Unlocking the Mystery: What Mutation Testing Really Is
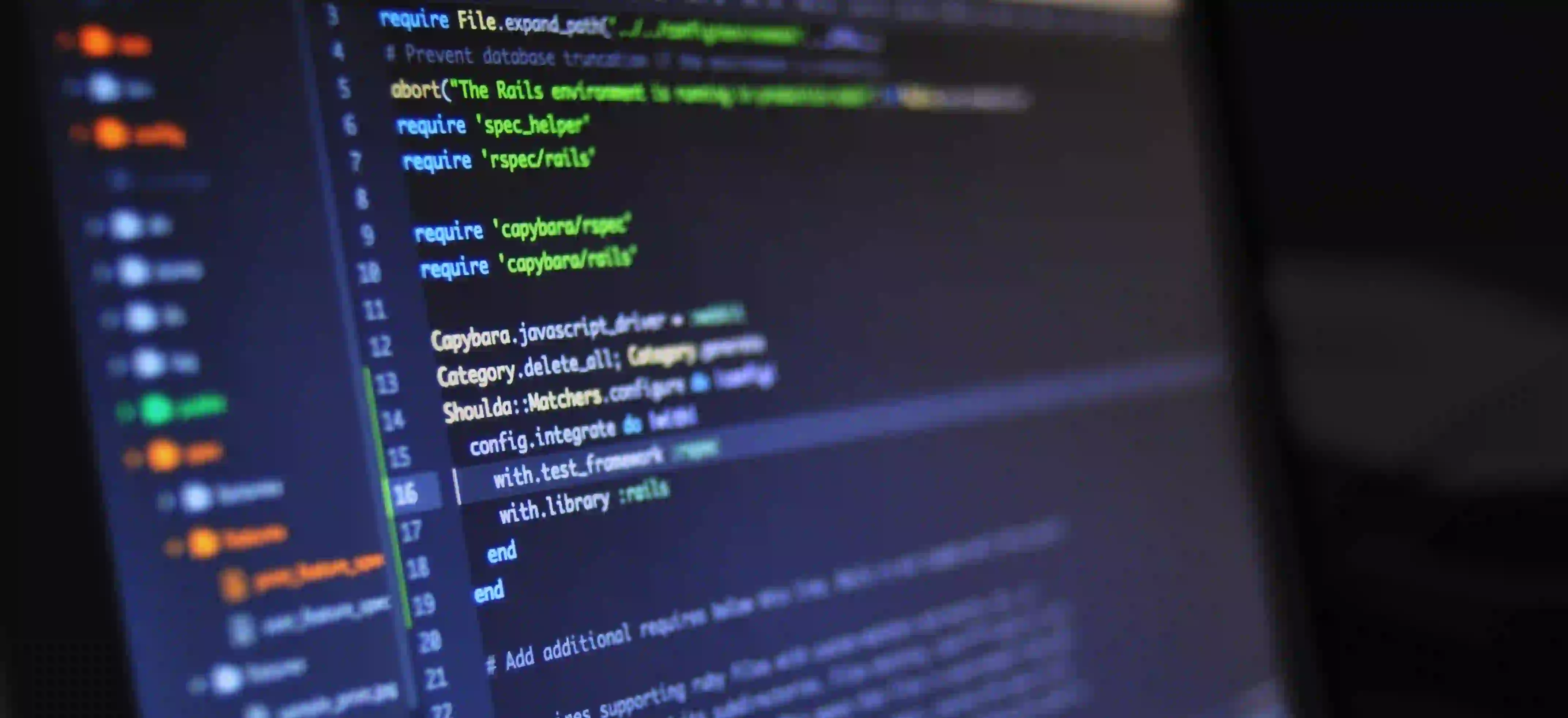
Unlocking the Mystery: What Mutation Testing Really Is
Mutation Testing is an advanced technique used to evaluate the quality of your test cases by making deliberate changes, or mutations, to your code. These changes help identify weaknesses in your tests. In a world where software reliability and robustness are paramount, mutation testing stands as a beacon for developers aiming for high-quality code.
What is Mutation Testing?
Mutation Testing involves modifying your program's source code in small ways to create a set of "mutants." Each mutant is checked to see if existing test cases can detect the changes. If a test case fails to detect a mutant, it indicates that the test suite may be inadequately designed. This systematic approach not only exposes weaknesses but also reinforces the importance of thorough testing.
