Common Pitfalls When Integrating Spring and JSF
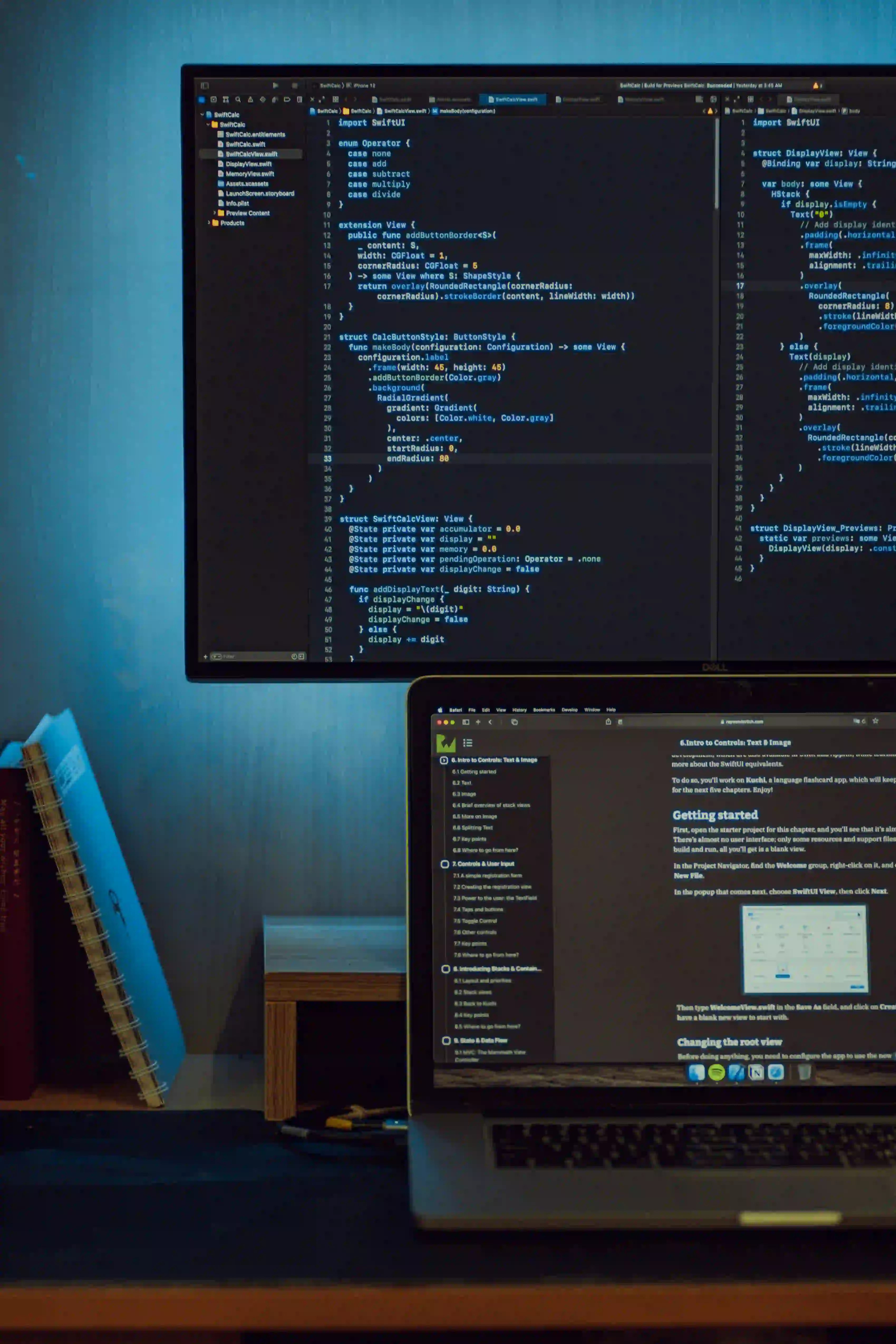
Common Pitfalls When Integrating Spring and JSF
Integrating Spring Framework with JavaServer Faces (JSF) can significantly enhance your application by combining the powerful backend capabilities of Spring with the robust frontend features of JSF. However, this integration is not without its hurdles. In this blog post, we will explore some common pitfalls when integrating Spring and JSF, providing you with practical insights and solutions to avoid or resolve them.
