Mastering JDK 11: Common Pitfalls in File String Handling
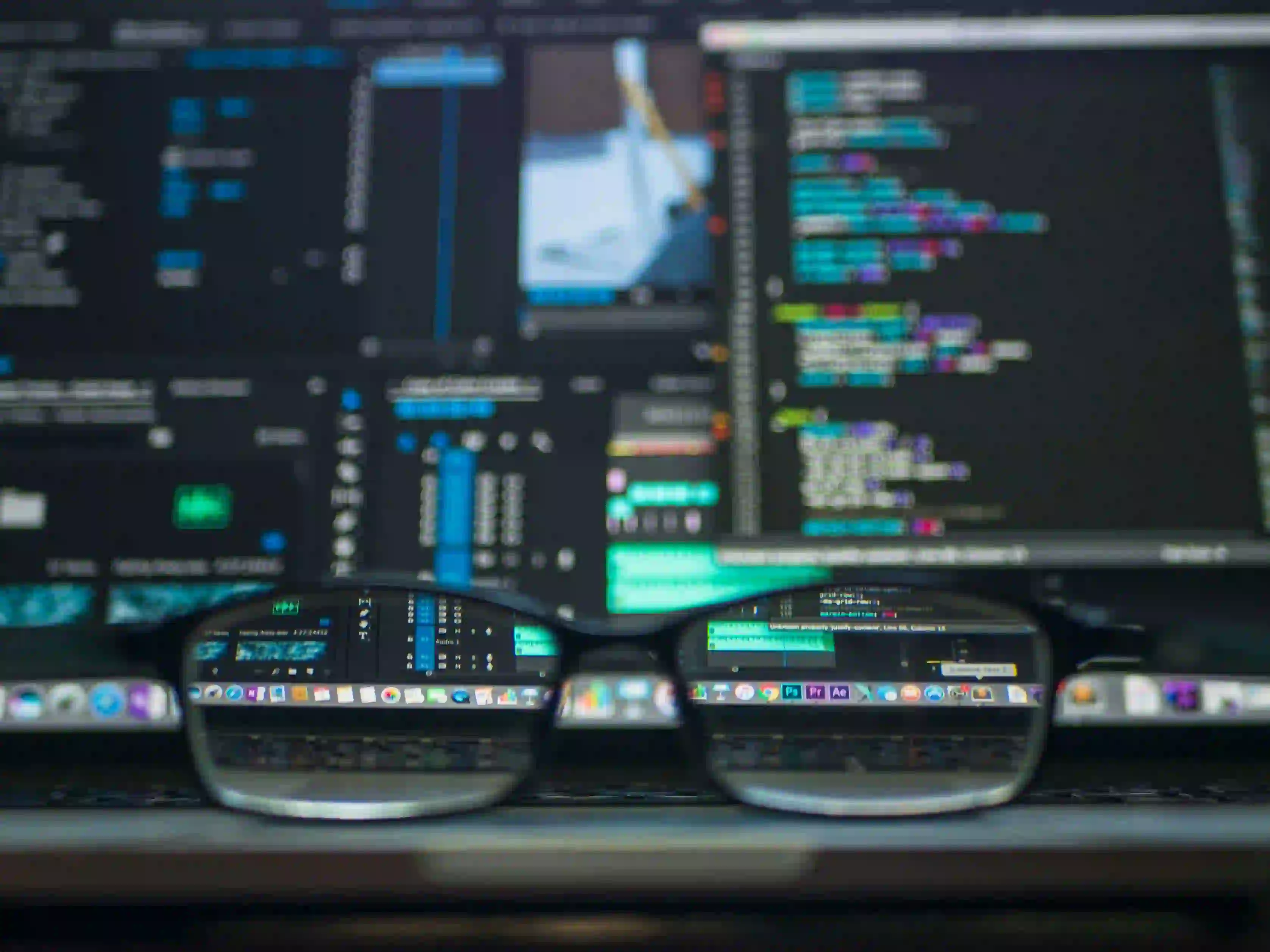
Mastering JDK 11: Common Pitfalls in File String Handling
The Java Development Kit (JDK) 11 introduced numerous enhancements and clarifications, particularly in file handling. Although Java provides several classes for managing file input and output, developers can face challenges when manipulating strings from files. This blog post will explore common pitfalls in file string handling while using JDK 11, providing solutions and best practices to master this essential aspect of Java programming.
