Managing Dependencies in Private Jenkins Shared Libraries
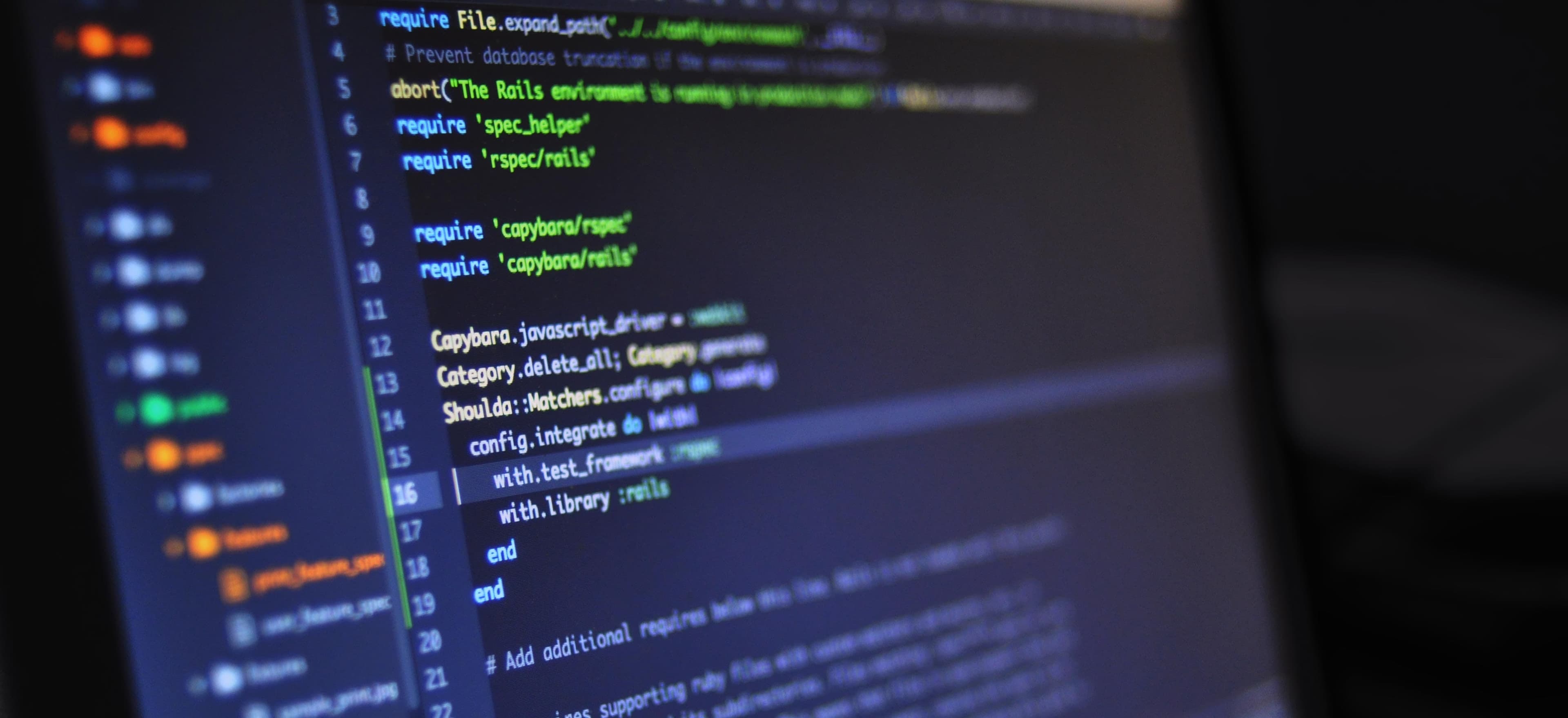
- Published on
Managing Dependencies in Private Jenkins Shared Libraries
In the rapidly evolving CI/CD landscape, Jenkins remains a primary choice for many organizations. One of its powerful features is the ability to create shared libraries, which allow teams to share and reuse code seamlessly. However, as your libraries expand, managing dependencies can become a complex task. In this blog post, we will explore best practices for managing dependencies in private Jenkins shared libraries, ensuring you maintain a clean, maintainable, and efficient environment.
