Common Spring MVC Form Handling Mistakes to Avoid
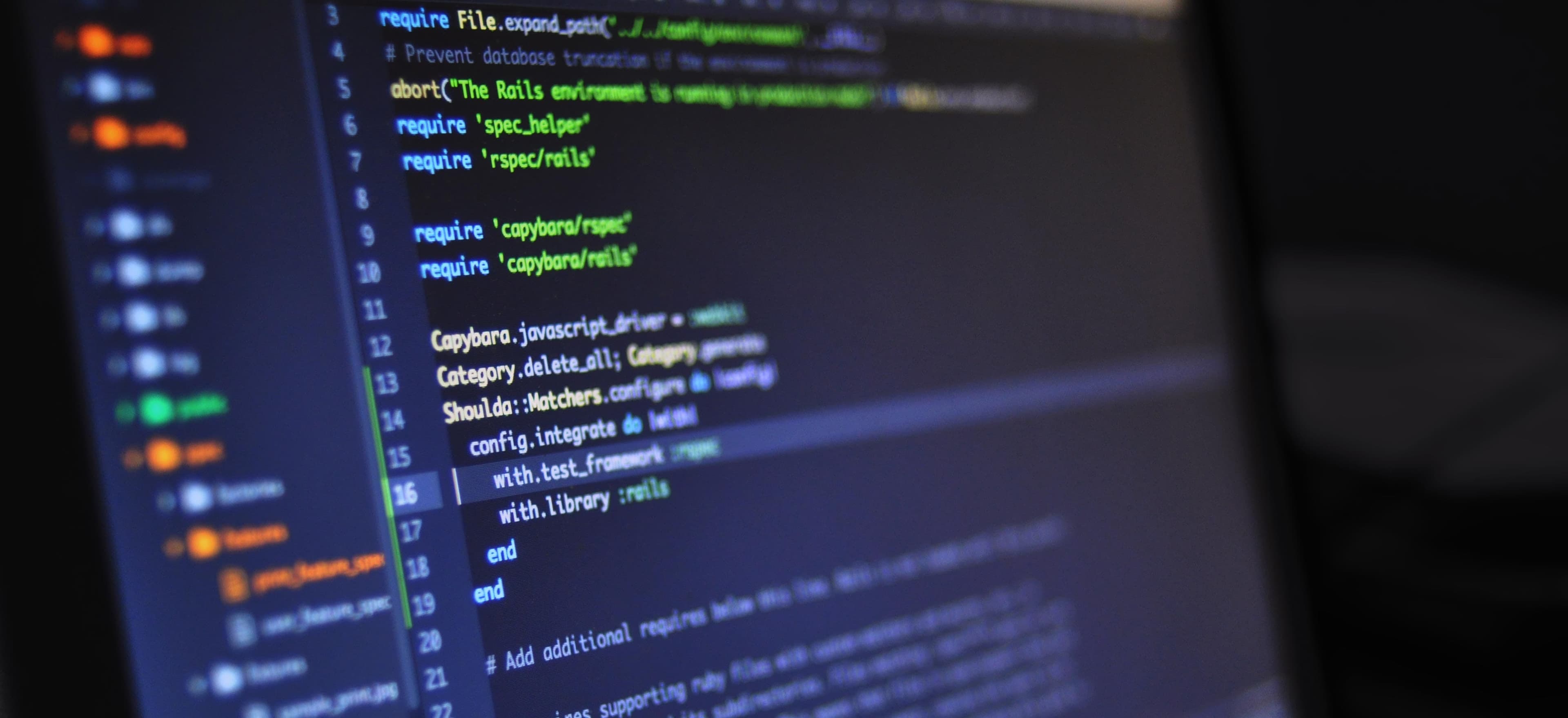
- Published on
Common Spring MVC Form Handling Mistakes to Avoid
Spring MVC is a powerful framework that simplifies Java web application development. Among its many features, form handling is a critical component. While working with forms in Spring MVC, developers often encounter pitfalls that can lead to bugs, inefficient code, and a poor user experience. In this article, we will explore common mistakes in Spring MVC form handling and how to avoid them. This discussion will provide valuable insights for both seasoned developers and newcomers to the framework.
Understanding Spring MVC Form Handling
Before diving into specific mistakes, let's briefly outline how form handling works in Spring MVC. The framework leverages a Model-View-Controller architecture that separates the application logic into three interconnected components:
- Model: Represents the data and business logic.
- View: Responsible for rendering the user interface.
- Controller: Handles user input and interacts with the model and the view.
Form data is typically submitted via POST requests, where the controller processes the input, validates it, and returns an appropriate view. Understanding this workflow is essential for efficient form handling.
The Basic Setup
Before we look at the common mistakes, let’s set up a simple form handling example to contextualize our discussion.
