Boost MongoDB Performance: Choosing the Right Index Type
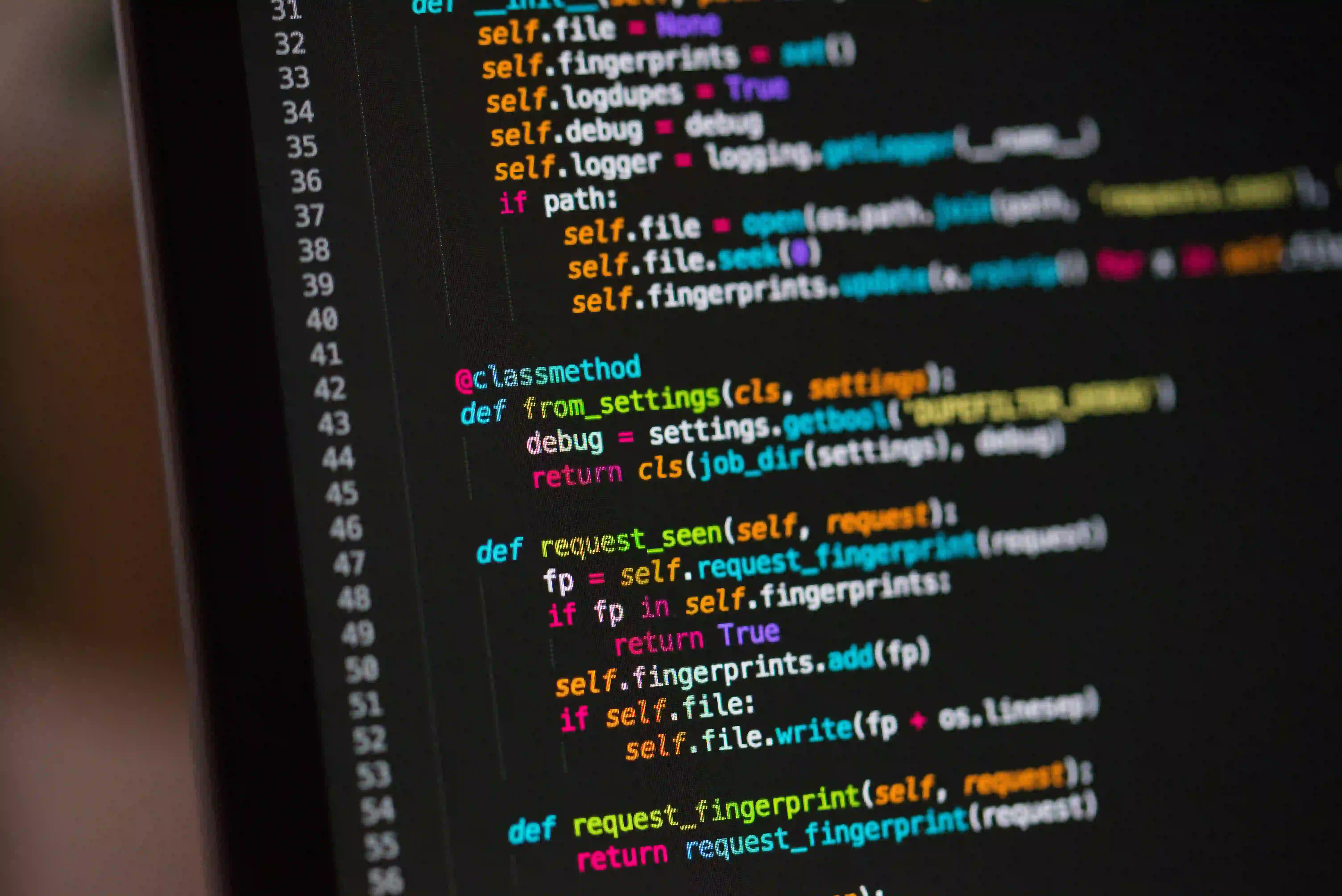
Boost MongoDB Performance: Choosing the Right Index Type
When it comes to working with MongoDB, one of the most critical factors influencing application performance is the way you index your collections. Proper indexing can transform your application's speed, reducing query times from minutes to milliseconds. In this blog post, we’ll delve into the various types of indexes available in MongoDB, how to choose the right one for your use case, and practical examples to illustrate these concepts.
Understanding Indexes in MongoDB
Indexes in MongoDB are akin to indexes in a book: they allow the database to find information quickly without scanning every document. Otherwise, as your dataset grows, queries will become slower. The basic premise is that an index is a data structure that holds a small portion of the data set’s key values and corresponding document references.
