Common JavaFX Programming Pitfalls and How to Avoid Them
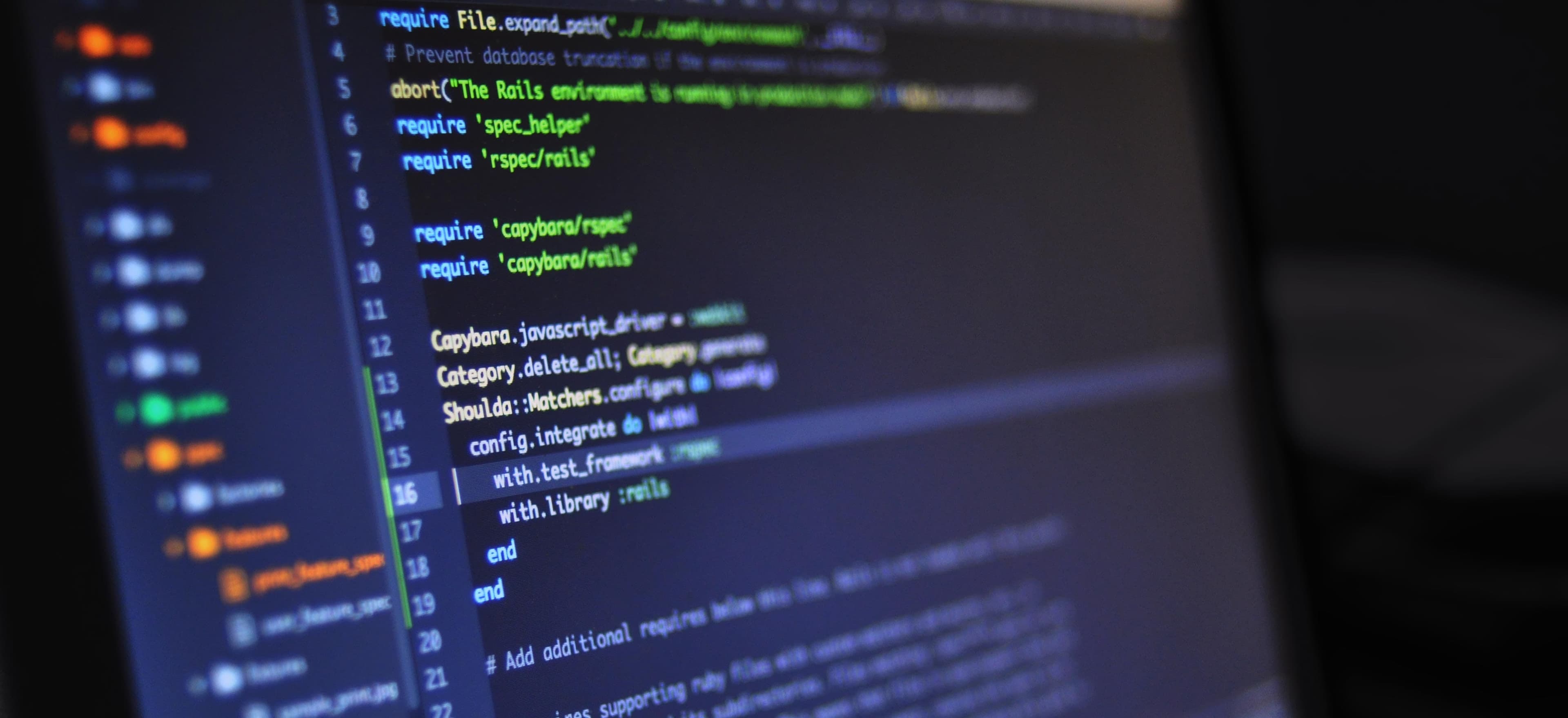
- Published on
Common JavaFX Programming Pitfalls and How to Avoid Them
JavaFX, a powerful framework for building rich desktop applications in Java, provides an array of features that simplify the development of dynamic User Interfaces (UIs). However, like any technology, it has its quirks and pitfalls that can trip up even seasoned developers. This post will explore common pitfalls in JavaFX programming and offer tips on how to avoid them effectively.
1. Blocking the JavaFX Application Thread
One of the cardinal rules of JavaFX is that the UI components must be manipulated on the JavaFX Application Thread. If you perform long-running tasks on this thread, it can cause the UI to freeze, leading to a poor user experience.