What are Jenkins Shared Libraries?
Jenkins Shared Libraries allow teams to create reusable code that can be shared across multiple pipelines. This promotes code reuse and simplifies maintenance. Shared libraries can contain Groovy scripts that define specific functions, classes, or even entire pipelines, enabling developers to create modular Jenkins jobs.
Benefits of Using Shared Libraries
- Code Reusability: Reduce duplication by reusing code across multiple jobs.
- Centralized Management: Easier to update and manage dependencies and functionalities.
- Better Organization: Structure code in a way that makes it easier to navigate.
- Version Control: Use a version control system (like Git) for your library, allowing for rollback if necessary.
Setting Up a Shared Library
Before diving into dependency management, let's quickly set up a basic shared library. Here’s a minimal setup:
-
Folder Structure:
(root) ├── vars │ └── myLibrary.groovy ├── src │ └── com │ └── example │ └── MyUtility.groovy ├── README.md └── build.gradle
-
sample
myLibrary.groovy
:def call(String name) { echo "Hello, ${name}!" }
Basic Pipeline Usage
You can then use your shared library in a pipeline as follows:
@Library('your-shared-library') _
pipeline {
agent any
stages {
stage('Greeting') {
steps {
myLibrary('World')
}
}
}
}
This setup calls a simple greeting method from the shared library, demonstrating how easy it is to integrate your library into a pipeline.
Managing Dependencies in Shared Libraries
As your shared library grows, managing dependencies becomes crucial. Dependencies not only include other libraries but can also encompass external tools or scripts that your shared library may require. Proper management ensures that your library functions consistently across different Jenkins instances.
1. Use Gradle or Maven
Using a build tool like Gradle or Maven for dependency management is ideal. Here’s a brief overview of how to set it up using Gradle.
build.gradle:
plugins {
id 'groovy'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.codehaus.gpars:gpars:1.2.4' // Example dependency
}
task copyLibs(type: Copy) {
from configurations.runtimeClasspath
into "$buildDir/libs"
}
This Gradle file declares a dependency on the GPars library. Gradle automatically downloads this dependency during execution.
2. Isolate Dependencies
It’s essential to isolate dependencies to avoid version conflicts. This can be achieved through class loading mechanisms or by utilizing dependency scopes in tools like Gradle.
3. Version Control
Maintain version control of your shared library using Git. Adopt a clear branching strategy (like GitFlow) to handle different versions and releases of your library.
4. Use Jenkins Pipeline Libraries
Jenkins allows you to configure your libraries in Jenkinsfile
or through the Jenkins UI. You can define specific versions of your libraries using the @Library
annotation.
Example:
@Library('your-shared-library@v1.0') _
This example uses the specified version of your shared library, which can help prevent issues with breaking changes when you upgrade your library.
5. Dependency Injection
Utilizing dependency injection (DI) can make your libraries more flexible. DI allows you to pass dependencies at runtime, making it easier to swap out implementations without altering the dependent code.
Example:
class MyUtility {
def someService
MyUtility(def someService) {
this.someService = someService
}
def performAction() {
someService.execute()
}
}
In this example, you can inject different instances of someService
depending on the context in which MyUtility
is used.
6. Binding and Configuration
Utilizing configuration files or binding properties can help manage environment-specific settings and simplify dependency management. You can maintain different settings for different environments (development, testing, production) using properties files.
For example, create a configuration file config.groovy
:
def config = [
dbUrl: 'jdbc:mysql://localhost:3306/mydb',
dbUser: 'user',
dbPassword: 'password'
]
return config
7. Documentation
Document your library adequately. This includes creating a README.md
file and maintaining inline documentation. Use tools like Groovydoc to generate documentation automatically.
Final Considerations
Managing dependencies in private Jenkins shared libraries is essential for maintaining a clean, efficient, and flexible CI/CD pipeline. By adopting best practices such as using build tools, isolating dependencies, utilizing version controls, and documenting thoroughly, you can unleash the full potential of Jenkins shared libraries.
For further reading, consider exploring the following resources:
By implementing these practices, you ensure your Jenkins shared libraries remain robust, maintainable, and scalable for future development. Happy coding!