Solving Java Mars Rover Challenges: Common Pitfalls to Avoid
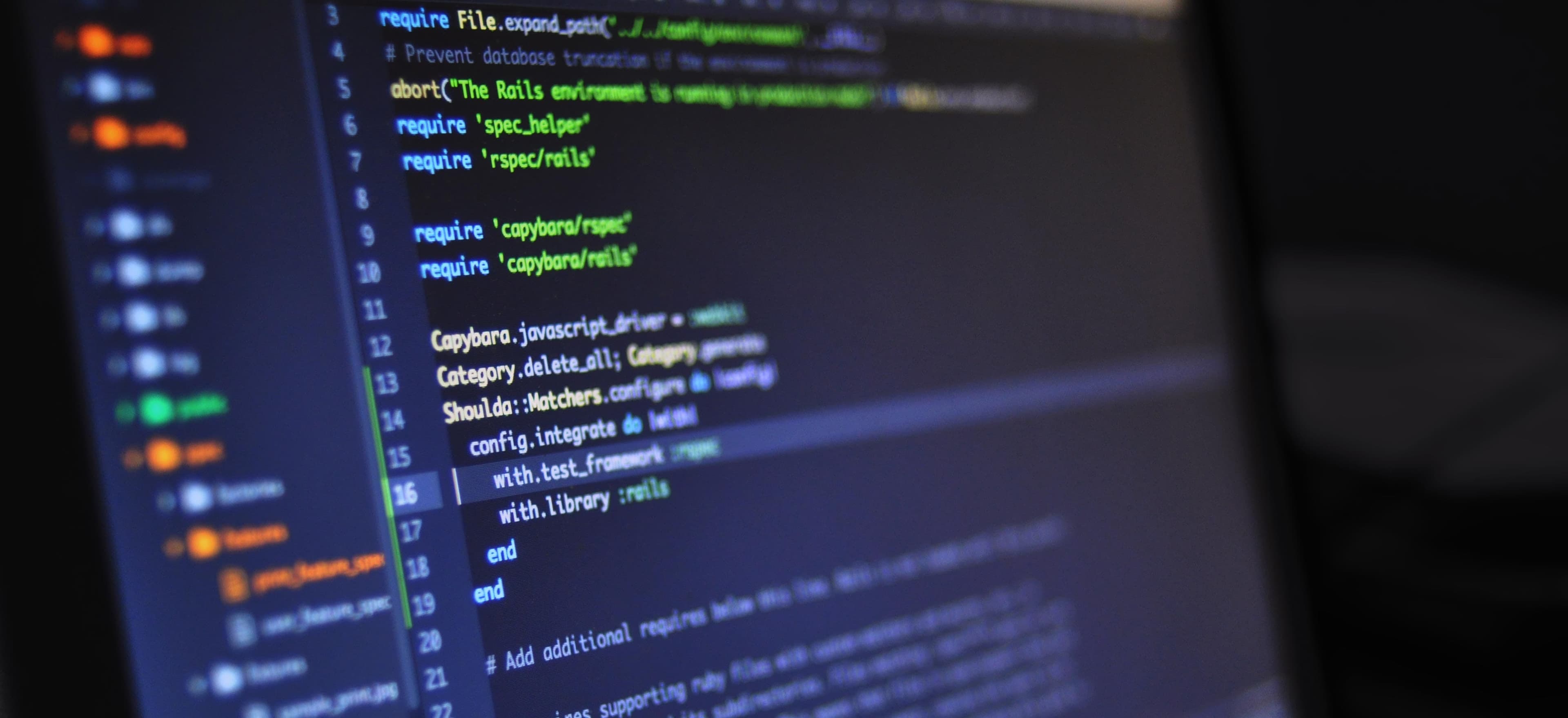
- Published on
Solving Java Mars Rover Challenges: Common Pitfalls to Avoid
The Mars Rover challenges have become a popular way to test your programming skills, particularly if you're using Java. It’s not just a fun project; it also serves as an opportunity to enhance problem-solving capabilities and grasp core programming concepts. However, many developers face pitfalls that can derail their success. In this post, we will explore common mistakes to avoid while tackling the Mars Rover challenges, offer tips for effective coding, and provide insightful code snippets with commentary.
