5 Common Android Pitfalls and How to Avoid Them
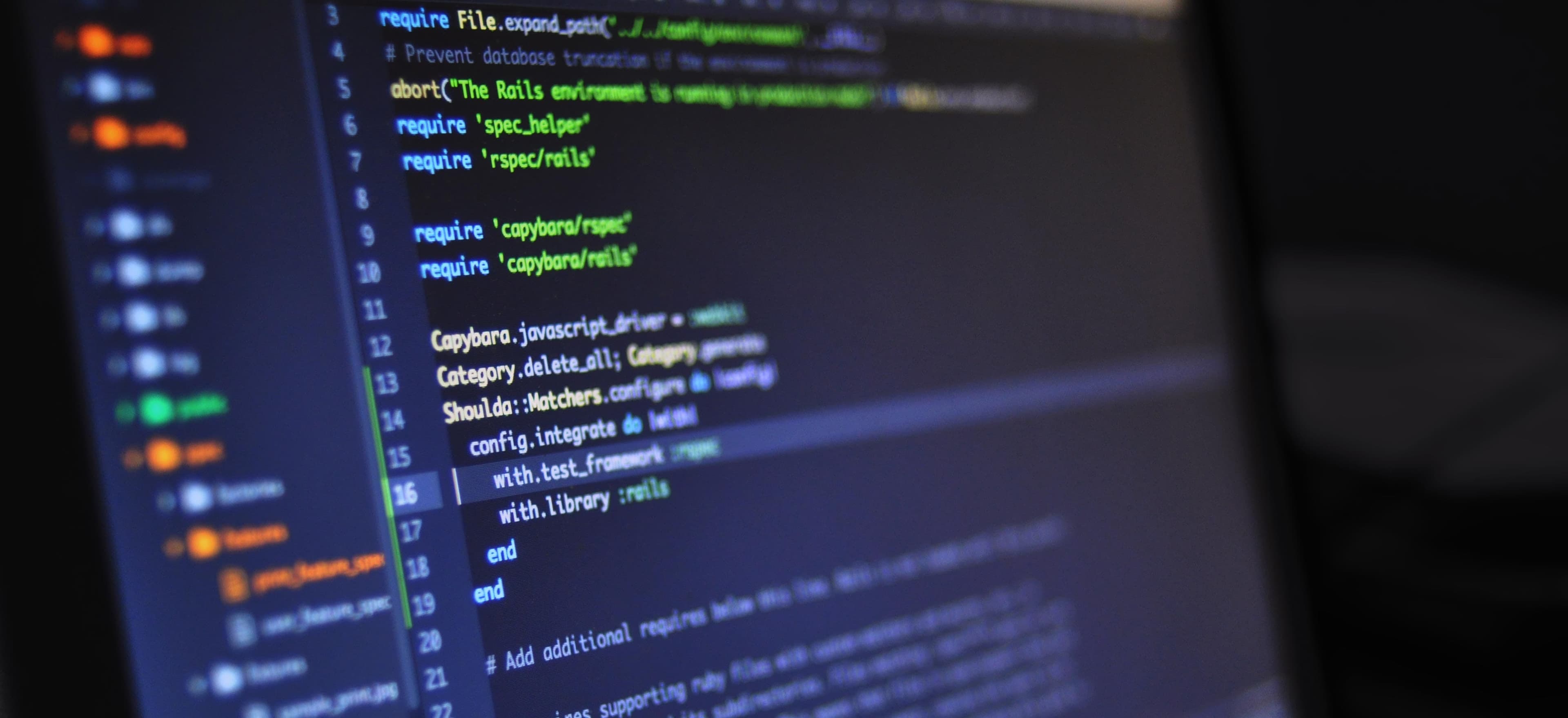
- Published on
5 Common Android Pitfalls and How to Avoid Them
Developing Android applications can be an exciting journey. However, it's littered with potential pitfalls that can lead to frustrating bugs, poor performance, or bad user experience. In this blog post, we will explore five common pitfalls faced by Android developers and provide solutions to avoid them.
1. Neglecting Performance Optimization
Android devices come in various specifications and capabilities. Neglecting performance can lead to a sluggish app, causing users to abandon it.
Solutions:
- Use Lazy Loading: Load data as needed instead of all at once.
// Load images efficiently using Glide
Glide.with(context)
.load(imageUrl)
.placeholder(R.drawable.placeholder)
.into(imageView);
By only loading images when needed, you prevent your app from consuming excessive resources upfront.
