Common Pitfalls of Using HttpURLConnection in Java
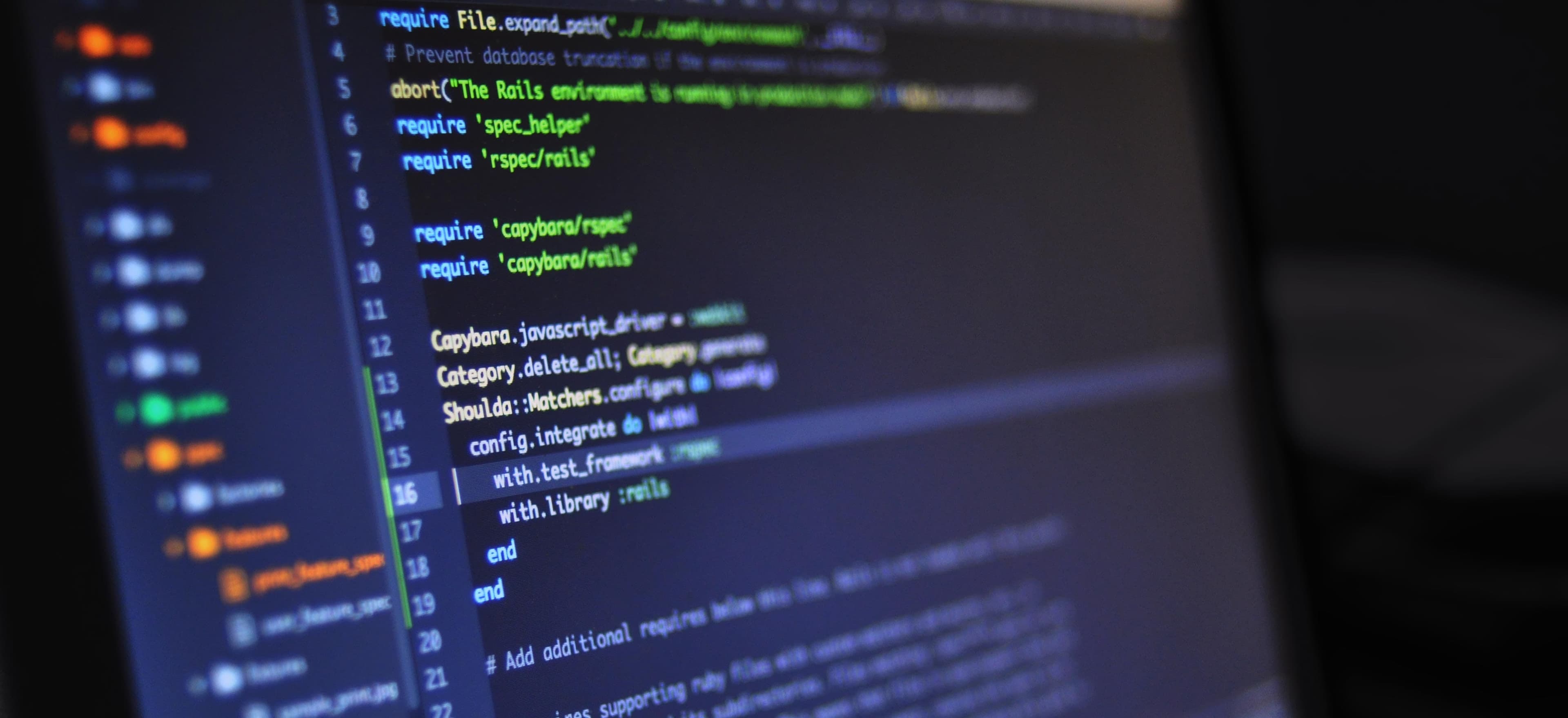
- Published on
Common Pitfalls of Using HttpURLConnection in Java
In the realm of Java programming, network communication is a crucial aspect, particularly when it comes to web services. One way to achieve this is by using the HttpURLConnection
class, which provides a simple yet powerful way to manage HTTP requests and responses. However, while it's a versatile tool, there are common pitfalls that developers often encounter. In this blog post, we will delve into these pitfalls and present solutions to enhance your code quality and performance.
Understanding HttpURLConnection
Before diving into the common pitfalls, let's take a brief look at what HttpURLConnection is. It is a class in the java.net
package that provides a way to connect to a specified URL and communicate with web resources. You can create GET, POST, and other HTTP request methods using this class.
