Fixing Memory Leaks in JavaFX Touch Gesture Implementations
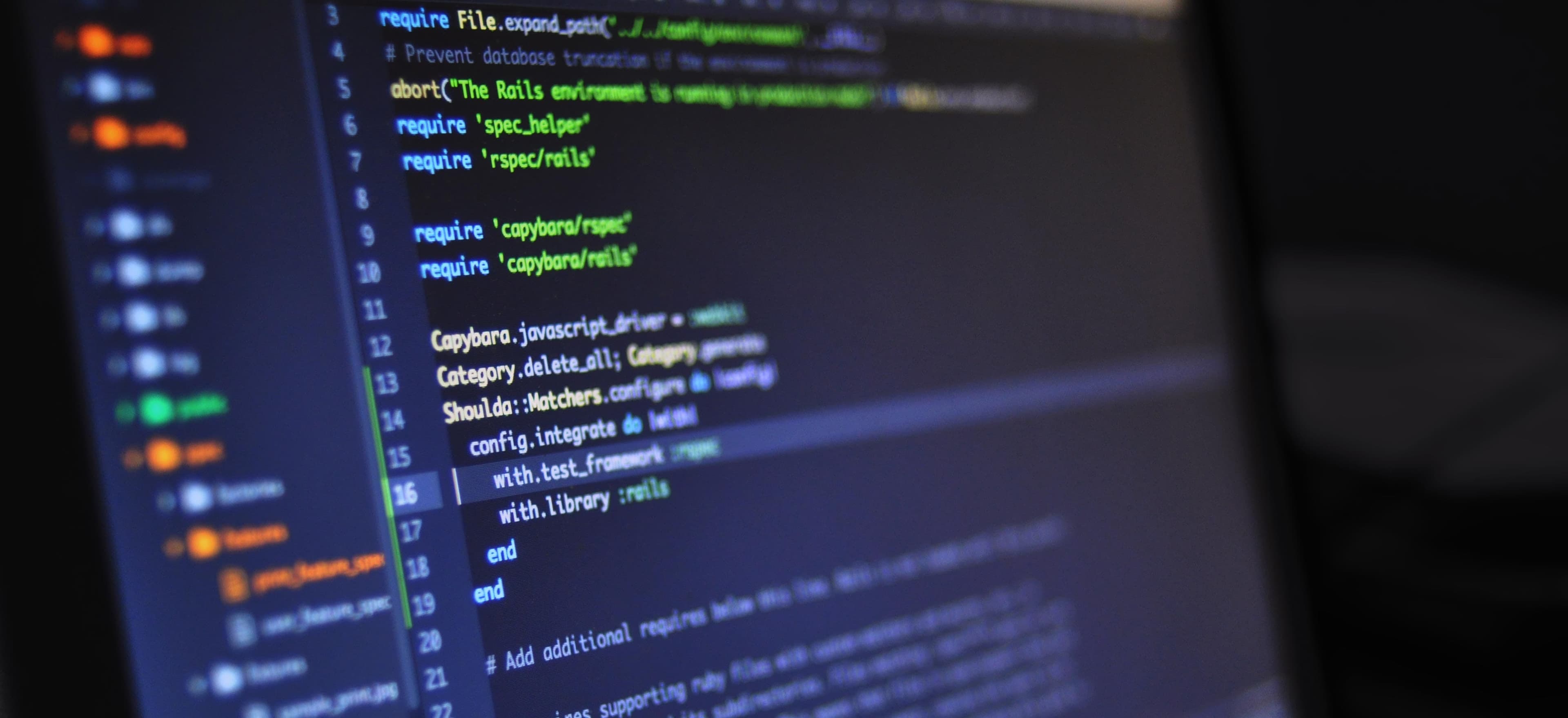
- Published on
Fixing Memory Leaks in JavaFX Touch Gesture Implementations
Memory leaks are a common challenge in Java applications, and they can be particularly troublesome when using JavaFX for GUI development. JavaFX offers a rich set of APIs for creating touch-based applications, but incorrect handling of event listeners and object references can lead to memory leaks.
In this blog post, we will explore the concept of memory leaks with a focus on touch gesture implementations in JavaFX. We will discuss what memory leaks are, how they occur in JavaFX applications, especially with touch gestures, and most importantly, how to identify and fix them.
What is a Memory Leak?
A memory leak occurs when an application allocates memory for objects that are no longer in use but fails to release that memory back to the environment. In Java, this typically happens when references to unused objects are still retained. The garbage collector cannot reclaim this memory, leading to increased memory consumption and potentially causing application performance issues or crashes.
