Overcoming Common Code Review Pitfalls for Developers
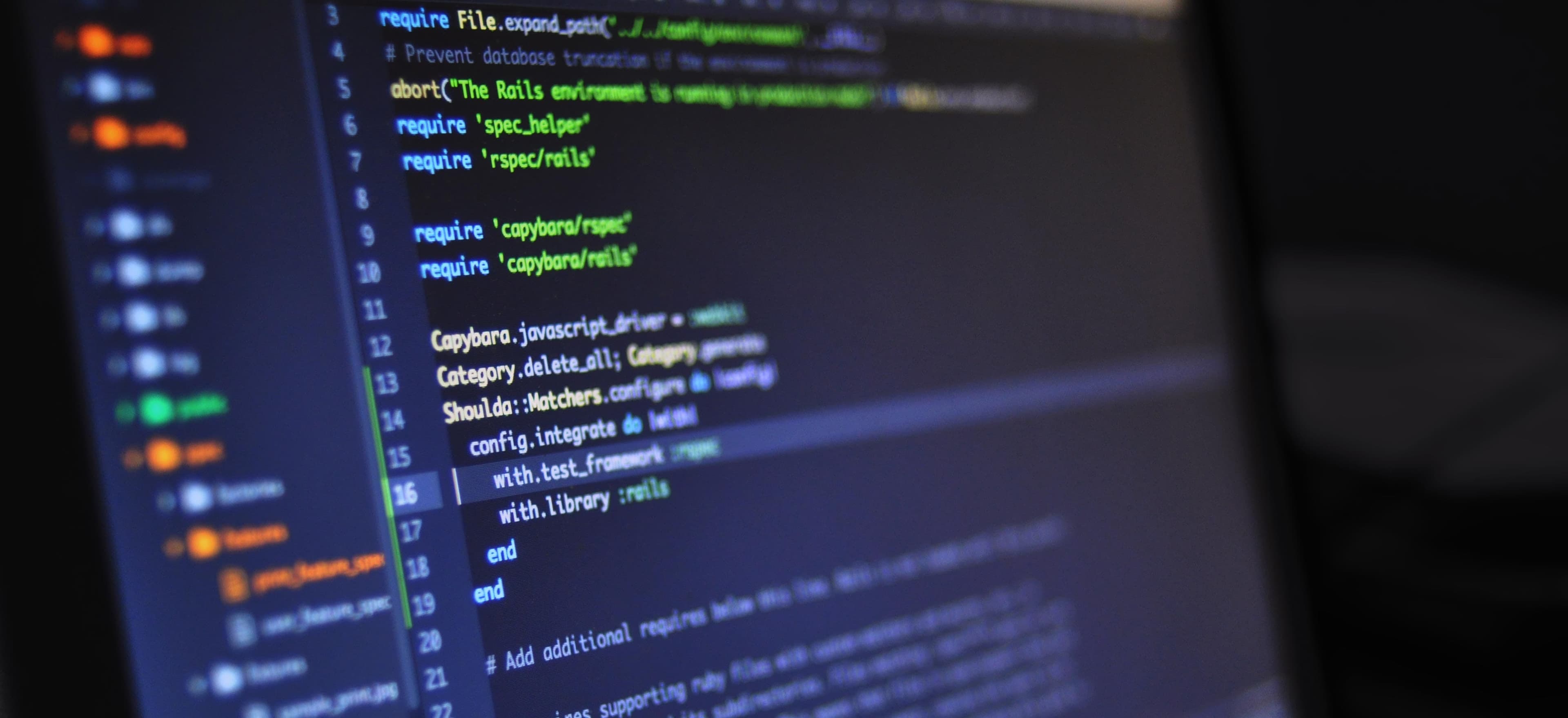
- Published on
Overcoming Common Code Review Pitfalls for Developers
Code reviews play a pivotal role in maintaining quality within software development. They not only ensure that code is clean and functional but also promote collaboration and shared knowledge among team members. However, both reviewers and developers can fall prey to common pitfalls during this crucial process. In this post, we'll explore some of these challenges as well as best practices for overcoming them.
Understanding the Importance of Code Reviews
Before diving into common pitfalls, it's essential to recognize why code reviews are so valuable. The primary reasons include:
- Improving Code Quality: Code reviews help identify bugs, enhance readability, and enforce coding standards.
- Knowledge Sharing: They enable team members to share insights and best practices, spreading knowledge throughout the team.