// User.java
package com.example.model;
import javax.validation.constraints.NotBlank;
public class User {
@NotBlank(message = "Name is mandatory")
private String name;
// Getters and Setters
}
// UserController.java
package com.example.controller;
import com.example.model.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import javax.validation.Valid;
@Controller
public class UserController {
@RequestMapping("/register")
public String showRegistrationForm(Model model) {
model.addAttribute("user", new User());
return "registration";
}
@PostMapping("/register")
public ModelAndView registerUser(@Valid User user, BindingResult result) {
if (result.hasErrors()) {
return new ModelAndView("registration", "user", user);
}
// Save the user (omitted for brevity)
return new ModelAndView("success", "user", user);
}
}
In this example, we have a simple registration form mapped to a user object. Now, let’s discuss the common mistakes associated with handling forms in Spring MVC.
Common Mistakes in Spring MVC Form Handling
1. Failing to Validate User Input
Mistake: Neglecting input validation can lead to security vulnerabilities and data corruption.
Why it's a problem: Without validation, you might end up saving invalid data to your database, or worse, open your application to attacks like SQL Injection.
Solution: Always use annotations from javax.validation
to enforce validation rules on your model. In our example, we use @NotBlank
.
@NotBlank(message = "Name is mandatory")
private String name;
2. Ignoring Binding Errors
Mistake: Not checking BindingResult
to handle form submission errors appropriately.
Why it's a problem: Leaving users unaware of what went wrong when they submit an incorrect form can frustrate them.
Solution: Implement error handling by checking BindingResult
. If there are errors, return to the original form with error messages.
if (result.hasErrors()) {
return new ModelAndView("registration", "user", user);
}
3. Hardcoding URLs
Mistake: Hardcoding URLs directly in controller methods can create maintenance headaches.
Why it's a problem: If you need to change your URL structure, you’d have to modify your code in multiple places, increasing the likelihood of introducing bugs.
Solution: Use @RequestMapping
with constants or Spring's URL building utilities.
@RequestMapping(value = "/register", method = RequestMethod.POST)
4. Not Using Model Attributes
Mistake: Forgetting to leverage @ModelAttribute
to bind request parameters to complex objects.
Why it's a problem: Without using @ModelAttribute
, binding forms to Java objects becomes cumbersome and error-prone.
Solution: Use @ModelAttribute
to automatically bind request parameters to your model.
@PostMapping("/register")
public String registerUser(@ModelAttribute User user, BindingResult result) {
// Handling logic
}
5. Not Returning to the Same View
Mistake: Returning a different view upon validation failure, losing form data.
Why it's a problem: Users will lose their input, leading to frustration and possible data entry redundancy.
Solution: Always return to the original view when there are binding errors, passing the user object back to repopulate the form.
if (result.hasErrors()) {
return new ModelAndView("registration", "user", user);
}
6. Using Deprecated or Obsolete Annotations
Mistake: Continuing to use deprecated methods or annotations.
Why it's a problem: This can make your codebase difficult to maintain and could lead to unexpected behavior as newer versions of Spring MVC are released.
Solution: Stay updated with the latest documentation and practices. Regularly refactor your code to keep it current with the framework.
7. Not Handling Exceptions Gracefully
Mistake: Failing to manage exceptions that may occur during form processing.
Why it's a problem: When an exception occurs and goes unhandled, it could leave users confused and present a bad user interface.
Solution: Utilize Spring's exception handling features such as @ControllerAdvice
to handle exceptions globally.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ModelAndView handleException(Exception e) {
ModelAndView modelAndView = new ModelAndView("error");
modelAndView.addObject("message", e.getMessage());
return modelAndView;
}
}
8. Lack of User Feedback
Mistake: Not providing feedback after form submission.
Why it's a problem: Users need to know whether their actions have been successful or if there were issues.
Solution: Use model attributes to convey messages after a successful action or validation failure.
if (result.hasErrors()) {
model.addAttribute("errorMessage", "There were validation errors!");
}
9. By-passing the MVC Pattern
Mistake: Mixing business logic with controller methods.
Why it's a problem: This defeats the purpose of the MVC architecture, making it hard to maintain, test, and manage your application.
Solution: Keep your controllers lean. Delegate business logic to services or separate classes, which can be tested independently.
@Service
public class UserService {
public void saveUser(User user) {
// Saving logic
}
}
A Final Look
While Spring MVC offers a robust framework for developing web applications, understanding and avoiding common mistakes in form handling is critical for building reliable, user-friendly applications. By validating inputs, managing binding errors, and following best practices, developers can significantly enhance the overall quality of their applications.
Implementing effective error handling and providing user feedback fosters a smooth user experience, which is essential in today's competitive landscape. Remember, maintaining clean code that adheres to the MVC principle not only helps your application scale but also makes your job as a developer much easier.
For more comprehensive information and official documentation, visit the Spring MVC documentation.
Stay diligent in your coding practices, and you will avoid many of the common pitfalls associated with Spring MVC form handling. Happy coding!