Debugging JSAP: Common Pitfalls in Java Command-Line Interfaces
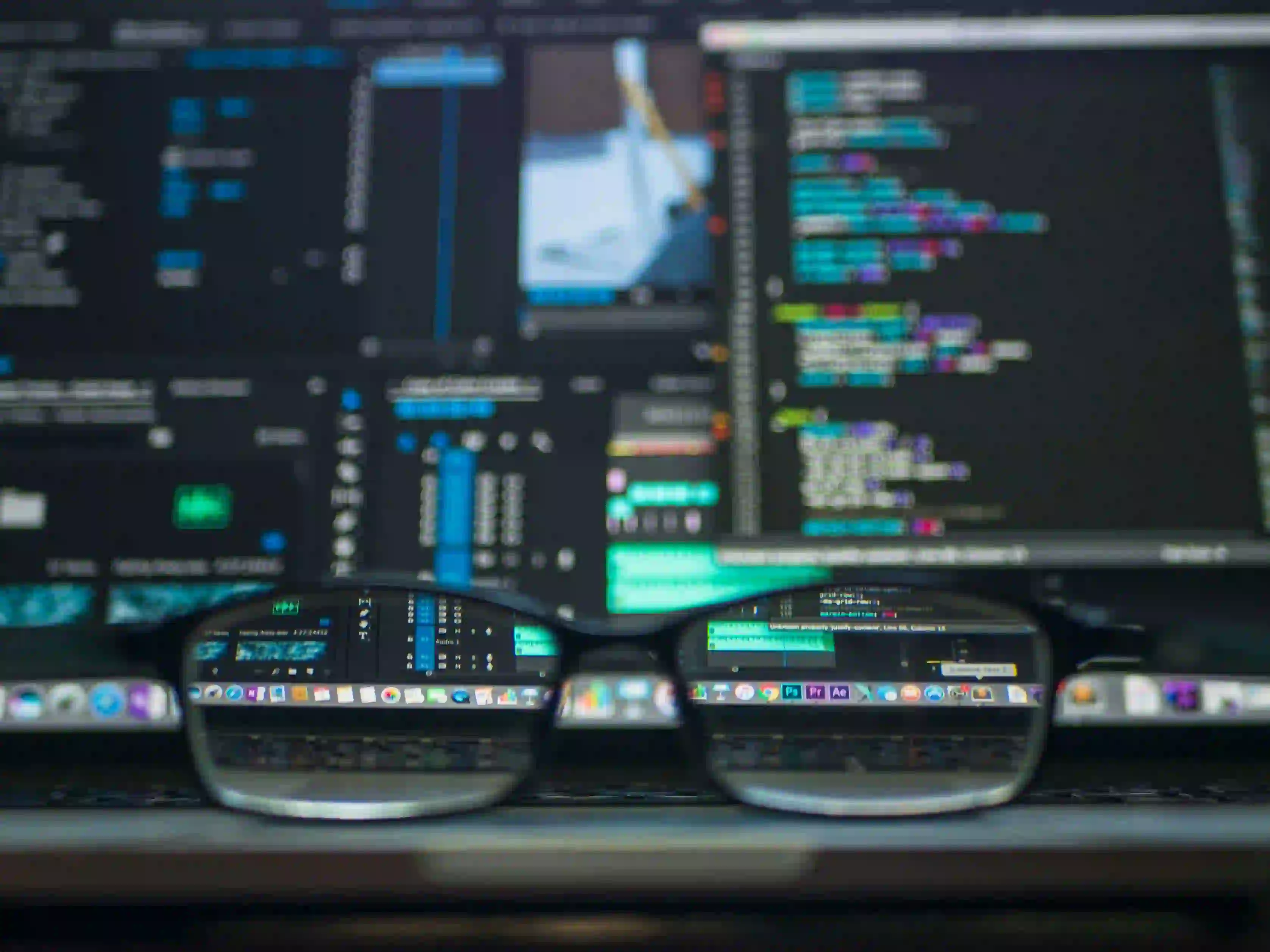
Debugging JSAP: Common Pitfalls in Java Command-Line Interfaces
Java is a powerful programming language celebrated for its flexibility and robustness. When it comes to building command-line interfaces (CLIs) in Java, the Java Simple Argument Parser (JSAP) proves to be an invaluable tool. However, like many frameworks, it comes with its own set of challenges. This blog post aims to cover common pitfalls you might encounter when using JSAP and how to debug them effectively.
What is JSAP?
JSAP is a library designed to help developers handle command-line arguments in Java easily and efficiently. It allows for easy parsing of command-line options, providing both positional and named parameters seamlessly. If you're looking to learn more about JSAP, you can visit the JSAP Documentation for more details.
Why Use JSAP?
Using JSAP offers numerous advantages:
- Ease of Use: With JSAP, you can define your command-line options declaratively.
- Type Safety: It automatically converts input strings to appropriate data types.
- Comprehensive Error Reporting: Provides clear feedback if necessary arguments are missing.