Understanding the Mars Rover Challenge
Before diving into pitfalls, let’s clarify what the Mars Rover challenge typically involves. Generally, the objective is to control a rover on a grid representing the Martian surface. The rover must navigate, receive commands, and report its location. This requires an understanding of object-oriented programming (OOP) principles, data structures, and algorithm design.
Common Pitfalls
-
Ignoring OOP Principles
Object-Oriented Programming is crucial for the rover's design. Developers often hastily create procedural code without encapsulating behaviors and properties.
Why It Matters: Proper use of OOP allows for modularity and reusability of code.
Solution: Design classes for the Rover, the Grid, and possibly Command. Here’s how you could structure the Rover class:
public class Rover { private int x; private int y; private char direction; public Rover(int x, int y, char direction) { this.x = x; this.y = y; this.direction = direction; } public void moveForward() { // implementation } // Other relevant methods... }
In this snippet, encapsulating the rover’s position and the direction makes the rover easier to manage and extend.
-
Not Validating Input Commands
It's essential to validate commands sent to the rover to avoid unexpected behaviors. Many beginners skip this step.
Why It Matters: Command validation prevents exceptions and incorrect rover movements.
Solution: Implement an
isCommandValid
method:public boolean isCommandValid(String command) { return command.matches("[LRM]"); }
This regex checks that the command consists of 'L', 'R', or 'M'. Validation ensures robustness amid user error.
-
Failure to Handle Edge Cases
Beginning programmers might overlook how the rover handles boundaries of the grid. For instance, what happens if the rover tries to move beyond the grid?
Why It Matters: Edge case handling is crucial for building reliable applications.
Solution: Include boundary checks in the move logic.
public void moveForward() { switch (direction) { case 'N': if (y + 1 < gridHeight) y++; break; case 'E': if (x + 1 < gridWidth) x++; break; case 'S': if (y - 1 >= 0) y--; break; case 'W': if (x - 1 >= 0) x--; break; } }
This method includes checks to ensure the rover doesn’t move out of bounds, promoting stability in your application.
-
Neglecting Unit Testing
Many new developers underestimate the importance of unit testing. They might think, "My code works, so why test it?"
Why It Matters: Unit tests help catch bugs early and ensure consistent functionality as your codebase grows.
Solution: Adopt a testing framework, such as JUnit, and write tests for your rover's methods.
@Test public void testMoveForward() { Rover rover = new Rover(0, 0, 'N'); rover.moveForward(); assertEquals(0, rover.getY()); assertEquals(1, rover.getX()); }
This test checks that the rover moves forward correctly. As you add features, tests will ensure that nothing breaks.
-
Overcomplicating Direction Changes
Handling direction may seem straightforward, but a lot of rookie developers complicate it unnecessarily.
Why It Matters: A complicated implementation can lead to bugs and hard-to-read code.
Solution: Use enums to represent directions clearly.
public enum Direction { NORTH, EAST, SOUTH, WEST } private Direction direction; public void turnLeft() { direction = Direction.values()[(direction.ordinal() + 3) % 4]; }
Using enums makes direction management cleaner and easier to understand. Each method can clearly define what happens on turning.
Tips for Successful Implementation
-
Break Down the Problem: Tackle one feature or command at a time. This method makes debugging easier and helps you maintain focus.
-
Use Comments Wisely: Comments are not just for others but for your future self. Annotate complex pieces of code to explain your thought process.
-
Refactor Regularly: After getting something to work, revisit your code and see where you can simplify or enhance readability without changing behavior.
-
Consult Resources: Use online platforms like Stack Overflow and the Java documentation regularly.
Lessons Learned
The Mars Rover challenge serves as an excellent education tool tapping into critical programming concepts. By avoiding common pitfalls such as ignoring OOP principles, failing to validate commands, neglecting edge cases, and cutting corners on testing, you can significantly enhance your programming abilities. By taking the time to build your rover with care and structure, you will not only solve the challenge but also create a codebase that you can be proud of.
Whether you're a beginner or an experienced developer, remember that writing clean, maintainable code will always pay dividends in the long run. Dive into these practices, and you’ll navigate the roads of Mars with confidence.
Happy coding!