Eliminating Common Pitfalls in Version Control Systems
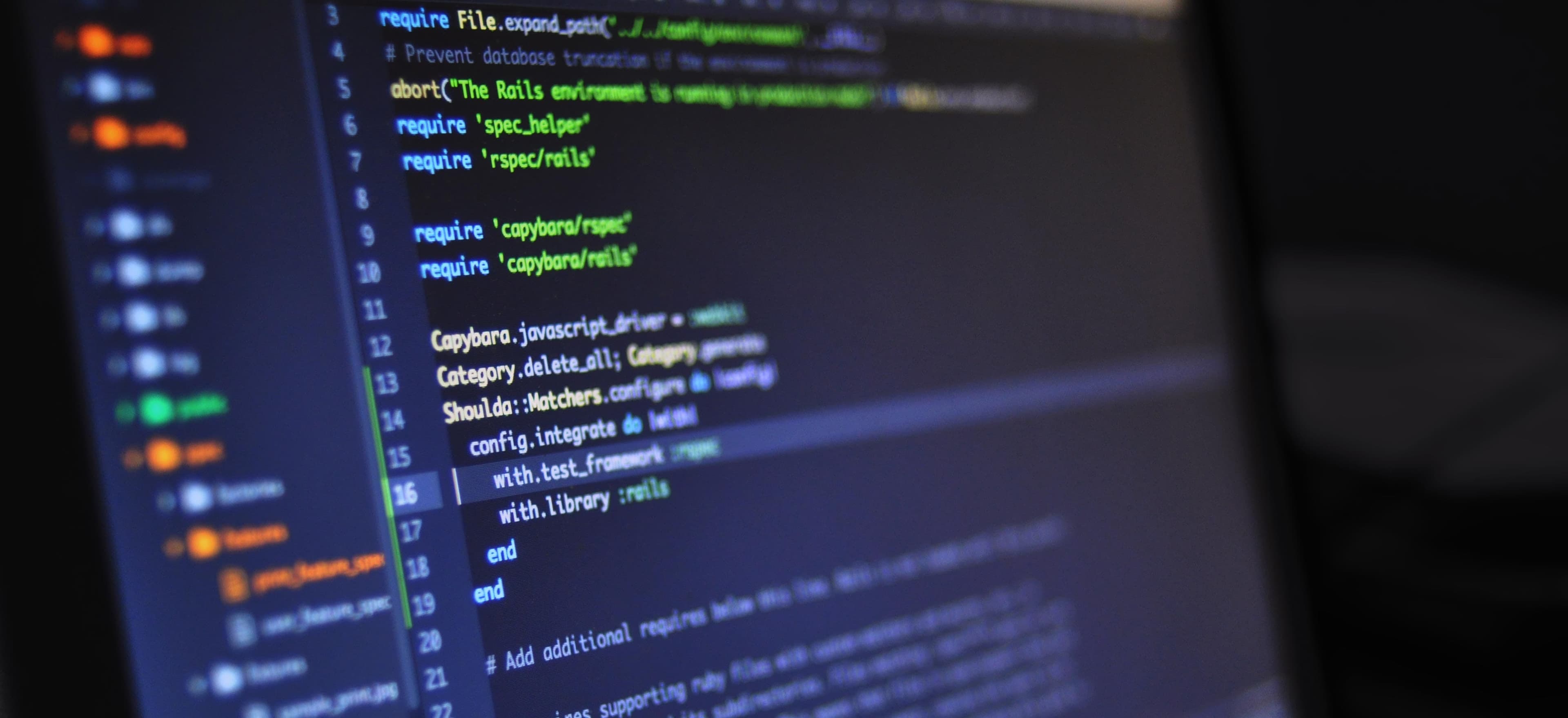
- Published on
Eliminating Common Pitfalls in Version Control Systems
Version control systems (VCS) are crucial tools in modern software development, providing a framework for managing changes over time. They help teams collaborate, maintain code history, and ensure code quality. However, developers often stumble over common pitfalls that can derail their efforts. This article explores these pitfalls and offers solutions to eliminate them, ensuring a smooth version control experience.
Understanding Version Control Systems
Before diving into the pitfalls, let’s briefly discuss what a version control system is. A VCS records changes to files or sets of files over time. This allows developers to revert to earlier versions, track modifications, and collaborate without overwriting each other's work.
Popular VCS tools include:
- Git
- Subversion (SVN)
- Mercurial
Among these, Git is widely adopted due to its robustness, flexibility, and support for branching and merging.
Common Pitfalls in Version Control Systems
1. Lack of Clear Branching Strategies
Problem: Many teams start using a VCS without a clear branching strategy. This can lead to confusion and merge conflicts.