- Mentorship Opportunity: Experienced developers can guide newcomers, fostering growth and learning.
Despite these benefits, many developers struggle with the review process. Here are some common pitfalls and practical ways to address them.
Common Pitfalls in Code Reviews
1. Lack of Clear Guidelines
One major issue in code reviews is the absence of clear review guidelines. A review without a structured approach can feel haphazard and subjective, leading to confusion and frustration.
Solution:
Establish a well-defined set of coding guidelines and criteria for reviews. This can include specific standards for style, architecture, and testing practices.
Example: A simplified standard might look like this:
# Code Review Guidelines
1. Code must adhere to our style guide (see [this link](https://google.github.io/styleguide/jsguide.html) for reference).
2. Ensure all unit tests are present and passing.
3. Code should be modular and maintainable.
4. Follow the DRY principle (Don't Repeat Yourself).
2. Insufficient Context
Reviewers often lack adequate context about the code changes being submitted. Without a solid understanding, their feedback may be off the mark.
Solution:
Encourage developers to provide descriptive commit messages and, if necessary, supplementary documentation. Let them explain the reasons behind their changes.
Example of a Descriptive Commit Message:
Fix user authentication flow
- Refactored middleware to properly handle JWT tokens.
- Updated user model to include roles.
- Improved error handling for invalid tokens.
Including context allows reviewers to focus on critical aspects rather than getting lost in the details.
3. Focusing Only on Syntax
Many reviewers become too engrossed in syntax errors at the expense of exploring the broader logic and design of the code. While formatting is important, functionality and structure should remain the focal point.
Solution:
Shift the focus toward the architecture and logic of the code instead of just syntax. A good code review should evaluate the design choices made by the developer.
Example:
Instead of only pointing out a missing semicolon:
public String getUserName(User user) // Syntax Error: Missing Semicolon
{
return user.getName();
}
Engage in a discussion about:
- Is the user object correctly designed?
- Are there opportunities for refactoring?
4. Ignoring the Emotional Impact
Often, developers may take feedback too personally, leading to tension and resentment. Conversely, reviewers might overlook the impact of their critiques.
Solution:
Cultivate a supportive atmosphere that emphasizes constructive feedback, focusing on code rather than the coder. Encourage team members to frame comments positively.
Example Phrasing: Instead of saying, "This part of your code is incorrect," try: "Have you considered this approach? It may enhance performance."
5. Overloading Reviewers
Another common pitfall occurs when reviewers are overwhelmed with excessive code to review at once. This can result in overlooking critical issues.
Solution:
Limit the size of the code changes being reviewed. A good rule of thumb is to keep pull requests small enough to ensure that they can be fully assessed without causing fatigue.
Example: Encourage teams to submit smaller, more frequent pull requests as opposed to massive ones. This can be structured like:
# Commit Message
PR 1: Refactor Authentication Middleware (100 lines)
PR 2: Update User Role Implementation (50 lines)
PR 3: Add Tests for the Above Changes (30 lines)
6. Not Collaborating
Discussing code with peers can be tremendously beneficial, yet reviewers often work in isolation. This disconnection can lead to missed opportunities for collaboration.
Solution:
Promote collaborative reviews where developers discuss the code in real-time, perhaps even conducting pair programming sessions.
Example Practice: Regularly hold review sessions using tools that enable group discussions, like Zoom or even team-specific channels on Slack.
7. Rigid Review Policies
Some teams utilize overly rigid review policies that do not allow flexibility for different situations. This can stifle creativity and innovation.
Solution:
Adapt policies to suit the context of the project and team. A balanced approach that values both consistency and adaptability can be effective.
Example Adjustment: In situations of urgent bug fixes, allow expedited reviews rather than adhering to strict timelines.
A Final Look
Overcoming common pitfalls in code reviews is pivotal for enhancing team dynamics, improving code quality, and fostering a culture of continuous learning. By establishing clear guidelines, encouraging context, focusing on code quality rather than syntax, and fostering collaborative environments, teams can create a more productive and positive review process.
To further delve into best practices, check out resources like Martin Fowler's Refactoring and Code Complete by Steve McConnell. They offer valuable insights into maintaining code quality and the importance of collaborative coding practices.
Remember, a successful code review is a blend of art and science—both technical skills and interpersonal communication play a role in its effectiveness. Keep these tips in mind, and watch your team's productivity and morale soar.