Understanding Spring and JSF Integration
Spring is a comprehensive framework that provides various capabilities, including dependency injection, transaction management, and more. JSF, on the other hand, is a Java specification for building component-based user interfaces for web applications. The combination of these two frameworks can lead to a well-structured MVC architecture— if done correctly.
Key Considerations
-
Context Configuration: One of the most common pitfalls is improper context configuration. Make sure that the Spring context and JSF context are correctly set up to work together.
-
Lifecycle Management: Understanding the life cycle of both frameworks is critical. JSF has its own component lifecycle, which can run into trouble when Spring beans are not managed appropriately.
-
Managed Beans vs Spring Beans: Confusion often arises between JSF managed beans and Spring beans. While both serve similar purposes, they differ in how they are managed and utilized.
Pitfall 1: Inconsistent Scopes of Beans
One of the frequent issues developers encounter is mismatched scopes between Spring beans and JSF managed beans.
Solution
Always align the scopes of your beans. JSF supports various scopes: @RequestScoped
, @ViewScoped
, @SessionScoped
, and @ApplicationScoped
. Spring also offers similar scopes, but it's crucial to understand how these scopes align with each other during the lifecycle of your application.
Example:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
@ManagedBean(name = "myBean")
@RequestScoped
@Component
@Scope("request")
public class MyBean {
// Your code logic here
}
Here, we utilize both @ManagedBean
for JSF and @Component
with @Scope
for Spring with the same RequestScoped
, maintaining consistency in bean scopes.
Pitfall 2: Improper Injection of JSF Components
Another common mistake developers make is trying to inject JSF components directly into Spring beans. This results in runtime exceptions as JSF components are not managed by Spring.
Solution
To resolve this issue, use @Context
from JNDI to inject JSF managed beans into Spring.
Example:
import javax.faces.context.FacesContext;
import javax.inject.Inject;
public class SomeService {
@Inject
private SomeManagedBean managedBean;
public void someMethod() {
// Use the managed bean safely here
}
}
Alternatively, if your JSF component is being used in a Spring-managed bean, it's good practice to use FacesContext
to obtain the bean dynamically.
Pitfall 3: Mixing Navigation Handlers
In JSF, navigation is typically handled using the Faces navigation rules or navigation handlers. Mixing these with Spring's MVC handlers can lead to unpredictable behavior.
Solution
It's best to stick with one navigation strategy. If you are primarily using JSF, define the navigation rules inside your faces-config.xml
instead of mixing with Spring MVC’s view resolvers.
Pitfall 4: Inadequate Error Handling
Error handling in a combined Spring and JSF application can be quite challenging. Advanced error handling mechanisms in Spring can be undermined by JSF's component-based approach to error rendering.
Solution
Leverage the exception handling capabilities of JSF to ensure that your users are presented with meaningful messages. Utilize ExceptionHandler
for this purpose.
Example:
import javax.faces.context.ExceptionHandler;
import javax.faces.context.ExceptionHandlerFactory;
import javax.faces.event.ExceptionHandlerEvent;
public class CustomExceptionHandler extends ExceptionHandlerWrapper {
@Override
public void handle() throws FacesException {
for (Throwable t : getUnhandledExceptionQueuedEvents()) {
// Handle exception
}
}
}
In this custom handler, you can dictate how exceptions are treated and displayed, maintaining a user-friendly experience.
Pitfall 5: Inconsistent Configuration Files
Having multiple configuration files can often lead to discrepancies, resulting in a failure to wire the beans correctly.
Solution
Keep your configuration files organized and ensure they are loaded in proper sequence. In a Spring context XML configuration, ensure that you explicitly declare JSF support where needed.
Final Considerations
Integrating Spring and JSF can unleash powerful features in your applications. However, by being aware of common pitfalls, you can create more robust, maintainable applications. Ensuring that your bean scopes are aligned, properly injecting components, managing navigation effectively, handling errors consistently, and maintaining clear configuration are all crucial to a successful integration.
Additional Resources
- Spring Framework Documentation
- JSF Documentation
- Java EE Patterns - Best Practices in JSF Development
By adhering to the strategies and solutions mentioned above, you can save yourself and your team time and headache in the integration process. Happy coding!