Common Pitfalls When Starting with JBoss Drools
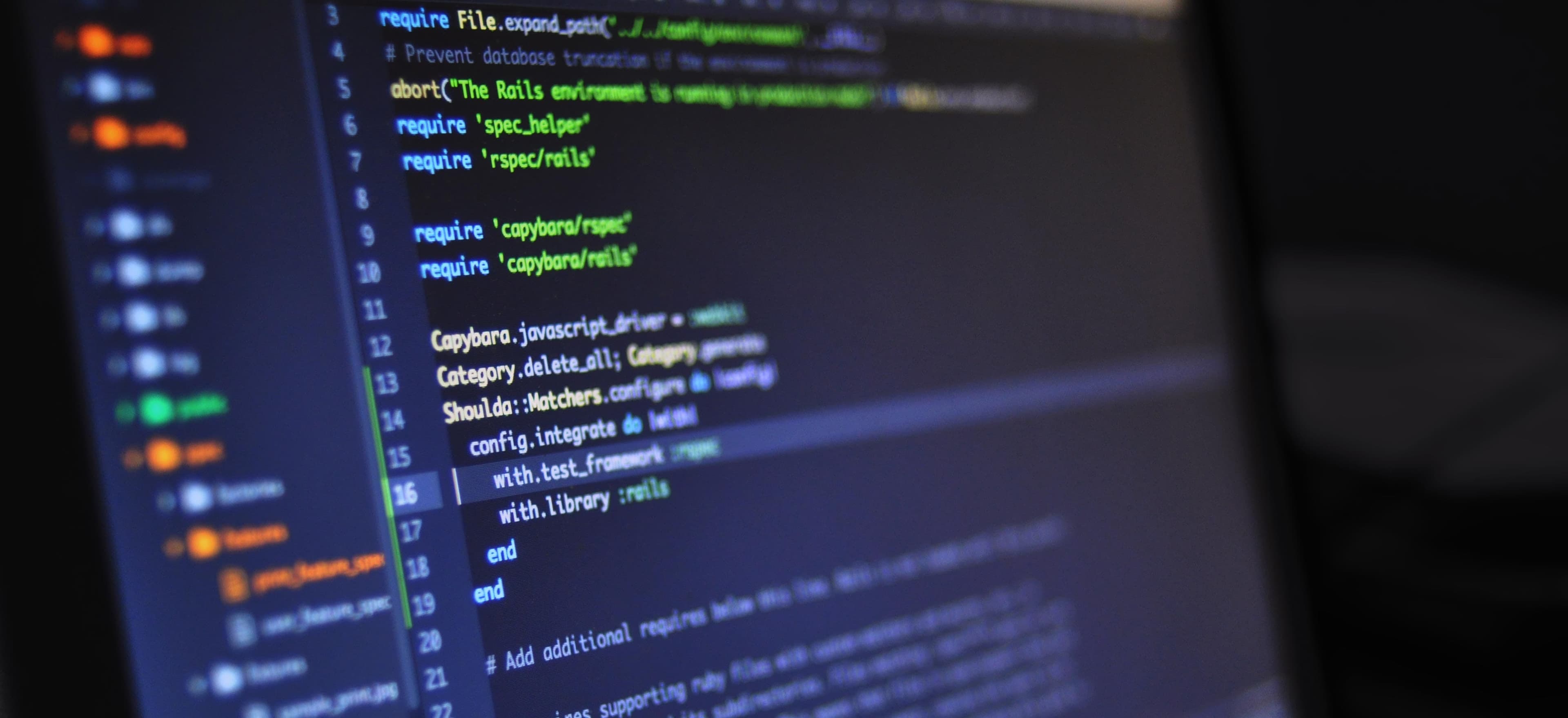
- Published on
Common Pitfalls When Starting with JBoss Drools
As businesses increasingly leverage business rules engines to enhance decision-making processes, JBoss Drools has emerged as a popular choice. However, starting with Drools is not as straightforward as it may seem. New users often encounter various pitfalls that can hinder their experience or lead to inefficient implementations. In this blog post, we will explore these common pitfalls, providing insights and solutions to help you navigate your journey with JBoss Drools effectively.
Overview of JBoss Drools
JBoss Drools is a business rules management system (BRMS) that allows for complex event processing, rules definition, and execution. It promotes the separation of business rules from application code, making it easier to manage, update, and maintain rules.
In essence, Drools enables organizations to:
- Define business logic declaratively.
- Evaluate rules against incoming data, producing actionable insights.
- Change rules without modifying the underlying application code.
Drools is based on the Rete algorithm, making it efficient for evaluating rules in real time. However, to maximize its potential, understanding common pitfalls is crucial.
