Mastering NoSQL Queries: Common Pitfalls with Hibernate OGM
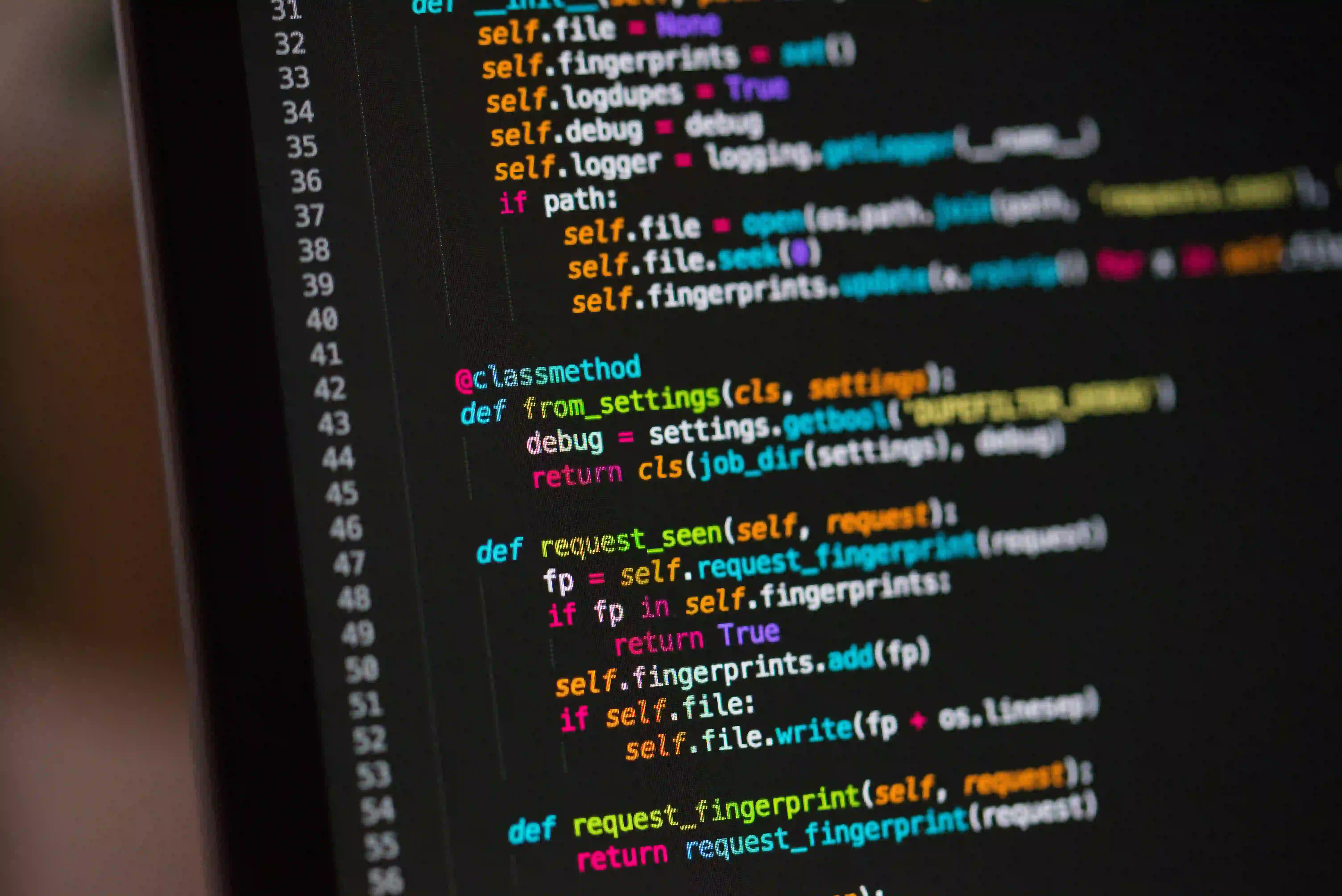
Mastering NoSQL Queries: Common Pitfalls with Hibernate OGM
As the landscape of data continues to evolve, the need for flexible, scalable storage solutions has grown significantly. NoSQL databases have emerged as a viable alternative to traditional relational databases, particularly for applications that require high speed and flexibility. When working with NoSQL databases in Java, Hibernate Object/Grid Mapper (OGM) provides a robust framework that simplifies this process. However, developers may encounter several common pitfalls. In this blog post, we'll explore these pitfalls and provide strategies to avoid them while mastering NoSQL queries with Hibernate OGM.
What is Hibernate OGM?
Hibernate OGM (Object/Grid Mapper) is an extension of Hibernate that allows developers to interact with NoSQL databases using the familiar Hibernate programming model. OGM is not limited to just one NoSQL database but supports various databases like MongoDB, Neo4j, and CouchDB. This compatibility allows developers to switch databases without changing the entire codebase.
