Don't Skip Tests: How to Automate Writing Them Efficiently
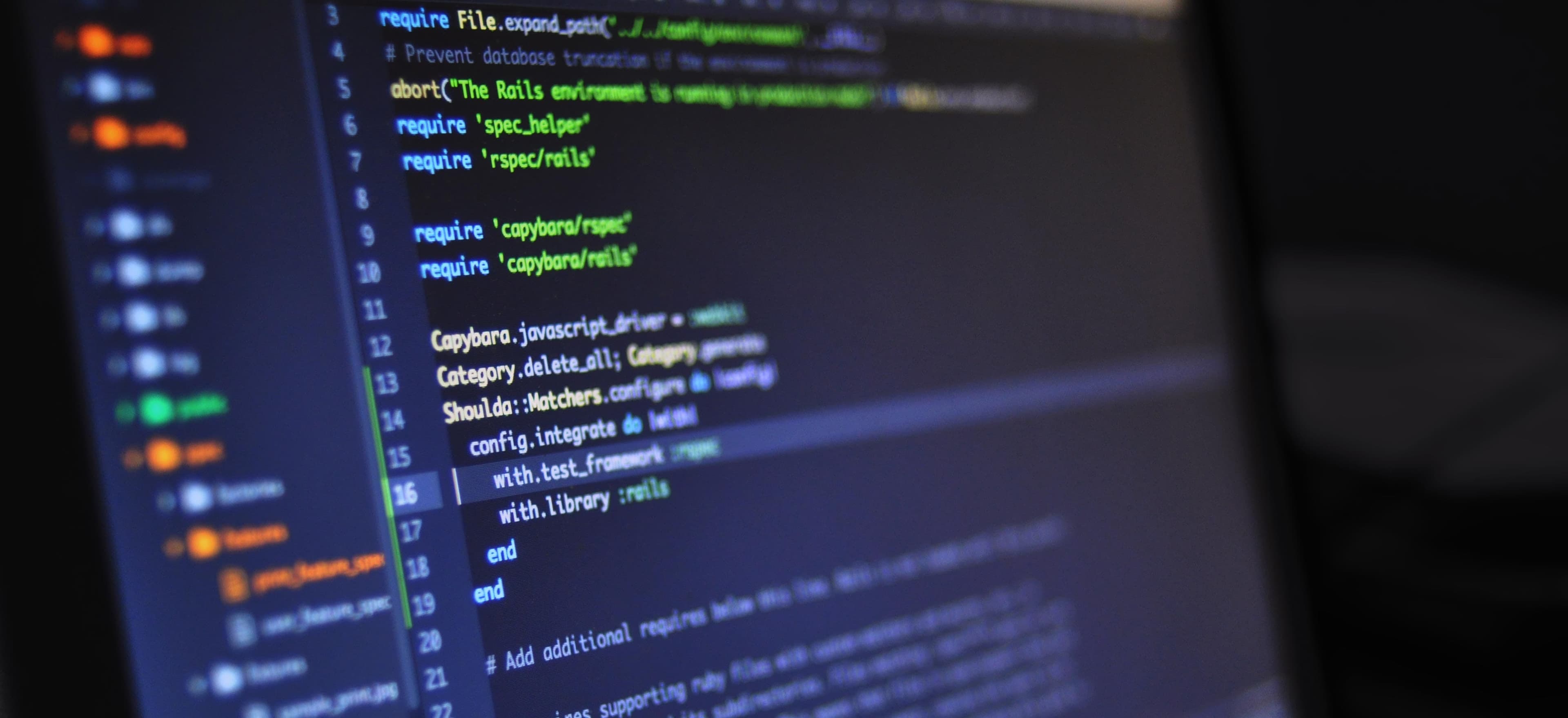
- Published on
Don't Skip Tests: How to Automate Writing Them Efficiently
Automated testing in software development has become more than just a necessity; it is now a fundamental practice. In a world driven by the demand for rapid delivery and high-quality applications, testing automation can significantly improve the development workflow. However, the challenge often lies in creating and managing efficient automated tests. This blog post will delve into techniques for automating test writing effectively in Java, emphasizing the "why" behind the methods to enhance your understanding and implementation.
The Importance of Testing
Before diving into automation, it's crucial to understand why testing is vital:
- Quality Assurance: Automated tests help ensure that your code behaves as expected, reducing the chances of bugs making it to production.
- Faster Feedback Loop: Automation provides immediate feedback on changes in code, allowing developers to detect issues early.
- Consistency: Automated tests can be run multiple times without variance, providing consistent results.
- Refactoring Confidence: With a comprehensive suite of automated tests, developers can refactor code with confidence, knowing that tests will catch any errors introduced during the refactor.
The key takeaway here is that automated testing not only saves time but also enhances the overall quality of the software.
