Why Microservices Fail: Common Pitfalls to Avoid
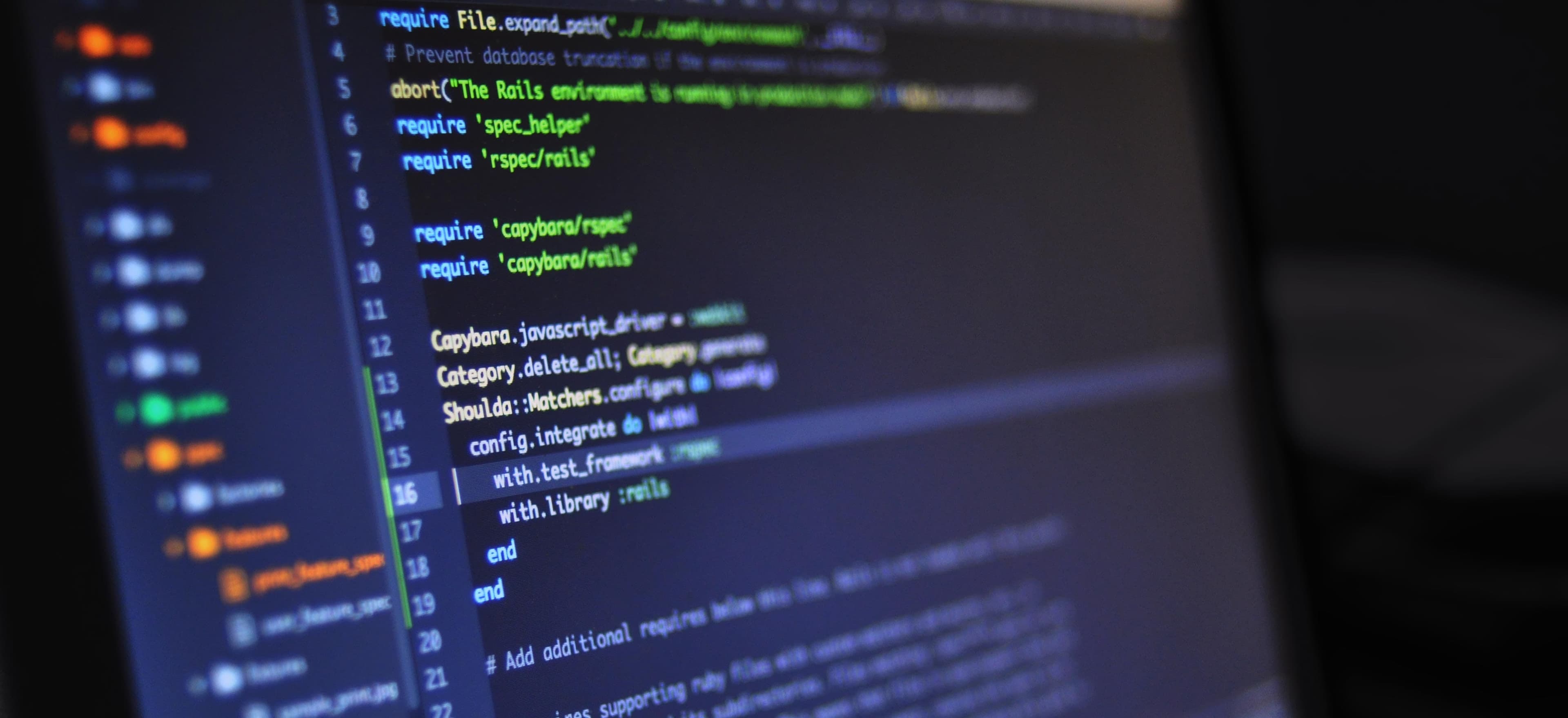
- Published on
Why Microservices Fail: Common Pitfalls to Avoid
The transition to microservices has become a leading strategy for organizations aiming to enhance flexibility and scalability. However, along this enticing journey, many teams experience setbacks and failures. This blog post explores common pitfalls that lead to microservices failure, emphasizing why they occur and how to avoid them.
Understanding Microservices
Before diving into the pitfalls, it's essential to grasp what microservices entail. Microservices architecture is an approach to software development where applications are broken down into small, independent modules. Each service performs a discrete business function and communicates with others through APIs. This structure is celebrated for its scalability, maintainability, and agile deployment.
!Microservices Architecture
Common Pitfalls of Microservices
1. Lack of Proper Service Boundaries
One of the most prevalent mistakes organizations make is poorly defining service boundaries. Services should be fine-grained enough to maintain their functionality without being excessively fragmented.
