Mastering Inline Class Properties: A Java Developer's Guide
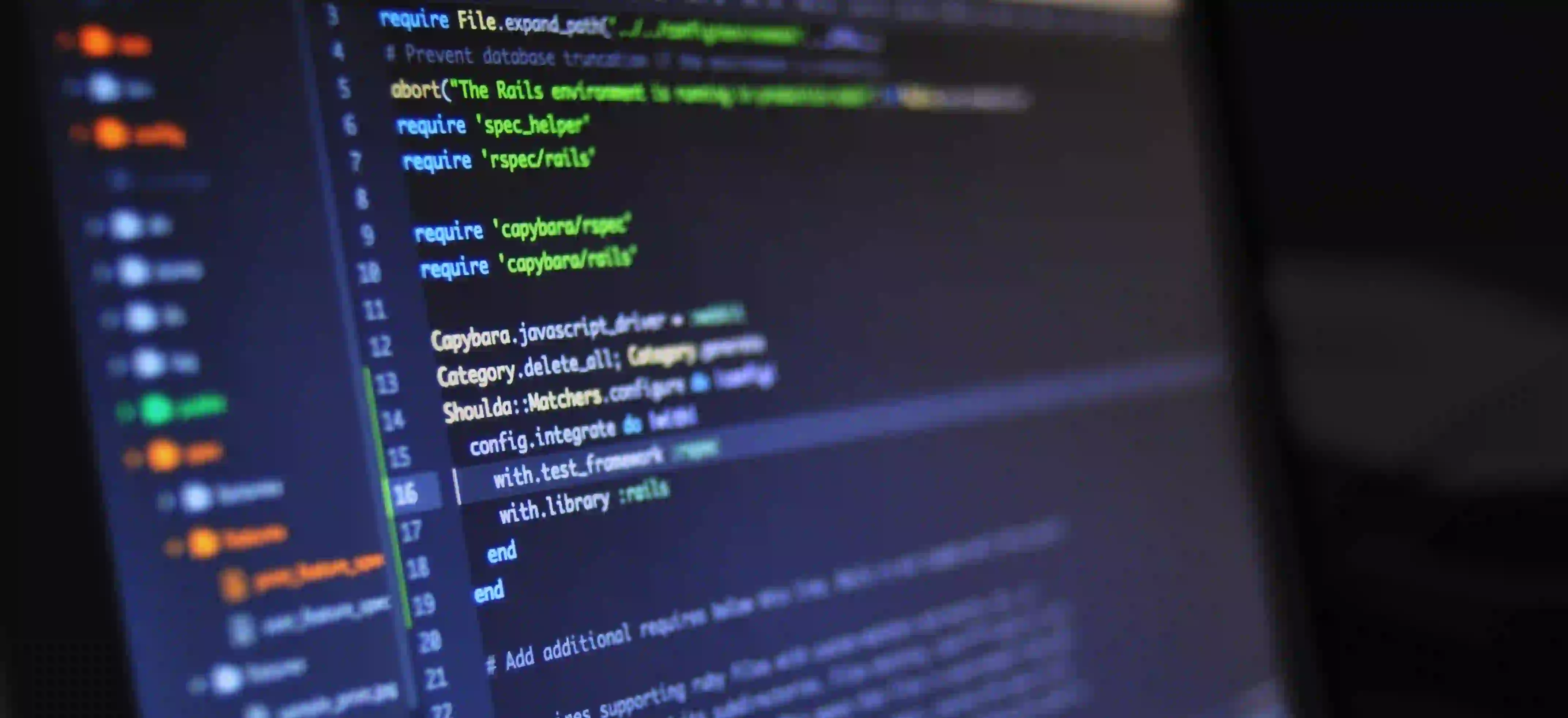
Mastering Inline Class Properties: A Java Developer's Guide
In modern Java development, creating clean and efficient code is a top priority. One of the powerful tools at our disposal is the use of inline class properties. Inline properties let developers define the properties of a class directly in a streamlined manner, enhancing readability and maintainability. In this post, we will explore inline class properties, how to implement them, and review best practices that can help elevate your Java coding skills.
What Are Inline Class Properties?
