Mastering Java 8 Date and Time: Common Pitfalls to Avoid
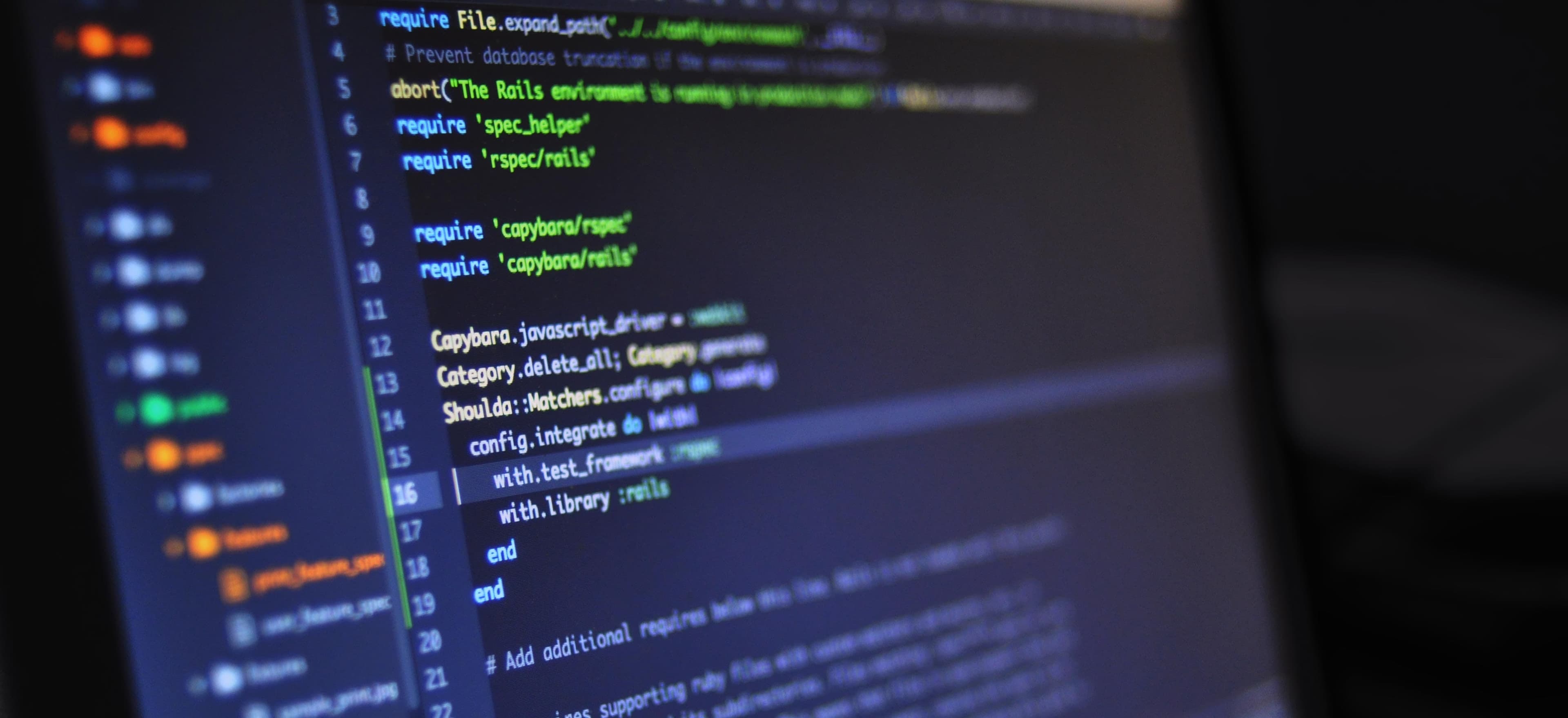
- Published on
Mastering Java 8 Date and Time: Common Pitfalls to Avoid
Java 8 introduced a new date and time API that fundamentally changed how developers handle date and time manipulation in Java applications. While this new API solved many problems present in previous versions, it also brought its own set of challenges. In this article, we will explore some common pitfalls associated with the Java 8 date and time API, how to avoid them, and provide practical code examples to help you master it.
The Introduction of the New API
Before Java 8, the main classes used for date and time manipulation were java.util.Date
and java.util.Calendar
. These classes were often criticized for being cumbersome and inconsistent. The new java.time package introduced in Java 8 offers a more comprehensive and efficient approach to handling date and time.
