Mastering Hibernate Logging: Common Pitfalls and Fixes
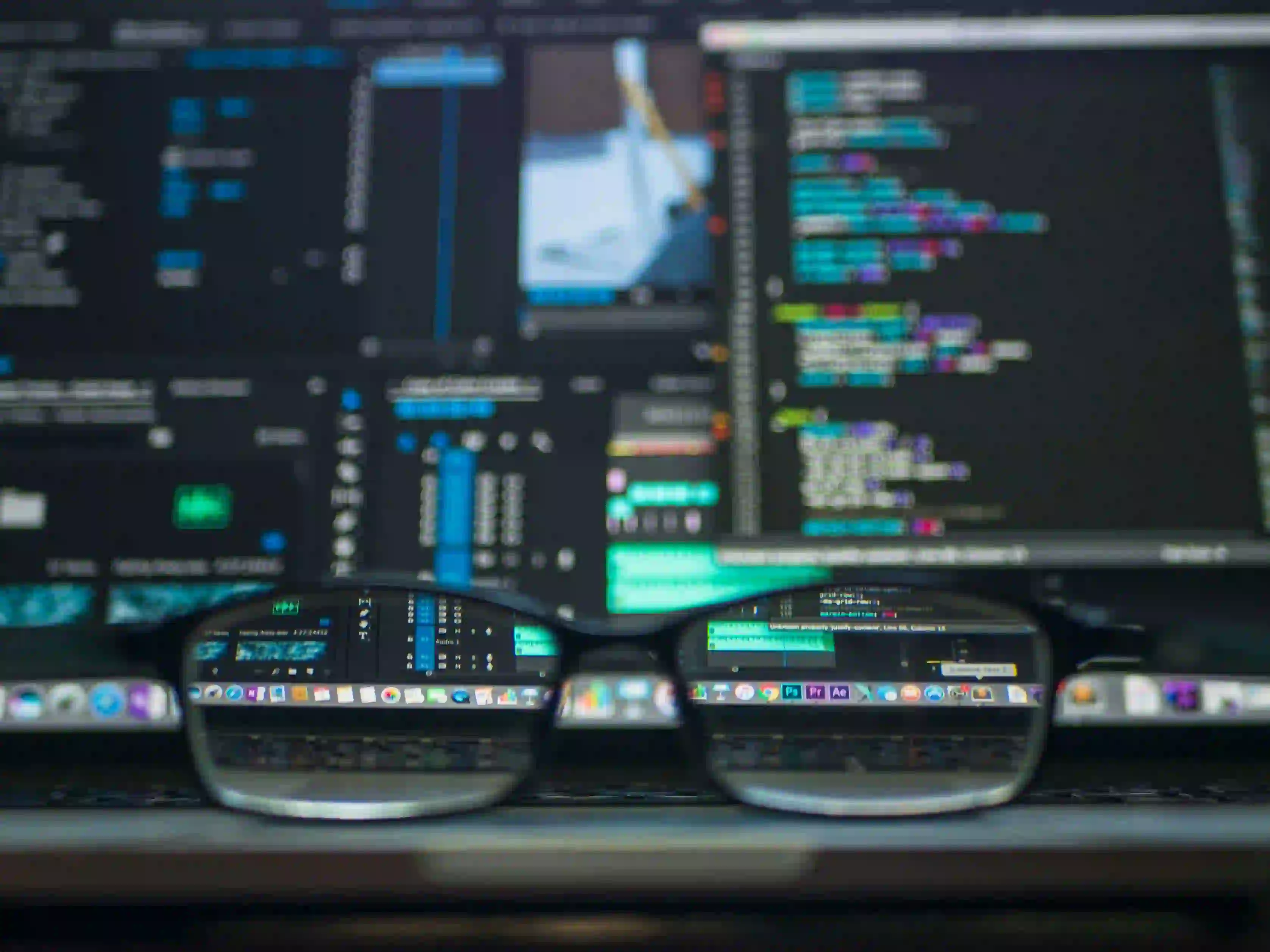
Mastering Hibernate Logging: Common Pitfalls and Fixes
Hibernate is a powerful ORM (Object-Relational Mapping) framework in Java that simplifies database operations. While its capabilities are extensive, many developers encounter challenges when implementing logging with Hibernate. In this blog post, we will explore common pitfalls in Hibernate logging and discuss effective fixes. By the end of this article, you will have a comprehensive understanding of how to set up and manage logging in Hibernate efficiently.
