Visualizing SQL Data: Common JOOQ & JavaFX Pitfalls
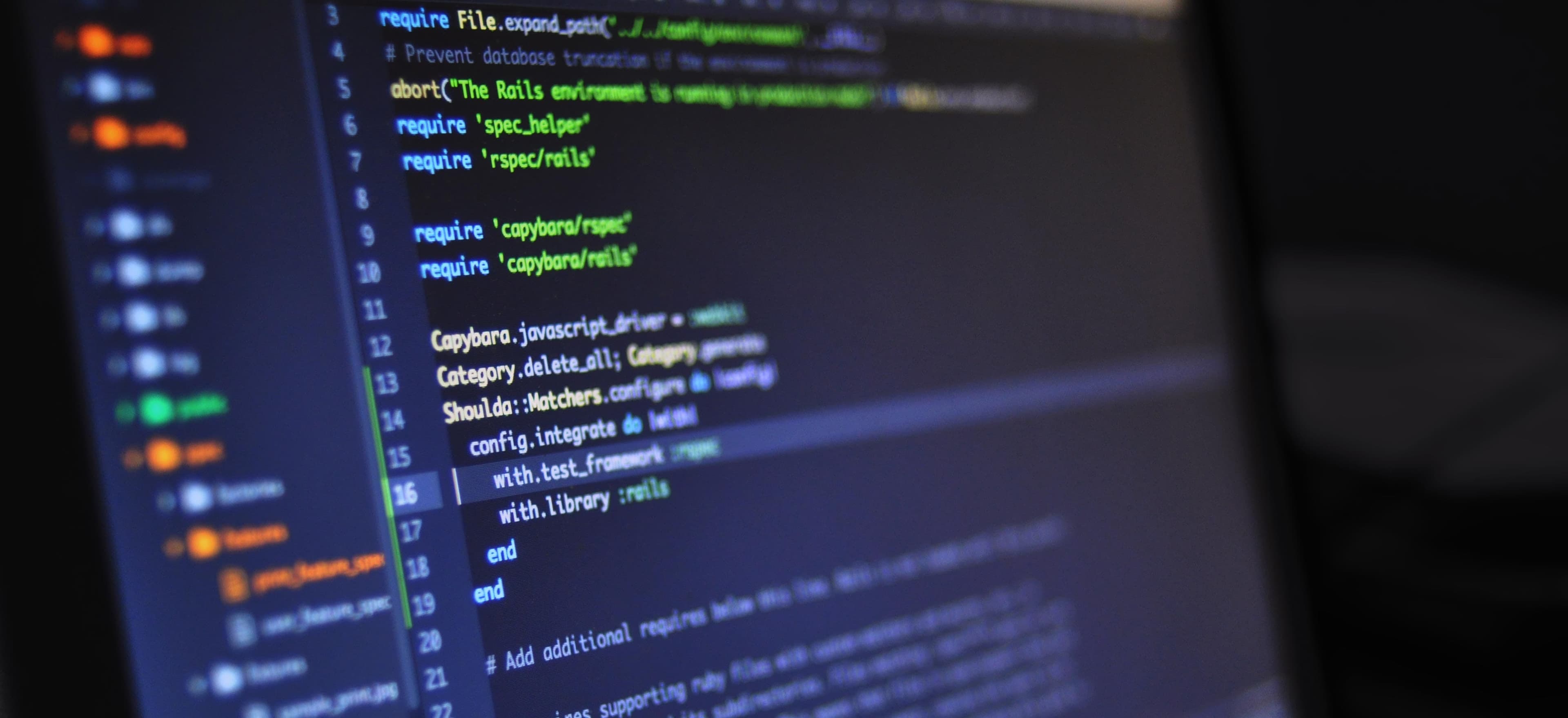
- Published on
Visualizing SQL Data: Common JOOQ & JavaFX Pitfalls
Data visualization plays a vital role in understanding the intricacies of large datasets. By leveraging powerful libraries like JOOQ for database interaction and JavaFX for user interface design, developers can create insightful visualizations efficiently. However, pitfalls can arise in the integration of these technologies. In this post, we will discuss common JOOQ and JavaFX pitfalls when visualizing SQL data and how to avoid them.
Table of Contents
Why JOOQ and JavaFX?
JOOQ (Java Object Oriented Querying) is a Java library that enables developers to construct SQL queries programmatically. It emphasizes type safety and concise query writing, making it a popular choice for interacting with relational databases. JavaFX, on the other hand, is a rich client framework for building user interfaces in Java.
