Resolving Data Node Failures in Hadoop on Mesos Framework
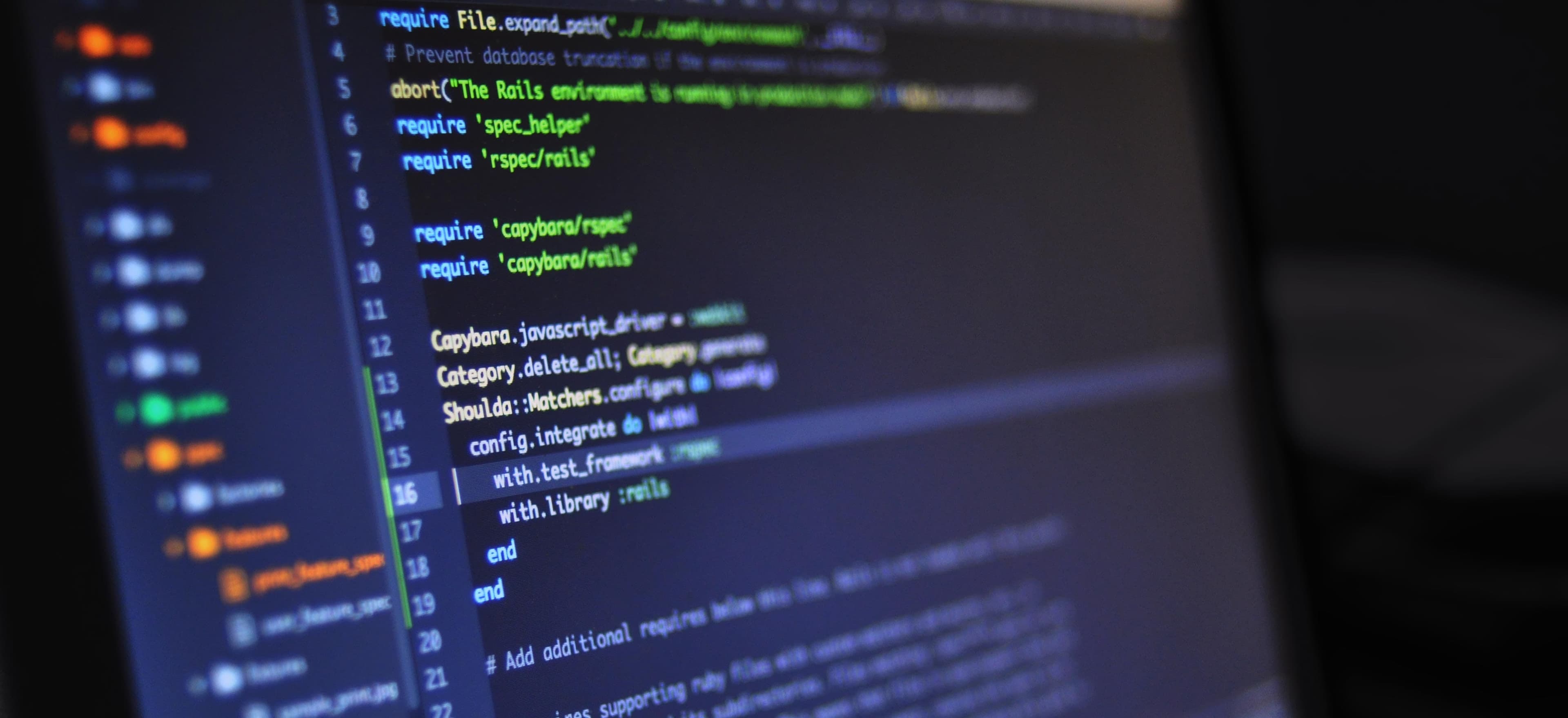
- Published on
Resolving Data Node Failures in Hadoop on Mesos Framework
In today's data-driven world, big data technologies like Hadoop are essential. Combine Hadoop with Apache Mesos, a cluster manager, and you have a powerful environment for managing resources across frameworks. However, as with any distributed system, failures are inevitable. One common issue that arises is the failure of Data Nodes in Hadoop clusters running on the Mesos framework. This blog post discusses how to effectively resolve these failures.
Understanding the Basics
First, let's clarify the technologies at play:
-
Hadoop: A software framework that allows for the distributed processing of large data sets across clusters of computers using simple programming models.
