Common Pitfalls When Integrating LangChain4j with Spring Boot
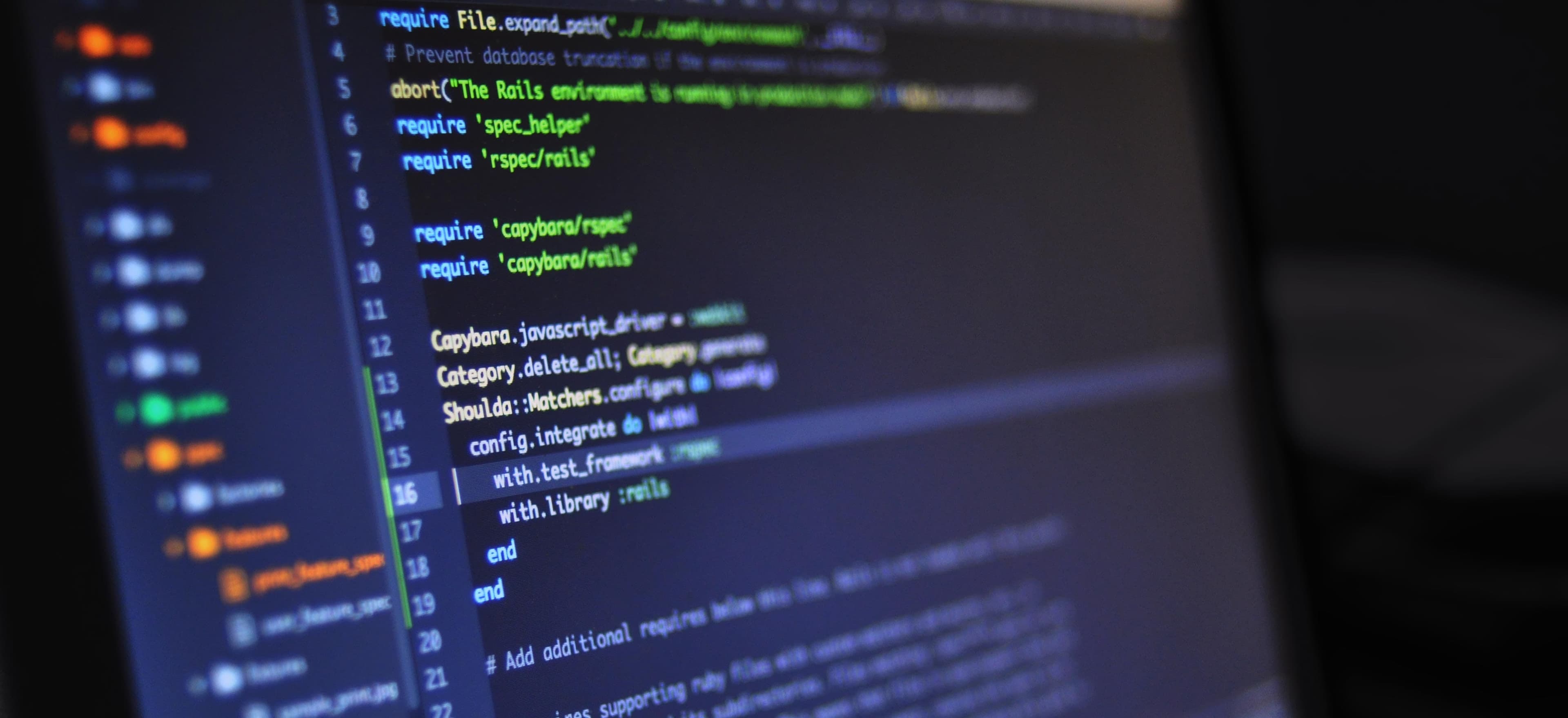
- Published on
Common Pitfalls When Integrating LangChain4j with Spring Boot
Integrating LangChain4j, a powerful framework for building applications with language models, into a Spring Boot application can unlock immense potential. However, it can also introduce challenges that developers must navigate carefully. In this blog post, we will explore common pitfalls when integrating LangChain4j with Spring Boot and provide solutions and recommendations to overcome these issues.
Table of Contents
- Understanding LangChain4j and Spring Boot
- Pitfall 1: Dependency Management
- Pitfall 2: Configuring Beans
- Pitfall 3: Language Model Initialization
- Pitfall 4: Error Handling
- Pitfall 5: Testing
- Conclusion
Understanding LangChain4j and Spring Boot
LangChain4j serves as an interface for interacting with different language models, simplifying processes like tokenization, text generation, and handling large datasets. On the other hand, Spring Boot streamlines the Java-based challenge of building applications. Together, they promise to enhance your application's capabilities with intelligent language processing features.