Benefits of Using Indexes
-
Improved Query Performance: By providing a sorted structure, indexes allow MongoDB to optimize query execution paths.
-
Minimized I/O Operations: When queries can be answered using indexes rather than full scans, disk I/O operations decrease, resulting in improved performance.
-
Enhanced Sort Performance: Queries that sort results can leverage indexes to minimize sorting overhead.
-
Filtering: Indexes help MongoDB filter documents more efficiently based on field values.
Types of Indexes
In MongoDB, there are several types of indexes you can create:
- Single Field Indexes
- Compound Indexes
- Multikey Indexes
- Geospatial Indexes
- Text Indexes
- Hashed Indexes
- Wildcard Indexes
1. Single Field Indexes
A single-field index is the most basic type of index. It can be established on a single field of a document.
db.collection.createIndex({ fieldName: 1 }) // 1 for ascending, -1 for descending
Why Use It? When your queries frequently filter or sort by a specific field, using a single-field index can significantly enhance performance.
2. Compound Indexes
As the name suggests, a compound index involves multiple fields. It is particularly useful for queries that state conditions on more than one field.
db.collection.createIndex({ fieldA: 1, fieldB: -1 });
Why Use It? If your application requires filtering or sorting based on combinations of fields, compound indexes can optimize these operations by allowing multiple fields to be considered in a single index.
3. Multikey Indexes
When your documents contain arrays, you should utilize multikey indexes. MongoDB automatically creates multikey indexes when you index a field that contains an array.
db.collection.createIndex({ tags: 1 });
Why Use It? Without a multikey index, MongoDB would have to scan every document in the collection rather than jump directly to the relevant ones.
4. Geospatial Indexes
If your application requires location-based queries, geospatial indexes are essential.
db.collection.createIndex({ location: '2dsphere' });
Why Use It? These indexes are tailored for geographic data, allowing for efficient querying based on geo-coordinates.
5. Text Indexes
Text indexes are designed for searching string content within your documents.
db.collection.createIndex({ content: "text" });
Why Use It? Full-text search capabilities enable users to find relevant documents based on keywords more efficiently than without indexes.
6. Hashed Indexes
Hashed indexes compute a hash of the indexed field's value. They are optimal for equality checks.
db.collection.createIndex({ userId: "hashed" });
Why Use It? If you need to ensure that documents are evenly distributed across shards in a sharded cluster, hashed indexes come into play.
7. Wildcard Indexes
Wildcard indexes allow you to index fields in documents with varying schemas or deeply nested objects.
db.collection.createIndex({ "$**": 1 });
Why Use It? This is particularly useful when dealing with documents that can have many different fields and structures.
Choosing the Right Index Type
When it comes to choosing the right index type, consider the following steps:
-
Analyze Query Patterns: Understand how your application interacts with your MongoDB collections. Use the MongoDB Atlas Performance Advisor to identify queries that could benefit from indexing.
-
Consider Read vs. Write Performance: While indexes improve read operations, they do add overhead during write operations. Consider your application's needs.
-
Use Compound Indexes Wisely: Ensure you include fields in the correct order based on your query patterns. The order can drastically change how effectively the index is utilized.
-
Limit Index Counts: A plethora of indexes can lead to increased maintenance overhead. Aim to create indexes judiciously based on query needs.
-
Test and Monitor: Continuously monitor your application's performance and adjust indexing strategy by analyzing the
explain()
output.
Example Scenario
Let’s put this knowledge into perspective with a real-world example. Assume you manage a blog application where posts have various fields like title
, author
, tags
, and createdAt
. The following queries might come up frequently:
- Find posts by title.
- Get posts by author and sort by creation date.
- Search posts by tags.
For these use cases, the following indexes can be beneficial:
// Single field index for title searches
db.posts.createIndex({ title: "text" });
// Compound index for author and creation date
db.posts.createIndex({ author: 1, createdAt: -1 });
// Multikey index for tag searches
db.posts.createIndex({ tags: 1 });
Monitoring and Adjusting Indexes
After implementing the indexes, you should monitor how your queries perform using the db.collection.find().explain("executionStats")
command. This will help you determine if the indexes are being used effectively or if further adjustments are required.
To Wrap Things Up
Choosing the right index type in MongoDB can dramatically enhance performance, optimizing the way queries are processed. By understanding the distinct advantages of each index type and applying them to your specific use case, you can achieve efficient data retrieval and make your application snappier.
Remember always to assess the impact of your indexing strategy on both read and write performance. As best practices, continually monitor and adapt your database indexing as your application scales or evolves.
For more information on MongoDB indexing, consider checking the official MongoDB documentation on Indexes. Your application’s performance might just depend on the right indexes!