Common Mistakes When Using the Java Comparable Interface
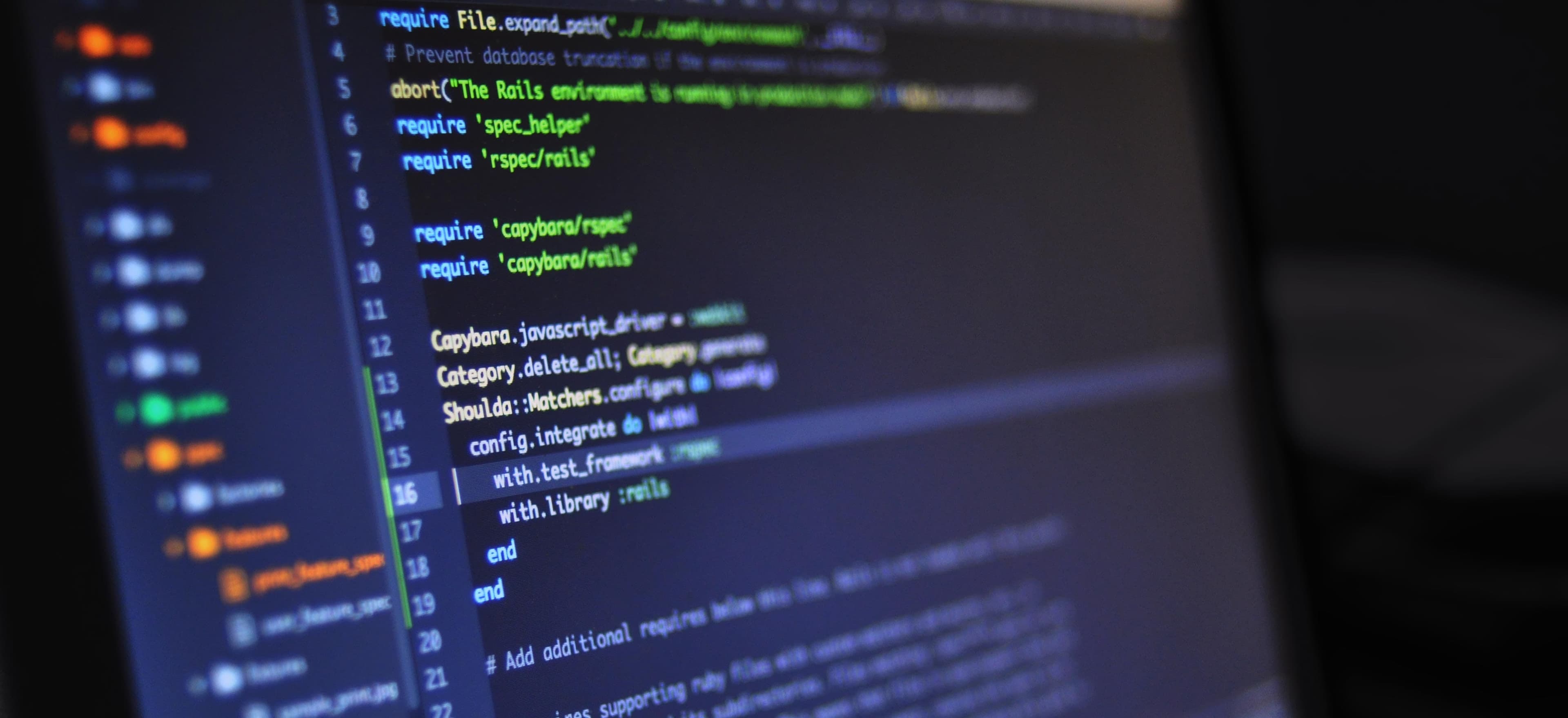
- Published on
Common Mistakes When Using the Java Comparable Interface
When working with Java, one of the most frequently encountered interfaces is the Comparable
interface. It plays a crucial role in sorting and ordering objects. Hence, understanding its intricacies can significantly enhance the quality of coding. Missteps in its implementation can lead to subtle bugs that are challenging to trace. This article elaborates on the common mistakes developers make when using the Comparable
interface and how to avoid them.
