dbUser, dbPassword);
}
}
Explanation of the MySQL Code
-
JDBC URL: The JDBC connection string uses the local port allocated during the SSH tunneling.
-
DriverManager: This manages the database connection using the JDBC URL, username, and password.
Step 3: Putting It All Together
Now that we have both the SSH connection and MySQL connection methods, let’s create the driver code to use them:
public class DatabaseAccess {
public static void main(String[] args) {
String sshHost = "ssh.example.com";
String sshUser = "sshUser";
String sshPassword = "sshPassword";
String remoteHost = "127.0.0.1";
int remotePort = 3306; // MySQL default port
int localPort = 3307; // Local port for SSH tunnel
try {
Session sshSession = SSHTunnel.createSSHTunnel(sshHost, 22, sshUser, sshPassword, remoteHost, remotePort, localPort);
System.out.println("SSH Tunnel established!");
// Connect to MySQL
Connection connection = MySQLConnection.getConnection("127.0.0.1", localPort, "mysqlUser", "mysqlPassword", "myDatabase");
System.out.println("MySQL Connection established!");
// Use the connection for your queries...
// Example query can be performed here.
// Close connections
connection.close();
sshSession.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Putting It All Together
Here, we combine the previous functionalities to create a seamless flow, from establishing the SSH tunnel to connecting to the MySQL database.
The Closing Argument
SSH tunneling is an effective method for securing your MySQL connections in Java. By encapsulating your database traffic in an encrypted tunnel, you enhance your application's security posture significantly. This blog post has provided an overview of how to set up SSH tunneling and connect to MySQL using Java.
If you want to dive deeper into JDBC or explore more about encryption technologies, you can check out Oracle’s JDBC documentation and OpenSSH documentation.
Make sure to adapt the configurations concerning your infrastructure and security needs, especially in a production setup. Happy coding, and may your connections always be secure!
Checkout our other articles