Setting Up Your Java Testing Environment
To begin, ensure you have a suitable testing framework in place. The popular choices include:
- JUnit: A widely-used framework for writing unit tests in Java.
- TestNG: An advanced, more flexible framework that supports group testing and data-driven testing.
We will focus on JUnit for this blog, as it is simple and highly effective for unit testing.
Maven Dependencies
If you're using Maven, you'd need to add the following dependencies to your pom.xml
:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
This ensures that JUnit is available in your development environment, allowing you to write and execute tests seamlessly.
Writing Your First Test
Let's examine a straightforward example. Suppose you have a method that adds two integers. Your class might look like this:
public class MathUtils {
public int add(int a, int b) {
return a + b;
}
}
Now, let's see how to automate the writing of tests for this method using JUnit.
import org.junit.Assert;
import org.junit.Test;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
int result = mathUtils.add(5, 10);
// Assert that the result is as expected
Assert.assertEquals(15, result);
}
}
Why This Approach?
- Isolation: Each test case is independent. A failure in one does not affect others.
- Clear Intent: The use of assertions immediately communicates the intention of the test.
- Automatic Execution: Using JUnit, the tests can be executed automatically, providing quick feedback.
For a more in-depth introduction to JUnit and its features, consider visiting JUnit 5 User Guide.
Strategies for Writing Efficient Tests
1. Use Test-Driven Development (TDD)
TDD is a proven methodology where you write tests before writing the actual code. Although it may seem counterintuitive initially, it forces you to think through your requirements before you start coding. Here’s how you might approach it:
- Write a failing test.
- Write the minimal code to pass the test.
- Refactor the code and ensure all tests still pass.
This cycle not only improves the design of your code but also ensures thorough coverage.
2. Parameterized Testing
JUnit allows parameterized tests, enabling you to run the same test with different inputs. This is particularly useful for testing methods that handle various parameters and edge cases.
Example:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class MathUtilsTestParameterized {
private int a;
private int b;
private int expectedSum;
public MathUtilsTestParameterized(int a, int b, int expectedSum) {
this.a = a;
this.b = b;
this.expectedSum = expectedSum;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, 2, 3},
{10, 20, 30},
{100, 200, 300}
});
}
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
Assert.assertEquals(expectedSum, mathUtils.add(a, b));
}
}
Why Parameterized Testing?
- Efficiency: One test class can cover multiple scenarios, making your tests cleaner and easier to maintain.
- Clarity: All inputs and their expected outputs are visible in one place.
- Scalability: As your application logic grows, new scenarios can simply be added to the
data()
method.
3. Leverage Mocking Frameworks
Using mocking, you can simulate the behavior of complex classes and isolate the class under test. Libraries like Mockito are prevalent for mocking in Java.
Example:
import static org.mockito.Mockito.*;
public class ServiceTest {
@Test
public void testServiceMethod() {
// Arrange
Dependency dependency = mock(Dependency.class);
when(dependency.call()).thenReturn("Mocked Result");
Service service = new Service(dependency);
// Act
String result = service.serviceMethod();
// Assert
Assert.assertEquals("Expected Result", result);
}
}
Why Use Mocking?
- Isolation: It allows you to isolate the class you're testing by replacing its dependencies.
- Control: Easily control the behavior of mock objects, ensuring specific outcomes for various scenarios.
- Speed: Tests run faster since they avoid the overhead of slow operations like network calls or database queries.
Continuous Integration and Test Automation
Setting up a Continuous Integration (CI) pipeline is crucial for consistently running automated tests. Tools like Jenkins, Travis CI, or GitHub Actions allow you to automatically execute your test suite every time code is pushed to the repository.
Steps to Configure CI:
- Create a configuration file specific to the CI tool you choose.
- Define the build process, including running tests after compiling.
- Set notifications for failed builds, allowing developers to address issues promptly.
For example, GitHub Actions configuration might look like this:
name: Java CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
- name: Run tests
run: mvn test
The Benefits of CI
- Immediate Feedback: Developers receive quick feedback on their code.
- Reduced Integration Problems: Early detection of issues when merging changes.
- Discipline: Encourages a culture of maintaining tests and clean code.
Final Thoughts
Automating tests in Java is not just about writing code to ensure your methods work - it's about creating a robust environment for quality software development. With the strategies outlined above, including TDD, parameterized testing, and mocking, you can enhance your testing process significantly. These practices don’t merely save you time; they also fortify your code, allowing you to innovate faster and with greater confidence.
Don't skip tests. Automate them effectively, and watch your productivity and code quality soar. Happy coding and testing!
For further reading on effective Java testing, refer to Effective Java by Joshua Bloch for insightful best practices in Java programming.
Checkout our other articles