Common TTS Issues in Android and How to Fix Them
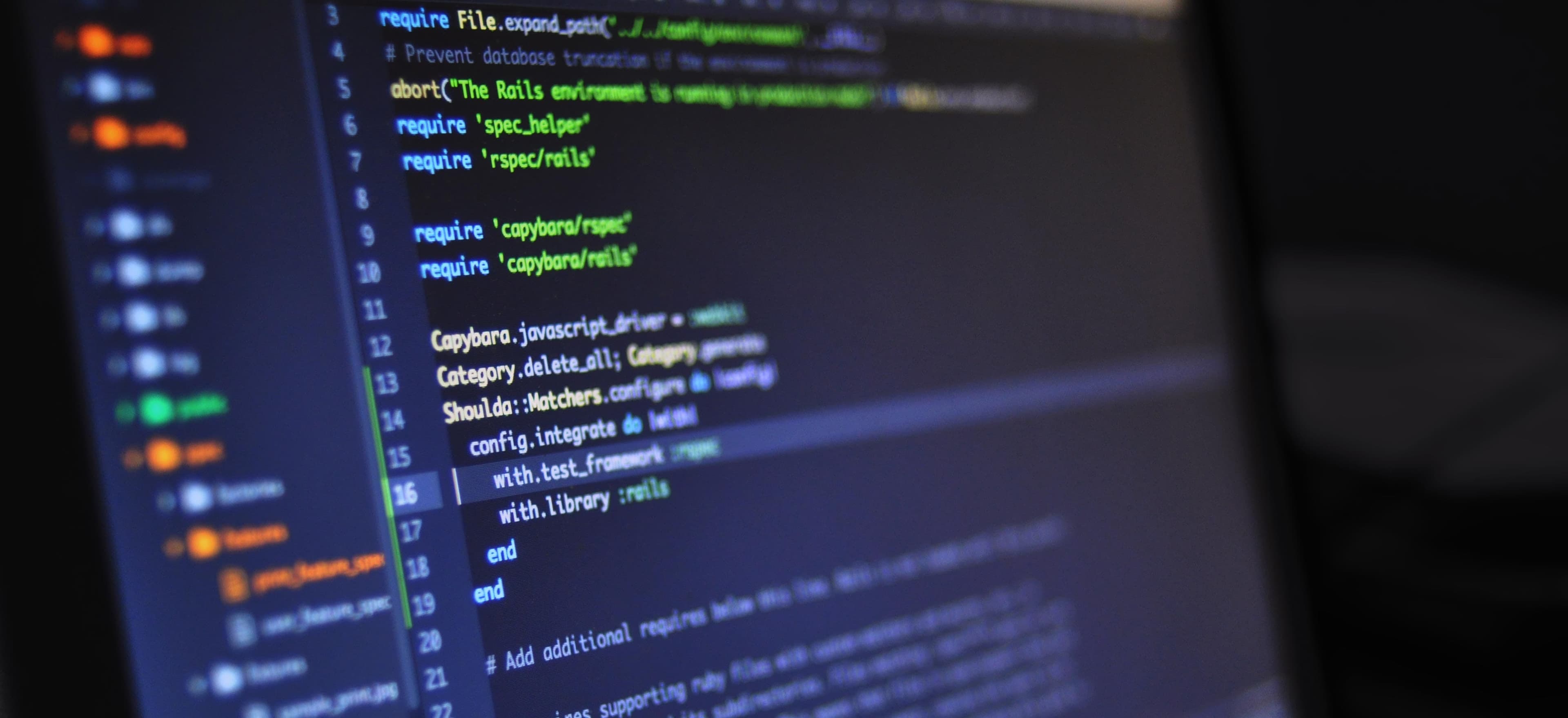
- Published on
Common TTS Issues in Android and How to Fix Them
Text-to-Speech (TTS) functionality has become an integral part of Android applications, enhancing accessibility and user experience. However, developers and users often encounter common issues that can disrupt seamless interaction. In this blog post, we're going to explore recurring TTS problems in Android and offer practical solutions.
Understanding TTS in Android
Text-to-Speech in Android uses the TextToSpeech
class to convert readable text into spoken voice. This feature is essential for various applications, including reading apps, language learning tools, and accessibility services.