Pitfall 1: Inadequate Understanding of Rule Syntax
Why It Matters
Drools uses a domain-specific language (DSL) for writing rules. Beginners often misinterpret the syntax or misconfigure rule definitions, leading to ineffective rules and suboptimal performance.
Solution
Familiarize yourself with the Drools rule syntax. Below is a simple example of a rule:
rule "Discount Calculation"
when
Customer( $status : status == "VIP" )
then
$status.setDiscount(0.2);
end
In this snippet, we define a rule that applies a 20% discount to VIP customers. The when
clause specifies the condition to trigger the rule, while the then
clause executes the action.
Best Practices
- Review the Drools documentation to deepen your understanding.
- Test rules frequently during development to catch syntax errors early.
Pitfall 2: Ignoring Rule Prioritization
Why It Matters
Rules in Drools can overlap or conflict. Without explicit prioritization, unpredictable behaviors may arise, leading to incorrect outcomes.
Solution
Use the salience
property to prioritize rules. Here’s an example:
rule "High Priority Discount"
salience 100
when
Customer( $status : status == "VIP" )
then
$status.setDiscount(0.3);
end
rule "Low Priority Discount"
salience 10
when
Customer( $status : status == "Loyal" )
then
$status.setDiscount(0.1);
end
With salience set, the "High Priority Discount" rule will execute before the "Low Priority Discount" rule if a customer is both VIP and Loyal.
Best Practices
- Regularly review rules for potential overlaps and prioritize wisely.
- Use descriptive names for rules to reflect their relevance in your business domain.
Pitfall 3: Poor Data Modeling
Why It Matters
The architecture of your data model can affect the performance of your rules. A poorly structured model may lead to inefficient rule evaluations.
Solution
Develop a well-structured data model using Java classes and ensure your facts align with Drools best practices. For example:
public class Customer {
private String name;
private String status;
private double discount;
// Getters and setters...
}
Best Practices
- Utilize object-oriented principles for designing data models.
- Keep your model simple and relevant to the business context. This alignment allows Drools to perform optimally.
Pitfall 4: Neglecting Testing and Validation
Why It Matters
Skipping testing phases is a common misstep. Effective rules require rigorous testing to confirm they behave as expected.
Solution
Implement a comprehensive testing strategy using JUnit and the Drools testing framework. This helps validate your rules. Here’s a simple JUnit test:
@Test
public void testDiscountCalculation() {
KieSession kSession = getKieSession();
Customer customer = new Customer("John Doe", "VIP", 0);
kSession.insert(customer);
kSession.fireAllRules();
assertEquals(0.2, customer.getDiscount(), 0.001);
}
Best Practices
- Create a suite of unit tests for every rule.
- Use mocks to simulate different scenarios and ensure your rules react appropriately.
Pitfall 5: Overcomplicating Rules
Why It Matters
Creating overly complex rules can make them difficult to understand and maintain. Complex conditions can also slow down performance.
Solution
Break down complex rules into simpler components. Instead of one sizeable complex rule, create smaller rules that are easier to manage.
Example Simplification
Instead of:
rule "Complex Rule"
when
Customer( $status : status == "VIP" || status == "Loyal" )
Product( $price : price > 100 && $category == "Electronics" )
then
applyDiscount($status, $price);
end
Use smaller, distinct rules:
rule "VIP Rule"
when
Customer( status == "VIP" )
then
applyDiscountForVIP();
end
rule "Loyalty Rule"
when
Customer( status == "Loyal" )
then
applyDiscountForLoyal();
end
Best Practices
- Regularly refactor rules to enhance readability.
- Document complex logic for future reference.
Pitfall 6: Missing Out on the Knowledge Base and Sessions
Why It Matters
Proper management of Knowledge Base (KBase) and KieSessions ensures optimal performance and memory usage. Beginners sometimes neglect the benefits of these constructs.
Solution
Create a Knowledge Base and a KieSession as follows:
KieServices kieServices = KieServices.Factory.get();
KieContainer kContainer = kieServices.getKieClasspathContainer();
KieSession kSession = kContainer.newKieSession("ksession-rules");
Best Practices
- Ensure to release sessions after use to avoid memory leaks.
- Familiarize yourself with these constructs via the Kie API.
The Bottom Line
Starting your journey with JBoss Drools can unlock the potential of business rule management, but new users often face common pitfalls that can lead to frustration and inefficiencies. By understanding the importance of rule syntax, prioritization, data modeling, testing, rule simplification, and proper use of Knowledge Bases and KieSessions, you can avoid these traps.
Implementing best practices and leveraging the rich resources available in the Drools community will enhance your experience. Remember, the key to mastering any technology lies in continuous learning and adapting to challenges as they arise.
For more in-depth information on Drools, check out the official Drools website and join community forums for updates and best practices.
Effective rule management can transform your applications, making them intelligent and adaptable to changing business conditions. Happy coding!