Mastering Quarkus: Overcoming Common Beginner Challenges
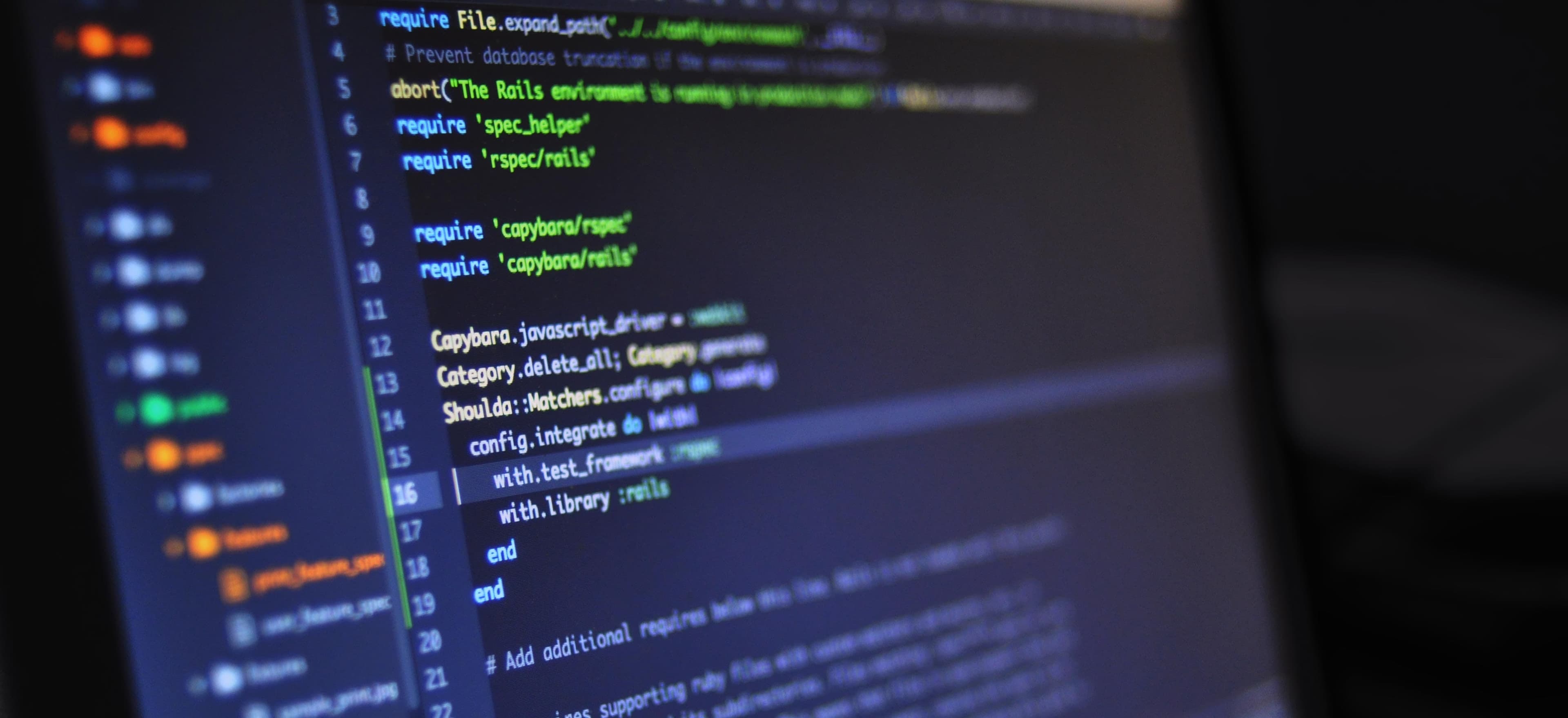
- Published on
Mastering Quarkus: Overcoming Common Beginner Challenges
Quarkus is a cutting-edge Java framework tailored for Kubernetes and cloud-native environments. With its rapid startup time, low memory footprint, and developer-friendly features, it has become increasingly popular among Java developers. However, beginners often face challenges when starting with Quarkus. In this blog post, we will explore some of the common hurdles new users encounter and how to overcome them effectively.
Table of Contents
- Understanding Quarkus: What Makes It Unique?
- Setting Up Your Development Environment
- Creating Your First Quarkus Application
- Working with Extensions
- Testing in Quarkus
- Optimizing Application Performance
- Conclusion
Understanding Quarkus: What Makes It Unique?
