Mastering Java 17: Common Pitfalls with Pattern Matching in Switch
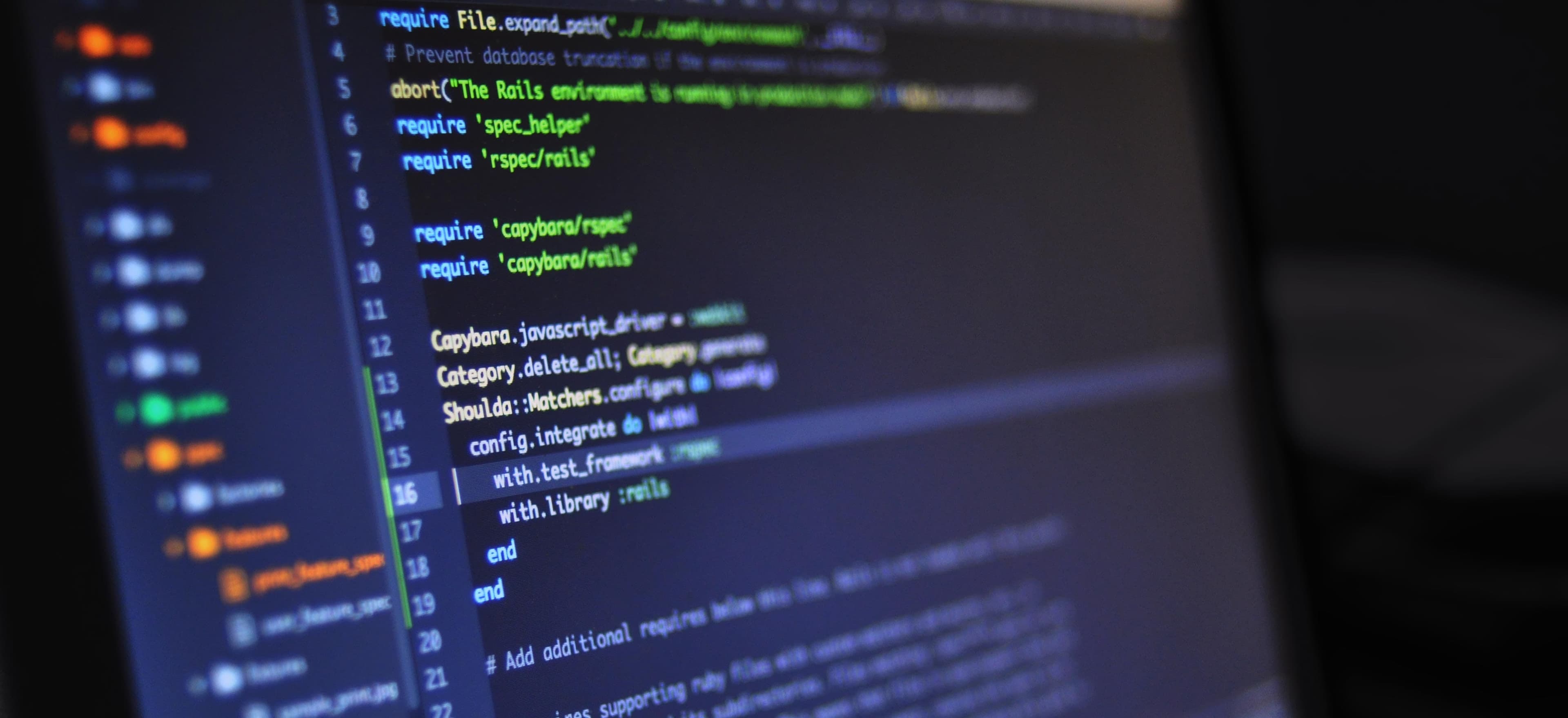
- Published on
Mastering Java 17: Common Pitfalls with Pattern Matching in Switch
Java is an evolving programming language with continuous advancements aimed at improving developer experience and efficiency. One of the most significant features introduced in Java 17 is Pattern Matching for switch, which enhances the switch statement's capability to handle various data types and conditions seamlessly. However, as with all new features, there can be common pitfalls that developers must be aware of.