Solution: Adopt a branching model like Git Flow or GitHub Flow. These models provide a standardized way to manage features, fixes, and releases.
Git Flow Example
Using Git Flow, developers create dedicated branches for features, hotfixes, and releases. Here's a simplified version of the process:
# Start a new feature
git checkout -b feature/new-feature
# After completing the feature
git add .
git commit -m "Add new feature"
# Merge back to develop
git checkout develop
git merge feature/new-feature
# Delete the feature branch
git branch -d feature/new-feature
Why use Git Flow? It structures the workflow, reduces merge conflicts, and makes it easy to track changes.
2. Committing Large Changes
Problem: Developers sometimes commit too many changes at once, making it hard to identify which changes caused an issue.
Solution: Commit smaller, more focused changes with meaningful commit messages.
Example of Effective Commits
git commit -m "Fix issue with user login functionality"
git commit -m "Refactor database connection handling"
Why commit small changes? It simplifies debugging and improves collaboration since team members can easily see what was modified.
3. Ignoring .gitignore Files
Problem: Developers often forget to set up a .gitignore
file, leading to unnecessary files being tracked.
Solution: Use a .gitignore
file to specify files and directories that should not be tracked.
Example of a .gitignore File
# Ignore node modules
node_modules/
# Ignore log files
*.log
# Ignore environment configuration files
.env
Why use .gitignore
? It keeps your repository clean and ensures sensitive information is not shared inadvertently.
4. Not Using Pull Requests
Problem: Some developers bypass pull requests (PRs), merging directly into main branches.
Solution: Always use PRs for code review and discussion. This practice encourages collaboration and knowledge sharing, improves code quality, and reduces bugs.
Creating a Pull Request
Typically, after pushing a branch to remote, you can create a pull request on platforms like GitHub or GitLab. Here’s how it usually goes down:
-
Push your feature branch:
git push origin feature/new-feature
-
Head to your repository's page and open a new PR.
Why use pull requests? They facilitate discussion and code review, ensuring that multiple eyes keep watch over code changes before they are merged.
5. Forgetting to Pull the Latest Changes
Problem: Developers often forget to pull the latest changes from shared branches, resulting in conflicts when merging.
Solution: Regularly pull from the master branch to stay updated.
Example Command
# Pull changes from the remote master branch
git pull origin master
Why frequently pull changes? It reduces the likelihood of merge conflicts and keeps developers in sync with the latest code.
6. Abusing Force Push
Problem: Some developers use force push (git push --force
) to overwrite changes in shared branches, leading to confusion and lost work.
Solution: Avoid force pushing unless absolutely necessary. Instead, use git push --force-with-lease
to ensure you are not overwriting others' work.
Example of Safe Force Push
# Safe force push
git push --force-with-lease
Why avoid reckless force pushing? It maintains the integrity of the shared branch and prevents losing valuable work from teammates.
7. Neglecting Documentation of Changes
Problem: Failing to document changes can leave team members (and future you) confused about why certain changes were made.
Solution: Maintain a CHANGELOG or a README update in conjunction with commits.
Why document changes? It facilitates knowledge sharing and context for future code contributions.
Best Practices for Version Control Systems
Now that we've explored the common pitfalls, let's discuss some best practices that help eliminate them.
Regularly Review Code
Consistent code reviews can catch issues early and improve code quality. It’s a team effort.
Utilize Tags for Releases
Use tags to mark release points in your repository. This provides a snapshot of your code at important milestones.
# Tagging a release
git tag -a v1.0 -m "Version 1.0 release"
git push origin v1.0
Why tag releases? It simplifies tracking and deploying specific versions of your software.
Leverage Hooks
Git hooks can automate checks and processes in your workflow. For example, you can set a pre-commit hook to enforce formatting rules.
Automate CI/CD
Integrating Continuous Integration/Continuous Deployment (CI/CD) helps automate testing and deployment, ensuring a more reliable code base.
Tools you can use include:
- Jenkins
- Travis CI
- GitHub Actions
Conduct Training Sessions
Regular training on VCS usage can help onboard new developers and reinforce best practices across the team.
The Bottom Line
Version control systems are essential for successful software development, but avoiding common pitfalls can make a significant difference in your workflow. By implementing clear branching strategies, committing smaller changes, utilizing pull requests, and enforcing best practices, teams can streamline their processes and reduce the risk of errors.
For further reading on version control and best practices, consider checking out these resources:
With effective strategies in place, you'll be well on your way to mastering version control systems and enhancing your development workflow. Happy coding!
Checkout our other articles