Common Mistakes to Avoid in Pull Requests
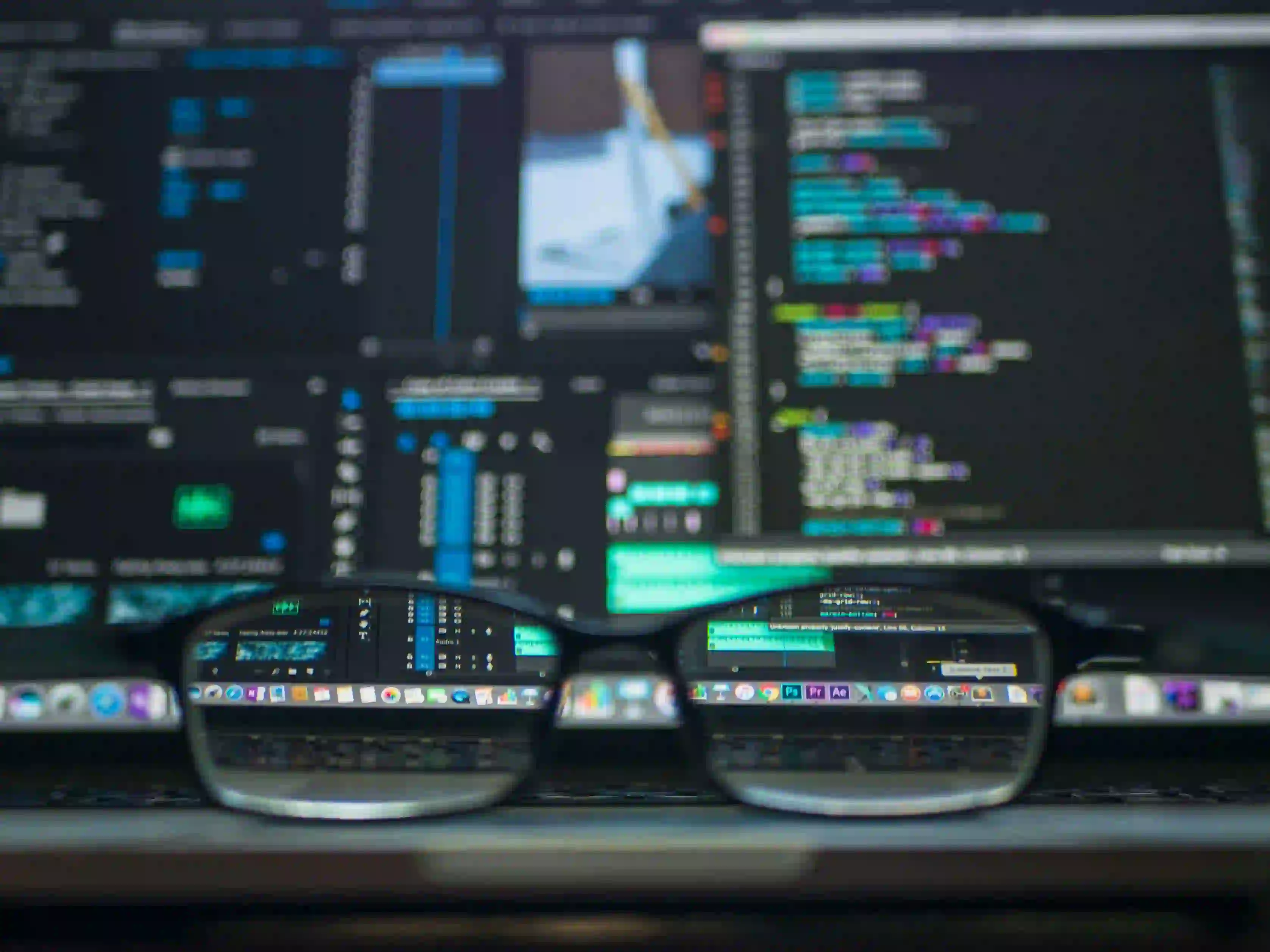
Common Mistakes to Avoid in Pull Requests
Pull requests (PRs) are a crucial part of modern software development. They facilitate code reviews, promote collaboration, and streamline the integration of new features or bug fixes. However, many developers, especially those who are new to the process, often make common mistakes that can hinder the effectiveness of PRs. In this blog post, we will discuss the most prevalent pitfalls to avoid when creating pull requests, along with best practices to enhance collaboration within your team.