-
Mesos: A cluster manager that provides efficient resource isolation and sharing across distributed applications or frameworks, enabling resource optimization.
Why Use Mesos with Hadoop?
Running Hadoop on Mesos allows for better resource allocation among multiple frameworks, enabling a more efficient use of resources. However, this added complexity can lead to unique challenges, particularly around node failures.
What Happens When a Data Node Fails?
A Data Node failure in Hadoop typically leads to loss of data access for clients. This situation can have cascading effects, including:
- Job Failures: MapReduce jobs may fail if they depend on data residing on the failed node.
- Data Loss: If not configured with high availability, this can lead to permanent data loss.
Detecting Data Node Failures
Mesos and Hadoop offer built-in mechanisms for monitoring and detecting failures. Hadoop uses heartbeats to check the health of Data Nodes. If a Data Node does not send a heartbeat signal within a specified timeframe, it is marked as failed.
// Sample Java code to simulate heartbeat mechanism
public class Heartbeat {
private boolean isAlive;
public void sendHeartbeat() {
isAlive = true;
}
public void checkHealth() {
if (!isAlive) {
System.out.println("Data Node is down!");
// Logic to handle failure
}
isAlive = false; // Reset for next check
}
}
This code snippet illustrates a basic concept of heartbeats in a Data Node. It simulates the sending of a heartbeat and checking the health of the node.
Recovery Strategies for Data Node Failures
1. Automatic Recovery using Replication
Hadoop's built-in replication feature allows for recovery from Data Node failures. By default, Hadoop replicates data blocks across multiple Data Nodes. When a Data Node fails, Hadoop can automatically redirect requests to replicas on other active nodes.
// Sample code for fetching replicated data
public class DataRetriever {
public void fetchData(String blockId) {
List<DataNode> replicatedNodes = getReplicatedNodes(blockId);
for (DataNode node : replicatedNodes) {
if (node.isAlive()) {
node.sendData(blockId);
return; // Successfully retrieved
}
}
System.out.println("All replicas are unavailable");
}
}
Here, we demonstrate how to request data from replicated nodes, ensuring that even if one node goes down, the data can still be retrieved from others.
2. Utilizing Mesos for Faster Resource Recovery
Mesos aids in the rapid reallocation of resources when a Data Node fails. When a failure is detected, tasks previously assigned to the failed node can be quickly reallocated to other healthy nodes.
// Sample code for reallocating task in Mesos
public class TaskAllocator {
public void allocateTask(String failedNodeId, String taskId) {
DataNode newNode = findAvailableNode();
if (newNode != null) {
newNode.allocate(taskId);
} else {
System.out.println("No available nodes to allocate the task.");
}
}
private DataNode findAvailableNode() {
// Logic to find a new Data Node
return new DataNode(); // For demonstration
}
}
In this code snippet, the TaskAllocator
class reallocates a task from a failed Data Node to a healthy node seamlessly.
3. Enhancing Fault Tolerance with High Availability
To minimize the risk of data loss, enabling High Availability (HA) is crucial. In a Hadoop ecosystem, this means configuring NameNodes in an active/passive setup, allowing for immediate failover.
- Configure HDFS HA.
- Use Zookeeper for managing failover.
Best Practices for Handling Data Node Failures
Regular Monitoring
Monitor your Hadoop cluster continuously using tools like Apache Ambari or Cloudera Manager. They provide real-time insights into the system's health and help in proactive problem resolution.
Set Up Alerts
Implement an alerting mechanism that notifies administrators upon Data Node failure. Using tools like Nagios or Grafana can help in this.
Implement a Backup Strategy
Hold regular backups of critical data. Having a robust backup and restore strategy ensures that you can recover your data quickly in the event of a catastrophic failure.
Resources for Further Reading
- Apache Hadoop Documentation - The official documentation is a great resource for deep diving into Hadoop configurations and features.
- Apache Mesos Documentation - Understanding how to optimize resource allocation in Mesos is pivotal to ensuring system resilience.
Final Thoughts
Understanding how to resolve Data Node failures in a Hadoop environment running on Mesos is crucial for ensuring high availability and data integrity. Implement various strategies such as replication, resource reallocation, and high availability configuration to mitigate the effects of node failures. By monitoring the cluster continually and setting alerts, administrators can ensure a robust and resilient data infrastructure.
Checkout our other articles