Why Logging Matters in Hibernate
Logging is crucial for monitoring the behavior of an application in real-time. In the context of Hibernate, it helps you:
- Troubleshoot Issues: Identify problems related to database queries and connection issues.
- Enhance Performance: Monitor SQL statements and optimize them if needed.
- Understand Application Flow: Get visibility into transactions and data handling.
For further reading on ORM best practices, feel free to check out Hibernate Best Practices.
Setting Up Hibernate Logging
Hibernate can be configured to use various logging frameworks like Log4j, SLF4J, and JUL (Java Util Logging). In this tutorial, we'll focus on SLF4J, which is popular for its versatility and ease of integration.
Step 1: Include Dependencies
If you're using Maven, the first step is to include the necessary dependencies in your pom.xml
file. Here's an example:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.30.Final</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
The above dependencies include Hibernate, SLF4J, and Log4j. Always ensure you specify the latest versions compatible with your project.
Step 2: Create Log4j Configuration
In your classpath, create a log4j.properties
file for configuring Log4j. A basic configuration file might look like this:
log4j.rootLogger=DEBUG, console
log4j.appender.console=org.apache.log4j.ConsoleAppender
log4j.appender.console.layout=org.apache.log4j.PatternLayout
log4j.appender.console.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n
# Hibernate specific logging
log4j.logger.org.hibernate=DEBUG
log4j.logger.org.hibernate.SQL=DEBUG
log4j.logger.org.hibernate.type=TRACE
Explanation of Configuration
- log4j.rootLogger: Sets the logging level and appender.
- console: This appender outputs logs to the console.
- ConversionPattern: Defines the format in which logs are displayed.
- Hibernate Log Levels: Adjust logging for Hibernate itself—SQL statements and parameter bindings for detailed insights.
Common Pitfalls and How to Fix Them
Pitfall 1: No Output from Hibernate Logging
Symptom: You do not see any logs even though you expect them.
Fix: Ensure that your logging configuration file is in the right place (src/main/resources
for Maven). Also, confirm the logging level set is sufficient to display the logs you need.
log4j.rootLogger=DEBUG, console
Pitfall 2: Excessive Logging
Symptom: The logs contain too much output, making it difficult to trace specific issues.
Fix: Adjust the logging levels. If you're primarily interested in SQL logs, you can reduce the verbosity of other logs:
log4j.logger.org.hibernate=ERROR
log4j.logger.org.hibernate.SQL=DEBUG
Here, setting Hibernate's logging level to ERROR reduces clutter while still providing useful SQL information.
Pitfall 3: Missing SQL Parameters
Symptom: The SQL statements logged do not show bound parameters.
Fix: Use TRACE level logging for Hibernate types to log parameters.
log4j.logger.org.hibernate.type=TRACE
This gives you detailed insight into the parameters being passed, making troubleshooting easier.
Pitfall 4: Performance Issues Due to Logging
Symptom: The application runs slower than expected.
Fix: Excessive logging can bottleneck performance. Check your logging configuration to avoid logging unnecessary details during production.
For production environments, refine your logging like this:
log4j.logger.org.hibernate.SQL=ERROR
This way, you get less noise in the logs while avoiding performance issues.
Pitfall 5: Unclear Log Messages
Symptom: Log messages aren't informative enough.
Fix: Customize log messages in your application where applicable. Use SLF4J’s logging capabilities efficiently:
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void saveData(Data data) {
logger.info("Saving data: {}", data);
session.save(data);
logger.info("Data saved successfully");
}
This log clearly indicates what happens in the application, providing context that can help during debugging.
A Final Look
Logging is an essential part of mastering Hibernate. Understanding common pitfalls and how to address them ensures you're well-equipped to monitor your application effectively. By integrating proper logging configuration and keeping an eye on performance, you can make the most of Hibernate while maintaining healthy application behavior.
For more detailed Hibernate documentation, visit Hibernate Documentation.
Feel free to comment below if you have any additional questions or insights about Hibernate logging! Happy coding!