Accessing Private Fields in Unit Tests: The Hidden Dilemma
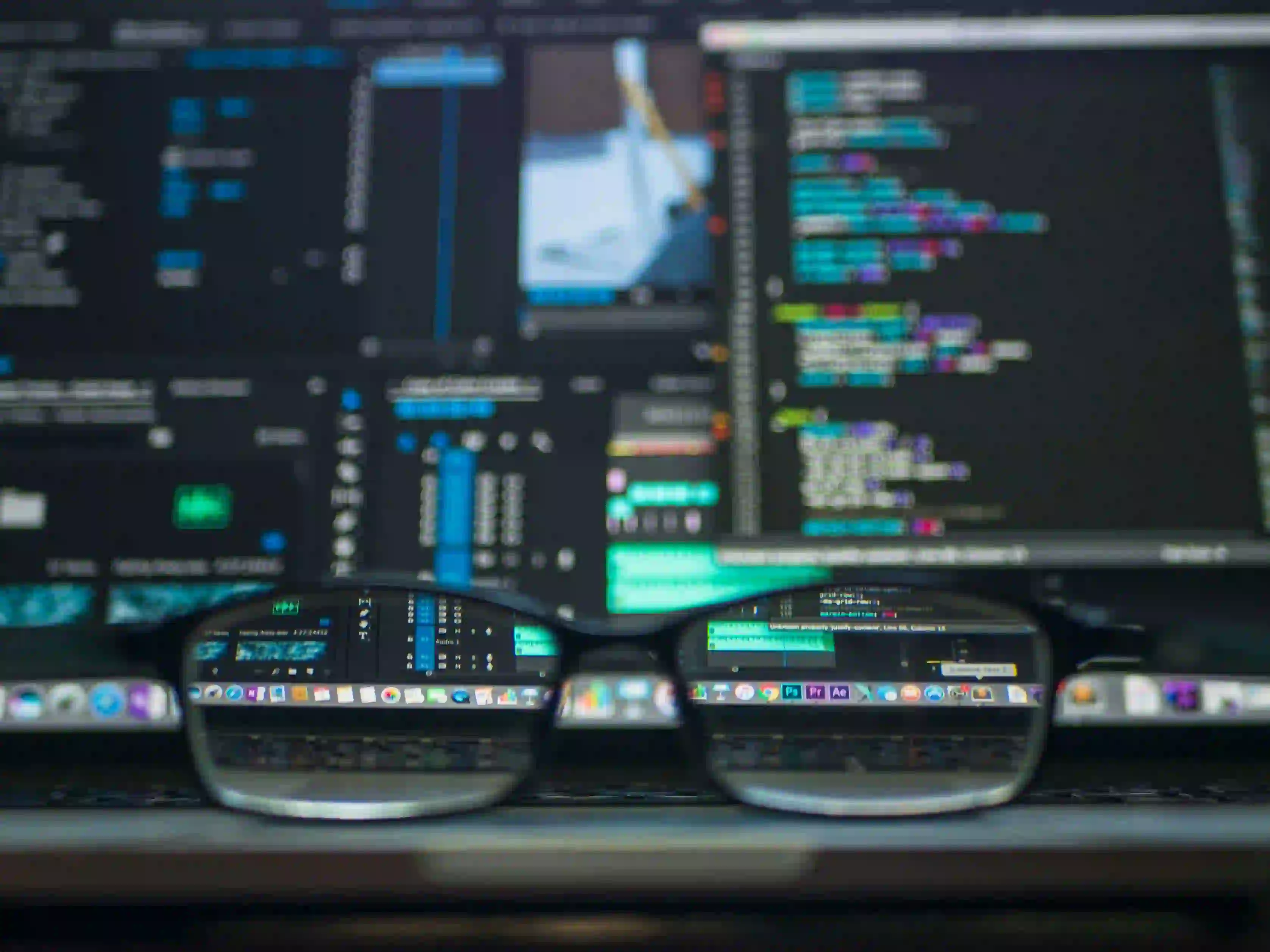
Accessing Private Fields in Unit Tests: The Hidden Dilemma
When it comes to unit testing in Java, developers often find themselves standing at a crossroads. On one side is the pursuit of a clean and effective testing strategy, while on the other is the challenge of accessing private fields from the classes they are testing. This dilemma raises questions about the principles of encapsulation and how they affect the testability of code. In this blog post, we'll explore the implications of accessing private fields in unit tests, practical solutions, and best practices to maintain clean, testable code.
Understanding Encapsulation in Java
Encapsulation is one of the four fundamental Object-Oriented Programming (OOP) principles. It promotes keeping the internal state of an object hidden from the outside world and only allows controlled access through public methods. This principle is a cornerstone of robust design but can become problematic when it comes to unit testing.
Why Private Fields Matter
Private fields help ensure that an object's state cannot be altered in unintended ways. They define a contract where the class is responsible for managing its own state, which enhances maintainability and reduces bugs.
However, in unit testing, we often need to validate the internal state of the class after interacting with its public methods. Directly accessing private fields can lead to several issues:
- Tight Coupling: Tests that depend on private fields can become tightly coupled to the implementation details, making them fragile and prone to breaking when refactoring.