Key Link
For more information on [Spring Boot], check out the official documentation.
Pitfall 1: Dependency Management
One of the first steps in integration is correctly setting up Maven or Gradle dependencies. Forgetting to add the necessary dependencies can lead to runtime errors that can be quite frustrating.
Solution
Ensure you have all required dependencies in your pom.xml
or build.gradle
. Here's how to do that:
Maven (pom.xml)
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>com.langchain4j</groupId>
<artifactId>langchain4j-core</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Gradle (build.gradle)
dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
implementation 'com.langchain4j:langchain4j-core:1.0.0'
}
Explanation
Including the LangChain4j dependency ensures you have access to its classes, interfaces, and functions, whereas Spring Boot Starter provides the necessary infrastructure for your application.
Pitfall 2: Configuring Beans
Another common issue arises when configuring LangChain4j beans within your Spring Boot app. Developers sometimes neglect the Spring context, which can lead to NoSuchBeanDefinitionException
.
Solution
Use @Configuration
to define your LangChain4j beans correctly. Here’s an example of how to set up a configuration class:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.langchain4j.LangChain;
@Configuration
public class LangChainConfig {
@Bean
public LangChain langChain() {
return new LangChain(); // Configure any specific settings here
}
}
Explanation
The @Configuration
annotation informs Spring to treat this class as a source of bean definitions. This will help manage the lifecycle of your LangChain object, allowing it to be injected where needed.
Pitfall 3: Language Model Initialization
Initializing your language model isn’t just about creating an instance; it’s crucial to understand the implications of different configurations. Users frequently encounter issues with model parameters or missing initialization data.
Solution
Make sure to initialize your model correctly according to its requirements. Here’s a brief example:
import com.langchain4j.models.YourModel;
@Service
public class LangChainService {
private final YourModel model;
public LangChainService(LangChain langChain) {
this.model = langChain.initModel("path/to/your/model");
}
public String generateText(String prompt) {
return model.generate(prompt);
}
}
Explanation
Initializing the language model using the initModel
method sets up the model with necessary parameters. Ensure the path to your model is correct, or you will encounter FileNotFoundExceptions or similar issues.
Pitfall 4: Error Handling
Error management can often be overlooked, leading to unhandled exceptions that disrupt the application flow. Neglecting this can result in a poor user experience.
Solution
Implement comprehensive exception handling around your language model operations. Here is how you can do it:
import org.springframework.stereotype.Service;
@Service
public class LangChainService {
// previously defined code...
public String safeGenerateText(String prompt) {
try {
return generateText(prompt);
} catch (Exception e) {
// Log exception and provide fallback/friendly message
System.err.println("Error generating text: " + e.getMessage());
return "An error occurred while generating text.";
}
}
}
Explanation
Using a try-catch block allows you to manage exceptions gracefully. It ensures that your application can recover from unexpected failures and continue to operate smoothly.
Pitfall 5: Testing
Testing is a crucial part of software development, yet it is often incomplete when integrating libraries. Without proper testing, you may unknowingly introduce bugs into your application.
Solution
Utilize unit tests to verify your LangChain4j integration. Here’s a simple example:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
public class LangChainServiceTest {
@InjectMocks
private LangChainService langChainService;
@Mock
private YourModel mockModel;
@BeforeEach
public void init() {
MockitoAnnotations.openMocks(this);
}
@Test
public void testGenerateText() {
String prompt = "Hello World!";
String expectedOutput = "Generated text";
when(mockModel.generate(prompt)).thenReturn(expectedOutput);
String actualOutput = langChainService.generateText(prompt);
assertEquals(expectedOutput, actualOutput);
}
}
Explanation
This unit test uses Mockito to create a mock of the YourModel interface, enabling you to isolate the LangChainService for testing. You can verify the behavior of your application without needing the actual model, streamlining your testing process.
Closing Remarks
Integrating LangChain4j into a Spring Boot application can be an enriching experience, provided you are mindful of common pitfalls. By carefully managing dependencies, configuring beans, initializing models, handling errors, and thoroughly testing, you can enhance your application's robustness and performance.
Key Takeaway
Always maintain a proactive approach towards debugging and testing to secure your application's stability in production. For more information on efficient integration patterns, you may refer to [Spring Framework Documentation].
With these strategies in hand, you're well on your way to mastering the integration of LangChain4j with Spring Boot. Happy coding!