Now that we have an understanding of JSAP, let’s delve into some common pitfalls you may encounter while using it and how to troubleshoot them.
Common Pitfalls in JSAP
1. Not Defining Required Options Properly
A common mistake is not marking required options correctly. If a required option is not provided by the user, JSAP does not execute, resulting in no output, potentially perplexing developers.
Example Code Snippet
import com.mks.jsap.*;
public class CommandLineExample {
public static void main(String[] args) {
JSAP jsap = new JSAP();
// Defining a required string option
FlaggedOption name = new FlaggedOption("name")
.setStringParser(new StringParser())
.setRequired(true) // Marking as required
.setHelp("Your name.");
jsap.registerParameter(name);
// Parsing the command line arguments
JSAPResult config = jsap.parse(args);
if (!config.success()) {
System.err.println("Missing required options, check your command.");
System.exit(1);
}
String userName = config.getString("name");
System.out.println("User Name: " + userName);
}
}
Commentary
In the above snippet, we have created a simple JSAP application that expects a required option --name
to be specified. The setRequired(true)
method ensures that if the user does not provide this option, an error message is displayed. Always confirm that mandatory options are clearly indicated to avoid confusion during execution.
2. Misunderstanding Positional vs. Flagged Options
Another common pitfall is confusing positional arguments with flagged ones. In JSAP, positional arguments must be provided in a specific order, while flagged options can be provided in any order.
Example Code Snippet
import com.mks.jsap.*;
public class MyProgram {
public static void main(String[] args) {
JSAP jsap = new JSAP();
// Defining a positional argument
UnflaggedOption inputFile = new UnflaggedOption("input_file")
.setRequired(true)
.setHelp("Input file to process.");
// Defining a flagged argument
FlaggedOption verbose = new FlaggedOption("verbose")
.setDefault("false")
.setStringParser(new BooleanParser())
.setHelp("Enable verbose mode.");
jsap.registerParameter(inputFile);
jsap.registerParameter(verbose);
JSAPResult config = jsap.parse(args);
if (!config.success()) {
System.err.println("Check command line options.");
System.exit(1);
}
String file = config.getString("input_file");
boolean isVerbose = config.getBoolean("verbose");
System.out.println("Processing file: " + file + ", Verbose mode: " + isVerbose);
}
}
Commentary
In this example, we introduced a positional argument (input_file
) and a flagged option (--verbose
). If users input the values in an incorrect format (for instance, supplying --verbose
before input_file
), they might encounter parsing errors. Remember, positional arguments are strict about order, while flagged ones are flexible.
3. Improper Error Handling
Sometimes, developers might ignore the error states provided by JSAP, resulting in programs crashing without informative messages.
Example Code Snippet
import com.mks.jsap.*;
public class ErrorHandlingExample {
public static void main(String[] args) {
JSAP jsap = new JSAP();
FlaggedOption debug = new FlaggedOption("debug")
.setDefault("false")
.setStringParser(new BooleanParser())
.setHelp("Enable debugging.");
jsap.registerParameter(debug);
JSAPResult config = jsap.parse(args);
if (!config.success()) {
for (String error : config.getErrorMessage()) {
System.err.println("Error: " + error);
}
System.exit(1);
}
// Application logic
}
}
Commentary
In this snippet, we added robust error reporting. Iterating over each error in the result helps users understand what went wrong. Don’t overlook error handling — it’s crucial for delivering a good user experience. Clear feedback on what needs to be corrected can save users time and frustration.
4. Failing to Consider Different Input Formats
JSAP provides various parsers for different data types, such as integers, booleans, and even user-defined objects. It is crucial to utilize the right parser; otherwise, you risk runtime errors due to unexpected input types.
Example Code Snippet
import com.mks.jsap.*;
public class InputFormatExample {
public static void main(String[] args) {
JSAP jsap = new JSAP();
FlaggedOption age = new FlaggedOption("age")
.setStringParser(new IntegerParser().setRange(0, 150))
.setHelp("Your age (between 0-150).");
jsap.registerParameter(age);
JSAPResult config = jsap.parse(args);
if (!config.success()) {
System.err.println("Invalid age format. Please enter a valid integer.");
System.exit(1);
}
int userAge = config.getInt("age");
System.out.println("User Age: " + userAge);
}
}
Commentary
In this example, we defined an age parameter and enforced a range using IntegerParser
. Parameters are essential for managing user input effectively. Java developers can do well to specify not just the type but also constraints on the accepted values. Wrong input types can throw exceptions; hence they need to be validated properly.
Wrapping Up
In summary, while JSAP simplifies argument parsing, it isn't without its pitfalls. By defining required options accurately, understanding the difference between positional and flagged parameters, implementing proper error handling, and choosing the correct parser types, we can significantly reduce the number of issues we face when using JSAP.
Using JSAP correctly helps streamline the development of Java command-line interfaces, making your applications more robust and user-friendly. For more information, refer to the JSAP Tutorial for further insights and advanced usage examples.
Happy coding!