Basic Setup of TTS
Before delving into problems, let's look at how to set up TTS:
import android.speech.tts.TextToSpeech;
import android.speech.tts.TextToSpeech.OnInitListener;
import java.util.Locale;
public class MyActivity extends AppCompatActivity implements OnInitListener {
private TextToSpeech textToSpeech;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
textToSpeech = new TextToSpeech(this, this);
}
@Override
public void onInit(int status) {
if (status == TextToSpeech.SUCCESS) {
int result = textToSpeech.setLanguage(Locale.US); // Setting the speech language
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Handle language data missing or not supported
}
} else {
// Handle initialization failure
}
}
// Clean up TTS when done
@Override
protected void onDestroy() {
if (textToSpeech != null) {
textToSpeech.stop();
textToSpeech.shutdown();
}
super.onDestroy();
}
}
This basic setup helps in initializing TTS and setting the desired language. But problems might arise. Let’s look into the common issues.
Common TTS Issues and Fixes
1. Initialization Failure
Issue: Sometimes, TTS doesn’t initialize properly, which means your application will not be able to synthesize speech.
Fix: Check for the availability of the Google Text-to-Speech Engine.
int status = TextToSpeech.Engine.CHECK_VOICE_DATA;
if (status != TextToSpeech.SUCCESS) {
Intent installIntent = new Intent();
installIntent.setAction(TextToSpeech.Engine.ACTION_INSTALL_TTS_DATA);
startActivity(installIntent); // Prompt the user to install the TTS data
}
This prompts the user to install necessary data. Correct setup and checking are essential for smooth functioning.
2. Language Not Supported
Issue: Some languages or accents may not be supported by the installed TTS engine.
Fix: Always check for language support after setting the language.
int result = textToSpeech.setLanguage(Locale.FRANCE);
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
// Notify user that language data is missing or unsupported
}
By doing this, you'll be able to handle cases when the required language is unavailable, ensuring a fallback plan is presented.
3. Inconsistent Speech Output
Issue: Users often report that the speech output can be choppy or unclear.
Fix: Adjust the pitch and speech rate, which can significantly enhance voice quality.
textToSpeech.setPitch(1.0f); // Normal pitch
textToSpeech.setSpeechRate(1.0f); // Normal speech rate
Experimenting with these settings might improve the overall output quality. For more information, you can refer to the Android Developers Documentation on Text-to-Speech.
4. Device Compatibility Issues
Issue: TTS might work on some devices but not on others due to software compatibility.
Fix: Always include checks for compatibility in your code.
if (textToSpeech.isLanguageAvailable(Locale.US) == TextToSpeech.LANG_MISSING_DATA) {
// Prompt for TTS installation or suggest alternatives
}
Integrating checks is necessary to ensure that your app adapts to various devices smoothly.
5. Voice Quality and Accent Issues
Issue: The default voice might not be ideal for every user, leading to complaints about accents or the clarity of the voice produced.
Fix: Allow users to select their preferred voice.
for (Voice voice : textToSpeech.getVoices()) {
// Present available voices to the user for selection
}
This creates a more personalized experience, as users can select a voice that caters to their preference or clarity needs.
Debugging and Testing Tips
To ensure that your TTS functionality runs flawlessly:
-
Utilize Log Statements: Log the state of the TTS engine at various stages.
Log.d("TTS", "TTS initialized: " + (textToSpeech != null));
-
Check Permissions: Ensure you have the necessary permissions in your
AndroidManifest.xml
. -
Test on Multiple Devices: Always conduct testing on different Android devices and versions to identify potential issues.
-
Engage User Feedback: Create a feedback loop for users to report any TTS issues they encounter for continual improvement.
The Closing Argument
Troubleshooting TTS issues in Android can sometimes feel daunting. However, by understanding the common pitfalls and implementing the right checks, you can create a reliable and engaging speech synthesis experience.
Whether it's handling language support, adjusting settings, or ensuring device compatibility, a little attention can significantly enhance your application's TTS functionality. Make these changes and watch as users interact more fluidly with your app.
For more information on improving accessibility using TTS, consider exploring the Android Accessibility Guidelines.
Feel free to share your experiences with TTS issues in the comments below, and let’s create a better experience together!