Common Pitfalls to Avoid with Abstract Factory Pattern
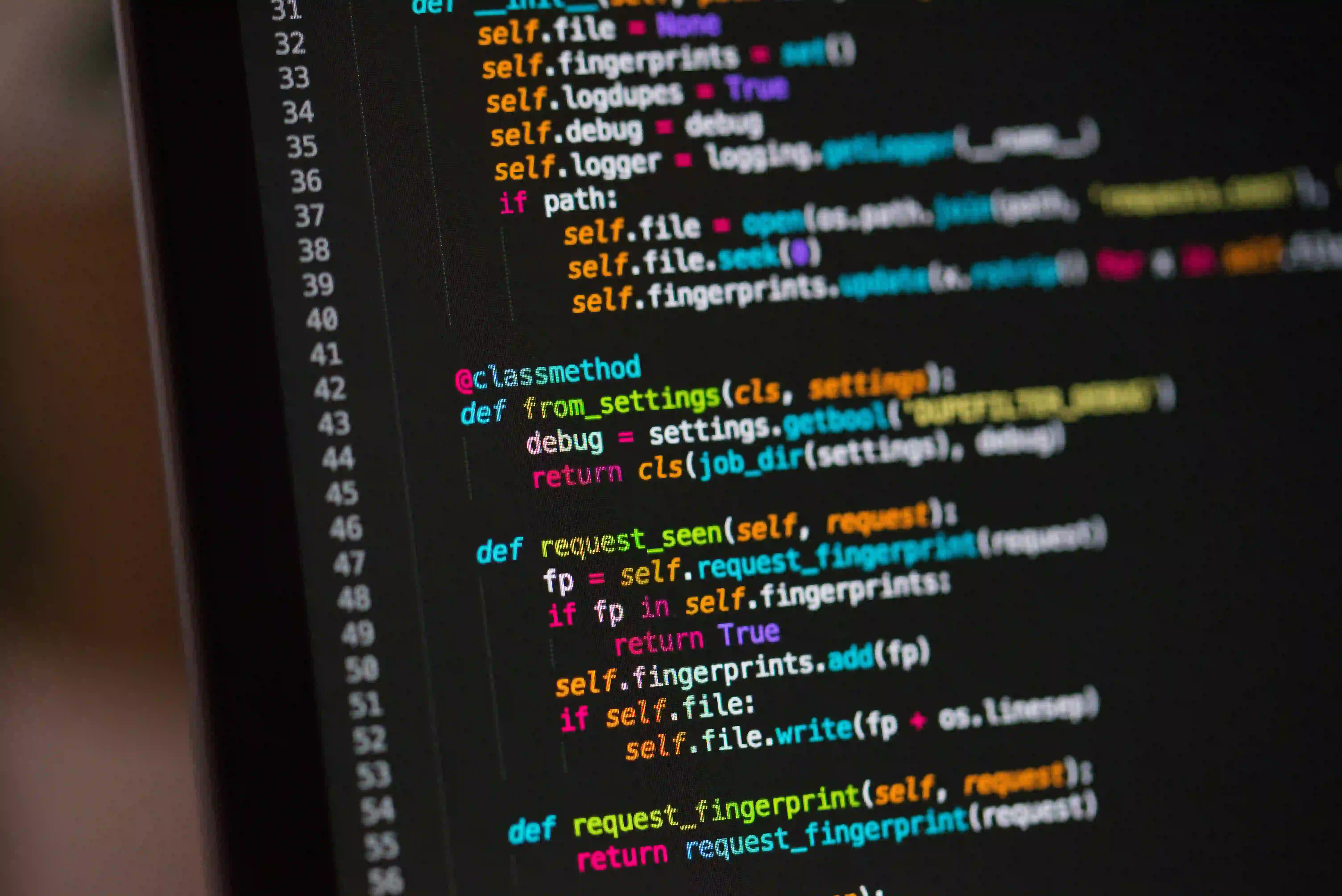
Common Pitfalls to Avoid with the Abstract Factory Pattern in Java
The Abstract Factory Pattern is a crucial design pattern in software development, especially within the realms of object-oriented design. It provides a way to create families of related or dependent objects without specifying their concrete classes. However, despite its advantages, developers often encounter pitfalls when implementing this pattern. This blog post aims to explore common mistakes and best practices associated with the Abstract Factory Pattern in Java.
What is the Abstract Factory Pattern?
Before delving into pitfalls, it's essential to understand what the Abstract Factory Pattern entails.
At its core, this pattern allows you to create an interface for creating families of related or dependent objects. It abstracts the instantiation process, thereby enhancing code modularity and reducing dependencies between classes.
For instance, suppose you are building a UI toolkit. Instead of having direct instantiation of button and window classes, you could create abstract factories for different styles of UI components (e.g., Windows style, Mac style) that provide the specific implementation of buttons and windows.
Basic Implementation
Here’s a simple example to illustrate the Abstract Factory Pattern in Java.
