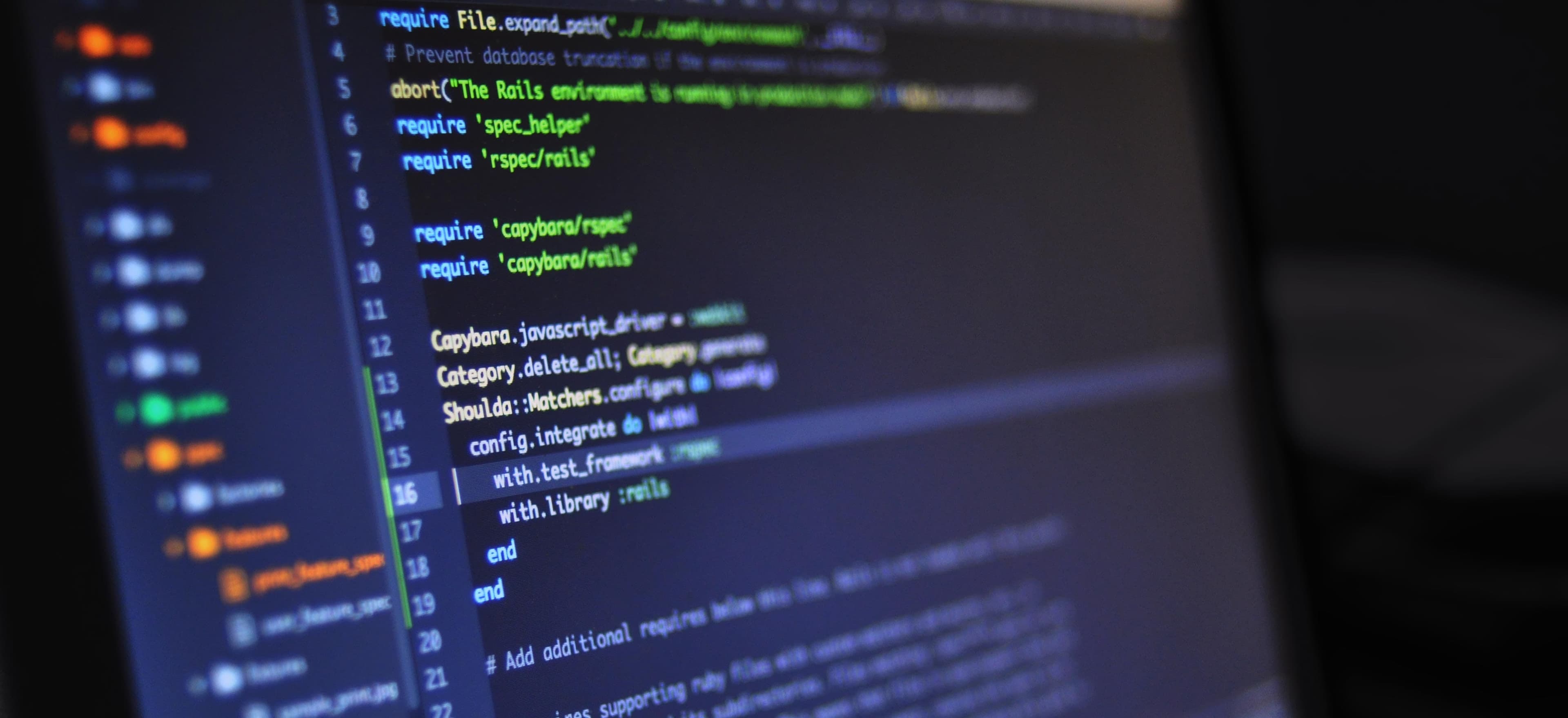
- Published on
Conquering Interface Design: Overcoming User Confusion
In the fast-paced world of software development, the importance of user interface (UI) design cannot be overstated. The effectiveness of an interface can make or break the user experience. As developers and designers, we must ensure that our applications not only function well but also provide clarity and ease of use. In this blog post, we will explore strategies to conquer interface design, focusing on how to eliminate user confusion.
Understanding User Confusion
User confusion often arises from unclear design, complex navigation, or an overwhelming amount of information presented to the user. As developers, we need to empathize with our users. Their perspective guides us in creating better interfaces. According to research, users decide within seconds whether they find a UI intuitive or not.
Here's why confusion can be detrimental:
- Increased Bounce Rates: Users are likely to abandon a site or application if they encounter confusion.
- Negative Brand Image: A disorganized interface can stain your brand's reputation long-term.
