Could Every Object as an Array Solve Null Pointer Issues?
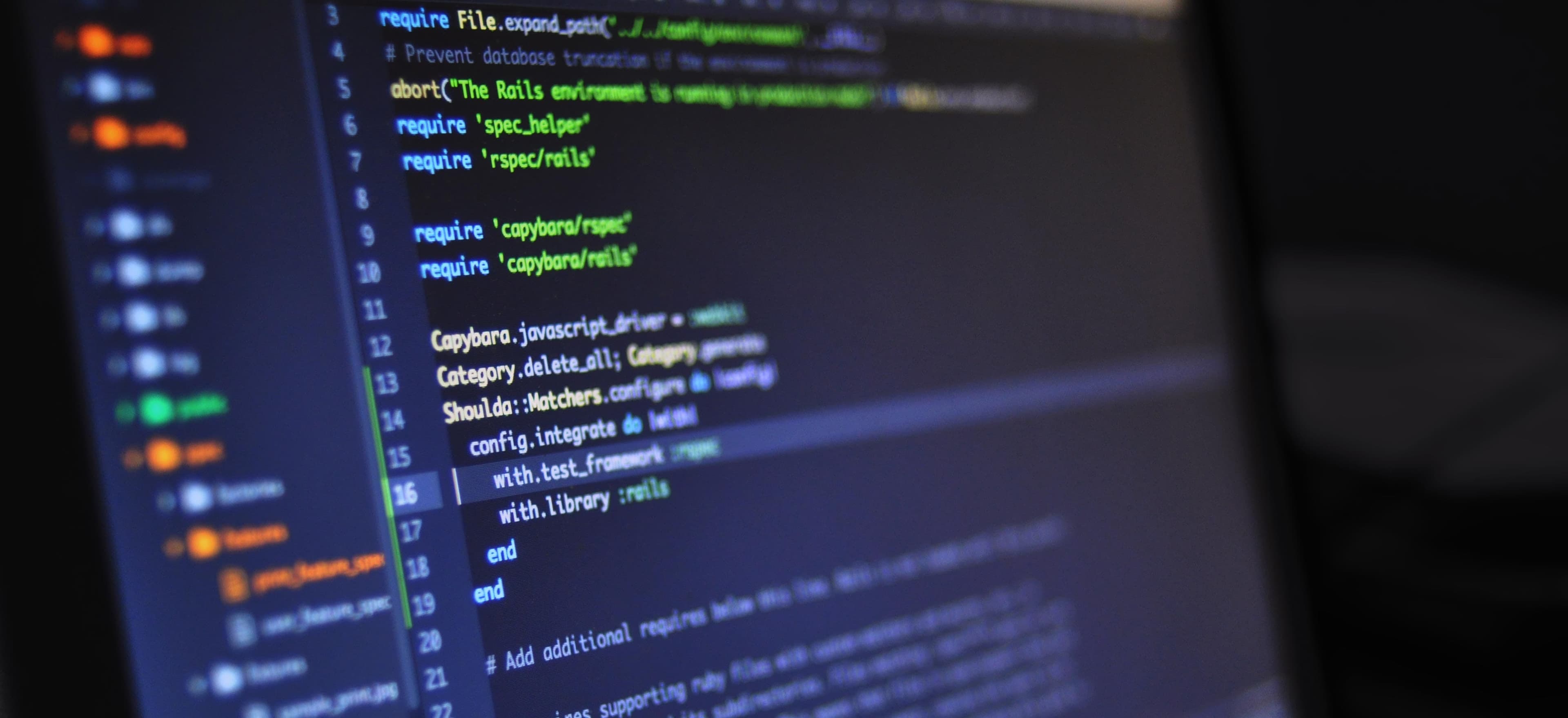
- Published on
Could Every Object as an Array Solve Null Pointer Issues?
Null Pointer Exceptions (NPEs) are one of the most common pitfalls developers face in Java programming. These exceptions occur when the program attempts to use an object reference that has not been initialized. NPEs lead to crashes and can cause significant downtime, frustrating both developers and users.
In this blog post, we'll explore a thought-provoking concept: what if every object in Java were treated as an array? Could this potentially eliminate the dreaded null pointer issue? Let’s dive deep into the mechanics of Java, array structures, and NPEs.