- Background Processing: Use background threads for tasks that take time.
new Thread(() -> {
// Simulate time-consuming task
Thread.sleep(2000);
runOnUiThread(() -> {
// Update UI once task is complete
});
}).start();
This prevents the UI from freezing, maintaining a smooth user experience.
Additional Readings:
If you're interested in learning more about performance optimization, consider checking out Android's official performance guidelines for an in-depth understanding.
2. Ignoring the Lifecycle of Activities and Fragments
Android’s activity and fragment lifecycles are critical to managing resources properly. Mismanagement can lead to memory leaks or incorrect app behaviors.
Solutions:
- Understand Lifecycle Events: Familiarize yourself with the lifecycle methods like
onCreate()
,onPause()
, andonDestroy()
.
@Override
protected void onPause() {
super.onPause();
// Stop tasks or release resources
}
By stopping tasks in onPause()
, you free up resources when the app is not in the forefront, improving performance.
- Use ViewModels with LiveData: It helps to manage UI-related data in a lifecycle-conscious way.
public class MainViewModel extends ViewModel {
private MutableLiveData<String> data;
public LiveData<String> getData() {
if (data == null) {
data = new MutableLiveData<>();
loadData();
}
return data;
}
private void loadData() {
// load data here
}
}
Using ViewModels ensures that data survives configuration changes like screen rotations.
3. Poor Resource Management
When it comes to app resources—like images, strings, and layouts—many developers overlook how properly managing these can improve performance and maintainability.
Solutions:
- Use Vector Drawables: Instead of bitmaps, vector drawables scale better and use less memory.
<androidx.appcompat.widget.AppCompatImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="@drawable/ic_vector" />
With vector drawables, you’re reducing the memory complexity and ensuring sharper images on high-resolution screens.
- Avoid Hardcoding Strings: Store strings in the
strings.xml
file for easier localization and maintenance.
<resources>
<string name="app_name">My Android App</string>
</resources>
This makes your app easily translatable and keeps your code cleaner.
Learn More:
For thorough insights on optimizing resources in Android, visit Android's official documentation on best practices.
4. Not Testing on Multiple Devices
One of the biggest oversights in Android development is failing to test applications on various devices and OS versions.
Solutions:
- Utilize Android Emulators: Test your application in different configurations with the Android Virtual Device (AVD) manager.
# Command to start a new emulator from the command line
emulator -avd Pixel_3_API_30
By simulating multiple devices, you gain insights into how your app performs under varying conditions.
- Manual Testing on Real Devices: Whenever possible, test your app on physical devices.
Automation Can Help:
Incorporating testing frameworks like Espresso for UI testing can catch issues early.
// Espresso test example
onView(withId(R.id.my_button)).perform(click());
Automated UI tests can simulate user interactions and verify that the app behaves as expected.
5. Overlooking Error Handling
Proper error handling enhances user experience and makes debugging easier. Ignoring it can lead to crashes or bad user interactions.
Solutions:
- Use Try-Catch Blocks: Wrap potentially risky code in try-catch statements to manage exceptions gracefully.
try {
// Code that might throw exceptions
} catch (Exception e) {
Log.e("TAG", "An error occurred", e);
}
Proper logging allows you to understand the context of errors when they occur.
- Global Exception Handling: Implement a custom UncaughtExceptionHandler to capture unhandled exceptions.
Thread.setDefaultUncaughtExceptionHandler((thread, throwable) -> {
// Handle exception here, perhaps log it or show a message
});
This ensures that your app doesn't crash silently and allows for recovery or reporting.
Bringing It All Together
The journey of Android development can be full of obstacles, but awareness of common pitfalls helps in crafting better apps. By focusing on performance optimization, understanding the lifecycle, managing resources effectively, testing across devices, and implementing robust error handling, you will not only enhance the quality of your app but also improve the user experience.
To recap, here are five common pitfalls and their solutions:
- Neglecting Performance Optimization: Use lazy loading and background processing.
- Ignoring Activity and Fragment Lifecycles: Familiarize yourself with lifecycle events and utilize ViewModels.
- Poor Resource Management: Use vector drawables and store strings in
strings.xml
. - Not Testing on Multiple Devices: Use emulators and conduct manual testing.
- Overlooking Error Handling: Implement try-catch blocks and global exception handling.
Recognizing these areas and adopting a proactive approach will set you up for success in your Android development endeavors. Keep coding and never stop learning!
Checkout our other articles