Why Simple Testing Fails to Prevent Critical Errors
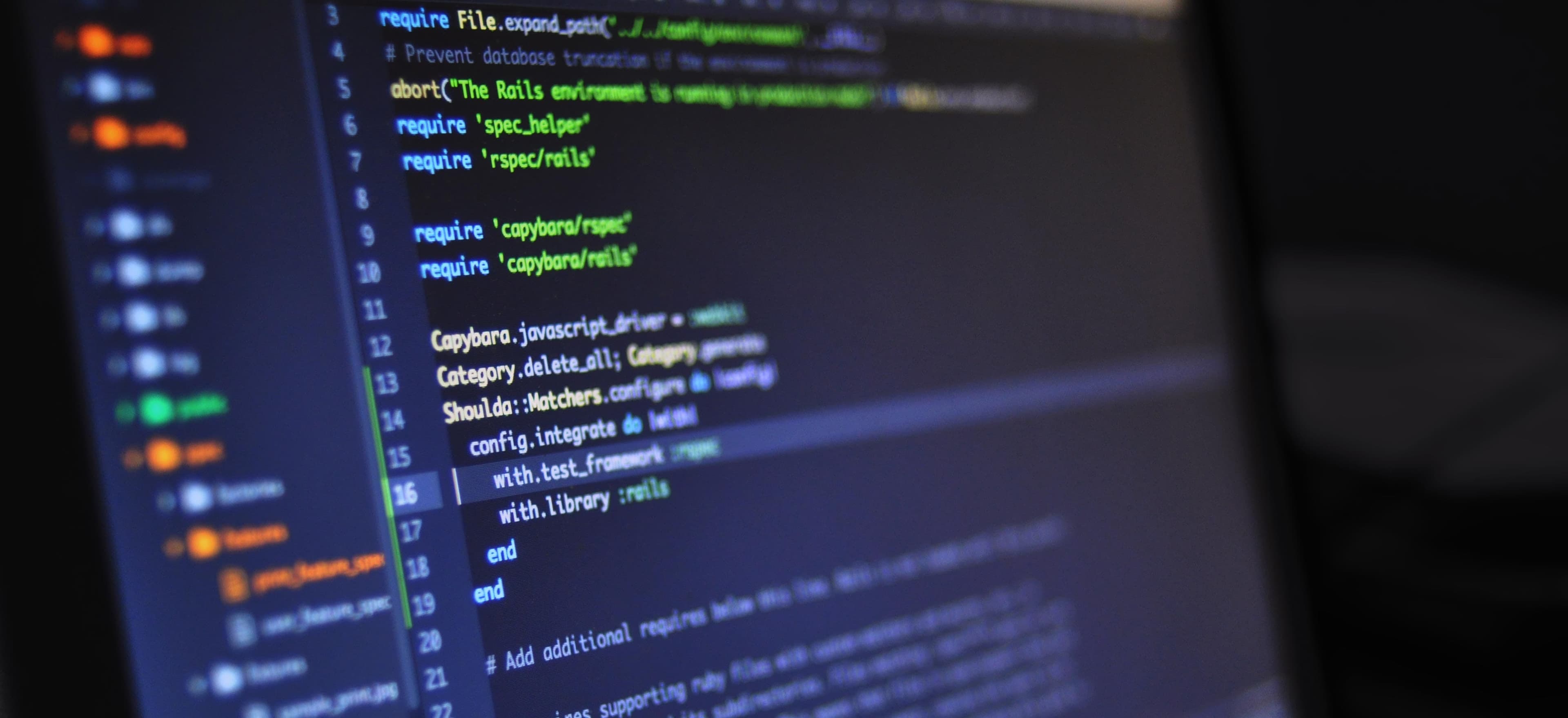
- Published on
Why Simple Testing Fails to Prevent Critical Errors
Software testing is an essential part of the software development lifecycle. It aims to identify issues before production, ensuring that applications run smoothly and meet user requirements. However, simple testing often fails to prevent critical errors, leading to significant consequences. In this blog post, we will explore the failures of basic testing methodologies, examine common pitfalls, and suggest effective strategies to mitigate risks.
Understanding Simple Testing
Simple testing methods usually consist of straightforward unit tests or manual testing procedures focused on a limited set of functions. These tests typically look for obvious bugs or performance issues but fall short in critical areas. Here are a few common characteristics of simple testing:
- Limited Scope: Simple tests often focus on individual components rather than their interaction with the whole system.