Basic Example
Here's a simple code snippet demonstrating how to make a basic GET request:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class SimpleHttpRequest {
public static void main(String[] args) {
String url = "http://example.com";
try {
URL obj = new URL(url);
HttpURLConnection connection = (HttpURLConnection) obj.openConnection();
// Optional default is GET
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
System.out.println("Response Code : " + responseCode);
// Read response
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Print result
System.out.println(response.toString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, we initiate a GET request, read the server's response, and print it out. However, there are several errors and inefficient patterns that can occur—so let's explore common pitfalls in more detail.
Common Pitfalls
1. Ignoring Proper Error Handling
Many developers overlook proper error handling while working with HttpURLConnection. When an HTTP request fails, not handling exceptions can lead to application crashes or undefined behaviors.
Solution: Always Handle Exceptions
Use robust exception handling to manage errors gracefully and log important information.
try {
// Your HTTP connection code
} catch (IOException e) {
System.err.println("IOException occurred: " + e.getMessage());
// Additional logging can be performed here.
}
2. Not Closing Connections
Failing to close input and output streams properly can lead to resource leaks. In Java, it's crucial to close these resources once you're done using them.
Solution: Use Try-With-Resources
The try-with-resources statement ensures that each resource is closed at the end of the statement.
try (BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()))) {
// Read response
} catch (IOException e) {
// Handle exception
}
3. Blocking Calls
HttpURLConnection operates in a blocking manner, which means that it can halt the execution of your program until a response is received. In a GUI application, this could freeze the entire application.
Solution: Use a Separate Thread
For GUI applications, consider using a separate thread or an asynchronous task to handle network requests.
Runnable task = () -> {
// Perform HTTP request here
};
new Thread(task).start();
4. Not Setting Timeouts
By default, HttpURLConnection does not impose deadlines on HTTP requests. Long network delays can hang your application indefinitely.
Solution: Set Connection Timeouts
You can set connection and read timeouts to avoid waiting too long for a response.
connection.setConnectTimeout(5000); // 5 seconds
connection.setReadTimeout(5000); // 5 seconds
5. Hardcoding URLs
Hardcoding URLs directly in your methods can lead to reduced flexibility and maintainability. If the URL changes, you'll have to modify your code in multiple places.
Solution: Use External Configuration
Consider loading URLs from a configuration file or as environment variables.
public class Config {
public static final String BASE_URL = System.getenv("BASE_URL");
}
6. Neglecting to Validate Server Responses
Even a successful HTTP status code (e.g., 200) does not guarantee that the server response is valid. Failing to verify the content type or parsing errors can result in unexpected behaviors.
Solution: Validate Responses
Check the response's content type and manage the payload accordingly.
String contentType = connection.getContentType();
if (!contentType.equals("application/json")) {
throw new IllegalArgumentException("Unexpected content type: " + contentType);
}
7. Inadequate Logging
Verbose logging is often seen as unnecessary, but it can be invaluable for identifying and debugging issues.
Solution: Implement Robust Logging
Use a logging framework to capture potential errors and added context.
import java.util.logging.Logger;
private static final Logger LOGGER = Logger.getLogger(SimpleHttpRequest.class.getName());
try {
// Your HTTP request code
} catch (IOException e) {
LOGGER.severe("HTTP request failed: " + e.getMessage());
}
8. Mismanaging Thread Safety
If you mishandle shared resources between multiple threads, you could face concurrency issues.
Solution: Synchronization
Ensure that any shared resources are accessed in a thread-safe manner.
synchronized (someSharedResource) {
// Access shared resource
}
Summary
While HttpURLConnection provides a fundamental way to engage in network communication, it’s essential to tread carefully. Armed with knowledge of the common pitfalls and equipped with effective solutions, you can improve the quality and reliability of your Java HTTP requests.
For more specialized use cases or if you're looking for enhanced HTTP client capabilities, consider looking into libraries like Apache HttpClient or OkHttp, as they provide more advanced features and better ease of use.
Additional Resources
By being aware of these common pitfalls and applying recommended best practices, you can ensure that your Java-based applications communicate effectively over HTTP, with robustness and efficiency. Happy coding!
Checkout our other articles