1. Not Providing a Clear Description
Why it Matters
A pull request without a clear description can create confusion for reviewers. They may struggle to understand the purpose of the changes, the reasoning behind them, and what specifically they should look at.
The Fix
Always include a detailed description of your PR. Explain the functionality you're adding, bugs you're fixing, or any other changes made. Use bullet points for clarity.
### Description
This pull request:
- Adds a new login feature using OAuth
- Fixes a bug where users could not reset passwords
- Improves UI for mobile devices
### Why:
These changes will enhance user experience and make our app more secure.
2. Large Pull Requests
Why it Matters
Large pull requests can be overwhelming for reviewers. They can lead to overlooked issues and make the review process tedious, increasing the likelihood of bugs slipping into the main codebase.
The Fix
Keep pull requests small and focused. Each PR should ideally encompass one feature or bug fix. This makes the review process faster and more efficient.
**Good Example:**
- PR focused on implementing the new user registration flow.
**Bad Example:**
- A single PR containing user registration, login, password recovery, and UI optimizations.
3. Ignoring Code Style Guidelines
Why it Matters
Inconsistent code style unifies the project's look and feel, making it easier for teams to collaborate. Ignoring style guidelines can lead to friction during reviews.
The Fix
Before submitting a PR, make sure your code adheres to established style guidelines. Use linters to automatically catch inconsistencies.
# Using ESLint for JavaScript
eslint . --fix
4. Failing to Test Your Code
Why it Matters
Submitting code without testing can lead to breaking changes in the codebase. It is essential to test your code to catch runtime errors and logic issues.
The Fix
Before submitting your PR, run all relevant tests, and ensure that they pass. Include new tests for any new functionality or bug fixes.
@Test
public void testUserRegistration() {
// Sample test case for user registration
User user = new User("test@example.com", "password123");
boolean registered = userService.register(user);
assertTrue("User should be registered successfully", registered);
}
5. Not Responding to Reviewer Feedback
Why it Matters
Ignoring reviewer feedback can lead to unresolved issues in your code and can strain team dynamics. Code reviews are an opportunity for learning and improvement.
The Fix
Be open to receiving feedback. Act quickly to address comments and questions, even if itβs to provide clarification or ask follow-up questions.
**Original Comment:**
"This function is too complex. Can we simplify it?"
**Your Response:**
"Thanks for the feedback! Iβll refactor it to improve clarity."
6. Omitting Related Issues or Links
Why it Matters
Not connecting your PR to existing issue trackers can create a disconnect. Reviewers may not see the broader context of your changes.
The Fix
Reference related tickets or issues in your PR description. This provides context and helps reviewers understand the necessity of the changes.
### Related Issues:
- Fixes #123 (Issue with user login)
- Part of the larger initiative in #456 (User security enhancements)
7. Not Using Descriptive Commit Messages
Why it Matters
Commit messages convey the reasoning behind changes. Vague or cryptic messages confuse others who later read through the commit history.
The Fix
Write clear, concise commit messages that explain what has changed and why. Follow a standard convention (like conventional commits) for uniformity.
git commit -m "feat: add OAuth login functionality"
git commit -m "fix: resolve issue with password reset"
8. Mixing Changes in a Single PR
Why it Matters
When multiple, unrelated changes are bundled into one PR, it complicates the review process and can lead to confusion about which aspects of the code belong to which changes.
The Fix
Separate changes into distinct pull requests. Each PR should contain code that relates to a single issue or feature.
**PR #1:** Implement OAuth login.
**PR #2:** Fix password reset issue.
9. Not Squashing Commits
Why it Matters
Having many small commits can clutter the project history, making it difficult to understand the evolution of features over time.
The Fix
Before merging, squash your commits into a single, logical commit. This keeps the commit history clean and easy to read.
# Squash commits using interactive rebase
git rebase -i HEAD~n
Wrapping Up
Pull requests are a vital part of collaborative software development. By avoiding these common mistakes and adhering to best practices, you can make your PRs more effective, facilitating better communication and collaboration within your team.
Remember, beautiful code deserves clear presentation, and clear presentation fosters effective collaboration. Always be open to feedback, be mindful of the code style, and strive to improve your PR practices continually. For further reading on pull request best practices, check out this GitHub guide on collaborating.
By avoiding these pitfalls, you'll not only enhance your code quality but also contribute to a positive and productive team environment. Happy coding!