The Pitfall
Here’s an example of code that blocks the JavaFX Application Thread:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class BlockingExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Long Task");
button.setOnAction(event -> {
// Simulating a long-running task
for(int i = 0; i < 100000; i++) {
System.out.println(i);
}
});
StackPane root = new StackPane();
root.getChildren().add(button);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
How to Avoid It
To avoid blocking the JavaFX Application Thread, use a separate thread for long-running tasks. You can achieve this with a Task
or an ExecutorService
.
Here's how you can do this using a Task
:
import javafx.application.Application;
import javafx.concurrent.Task;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class NonBlockingExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Long Task");
button.setOnAction(event -> {
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
for (int i = 0; i < 100000; i++) {
System.out.println(i);
}
return null;
}
};
new Thread(task).start();
});
StackPane root = new StackPane();
root.getChildren().add(button);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the corrected code, the long-running task is executed in a separate thread. This ensures that the UI remains responsive while the background task executes.
2. Misunderstanding Event Handling
JavaFX works heavily with events, such as user actions (mouse clicks, key presses, etc.). A frequent mistake is not understanding how events bubble and delegate.
The Pitfall
Consider the following misimplementation of an event handler:
button.setOnAction(event -> {
// Incorrect Usage
someStage.show();
System.out.println(event.getSource()); // Prints source of event
});
How to Avoid It
Ensure that your event handling logic is robust by properly managing the source of the event:
- Use
event.consume()
if you want to prevent further handling of the event. - Be mindful of event bubbling and ensure that the correct stage is being used.
Here's how you can improve it:
button.setOnAction(event -> {
event.consume(); // Prevent further propagation
Stage newStage = new Stage();
newStage.setTitle("New Window");
newStage.show();
System.out.println("New window opened from: " + event.getSource());
});
By consuming the event, you avoid unintentional propagation that may lead to unexpected behavior.
3. Improper Layout Management
One of the beauties of JavaFX lies in its versatile layout managers. However, improper usage can make layouts look cluttered or unresponsive.
The Pitfall
Developers often nest layout panes too deeply, thereby introducing unnecessary complexity and performance issues:
VBox vboxOuter = new VBox();
VBox vboxInner = new VBox();
// Many nested components
vboxOuter.getChildren().add(vboxInner);
How to Avoid It
Keep your layout hierarchy simple. Use suitable layout managers based on your use case. Evaluate alternate layouts like GridPane
for more control over positioning.
Here's a more streamlined example:
GridPane grid = new GridPane();
grid.setHgap(10);
grid.setVgap(10);
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
grid.add(button1, 0, 0);
grid.add(button2, 1, 0);
// Set grid to your scene or stage
In this way, layouts are easier to manage and look cleaner in the final application.
4. Failing to Use CSS for Styling
JavaFX supports CSS for styling, but many developers neglect this feature, opting instead for programmatic styling.
The Pitfall
Overusing Java code for styling can lead to hard-to-maintain code in the long run:
button.setStyle("-fx-background-color: red; -fx-text-fill: white;");
How to Avoid It
Leverage CSS to separate concerns effectively. Define styles in a .css
file:
.button {
-fx-background-color: red;
-fx-text-fill: white;
}
And then apply this stylesheet to your application:
scene.getStylesheets().add("path/to/stylesheet.css");
This allows you to maintain a clear separation between logic and presentation, improving code readability and maintainability.
5. Ignoring Binding Features
JavaFX provides powerful binding features that can help manage dependencies between properties, but developers sometimes underutilize them.
The Pitfall
Directly managing property values can lead to bugs and complicated code:
TextField textField = new TextField();
Label label = new Label();
textField.setOnKeyReleased(event -> {
label.setText(textField.getText());
});
How to Avoid It
Use property bindings to make your code cleaner and less error-prone:
label.textProperty().bind(textField.textProperty());
In this manner, changes in the TextField
are automatically reflected in the Label
, and you eliminate unnecessary event handling code.
In Conclusion, Here is What Matters
JavaFX is an impressive framework that gives developers the tools to create elegant desktop applications. By steering clear of these common pitfalls—such as blocking the Application Thread, mismanaging event handling, and ignoring CSS and binding—developers can build more responsive, maintainable applications.
Want to dive deeper into JavaFX? Explore the JavaFX documentation here or check out various JavaFX tutorials for a structured learning path.
Happy coding!
Checkout our other articles