By combining JOOQ for data retrieval and JavaFX for visualization, developers can create applications that provide dynamic views of database information.
Common Pitfalls
Pitfall 1: Mismanaging Database Connections
One of the first mistakes developers make is improper management of database connections. This can lead to resource leaks and sluggish application performance. Many developers tend to use a single connection instance throughout the application, which is not recommended.
Example Code
Connection connection = DriverManager.getConnection(url, user, password);
Why? Not closing the connection can lead to exhausting the database resources. It's a best practice to utilize connection pooling or try-with-resources statements to handle connections.
Improved Code
try (Connection connection = DriverManager.getConnection(url, user, password)) {
// Execute queries here
} // Connection is automatically closed
Pitfall 2: Ignoring Thread Safety
JOOQ and JavaFX are not thread-safe by default. Therefore, when you are fetching data using JOOQ and trying to visualize it using JavaFX, you might run into concurrency issues. JavaFX requires the UI updates to happen on the JavaFX Application Thread.
Example Code
Result<Record> result = DSL.using(connection).select().from("my_table").fetch();
Why? Executing the above code on a background thread may result in exceptions when attempting to update the UI.
Improved Code Using Platform.runLater
Platform.runLater(() -> {
Result<Record> result = DSL.using(connection).select().from("my_table").fetch();
// Update JavaFX UI components here
});
Pitfall 3: Inefficient Data Fetching
Another common issue arises from fetching large volumes of data all at once, leading to performance degradation. Instead, data should be fetched in manageable chunks or on demand.
Example Code
List<MyData> dataList = DSL.using(connection)
.select()
.from("my_table")
.fetchInto(MyData.class);
Why? Fetching too much data can overwhelm the application and slow down the UI.
Improved Code Using Pagination
int pageSize = 100;
List<MyData> dataList = DSL.using(connection)
.select()
.from("my_table")
.limit(pageSize)
.offset(currentPage * pageSize)
.fetchInto(MyData.class);
This approach allows for smoother UI interactions and avoids memory problems.
Pitfall 4: Lack of Robust Error Handling
Errors can arise from various sources: network issues, malformed SQL queries, or unexpected data types. Ignoring error handling can lead to a poor user experience.
Example Code
try {
Result<Record> result = DSL.using(connection).select().from("my_table").fetch();
} catch (Exception e) {
e.printStackTrace();
}
Why? Simply printing the stack trace does not provide a user-friendly solution nor does it properly log the issue.
Improved Code with Meaningful Error Handling
try {
Result<Record> result = DSL.using(connection).select().from("my_table").fetch();
} catch (SQLException e) {
showAlert("Database Error", "Failed to retrieve data: " + e.getMessage());
} catch (Exception e) {
showAlert("Unexpected Error", "An error occurred: " + e.getMessage());
}
The use of a helpful alert dialog notifies the user of the issue instead of simply logging it.
Best Practices for Data Visualization
When working with JOOQ and JavaFX, adhering to a set of best practices can further improve your application's performance and usability.
-
Use a Model-View-Controller (MVC) Architecture Implementing the MVC pattern can help decouple your application’s data, logic, and presentation layers, making it easier to manage.
-
Implement Pagination and Lazy Loading Fetch only the data you need at any given time. This keeps your UI responsive and improves the application's performance.
-
Keep Your UI Thread Responsive Utilize background threads for intensive processing tasks to avoid freezing the UI. The JavaFX
Service
class can simplify background tasks. -
Leverage Observable Collections Use
ObservableList
in JavaFX to automatically update your UI when the underlying data changes. -
Prioritize User Experience Make your application intuitive. Ensure that error handling conveys necessary information to users while maintaining a smooth workflow.
Wrapping Up
Visualizing SQL data with JOOQ and JavaFX comes with its own set of challenges, but these pitfalls are easy to avoid with the right strategies. By managing database connections efficiently, ensuring thread safety, fetching data thoughtfully, and implementing robust error handling, you can build applications that are both reliable and user-friendly.
For more on effective Java development practices, you may want to check out Java's official documentation and delve into topics like JavaFX threading and JOOQ's querying capabilities to further enhance your knowledge.
Further Reading
- Learn JavaFX: The Definitive Guide
- JOOQ 3.18 User Manual
- Effective Java (2nd Edition)
By avoiding common pitfalls and following best practices, you can create powerful, efficient applications that visualize SQL data effectively. Cheers to clean code and stunning data visualizations!
Checkout our other articles