Common Pitfalls of Android Geofencing with Google Maps
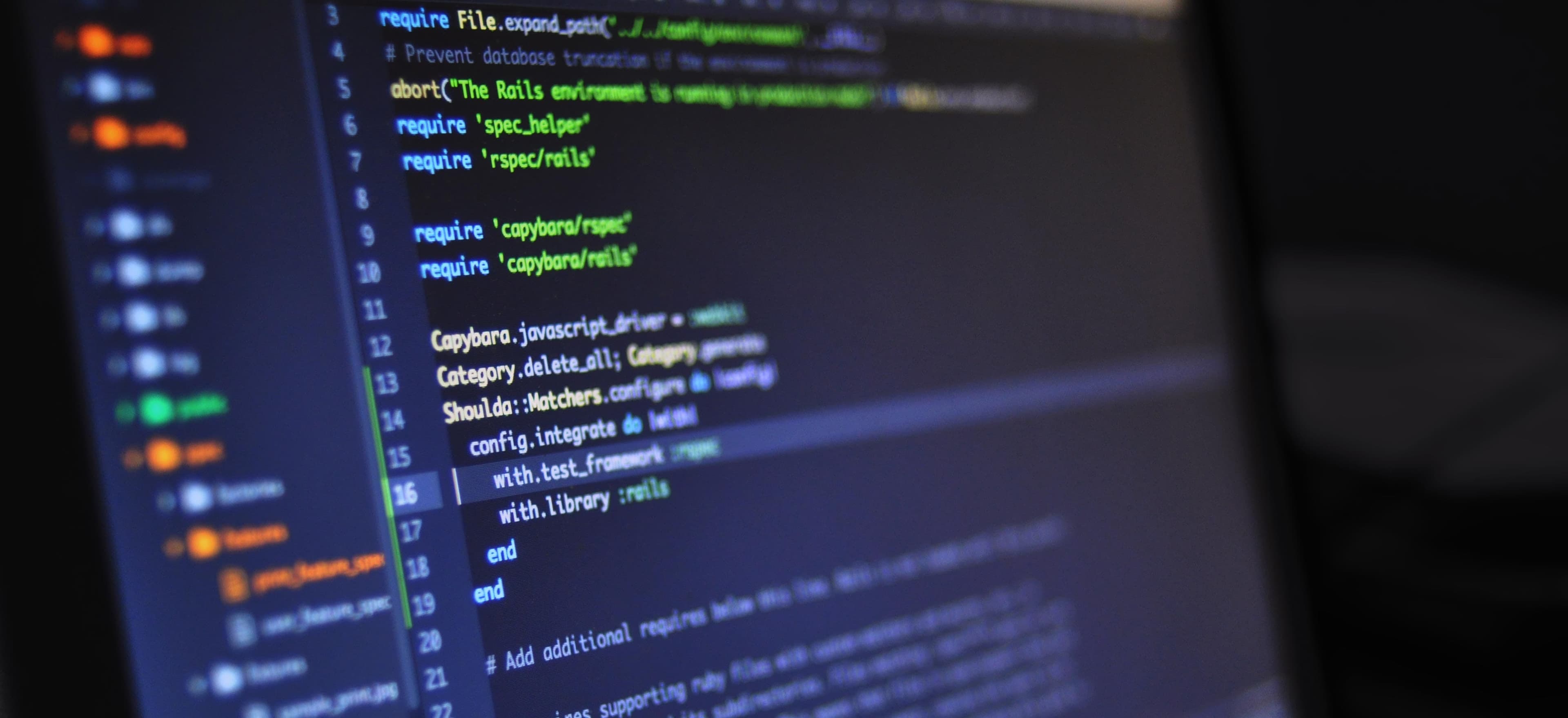
- Published on
Common Pitfalls of Android Geofencing with Google Maps
Geofencing is the technology that allows developers to create virtual boundaries around a real-world geographic area. In Android development, integrating geofencing with Google Maps can be a powerful feature that enhances user experiences in location-based applications. However, developers often encounter challenges during implementation. In this blog post, we’ll explore some common pitfalls associated with Android geofencing when using Google Maps and how to avoid them.
Understanding Geofencing
Before diving into the pitfalls, let's take a moment to understand what geofencing is. Geofencing uses GPS, RFID, Wi-Fi, or cellular data to establish a virtual perimeter around a specified area. When a device enters or exits this area, specific actions are triggered. Examples of applications include location-triggered push notifications, tracking delivery vehicles, and checking in users to locations.
Key Components of Geofencing in Android
To effectively use geofencing in your Android application, it’s crucial to identify the building blocks:
- Geofence: The actual geographical boundary defined by coordinates, radius, and transition type (enter, exit, dwell).