What is the Comparable Interface?
The Comparable
interface is designed to allow objects of a class to be compared with one another. This enables sorting of collections of that class using methods like Collections.sort()
or Arrays.sort()
. Here’s the declaration of the Comparable
interface:
public interface Comparable<T> {
int compareTo(T o);
}
The method compareTo
must return:
- A negative integer if
this
object is less than the specified object. - Zero if
this
object is equal to the specified object. - A positive integer if
this
object is greater than the specified object.
Common Mistakes
While implementing the Comparable
interface, developers often fall prey to several common mistakes. Let’s discuss them in detail.
1. Not Implementing Consistent Comparison Logic
One of the most critical mistakes is implementing inconsistent logic within the compareTo
method. The contract of the compareTo
method must adhere to the following rules:
- Transitivity: If
a.compareTo(b) > 0
andb.compareTo(c) > 0
, thena.compareTo(c) > 0
. - Anti-symmetry: If
a.compareTo(b) > 0
, thenb.compareTo(a) < 0
.
Failing to ensure these rules can lead to unpredictable behavior when sorting.
Example
class Person implements Comparable<Person> {
String name;
int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Person other) {
// Incorrect Implementation: Does not account for age when names are equal
return this.name.compareTo(other.name);
}
}
In the above code, if two Person
objects have the same name, their comparison based on age will not be consistent. To fix this:
@Override
public int compareTo(Person other) {
int nameComparison = this.name.compareTo(other.name);
if (nameComparison != 0) {
return nameComparison;
}
return Integer.compare(this.age, other.age);
}
By ensuring a secondary comparison condition (age), we uphold consistency.
2. Not Handling Null Values
Another common oversight is failing to handle null
values. If your objects can be null
, you must ensure compareTo
can handle such cases gracefully. Unchecked null
comparison may lead to NullPointerException
.
Example
@Override
public int compareTo(Person other) {
return this.name.compareTo(other.name); // May throw NPE if other is null
}
Fix
You can add a null check within your method:
@Override
public int compareTo(Person other) {
if (other == null) {
return 1; // Current object is greater than null
}
return this.name.compareTo(other.name);
}
3. Forgetting to Override equals
and hashCode
It is crucial to also override the equals
and hashCode
methods alongside compareTo
. When determining equality, the logic in compareTo
should align with that in equals
. Otherwise, your objects may not behave as expected when used in hash-based collections like HashMap
.
Example
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof Person)) return false;
Person other = (Person) obj;
return this.name.equals(other.name) && this.age == other.age;
}
@Override
public int hashCode() {
return Objects.hash(name, age); // Consistent with equals
}
4. Ignoring the Comparable
Interface in Subclasses
If you have a class hierarchy, you may neglect to implement Comparable
in subclasses. This oversight can break polymorphism and limit the flexibility of your code.
Example
class Employee extends Person {
String position;
public Employee(String name, int age, String position) {
super(name, age);
this.position = position;
}
// Potential oversight: Not overriding compareTo
}
Fix
Override compareTo
in subclass:
@Override
public int compareTo(Person other) {
// Call to super for primary comparison
int comparison = super.compareTo(other);
if (comparison != 0) {
return comparison;
}
return this.position.compareTo(((Employee) other).position);
}
5. Not Following the Natural Order Principle
The natural order of your objects should logically correspond to their comparison method. Many beginners implement custom orders which can be confusing.
Example
@Override
public int compareTo(Person other) {
return Integer.compare(this.age, other.age); // Age is a poor natural order; consider names
}
The natural ordering should be based on what makes sense for the application domain. For a Person
, perhaps names or ages could both serve well. Choose wisely based on your application’s context.
Final Thoughts
Implementing the Comparable
interface can significantly enhance your Java projects, but it comes with its set of pitfalls. By avoiding these common mistakes—such as inconsistent comparison logic, neglecting null handling, failing to override equals
and hashCode
, ignoring subclasses, and not adhering to the natural order—you’re more likely to implement robust and reliable classes.
For further reading, you may find the following resources helpful:
By adhering to best practices, you will ensure that your implementations are comprehensive and efficient, resulting in better performance and fewer bugs. Happy coding!