- Lack of Depth: Basic tests verify if a function produces the expected result, but they may overlook edge cases or error conditions.
- Manual Execution: Relying heavily on human testers can introduce bias and inconsistency in test execution.
The Pitfalls of Simple Testing
While the importance of testing cannot be overstated, simple testing approaches pose several risks:
1. Incomplete Test Coverage
One major drawback of simple testing is incomplete test coverage. A common analogy is testing an iceberg—what is visible above the water may differ greatly from what lies beneath.
Consider the following snippet:
public int divide(int a, int b) {
return a / b;
}
At first glance, one might think this function is straightforward, but it lacks error handling. If b
equals zero, an ArithmeticException
will be thrown. A simple test might check whether divide(10, 2)
returns 5
, but it won't account for the edge case of division by zero. Comprehensive testing methods, such as boundary testing, would catch this critical error.
2. Faulty Assumptions
Human testers often rely on their understanding of the software and make assumptions regarding its functionality. These assumptions may lead to overlooking important scenarios. For instance:
public String getUserAge(int userId) {
// Assume valid userId always exists
return userDatabase.findById(userId).getAge();
}
While the above code appears to function correctly, if an invalid userId
is passed, it may throw a NullPointerException
. A simple test checking getUserAge(1)
may not expose this flaw unless documented thoroughly.
3. Lack of Integration Testing
Integration testing is vital in ensuring that different modules of the software work seamlessly. Neglecting integration tests can result in unexpected behavior during runtime as components fail to communicate correctly.
Consider how a logging functionality might be impacted by changes to a core service:
public void logPayment(Payment payment) {
Logger.log(payment.getTransactionId(), payment.getAmount());
}
Simple tests may only verify that a log entry is being created, without testing for valid inputs from the Payment
class. If the payment object is modified or moved, existing simple tests may not fail, leading to silent failures that are only noticed in production.
Strategies for Improving Testing Approaches
To mitigate the risks associated with simple testing, developers can employ more robust strategies:
1. Emphasize Test Automation
Automated testing tools can save time and enhance consistency, reliability, and coverage. Tools like JUnit for Java allow developers to create several tests efficiently.
Example of a simple JUnit test:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testDivision() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.divide(10, 2));
}
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
Calculator calculator = new Calculator();
calculator.divide(10, 0); // Expect an ArithmeticException
}
}
This code highlights the need to consider edge cases. Automated tests ensure comprehensive coverage and can easily be incorporated into the CI/CD pipeline for real-time feedback.
2. Adopt Behavior-Driven Development (BDD)
Behavior-Driven Development focuses on the behavior of the application, linking tests closely to user requirements. Frameworks like Cucumber allow for natural language specifications that enhance collaboration among developers and non-technical stakeholders.
Consider a BDD example:
Feature: Payment Processing
Scenario: User makes a payment
Given the user has sufficient balance
When the user initiates a payment
Then the transaction should succeed
This style promotes understanding of user expectations and helps ensure that critical user paths are adequately tested.
3. Conduct Code Reviews
Incorporate code reviews as a domain for identifying potential pitfalls in testing. Engaging multiple perspectives can help uncover edge cases that a single developer might overlook. Encouraging cross-team visibility often leads to better test coverage and more robust testing strategies.
4. Stress Testing and Load Testing
For applications that must handle high traffic, stress testing and load testing are essential. These tests simulate high loads or unpredictable data inputs to identify weaknesses. Tools like Apache JMeter or Gatling can provide insights into the application's behavior under stress.
Example of a simple load test in JMeter:
- Setup: Create a Thread Group configured to simulate multiple concurrent users.
- Test Plan: Send requests to the application and analyze the response times and resource utilization.
5. Ensure Continuous Testing and Feedback
Incorporating continuous integration and continuous delivery (CI/CD) pipelines allows for automated testing and immediate feedback. As changes are made, automated test suites run, ensuring that critical errors are caught before deployment.
A Final Look
While simple testing has its place in software development, relying solely on it can lead to missed critical errors that significantly impact user experience and functionality. By adopting a comprehensive and automated testing strategy, including unit tests, integration tests, BDD, and load tests, development teams can enhance the robustness of their software and catch errors early in the development cycle.
Additional Resources
For those looking to deepen their understanding of testing methodologies, consider reading:
- The Art of Software Testing by Glenford Myers
- Test-Driven Development: By Example by Kent Beck
By taking a proactive stance on testing, we can reduce the risk of critical errors and deliver high-quality software products that meet both user needs and business objectives.
Checkout our other articles