The Purpose of Mutation Testing
The primary goal is to enhance your test suite's effectiveness. Here are a few reasons why you should consider incorporating mutation testing into your workflow:
- Test Suite Validation: It validates that your tests adequately cover various paths and scenarios in your code.
- Error Detection: It helps detect faults in the program that are not covered by current tests.
- Improved Code Quality: Continuous use of mutation testing leads to better-designed tests and higher quality code.
How Does Mutation Testing Work?
The process of mutation testing can be summarized in the following steps:
- Generate Mutants: Automatically or manually change certain code elements.
- Run Test Cases: Execute your existing test suite against the mutants.
- Analyze Results: Review the outcomes to determine which tests failed and which passed.
Let’s delve a bit deeper into each step along with code snippets for a clearer understanding.
Step 1: Generate Mutants
Generating mutants typically involves modifying certain elements of the code. Common mutation operators include:
- Arithmetic Operators: Change addition to subtraction.
- Relational Operators: Change equality checks (==) to inequalities (!=).
- Conditional Statements: Invert if-else conditions.
Here’s a simple Java code snippet demonstrating a possible mutant.
public int add(int a, int b) {
return a + b; // Original code
}
A mutant could change the addition operator to subtraction:
public int add(int a, int b) {
return a - b; // Mutant code
}
Step 2: Run Test Cases
Once the mutants are created, run your test suite against each mutant. This will help you identify if existing test cases are capable of catching these small intentional faults.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3)); // This should catch the mutant
}
}
Step 3: Analyze Results
After running the test cases, you will analyze which mutants were detected by the existing tests. If a mutant survives (the test passes), it indicates that the mutant was not covered by any tests, revealing a gap in your test coverage.
Example of Analysis
- Mutant Detected: If running the mutant that subtracts instead of adds causes the test to fail, then your test suite can be considered effective for that scenario.
- Mutant Survived: If the test suite still passes despite the mutation, the test needs to be improved or a new test needs to be added.
Benefits of Mutation Testing
Increased Confidence in Your Tests
Using mutation testing allows you to build confidence in your test suite. When tests are able to "kill" the majority of mutants, you can feel secure that your test cases cover a significant portion of potential errors in your code.
Finding Edge Cases
Mutation testing can expose edge cases that may not be initially obvious. Through the creation of mutants, developers can see how their code behaves under various scenarios. This perspective can lead to better boundary conditions and test cases.
Better Refactoring
Refactoring code while maintaining functionality can be tricky. Mutation testing helps by ensuring that after refactoring, your test suite still catches any errors that could arise from the changes.
Limitations of Mutation Testing
While mutation testing holds numerous advantages, it also comes with its own set of limitations, which should be considered:
- Performance Overhead: Running tests against multiple mutants can be time-consuming and resource-intensive.
- Complexity: Generating meaningful mutants that accurately reflect realistic faults can be complex.
- False Positives: In some cases, tests that appear to be failing due to mutants may actually be indicating genuine flaws in the code.
Tools for Mutation Testing
Several tools can aid in implementing mutation testing for Java projects. Here are a few popular ones:
- PIT: A powerful mutation testing tool specifically designed for Java.
- MUTPY: Python-based, but can offer insights into mutation testing principles that can be applied in Java.
These tools can automate the generation and evaluation of mutants, simplifying the overall process.
Example Pit with Java
Here’s a brief guide on using PIT with a sample JUnit test.
- Add PIT to your project dependencies (Maven):
<dependency>
<groupId>org.pitest</groupId>
<artifactId>pitest</artifactId>
<version>1.7.4</version>
</dependency>
- Run PIT:
You can integrate PIT into your CI/CD pipeline or run it directly from the command line to analyze your project's test coverage.
mvn org.pitest:pitest-maven:mutationCoverage
The results will help you identify how many mutants were killed vs. how many survived, offering a clear picture of your test suite's effectiveness.
The Bottom Line
Mutation testing is a powerful technique that enables developers to assess the effectiveness of their test suites by uncovering vulnerabilities that may otherwise go unnoticed. Despite its complexities and performance overhead, it offers invaluable insights into the health of your code and the quality of your tests.
By incorporating mutation testing into your development process, you are set to improve code quality, confidence in your tests, and the overall reliability of your application. As you embark on this journey, consider using tools like PIT to streamline the process, allowing you to focus more on writing robust test cases and less on the mechanics of mutation generation.
For further reading on software testing techniques, check resources such as Martin Fowler’s Refactoring and A Testing Guide to Software Testing.
Happy Coding!