In this blog post, we will explore these common pitfalls, provide clear examples to help you understand the correct usage of pattern matching in switch statements, and offer tips on how to avoid the most frequently encountered issues.
What is Pattern Matching in Switch?
The concept of pattern matching in switch statements streamlines the way developers match values against specific patterns. It simplifies code and improves readability by reducing boilerplate conditions and casting.
Basic Syntax
The new syntax enhances traditional switch expressions. Here's a basic example illustrating how to use it:
public String describe(Object obj) {
switch (obj) {
case String s -> "A string of length " + s.length();
case Integer i -> "An integer with value " + i;
case null -> "Null object";
default -> "Unexpected type";
}
}
Explanation
- Pattern Matching: In the switch expression, we are checking the type of
obj
. Instead of needing separateinstanceof
checks, we directly declare the type within the case. - Conciseness: By directly matching types, our code becomes easier to read and maintain.
For further details, check the official Java Documentation.
Common Pitfalls
1. Overlooking the Scope of Variables
One common mistake is to overlook the scope of variables declared in pattern matching cases. Variables defined in a case are only accessible within the scope of that case.
Example:
public String assess(Object obj) {
switch (obj) {
case String s -> {
// s is in scope here
return "String: " + s;
}
case Integer i -> {
// i is in scope here
return "Integer: " + i;
}
}
// s and i are NOT in scope here
return "Unrecognized type";
}
Tip: Make sure you utilize the variables as soon as they're defined within the case block.
2. Forgetting to Handle Null
Especially with reference types, failing to handle null values can lead to NullPointerExceptions. As seen in our first example, explicitly handling null can save debugging time.
Example:
public String evaluate(Object obj) {
switch (obj) {
case String s -> "String identified: " + s;
case Integer i -> "Integer identified: " + i;
case null -> "Received a null object"; // Important to check for null
default -> "Unknown type";
}
}
Meaning: Not accounting for null could lead to unexpected application behaviors.
3. Ignoring the Default Case
Omitting a default case might lead to a failure of the program logic since not all scenarios are accounted for. It's essential to have a catch-all case to ensure your code handles unexpected types gracefully.
Example:
public String classify(Object obj) {
switch (obj) {
case String s -> "This is a string";
case Integer i -> "This is an integer";
default -> "Unrecognized type"; // Safety net
}
}
4. Using Fall-Through Cases
In some scenarios, developers accustomed to traditional switch statements may mistakenly write fall-through cases. Pattern matching does not support fall-through in the manner classic switch statements do.
public String identify(Object obj) {
switch (obj) {
case String s:
case Integer i: // This will give a compile-time error
return "This is either a String or an Integer";
default:
return "Not a String or Integer";
}
}
Solution: Always explicitly separate cases when using pattern matching.
5. Overcomplex Patterns
While pattern matching allows for complex conditions, overusing this feature can lead to less readable code. Aim for clarity and simplicity.
Example of Overcomplexity:
public String complexIdentify(Object obj) {
switch (obj) {
case String s when s.length() > 10 -> "Long String";
case Integer i when i > 100 -> "Large Integer";
// This complexity could be simplified into individual cases or methods
default -> "Unrecognized type or condition";
}
}
Recommendation: If pattern conditions become too convoluted, consider breaking them into different methods for maintainability.
Best Practices
- Always Include the Default Case: Make sure to handle unexpected types.
- Use Clear Case Statements: Avoid overly complex expressions within your switch statements.
- Maintain Variable Scope: Understand that case variables are scoped only within their respective cases.
- Test for Null Values: Ensure your code gracefully handles null inputs.
- Make Use of Comments: When necessary, comment on non-obvious logic or patterns you implement.
Final Considerations
With Java 17's pattern matching for switch, developers have a powerful tool at their disposal for more robust and readable code. However, alongside these benefits come certain pitfalls that require careful consideration.
By being mindful of variable scope, handling nulls, remembering default cases, avoiding fall-through cases, and simplifying complex patterns, you can take full advantage of this feature without falling into common traps.
Equipped with this knowledge, you will not only enhance the robustness of your code but also ensure it remains maintainable and elegant. Happy coding!
For more insights on Java 17 features and best practices, feel free to explore additional resources linked below:
- Java SE Documentation
- Effective Java, 3rd Edition
- Java Magazine