Troubleshooting Common JGit Access Issues
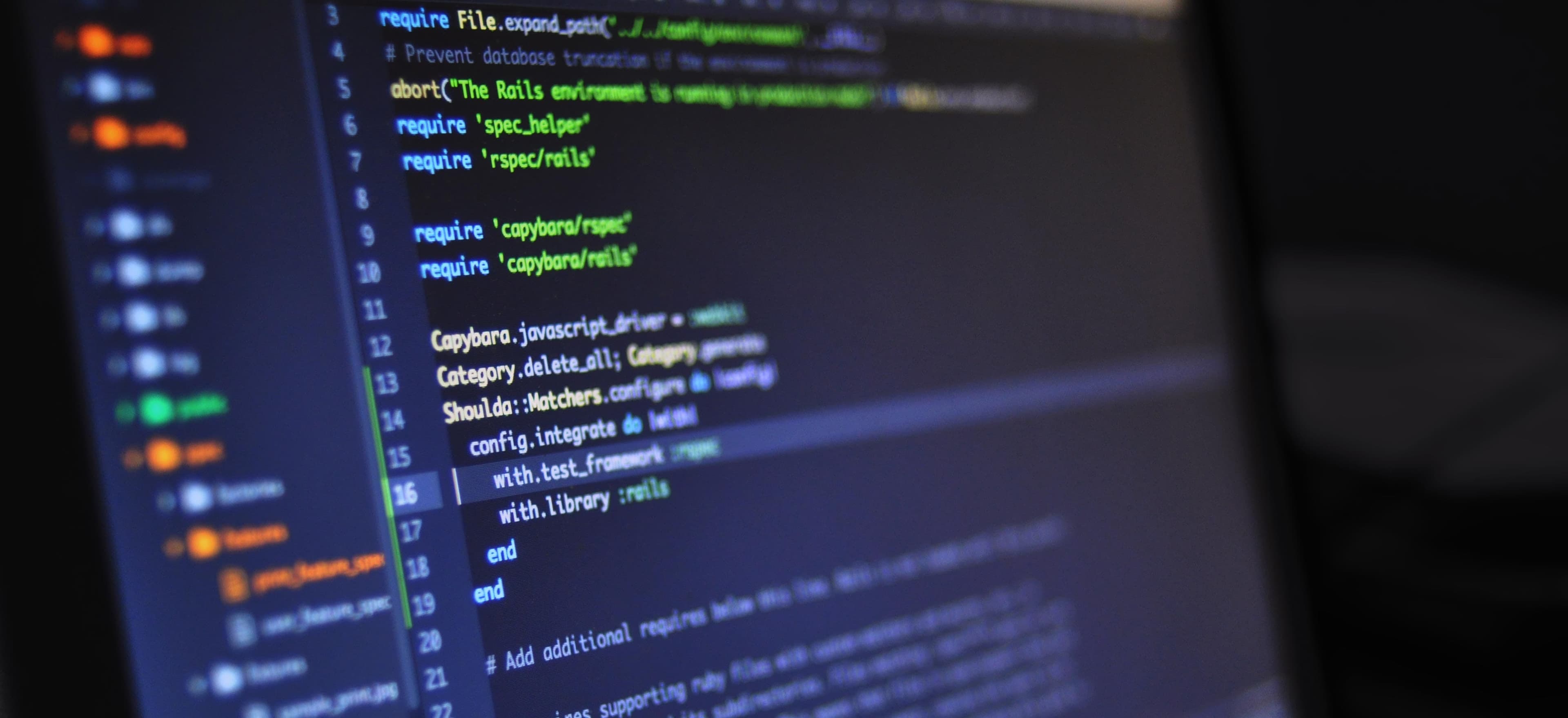
- Published on
Troubleshooting Common JGit Access Issues
JGit is a pure Java implementation of the Git version control system. Whether you are developing Java applications or integrating Git functionalities into your programs, JGit provides a powerful way to interact with repositories. However, encountering access issues can be a common hurdle among developers. Whether it's authentication failures, incorrect path configurations, or regular repository accessibility, learning how to troubleshoot these problems is crucial for a smooth development experience.
In this blog post, we will explore some common JGit access issues, troubleshoot them with effective solutions, and present insightful code snippets for better understanding. We will also look at how authentication works and the best practices involved in using JGit effectively.
Understanding JGit and Access Issues
Before diving into troubleshooting, it’s helpful to understand how JGit manages access to repositories. JGit primarily uses either the file protocol or the HTTP/HTTPS protocol. Based on the protocol in use, several factors can lead to access issues.