- Reduced Flexibility: You may find yourself unable to effectively test changes in the logic without exposing private fields.
- Violation of Principles: Direct access to private state undermines the encapsulation principles meant to protect an object's state.
Strategies for Accessing Private Fields
Despite the risks associated with accessing private fields, there are scenarios where it may be necessary. Here are several strategies with examples.
1. Reflection
Java's Reflection API allows us to inspect classes and manipulate their fields and methods dynamically. Here’s an example of using reflection to access a private field in a unit test:
import org.junit.jupiter.api.Test;
import java.lang.reflect.Field;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyClassTest {
@Test
public void testPrivateFieldAccess() throws Exception {
MyClass myClassInstance = new MyClass();
Field privateField = MyClass.class.getDeclaredField("myPrivateField");
privateField.setAccessible(true);
// Set the private field value
privateField.set(myClassInstance, "test value");
// Access the private field value
String value = (String) privateField.get(myClassInstance);
assertEquals("test value", value);
}
}
Commentary on Reflection
While powerful, using reflection is not without its drawbacks. It can obscure the intent of the code, making it harder to read and understand. Additionally, since reflection bypasses normal access control, it can lead to security vulnerabilities.
2. Package-Private Access
Another approach is to use package-private access for your fields and methods. This allows unit tests in the same package to access private members without exposing them to the world.
// In MyClass.java
class MyClass {
int myPackagePrivateField;
// Some methods that manipulate the field...
}
// In MyClassTest.java (in the same package)
public class MyClassTest {
@Test
public void testPackagePrivateField() {
MyClass myClass = new MyClass();
myClass.myPackagePrivateField = 10;
assertEquals(10, myClass.myPackagePrivateField);
}
}
Commentary on Package-Private Access
This strategy maintains the principle of encapsulation within the package while still providing access to unit tests. However, it may reduce the encapsulation benefits if your classes are used in a wider scope.
3. Extra Test Methods
Some developers opt to include additional testing methods to expose internal state for testing purposes. This involves adding public methods specifically for testing. These can be annotated with @VisibleForTesting
, which can serve as documentation.
// In MyClass.java
public class MyClass {
private int myPrivateField;
@VisibleForTesting
public int getMyPrivateField() {
return myPrivateField;
}
}
// In MyClassTest.java
public class MyClassTest {
@Test
public void testUsingVisibilityForTesting() {
MyClass myClass = new MyClass();
// Interact with public methods...
int privateFieldValue = myClass.getMyPrivateField();
assertEquals(expectedValue, privateFieldValue);
}
}
Commentary on Extra Test Methods
While this approach is straightforward and maintains encapsulation, it can clutter the class with methods solely for test validation, which is only useful during testing.
4. Mocking Frameworks
Mocking frameworks, like Mockito or PowerMock, can help simulate interactions and state checks without directly accessing private fields. With these frameworks, you can isolate the class being tested without the need to resort to reflection or exposing internal state.
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
public class MyClassTest {
@Test
public void testUsingMock() {
MyClass myClass = mock(MyClass.class);
when(myClass.getMyPrivateField()).thenReturn(42);
assertEquals(42, myClass.getMyPrivateField());
}
}
Commentary on Mocking Frameworks
Using mocks allows for thorough testing while keeping encapsulation intact. This method is often the preferred choice in unit tests, as it centers around interaction with the public API.
Best Practices for Unit Testing Private Fields
Here are some best practices to embrace when dealing with private fields in unit tests:
-
Test the Public API: Always strive to test the public interface of your classes. If the public methods work as intended, the private fields should naturally follow.
-
Use Reflection Sparingly: While reflection can solve immediate issues, consider its implications on maintainability and readability. Use it only when absolutely necessary.
-
Adhere to Single Responsibility Principle: Classes should be designed to focus on a single responsibility. This makes unit tests simpler and limits the need to access private fields.
-
Refactor for Testability: If a class is hard to test, it's often a sign that it needs refactoring. Break down large classes into smaller, more manageable ones. Enhance cohesion and minimize coupling.
-
Favor Composition Over Inheritance: Favoring composition can reduce dependencies, making unit testing simpler and more comprehensible.
-
Documentation: Use annotations such as
@VisibleForTesting
to indicate the purpose of public methods that are meant primarily for testing. This enhances maintainability.
My Closing Thoughts on the Matter
Accessing private fields during unit testing in Java is often a contentious topic. While encapsulation is crucial for maintainable and robust code, there are situations where testing private fields becomes necessary. By employing strategies like reflection, package-private access, and mocking frameworks judiciously, developers can navigate this hidden dilemma effectively.
Ultimately, embracing best practices and focusing on testing the public API of your classes will not only ensure your code remains clean and maintainable, but it will also enhance the reliability of your tests. It's a balancing act, but with due diligence and creativity, you can create a solid foundation for testing your Java applications.
For further reading on unit testing principles in Java, consider checking these resources:
With a scientific approach and a thoughtful design, you can master the art of unit testing while respecting Java's encapsulation principles. Happy testing!