How Memory Leaks Occur in JavaFX Touch Gestures
JavaFX applications commonly use event listeners to handle user interactions, including touch gestures. While registering for events is straightforward, removing these event listeners when they are no longer needed is crucial. If event listeners are not removed properly, they prevent the garbage collector from reclaiming memory occupied by the objects they reference.
Here's a typical pattern in JavaFX for handling touch gestures:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.input.GestureEvent;
import javafx.scene.input.TouchEvent;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class TouchGestureExample extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 250);
// Adding touch event handlers
root.addEventFilter(TouchEvent.TOUCH_PRESSED, this::handleTouch);
root.addEventFilter(GestureEvent.GESTURE_DETECTED, this::handleGesture);
primaryStage.setTitle("Touch Gesture Example");
primaryStage.setScene(scene);
primaryStage.show();
}
private void handleTouch(TouchEvent event) {
// Handle touch event
System.out.println("Touch detected at: " + event.getTouchPoint());
event.consume(); // Consume the event
}
private void handleGesture(GestureEvent event) {
// Handle gesture event
System.out.println("Gesture detected: " + event.getGestureSource());
event.consume(); // Consume the event
}
public static void main(String[] args) {
launch(args);
}
}
Why Memory Leaks Occur Here
In this example, we have registered event filters for touch and gesture events. If the StackPane
(root
) is removed from the scene graph or replaced, the listeners are still retained in memory if not removed properly. This leads to a memory leak because the StackPane
cannot be garbage collected.
Identifying Memory Leaks
To diagnose memory leaks, you can use various profiling tools, such as:
- VisualVM: A comprehensive monitoring and troubleshooting tool that comes with the JDK.
- Eclipse Memory Analyzer (MAT): An advanced tool for analyzing Java heap dumps.
When using these tools, look for instances of objects that you expect to be garbage collected still present in memory.
Fixing Memory Leaks in JavaFX
To fix memory leaks, you should ensure to unregister event listeners when they are no longer needed. This can typically be done in the following scenarios:
- If a node is removed from the scene graph.
- If an object that listens to events is no longer required.
Here’s how you can modify the previous example to ensure proper unregistration of listeners:
Example with Unregistration
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.input.GestureEvent;
import javafx.scene.input.TouchEvent;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class TouchGestureExample extends Application {
private StackPane root;
@Override
public void start(Stage primaryStage) {
root = new StackPane();
Scene scene = new Scene(root, 300, 250);
// Adding touch event handlers
root.addEventFilter(TouchEvent.TOUCH_PRESSED, this::handleTouch);
root.addEventFilter(GestureEvent.GESTURE_DETECTED, this::handleGesture);
primaryStage.setTitle("Touch Gesture Example");
primaryStage.setScene(scene);
primaryStage.setOnCloseRequest(event -> removeListeners()); // Unregister on close
primaryStage.show();
}
private void handleTouch(TouchEvent event) {
System.out.println("Touch detected at: " + event.getTouchPoint());
event.consume();
}
private void handleGesture(GestureEvent event) {
System.out.println("Gesture detected: " + event.getGestureSource());
event.consume();
}
private void removeListeners() {
root.removeEventFilter(TouchEvent.TOUCH_PRESSED, this::handleTouch);
root.removeEventFilter(GestureEvent.GESTURE_DETECTED, this::handleGesture);
System.out.println("Event listeners removed.");
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of Changes
-
Unregistering Listeners: The method
removeListeners()
is created to unregister the event filters. This method is called when the primary stage is closed. This prevents the listeners from holding references to theStackPane
. -
Increased Awareness: By carefully managing event listeners, you ensure that if the
root
is no longer part of the scene, it can be garbage collected properly.
The Last Word
Managing memory effectively in JavaFX applications is crucial, especially when implementing touch gestures. By understanding how memory leaks occur due to untracked event listeners, you can avoid potential pitfalls.
In this blog post, we covered:
- The nature of memory leaks.
- How they can occur in JavaFX applications, particularly with touch gestures.
- Effective strategies to identify and fix these leaks by properly managing event listeners.
You can learn more about JavaFX event handling in the official JavaFX documentation. Furthermore, consider using tools like VisualVM for memory leak diagnosis in your applications.
By implementing these techniques, you can create performant, memory-efficient JavaFX applications. Happy coding!
Checkout our other articles