// Abstract products
interface Button {
void render();
}
interface Window {
void paint();
}
// Concrete products for Windows
class WindowsButton implements Button {
@Override
public void render() {
System.out.println("Rendering a Windows Button");
}
}
class WindowsWindow implements Window {
@Override
public void paint() {
System.out.println("Painting a Windows Window");
}
}
// Concrete products for Mac
class MacButton implements Button {
@Override
public void render() {
System.out.println("Rendering a Mac Button");
}
}
class MacWindow implements Window {
@Override
public void paint() {
System.out.println("Painting a Mac Window");
}
}
// The Abstract Factory
interface GUIFactory {
Button createButton();
Window createWindow();
}
// Concrete Factory for Windows
class WindowsFactory implements GUIFactory {
@Override
public Button createButton() {
return new WindowsButton();
}
@Override
public Window createWindow() {
return new WindowsWindow();
}
}
// Concrete Factory for Mac
class MacFactory implements GUIFactory {
@Override
public Button createButton() {
return new MacButton();
}
@Override
public Window createWindow() {
return new MacWindow();
}
}
// Client code
public class Client {
private Button button;
private Window window;
public Client(GUIFactory factory) {
button = factory.createButton();
window = factory.createWindow();
}
public void renderUI() {
button.render();
window.paint();
}
public static void main(String[] args) {
GUIFactory factory = getGUIFactory();
Client client = new Client(factory);
client.renderUI();
}
private static GUIFactory getGUIFactory() {
// Customize this method to return the appropriate factory based on requirements.
return new WindowsFactory(); // or new MacFactory();
}
}
In this example, the Client
class uses GUI factories to create respective UI components. This structure enhances maintainability and scalability.
Common Pitfalls to Avoid
While the Abstract Factory Pattern can greatly simplify your code, certain pitfalls can undermine its benefits. Here, we highlight several common mistakes and how to navigate them.
1. Overcomplicating the Design
Issue
One of the most common pitfalls is overengineering the implementation. Developers may create unnecessarily complex abstractions and interfaces.
Solution
Keep it simple. Only use the Abstract Factory Pattern when you need to create families of objects that share a common theme and when the process of instantiation is likely to vary.
2. Ignoring the Liskov Substitution Principle (LSP)
Issue
According to the LSP, objects should be replaceable with instances of their subtypes without altering the correctness of the program. A violation occurs if the created concrete classes do not adhere closely to the expected behavior.
Solution
Ensure that subclasses correctly implement the behavior expected from their superclass. In our button example, both WindowsButton
and MacButton
should offer similar functionality, even if their implementations differ.
3. Not Following the Dependency Inversion Principle (DIP)
Issue
While the Abstract Factory Pattern abstracts the object creation process, it can lead to tight coupling if not implemented correctly. The client should ideally depend on abstractions, not on concrete implementations.
Solution
Utilize interfaces to achieve higher flexibility and loose coupling. The client should focus on using the abstract factories rather than concrete factory classes. By doing so, you promote more adaptable and testable code.
4. Missing Usability in Factory Creation
Issue
Sometimes developers may overlook how to instantiate the appropriate factory dynamically, causing the code to be less extensible or requiring changes in multiple places when new products are added.
Solution
Implement a factory producer method that decides on the factory instance at runtime based on some conditions. This allows you to swap implementations without altering the client code.
public class GUIFactoryProvider {
public static GUIFactory getFactory(String factoryType) {
if (factoryType.equalsIgnoreCase("WINDOWS")) {
return new WindowsFactory();
} else if (factoryType.equalsIgnoreCase("MAC")) {
return new MacFactory();
}
throw new IllegalArgumentException("Unknown factory type");
}
}
5. Inconsistent Object Creation
Issue
If your various factories create objects that differ in some fundamental way, it can lead to inconsistent application behavior.
Solution
Make sure that all factories producing the same family of products adhere to a similar design pattern. It is vital for maintaining a consistent API and overall system behavior.
6. Neglecting Testing
Issue
Due to its abstract nature, testing the configurations and variations of an Abstract Factory Pattern can be tricky. Developers may ignore unit testing these factories, leading to a fragility in the code.
Solution
Implement test cases for each of the created products and the factories themselves. Develop a strategy for unit testing by mocking dependencies or using test doubles to ensure each piece can be tested independently.
import static org.mockito.Mockito.*;
public class ClientTest {
@Test
public void testButtonRender() {
GUIFactory mockFactory = mock(GUIFactory.class);
Button mockButton = mock(Button.class);
when(mockFactory.createButton()).thenReturn(mockButton);
Client client = new Client(mockFactory);
client.renderUI();
verify(mockButton).render();
}
}
Bringing It All Together
The Abstract Factory Pattern offers a robust solution for encapsulating object creation, enabling cleaner code and better maintainability. However, it's crucial to avoid the common pitfalls we've discussed. By following best practices, understanding the underlying principles such as Liskov Substitution and Dependency Inversion, and ensuring retail usability and testing, you can leverage the Abstract Factory Pattern to its full potential.
For more information on design patterns, consider checking out the Gang of Four Design Patterns or delve into specific design principles on Martin Fowler's website.
Integrating these principles can elevate your professional coding skills and contribute to developing high-quality, maintainable software solutions. Happy coding!