Benefits of the New Date-Time API
- Immutable Objects: All classes in the new API are immutable, meaning that their state cannot be altered after creation.
- Clarity and Ease of Use: The API provides clear method names that express intent, improving code readability.
- Better Time Zones Handling: The inclusion of the
ZoneId
class simplifies the handling of time zones. - Enhanced Flexibility: The new API provides various classes like
LocalDate
,LocalTime
, andLocalDateTime
that allow developers to work with specific aspects of dates and times.
Common Pitfalls and How to Avoid Them
1. Confusing LocalDateTime
with ZonedDateTime
One of the frequent mistakes is confusing LocalDateTime
with ZonedDateTime
. LocalDateTime
represents date and time without time zone information, while ZonedDateTime
contains both date-time and time-zone data.
Pitfall Example:
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println("Local DateTime: " + localDateTime);
In the code above, if you accidentally use localDateTime
to represent time in a specific time zone, you might face unexpected behavior or errors especially if your application is deployed in different geographic locations.
Solution: Always use ZonedDateTime
when you need to represent a date and time with timezone information.
Code Example:
ZonedDateTime zonedDateTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("Zoned DateTime: " + zonedDateTime);
2. Not Using Java Time Formatters Correctly
Java 8's date and time API includes the DateTimeFormatter
class, which is essential for parsing and formatting dates. Developers sometimes overlook how to create custom formatters or use the default ones incorrectly.
Pitfall Example:
String dateString = "2023-10-31";
LocalDate localDate = LocalDate.parse(dateString);
System.out.println("Parsed LocalDate: " + localDate);
While the default format for LocalDate
is YYYY-MM-DD
, using different patterns can lead to errors.
Solution: To avoid parsing errors, always specify the expected pattern when dealing with custom date formats.
Code Example:
String customDateString = "31-10-2023"; // Day-Month-Year format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
LocalDate customDate = LocalDate.parse(customDateString, formatter);
System.out.println("Parsed Custom LocalDate: " + customDate);
3. Ignoring Daylight Saving Time Changes
When working with time zones, you must consider daylight saving time (DST) changes. Failing to handle these changes can lead to incorrect calculations, especially when adding or subtracting hours.
Pitfall Example:
ZonedDateTime zdt = ZonedDateTime.of(2023, 11, 1, 12, 0, 0, 0, ZoneId.of("America/New_York"));
System.out.println("Before DST ends: " + zdt.plusHours(24));
In the code above, adding 24 hours to a time near the end of daylight saving time yields an incorrect result.
Solution: Always perform date-time arithmetic operations with full awareness of whether DST is in effect.
Code Example:
ZonedDateTime zdtWithDST = ZonedDateTime.of(2023, 11, 1, 12, 0, 0, 0, ZoneId.of("America/New_York"));
ZonedDateTime nextDay = zdtWithDST.plusDays(1); // This accounts for DST automatically
System.out.println("Next Day (accounting for DST): " + nextDay);
4. Failing to Use Duration
and Period
Effectively
When developers need to calculate the intervals between two dates, they might overlook the utility classes Duration
and Period
. These two classes are specifically designed to operate with different units of time.
- Duration is used for time-based amounts (like seconds and nanoseconds).
- Period is used for date-based amounts, like years, months, and days.
Pitfall Example:
LocalDate startDate = LocalDate.of(2023, 1, 1);
LocalDate endDate = LocalDate.of(2023, 10, 31);
long daysBetween = ChronoUnit.DAYS.between(startDate, endDate);
System.out.println("Days between: " + daysBetween);
While using ChronoUnit
for days is fine, it might not capture the nuances of months or years.
Solution: Use Period
when working with date-based calculations.
Code Example:
LocalDate startDate = LocalDate.of(2023, 1, 1);
LocalDate endDate = LocalDate.of(2023, 10, 31);
Period period = Period.between(startDate, endDate);
System.out.println("Period: " + period.getMonths() + " months, " + period.getDays() + " days");
A Final Look
Mastering the Java 8 Date and Time API is essential for any Java developer. By avoiding these common pitfalls, developers can work more effectively with dates and times in their applications, ensuring correct, readable, and maintainable code.
If you’re looking to deepen your understanding, check out the Oracle Java documentation for the java.time package. Additionally, resources like Baeldung’s Date and Time in Java 8 provide in-depth tutorials and examples.
By paying attention to the nuances of Java 8's time-handling mechanisms, developers can enhance their coding practices and improve the overall quality of their applications. Happy coding!
Checkout our other articles