Benefits of Using Hibernate OGM
- Abstraction: Hibernate OGM abstracts the underlying NoSQL database, allowing developers to use familiar Hibernate features.
- Cross-Database Compatibility: Because OGM supports multiple NoSQL databases, it offers flexibility in choice of technology.
- Entity Mapping: Just like in traditional Hibernate, developers can map Java classes to NoSQL entities seamlessly.
Before we delve into the common pitfalls, let's set the stage with a simple code snippet illustrating how Hibernate OGM can be used to perform basic operations on a NoSQL database.
Example Code Snippet: Setting Up Hibernate OGM
import org.hibernate.ogm.datastore.mongo.MongoDatabaseConfiguration;
import org.hibernate.ogm.datastore.mongo.MongoDatabaseService;
import org.hibernate.ogm.session.session;
public class HibernateOGMExample {
public static void main(String[] args) {
Session session = createSession();
// Store an entity
MyEntity entity = new MyEntity("Hello, OGM!");
session.persist(entity);
session.flush();
// Retrieve and print the entity
MyEntity retrievedEntity = session.find(MyEntity.class, entity.getId());
System.out.println("Retrieved Entity: " + retrievedEntity.getMessage());
session.close();
}
private static Session createSession() {
// This method is assumed to return a Hibernate OGM session
}
}
In the above example, we create a basic setup for using Hibernate OGM. Here’s what happens in the code:
- We start by creating a
Session
, which serves as a context for unit of work with the database. - We create an instance of our entity,
MyEntity
, and persist it. - Finally, we retrieve the persisted entity based on its ID.
Common Pitfalls in Hibernate OGM Queries
1. Insufficient Indexing
Problem: In NoSQL databases, query performance heavily relies on indexing. Failing to create appropriate indexes can lead to slow queries.
Solution: Always ensure that you create indexes on fields that will be frequently queried. For instance, in MongoDB, you can create an index as follows:
@Document(collection = "my_entities")
public class MyEntity {
@Id
private String id;
@Indexed // This annotation will help to create an index
private String message;
// Getters and Setters
}
Why: Indexing significantly speeds up query operations, especially on large data sets. By adding the @Indexed
annotation, Hibernate OGM will ensure that a relevant index is created in the database for the message
field.
2. Lack of Transaction Management
Problem: NoSQL databases may not support transactions in the same way traditional databases do. Not managing transactions can lead to inconsistent data states.
Solution: Use session transactions to manage persistence operations effectively.
session.beginTransaction();
try {
session.persist(entity);
session.getTransaction().commit();
} catch (Exception e) {
if (session.getTransaction() != null) {
session.getTransaction().rollback();
}
throw e; // or handle exception accordingly
}
Why: Proper transaction management ensures that operations are executed atomically. If an error occurs, rolling back the transaction can prevent partial updates to the database, thus maintaining consistency.
3. Misunderstanding Data Types
Problem: Unlike relational databases, NoSQL databases can have flexible schemas. Misinterpreting data types or structures can lead to runtime errors.
Solution: Identify and use the correct data types as per the requirements of the NoSQL database you are using. For instance, when storing list types in MongoDB, use the appropriate Java collections.
@ElementCollection
private List<String> tags; // Ideally this should be an ArrayList or List
Why: Choosing the right data structure aligns with the underlying database's capabilities and avoids issues with serialization and queries.
4. Ignoring Lazy Loading
Problem: Hibernate OGM uses lazy loading, which means that data is loaded only when it is accessed. For some use cases, this can lead to unexpected delays or even exceptions if the session is closed before accessing the data.
Solution: Be mindful of the session’s lifecycle. Initialize required lazy-loaded properties before closing the session.
// Accessing lazy-loaded fields before closing the session
entity.getTags().size();
Why: Preventing lazy-loading pitfalls ensures that you can access all the data you need without encountering exceptions related to unmanaged sessions.
5. Not Utilizing Query Language Effectively
Problem: Many developers coming from an ORM background may struggle with NoSQL query languages (like MongoDB's query language). This can lead to inefficient queries.
Solution: Familiarize yourself with native queries specific to the NoSQL database you are using, and leverage Hibernate Criteria API when needed.
List<MyEntity> entities = session.createQuery("from MyEntity where message = :message")
.setParameter("message", "Hello, OGM!")
.list();
Why: Understanding how to craft efficient queries helps to enhance performance and ensures you're taking full advantage of the underlying database capabilities.
Closing Remarks
Hibernate OGM provides a powerful way for Java developers to leverage NoSQL databases, but it is not without its challenges. By understanding the common pitfalls, you can improve the reliability and efficiency of your NoSQL interactions. Remember that the key to mastering Hibernate OGM isn't just about knowing the API — it's also about understanding how to use NoSQL databases effectively.
For further reading on NoSQL databases, consider visiting MongoDB Documentation and Hibernate OGM Documentation for more extensive information and best practices. Happy coding!