Common Access Issues
-
Authentication Failures
- Incorrect credentials
- Missing certificates for HTTPS communication
- Incomplete user configuration in SSH settings
-
Path Configuration Issues
- Invalid file paths
- Incorrect repository URLs
- Repository not found at specified location
-
Network Connectivity Problems
- Network unavailability
- Firewalls and proxy settings blocking Git
- DNS issues when accessing remote repositories
Let's delve into each of these problems and discuss how to troubleshoot them effectively.
1. Authentication Failures
Authentication failures are some of the most frequent issues developers encounter when working with JGit. Here’s how to troubleshoot them:
a. Correct Credentials
When using the HTTP/HTTPS protocol, ensuring that your username and password (or personal access token) are correct is vital. Here's how you can confirm these credentials programmatically:
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.api.TransportCommand;
import org.eclipse.jgit.transport.UsernamePasswordCredentialsProvider;
public class GitAuthentication {
public static void cloneRepo(String repoUrl, String cloneDir, String username, String password) {
try {
Git.cloneRepository()
.setURI(repoUrl)
.setDirectory(new File(cloneDir))
.setCredentialsProvider(new UsernamePasswordCredentialsProvider(username, password))
.call();
System.out.println("Repository cloned successfully.");
} catch (GitAPIException e) {
System.out.println("Error cloning repository: " + e.getMessage());
e.printStackTrace();
}
}
}
This code snippet demonstrates how to use UsernamePasswordCredentialsProvider
to provide credentials for cloning a repository. Always ensure that your password does not include special characters that could interfere with URL parsing.
b. SSH Configuration
If you are using SSH for authentication, ensure your SSH keys are set up correctly. If you encounter "permission denied" errors, you may want to:
- Check your SSH key entry in the SSH agent.
- Confirm that the public key is correctly added to your Git hosting provider (e.g., GitHub, GitLab).
Here’s how you might set a custom SSH command in JGit:
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
public class GitSshConfiguration {
public static void cloneRepoWithSsh(String repoUrl, String cloneDir) {
try {
Git.cloneRepository()
.setURI(repoUrl)
.setDirectory(new File(cloneDir))
.setTransportConfigCallback(transport -> {
SshTransport sshTransport = (SshTransport) transport;
sshTransport.put("StrictHostKeyChecking", "no");
})
.call();
System.out.println("Repository cloned successfully.");
} catch (GitAPIException e) {
System.out.println("Error cloning repository: " + e.getMessage());
e.printStackTrace();
}
}
}
This configuration tells JGit to ignore strict host key checking, which is useful during testing. However, consider security implications when deploying similar code in a production environment.
2. Path Configuration Issues
Path configuration issues can stem from how the Git repository path is specified. Let’s take a closer look at solving these problems.
a. Invalid File Paths
When using the file protocol, ensure that the file path is correctly formatted. For local repositories, this means double-checking that the path truly points to an existing directory.
For example, this code checks if the local repository exists before attempting to access it:
import org.eclipse.jgit.api.Git;
public class LocalRepoAccess {
public static void openLocalRepo(String repoDir) {
File dir = new File(repoDir);
if (dir.exists() && dir.isDirectory()) {
try {
Git git = Git.open(dir);
System.out.println("Opened repository: " + git.getRepository().getDirectory());
} catch (IOException e) {
System.out.println("Could not open repository: " + e.getMessage());
e.printStackTrace();
}
} else {
System.out.println("Directory does not exist or is not a directory.");
}
}
}
b. Correct Repository URLs
For remote repositories, ensuring you have correctly formatted URLs is essential. Use absolute paths when referencing remote repositories.
If you're unsure about the remote URL format, please refer to JGit’s documentation and a Comprehensive Guide on Using Git.
3. Network Connectivity Problems
Network problems are a less frequent but still critical issue that needs troubleshooting. Here are the most essential checks:
a. Check Your Internet Connection
Before attempting to access a remote repository, ensure there’s any underlying connectivity issue. Pinging the Git server can quickly verify if it's reachable.
b. Firewall and Proxy Settings
Sometimes firewalls or proxy settings can block Git traffic. Make sure that any such settings allow communication on the necessary ports (usually TCP port 22 for SSH and 443 for HTTPS).
c. DNS Settings
If you can’t access certain remote repositories, DNS misconfiguration might be the issue. Testing your connectivity with nslookup
or ping
can help diagnose whether the DNS is resolving the URL.
Bringing It All Together
In conclusion, troubleshooting common JGit access issues requires understanding the specifics of authentication, path configurations, and network connectivity. By applying the correct coding practices and understanding the underlying tools, you can minimize the likelihood of such issues arising in your development workflow.
JGit’s flexibility also allows for extensibility through custom configurations, helping you tailor the Git experience to your needs. For further reading, you can check out the JGit Documentation for deep dives into the various features it offers.
Now that you have a fundamental understanding of troubleshooting JGit access issues, go ahead and apply these principles to ensure seamless repository interactions!