- Geofencing API: A part of Google Play services that handles the location updates and determines when a geofence is crossed.
- Location Services: To retrieve the user’s current location, so the geofencing API works correctly.
- BroadcastReceiver: This listens for geofence transition events and performs the necessary actions (e.g., notifications).
Common Pitfalls
1. Overlapping Geofences
One of the most frequent pitfalls is creating overlapping geofences. When two or more geofences overlap, the system may not accurately report transitions, leading to erratic behavior.
Solution: When designing your geofences, ensure they have non-overlapping radii when possible. If overlaps are necessary, be prepared to handle multiple transitions when entering or exiting a geofence.
2. Relying Solely on GPS
While GPS provides accurate location data, it can suffer from latency and accuracy issues, especially in urban environments with tall buildings (urban canyons). Relying solely on GPS can result in poor geofence triggering.
Solution: Use a combination of location providers. In addition to GPS, leverage Wi-Fi and cellular networks for a more robust location reading. Here’s how to implement it:
LocationRequest locationRequest = LocationRequest.create();
locationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
locationRequest.setInterval(10000); // 10 seconds
locationRequest.setFastestInterval(5000); // 5 seconds
The PRIORITY_BALANCED_POWER_ACCURACY
allows the device to utilize Wi-Fi and mobile networks under poor GPS conditions, ensuring better accuracy in location readings.
3. Inadequate Permissions
Android's permission model has tightened over the years, especially concerning location data access. Many developers overlook the importance of checking and requesting the appropriate permissions, leading to app failures.
Solution: Ensure that you implement runtime permission checks in your app. For example:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION},
LOCATION_PERMISSION_REQUEST_CODE);
}
This code snippet checks if the permission is granted and then requests it if not. Always handle the user's response to permit or deny access.
4. Ignoring Battery Optimization
Geofencing operations can significantly drain the device's battery, particularly if frequent updates are made. Failing to consider battery optimization measures can lead to poor user retention.
Solution: Implement battery-efficient geofencing by utilizing the Geofence
class's setExpirationDuration
method to define how long the geofence should remain active. For example:
Geofence geofence = new Geofence.Builder()
.setRequestId("unique_id")
.setCircularRegion(latitude, longitude, radius)
.setExpirationDuration(Geofence.NEVER_EXPIRE) // Consider using a finite duration after testing
.setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER | Geofence.GEOFENCE_TRANSITION_EXIT)
.build();
Using expiration helps alleviate unnecessary battery drains.
5. Lack of User Experience Consideration
User experience should never take a backseat to technical implementation. Users are more likely to disable location services if they are bombarded with notifications without context.
Solution: Ensure your notifications are relevant and contextual. For instance, if a user enters a shopping district, send them a coupon. The notification should be meaningful. Test the user experience with real-world scenarios to enhance notification relevance.
6. Failing to Register Listeners for Geofence Transitions
Another common mistake is neglecting to register a BroadcastReceiver
to listen for geofence transition events. When transitions occur, they won’t be captured unless properly implemented.
Solution: Register a BroadcastReceiver
correctly as follows:
private final GeofenceBroadcastReceiver geofenceReceiver = new GeofenceBroadcastReceiver();
@Override
protected void onStart() {
super.onStart();
LocalBroadcastManager.getInstance(this).registerReceiver(geofenceReceiver,
new IntentFilter(GeofenceTransitionsJobIntentService.ACTION_GEOFENCE_TRANSITION));
}
@Override
protected void onStop() {
super.onStop();
LocalBroadcastManager.getInstance(this).unregisterReceiver(geofenceReceiver);
}
Make certain that you manage the lifecycles of your receivers to prevent memory leaks.
7. Lack of Testing Across Different Conditions
Lastly, many developers skip extensive testing under various conditions, such as poor GPS signal, device sleep states, or different Android versions. This results in missed transitions and erratic behavior.
Solution: Test in various environments. Utilize emulators as well as physical devices. Make sure that your geofences trigger correctly under diverse scenarios like low battery, airplane mode, and various power-saving modes.
The Closing Argument
While implementing geofencing with Google Maps in Android can be rewarding, awareness of common pitfalls and best practices is essential. By understanding these challenges and their corresponding solutions, you can build a more robust geofencing capability in your applications. Building efficient geofences not only enhances user experience but also optimizes resource usage.
For further reading on Android development and geolocation, consider checking out the Android Developers Geofencing Documentation and Google Maps API documentation.
By avoiding these pitfalls, you can ensure your geofencing implementation shines in usability and reliability, making your application a valuable tool for users. Happy coding!