Quarkus excels in numerous areas, fundamentally altering how we develop Java applications for cloud environments. To appreciate its power, let's examine some of its core features:
- Fast Startup Time: Quarkus applications can start in milliseconds, which is crucial for modern microservices architecture.
- Low Memory Consumption: Quarkus runs Java in a more resource-efficient manner, making it ideal for serverless environments.
- Developer Experience: With live reload capabilities, Quarkus enhances productivity by allowing developers to see changes instantly.
As an alternative to traditional Java frameworks, Quarkus adopts a "supersonic subatomic Java" philosophy, which results in quicker iterations and enhanced efficiency.
Setting Up Your Development Environment
Setting up your development environment for Quarkus involves several steps. Here’s how to get started:
Prerequisites
- Java 11 or higher: Download and install from the OpenJDK website.
- Maven: While Quarkus supports Gradle, we will use Maven in this article. Install Maven from Apache Maven.
- Quarkus CLI (optional, but recommended): Install via SDKMAN with the command
sdk install quarkus
.
Verifying Your Installation
Run the following commands to verify that Java and Maven are installed correctly:
java -version
mvn -version
Ensure both commands return the correct version numbers.
Creating Your First Quarkus Application
Creating a Quarkus application is straightforward. Use the Quarkus command line interface or Maven directly. Here’s how to do it with Maven:
mvn io.quarkus:quarkus-maven-plugin:2.x.x:create \
-DprojectGroupId=com.example \
-DprojectArtifactId=hello-quarkus \
-DclassName="com.example.GreetingResource" \
-Dpath="/hello"
This command does the following:
- projectGroupId: Defines the package name.
- projectArtifactId: Specifies the project name.
- className: Generates a resource class for handling HTTP requests.
- path: Defines the endpoint for your REST service.
Running the Application
Navigate into your project directory and start the application:
cd hello-quarkus
./mvnw compile quarkus:dev
You can access your hello endpoint at http://localhost:8080/hello
, which returns a JSON response.
Here's a sample of the code generated:
package com.example;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class GreetingResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello, Quarkus!";
}
}
Why This Code Matters
- @Path: Annotates the class, defining the endpoint for the service.
- @GET: Specifies that this method is triggered for HTTP GET requests.
- @Produces: Indicates what media type the method will return.
This structure lays the foundation for building RESTful APIs effectively.
Working with Extensions
Quarkus comes with many extensions, enhancing functionality significantly. Extensions are reusable packages that add specific capabilities to your application.
Adding Extensions
To add a REST client, for instance, you would run:
./mvnw quarkus:add-extension -Dextensions="resteasy"
Extensions can also be added via the Quarkus CLI or directly to your pom.xml
.
Using Extensions
Once you've added the required extensions, refer to their specific documentation for implementation guidance. For example, the RESTEasy extension allows you to build powerful RESTful web applications quickly.
Testing in Quarkus
Testing is an integral part of the development lifecycle. Quarkus elegantly integrates testing capabilities.
Writing Unit Tests
Quarkus supports both JUnit and Mockito. Here’s a simple example of a unit test for the above REST endpoint:
package com.example;
import io.quarkus.test.junit.NativeImageTest;
@NativeImageTest
public class GreetingResourceIT extends GreetingResourceTest {
// Execute the same tests but in native mode.
}
Why Testing Matters
Testing ensures that your application remains functional and allows you to catch bugs early. Quarkus simplifies this process while providing flexibility in testing frameworks.
Optimizing Application Performance
Performance optimization involves numerous strategies, from JVM tuning to using native images.
Enabling Native Images
Quarkus can compile your application into a native image, allowing it to run instantly with minimal resource consumption. To enable this, you need GraalVM:
-
Download and install GraalVM.
-
Install the Native Image component:
gu install native-image
-
Build a native image with:
./mvnw package -Pnative
Native images significantly reduce startup time and memory usage.
The Last Word
Mastering Quarkus is a rewarding journey, albeit with its challenges. By understanding its unique features, properly setting up your environment, and leveraging its powerful extensions and testing capabilities, you can advance your skills in building cloud-native Java applications. The potential of Quarkus opens new avenues for creating efficient and scalable applications that meet modern demands.
For more information and resources on Quarkus, visit the official Quarkus documentation. Happy coding!
Checkout our other articles