Why This Happens:
- Teams may not understand domain-driven design (DDD), leading to an overlap in responsibilities among services.
- They may be motivated by technological aspects instead of the business value.
How to Avoid It:
Use DDD principles and clearly define potential services based on business functionalities. Each service should revolve around a single business capability.
Example:
// User Service
public class UserService {
public User getUserDetails(String userId) {
// Logic to fetch user details from the database
}
}
// Order Service
public class OrderService {
public Order createOrder(User user, List<Item> items) {
// Logic to create an order for the user
}
}
In the example above, the UserService
and OrderService
have well-defined responsibilities, making it easy for each service to evolve independently.
2. Ignoring the Complexity of Distributed Systems
While microservices offer many advantages, they also introduce the complexities inherent in distributed systems, including data consistency issues and network latency.
Why This Happens:
- Teams may underestimate the challenges accompanying network calls.
- Lack of robust infrastructure can complicate service interactions.
How to Avoid It:
Implement strong communication strategies and design patterns such as Circuit Breakers and Event Sourcing to manage these complexities.
Example:
// Using Circuit Breaker
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@HystrixCommand(fallbackMethod = "defaultUser")
public User getUser(String userId) {
return userRepository.findById(userId).orElseThrow(() -> new UserNotFoundException());
}
public User defaultUser(String userId) {
return new User("default", "default@domain.com");
}
}
In this code snippet, the Circuit Breaker ensures the system remains robust in case of failures while maintaining modularity.
3. Overengineering**
Some teams get carried away with the high potential of microservices, leading to overengineering. They may focus too much on implementing complex orchestration or intricate infrastructure setups without the need.
Why This Happens:
- The allure of cutting-edge technologies drives teams to complicate their architecture.
- They may lose sight of project goals amidst the microservices structure.
How to Avoid It:
Prioritize simplicity and stick to the essentials. Begin with a few well-defined services, and only extend complexity as the project requirements demand.
4. Data Management Challenges
Transitioning to microservices often disrupts how teams manage data. It typically leads to each service maintaining its database, which raises data inconsistency issues.
Why This Happens:
- Teams may not have a solid strategy for data ownership and sharing.
- They may rely on synchronous data sharing, causing bottlenecks.
How to Avoid It:
Implement patterns such as Database per Service or API Composition. Each microservice can manage its database and share data via API.
Example:
// API Composition
@RestController
public class UserOrderController {
@Autowired
private UserService userService;
@Autowired
private OrderService orderService;
@GetMapping("/user/{userId}/orders")
public UserOrdersDTO getUserOrders(@PathVariable String userId) {
User user = userService.getUser(userId);
List<Order> orders = orderService.getUserOrders(userId);
return new UserOrdersDTO(user, orders);
}
}
Here, UserOrderController
consolidates data from multiple services, allowing for an efficient data-gathering strategy without creating tight couplings among services.
5. Failing to Monitor Services
A critical element in managing microservices is monitoring performance and health metrics. Businesses that neglect this do not realize when systems fail until it is too late.
Why This Happens:
- Teams may lack the tools or systems in place to monitor service performance effectively.
- Not prioritizing observability in the selection of technologies.
How to Avoid It:
Employ monitoring tools like Prometheus, Grafana, or ELK Stack to keep track of service performance. Ensure logging and tracing are integral parts of your microservices platform.
6. Insufficient Team Skills
Microservices require diverse skill sets within teams, including cloud technologies, CI/CD practices, and API management. An absence of these competencies can hinder performance.
Why This Happens:
- Organizations may transition to microservices without adequate training.
- There may be a reliance on existing teams instead of investing in specialized training.
How to Avoid It:
Foster a culture of continuous learning. Encourage upskilling to enhance team competencies in cloud services, container orchestration (such as Kubernetes), and reactive programming.
Key Takeaways
Although the power of microservices can dramatically propel your organization forward, navigating this landscape carefully is essential to avoid common pitfalls. Stick to well-defined service boundaries, embrace data management principles, and continuously monitor service health.
Incorporate observability with modern tooling, support your team with adequate training, and most importantly, foster a culture that promotes learning. By avoiding these pitfalls, your transition to microservices can be successful, leading to more robust, scalable, and adaptive software solutions.
For more insights into microservices, check out the articles on Martin Fowler's Blog, and Microservices.io.
By recognizing and overcoming these challenges, you can harness the true potential that microservices offer. Happy coding!
Checkout our other articles