Inline class properties are attributes defined directly within the class definition, often using the new features introduced in recent Java versions. They allow developers to eliminate boilerplate code, making the Java programming experience cleaner and more enjoyable.
One notable addition to Java is the introduction of record types in Java 14, which allows developers to define classes in a more compact way while still maintaining all the essential features of a traditional class.
Key Benefits of Using Inline Class Properties
- Readability: Inline properties enhance code clarity.
- Less Boilerplate: Less code to write and manage means fewer opportunities for bugs.
- Immutable Data: Data defined in records are immutable by default, promoting clean architecture.
Getting Started with Inline Class Properties
A Simple Record Example
Consider a scenario where you need to represent a Point with x and y coordinates. You would typically define a class like this:
public class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
@Override
public String toString() {
return "Point(x=" + x + ", y=" + y + ")";
}
}
While this code works, it's unnecessarily verbose. With the introduction of records in Java, we can create a more elegant solution:
public record Point(int x, int y) {}
Why Use Records?
Using records helps in achieving concise representation of data structures. Here are the benefits illustrated above:
- Compact: The records syntax eliminates the need for boilerplate methods.
- Immutable by Default: Records don't allow modification of their properties once they are set, which is beneficial for thread safety and integrity.
- Automatic Methods: Java automatically generates
toString
,hashCode
, andequals
methods for you.
Advanced Use Cases of Inline Class Properties
Using Records with Additional Functionality
While records are great for simple data carriers, sometimes you need additional functionality. Let's enhance our Point
record by adding some methods:
public record Point(int x, int y) {
public double distanceFromOrigin() {
return Math.sqrt(x * x + y * y);
}
public String quadrant() {
if (x > 0 && y > 0) {
return "First Quadrant";
} else if (x < 0 && y > 0) {
return "Second Quadrant";
} else if (x < 0 && y < 0) {
return "Third Quadrant";
} else if (x > 0 && y < 0) {
return "Fourth Quadrant";
} else {
return "On an Axis";
}
}
}
Explanation of the Code Above
- Distance from Origin: This method calculates the distance from the origin (0,0) using the Pythagorean theorem.
- Identifying Quadrant: This method evaluates where the point lies in relation to the Cartesian plane.
You might want to refer to the Java Documentation for further exploration on records.
Using Inline Class Properties in Relation to Functional Programming
As Java evolves, integrating functional programming concepts into Java code is becoming increasingly common. Inline properties align perfectly with functional principles. Let's take a look at how using functional interfaces alongside records might look:
@FunctionalInterface
interface DistanceCalculator {
double calculate(Point p1, Point p2);
}
public class PointMain {
public static void main(String[] args) {
Point pointA = new Point(1, 2);
Point pointB = new Point(4, 6);
DistanceCalculator distanceCalculator = (p1, p2) -> {
return Math.sqrt(Math.pow(p2.x() - p1.x(), 2) + Math.pow(p2.y() - p1.y(), 2));
};
double distance = distanceCalculator.calculate(pointA, pointB);
System.out.println("Distance: " + distance);
}
}
Breakdown of the Above Code
- Functional Interface: We created a functional interface
DistanceCalculator
for the calculation of distance between two points. - Lambda Expression: Using a lambda expression, we implement the
calculate
method inline, thus achieving flexibility and clarity.
Best Practices
Here are some best practices for using inline class properties effectively:
- Use Records for Data Structures: When you have a class primarily used to hold data, consider using a record.
- Keep Methods Simple: If your record requires more complex methods, ensure they are easy to understand to keep the spirit of readability.
- Immutable Characteristics: Whenever possible, strive for immutability in your classes to promote safety and consistency.
Final Considerations
Mastering inline class properties in Java opens a world of convenience and elegance in your coding. By leveraging features like records and functional programming, you can write cleaner, more maintainable code while minimizing boilerplate.
As Java continues to evolve, embracing these features will not only enhance your development skills but also improve your projects' performance and reliability. For further reading, check out Effective Java and deepen your understanding of Java best practices.
Engage with the community, explore resources, and keep coding — the journey of mastering Java is as enjoyable as it is rewarding!