- Reduced Engagement: Confused users spend less time interacting with your application.
Key Principles of Effective Interface Design
Simplicity is Key
A clean, uncluttered interface allows users to focus on what matters. Use whitespace generously. It helps users navigate your interface without distraction.
Code Example: Simple Button Component
Here’s how to create a simple button in Java, emphasizing clarity and minimalism:
import javax.swing.JButton;
import javax.swing.JFrame;
public class SimpleButton {
public static void main(String[] args) {
JFrame frame = new JFrame("Simple Button Example");
JButton button = new JButton("Click Me");
button.setBounds(50, 100, 200, 40); // Position and size of the button
frame.add(button);
frame.setSize(300, 300);
frame.setLayout(null);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Why this approach?
- Minimalism: The button has a straightforward action and is easy to identify visually.
- Simplicity in Usage: Users know what to do when they see a button that says, "Click Me."
Consistency Matters
Consistency helps guide users through your interface. This includes consistent terminology, placement of elements, and color schemes. Users develop expectations based on their experiences.
Code Example: Consistent UI Themes
Here's a basic example of applying a consistent theme in a Java Swing application:
import javax.swing.*;
public class ConsistentTheme {
public static void main(String[] args) {
UIManager.put("Button.background", java.awt.Color.BLUE);
UIManager.put("Button.foreground", java.awt.Color.WHITE);
UIManager.put("Button.font", new java.awt.Font("Arial", java.awt.Font.BOLD, 14));
JButton button1 = new JButton("Save");
JButton button2 = new JButton("Cancel");
JFrame frame = new JFrame("Consistent Theme Example");
frame.setLayout(new java.awt.FlowLayout());
frame.add(button1);
frame.add(button2);
frame.setSize(300, 200);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Why enforce consistency?
- User Familiarity: Familiar elements reduce the learning curve. Users can quickly adapt to your interface.
- Professional Appeal: Consistent styling presents a polished and professional image.
Provide Clear Feedback
Feedback reassures users that their actions were recognized. This can be visual, auditory, or tactile. For example, a button that changes color when clicked or a sound that signals an error reinforces user interaction.
Code Example: Feedback Through Button States
Let’s demonstrate feedback in a button interaction:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FeedbackExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Feedback Example");
JButton button = new JButton("Submit");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
button.setText("Submitted!");
button.setEnabled(false); // Disable the button after action
}
});
frame.add(button);
frame.setSize(200, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why is feedback essential?
- User Assurance: Users feel in control when their actions lead to visible results.
- Smooth Transactions: Offers a sense of completion and success.
Optimize Navigation
Navigational elements should be intuitive. A user should understand how to move through the interface without guidance. Use labels and icons that foster understanding.
Code Example: Basic Navigation with JTabbedPane
In Java Swing, organizing content with tabs can simplify navigation:
import javax.swing.*;
public class NavigationExample {
public static void main(String[] args) {
JTabbedPane tabbedPane = new JTabbedPane();
tabbedPane.addTab("Home", new JLabel("Welcome to Home"));
tabbedPane.addTab("Settings", new JLabel("Adjust your preferences here"));
JFrame frame = new JFrame("Navigation Example");
frame.add(tabbedPane);
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why use navigation tabs?
- Efficiency: Allows users to switch between different views quickly.
- Logical Organization: Clear separation of functionalities makes comprehension easier.
Continuous User Testing
User testing is invaluable. Iteratively refining the interface based on user observation will uncover pain points that may not be immediately obvious.
Example of Conducting User Testing
Implementing user feedback can take various forms. One way is to gather real-time data on how users interact with your interface.
- Setup a Recorder: Use tools like Google Analytics or Hotjar.
- Observe Sessions: Look at where users struggle.
- Analyze Feedback: Direct feedback via surveys can yield insights.
Why conduct user testing?
- Informed Design Decisions: Testing reveals real-world usage patterns.
- Enhanced User Experience: Informs you on areas needing improvement, reduces confusion.
Final Considerations
Conquering interface design requires meticulous attention to detail. By adopting principles of simplicity, consistency, clear feedback, intuitive navigation, and continuous user testing, we can overcome user confusion effectively. Remember, investing in interface design today pays dividends in user satisfaction and retention tomorrow.
For more on UI design principles and user experience, consider checking out Nielsen Norman Group and Smashing Magazine. Creating an interface that users understand and appreciate is our goal as developers, and with these strategies, we can achieve that effectively. Happy coding!