Table of Contents
- Understanding File Handling in JDK 11
- Common Pitfalls in String Handling
- Pitfall 1: Ignoring Character Encodings
- Pitfall 2: Failing to Close Resources
- Pitfall 3: Mismanaging Whitespace
- Proper Techniques for File String Handling
- Conclusion and Additional Resources
Understanding File Handling in JDK 11
Java provides a rich set of APIs for file manipulation, primarily housed in the java.nio.file
package. This package enhances the capabilities laid out in earlier versions, such as the ability to read and write strings directly. Here is a simple example to get us started.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileReaderExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
String content = Files.readString(path);
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, Files.readString(Path)
is an elegant way to get the contents of "example.txt". However, it's essential to understand the nuances involved in dealing with strings read from files.
Common Pitfalls in String Handling
Pitfall 1: Ignoring Character Encodings
When reading files, failing to consider character encoding can lead to unreadable strings or unexpected characters. For instance, if you read a UTF-8 encoded file using a different encoding, the output may be corrupted.
How to Avoid This
Always specify the character encoding when reading or writing files. You can leverage the Files.newBufferedReader(Path, Charset)
method, which allows for more control over the character encoding.
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileReaderWithEncoding {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try (BufferedReader reader = Files.newBufferedReader(path, StandardCharsets.UTF_8)) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here, we specify StandardCharsets.UTF_8
to ensure that the file is read correctly. Ignoring encoding can be a subtle bug that is often overlooked.
Pitfall 2: Failing to Close Resources
Java 11 has introduced the try-with-resources
statement to simplify resource management, but many developers still forget to close their resources properly. Not closing resources such as file readers can lead to memory leaks or file locks.
How to Avoid This
Utilize the try-with-resources
feature for automatic resource management.
import java.nio.file.Files;
import java.nio.file.Path;
import java.io.IOException;
public class FileWritingWithResources {
public static void main(String[] args) {
Path path = Paths.get("output.txt");
String content = "Hello, World!";
try {
Files.writeString(path, content);
} catch (IOException e) {
e.printStackTrace();
}
// No need to manually close as try-with-resources handles it.
}
}
The above example ensures resources are correctly handled without needing explicit closing logic. Embracing this pattern can save you from many headaches in your file handling operations.
Pitfall 3: Mismanaging Whitespace
Whitespace can often behave unexpectedly in string manipulation. For example, when reading lines from a file, it's easy to overlook leading or trailing spaces, affecting further processing.
How to Avoid This
Utilize .trim()
and .replaceAll()
to handle whitespace when processing strings.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class WhitespaceManagement {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
Files.lines(path)
.map(String::trim) // Trim whitespace
.forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
This way, you ensure that leading and trailing spaces are removed, making your string processing cleaner and more predictable.
Proper Techniques for File String Handling
After identifying common pitfalls, let's discuss best practices in file string handling.
-
Use High-Level APIs: JDK 11 offers cleaner and more concise methods. Always prefer high-level methods whenever possible for better readability.
-
Always Handle Exceptions: File I/O operations are prone to errors. Ensure that you catch and handle exceptions properly to prevent your program from crashing unexpectedly.
☕snippet.javatry { // File operations } catch (IOException e) { // Handle exception }
-
Use Immutable Strings: String in Java is immutable. This property prevents unintentional changes to string content, making your code more predictable.
-
Stream for Processing: Use Java Streams API for resource-efficient operations on files. It allows for processing elements in a functional style.
☕snippet.javaFiles.lines(path) .filter(line -> !line.isEmpty()) // Process based on your criteria .forEach(System.out::println);
-
Document Your Code: Code can become convoluted when working with file I/O. Make sure to comment on the logic clearly so that it’s easier for others (and yourself) to understand.
Final Considerations
Mastering file string handling in Java, especially with the enhancements brought by JDK 11, will significantly impact your software development and overall code quality. By understanding and avoiding common pitfalls such as encoding issues, resource management, and whitespace mishaps, you can elevate your Java skills.
To further your knowledge, consider checking out the Java Official Documentation, which provides comprehensive resources. Additionally, frameworks like Apache Commons IO can simplify file manipulation tasks if you find yourself needing advanced features.
Keep your code clean, efficient, and always open to improvement. Happy coding!