Understanding Null Pointer Exceptions
Before we tackle the solution, it’s essential to understand what causes null pointer exceptions in Java. Java is an object-oriented language, and objects are often expected to hold meaningful references to data. If you try to perform operations on a null object reference, the JVM raises a NullPointerException
.
Example of NPE
Consider the following code snippet:
public class NPEExample {
private String message;
public void printMessage() {
System.out.println(message.length());
}
public static void main(String[] args) {
NPEExample example = new NPEExample();
example.printMessage(); // This will throw a NullPointerException.
}
}
In this example, since message
is never initialized, calling message.length()
leads to an NPE.
Arrays vs. Objects in Java
Java arrays are fundamentally different from standard object references. When you declare an array, Java initializes the elements to their default values, which reduces the chances of null references leading to NPEs.
Example of Default Initialization
Here's an example that highlights the default initialization of arrays:
public class ArrayExample {
public static void main(String[] args) {
String[] messages = new String[5]; // Initializes an array of size 5
System.out.println(messages[0]); // Outputs: null, instead of throwing a NPE.
// The length() method can safely be called like this:
if (messages[0] != null) {
System.out.println(messages[0].length());
} else {
System.out.println("No message available.");
}
}
}
In this case, since messages[0]
is initialized as null
, it doesn’t throw an NPE when accessed, allowing the code to handle this condition gracefully.
The Theoretical Shift: Treating Every Object as an Array
Concept Overview
The core idea here is that if every object were treated like an array, you could access individual elements without risking null pointer exceptions. This approach would inherently assign a default value or "dummy object" to every potential reference, considerably reducing the occurrence of NPEs.
Pros:
- Minimal NPEs: Minimal to no null references would exist.
- Default Values: Default initialization means safer operations and consistent access.
Cons:
- Performance Overhead: Creating an empty array instance for every object reference may lead to higher memory usage and performance eliminations.
- Semantic Differences: The meaning behind arrays and objects could become obscured, making code less readable.
Proposed Implementation
A Custom Object Wrapper
To illustrate how every object could behave like an array, let’s create a custom wrapper class that simulates this behavior. Using generics, we can store and manage objects while ensuring that null references are handled.
public class SafeArray<T> {
private T[] elements;
@SuppressWarnings("unchecked")
public SafeArray(int size) {
elements = (T[]) new Object[size]; // Create a safe array of objects
}
public T get(int index) {
return elements[index]; // Returns the element or null if not set
}
public void set(int index, T value) {
elements[index] = value;
}
public int size() {
return elements.length;
}
}
Explanation of the Code
- Generics: By making the class generic, we provide flexibility, allowing it to store any type of object.
- Empty Initialization: The
SafeArray
constructor initializes the array, setting each reference to null by default. - Getter and Setter: These methods provide access to the underlying array while keeping NPEs in check.
Usage of SafeArray
Here’s how you can use SafeArray
to avoid NPEs:
public class TestSafeArray {
public static void main(String[] args) {
SafeArray<String> safeMessages = new SafeArray<>(5);
// Safely setting a value
safeMessages.set(0, "Hello, World!");
// Using getter with null handling
String message = safeMessages.get(0);
if (message != null) {
System.out.println(message.length());
} else {
System.out.println("No message available.");
}
}
}
In this case, the SafeArray
successfully reduces the risk of null references while offering a structured way to access object data.
Naturally Linked Contexts
- How to Handle NullPointerException in Java: A detailed guide on managing null references in Java, providing alternative solutions to NPEs.
- Java Generics - A Complete Guide: A comprehensive resource explaining Java generics, which helps in creating flexible data structures like our
SafeArray
class.
To Wrap Things Up
While treating every object as an array in Java could theoretically mitigate null pointer issues, the practical implications draw a line. The significant increase in memory consumption and potential performance trade-offs may outweigh the benefits of reducing NPEs.
Nonetheless, combining the array concept with careful programming practices, as demonstrated with the SafeArray
class, can help devise a path toward safer code. Leveraging Java's existing features, such as Optional, also provides a robust strategy against null pointers.
By understanding the mechanics of JVM and effectively utilizing programming patterns, we can minimize null pointer issues and write cleaner, more elegant Java code.
Your Thoughts?
What strategies have you used to avoid null pointer exceptions in Java? Share your experiences below!