Visualizing Spring Application Startup: Key Challenges Explained
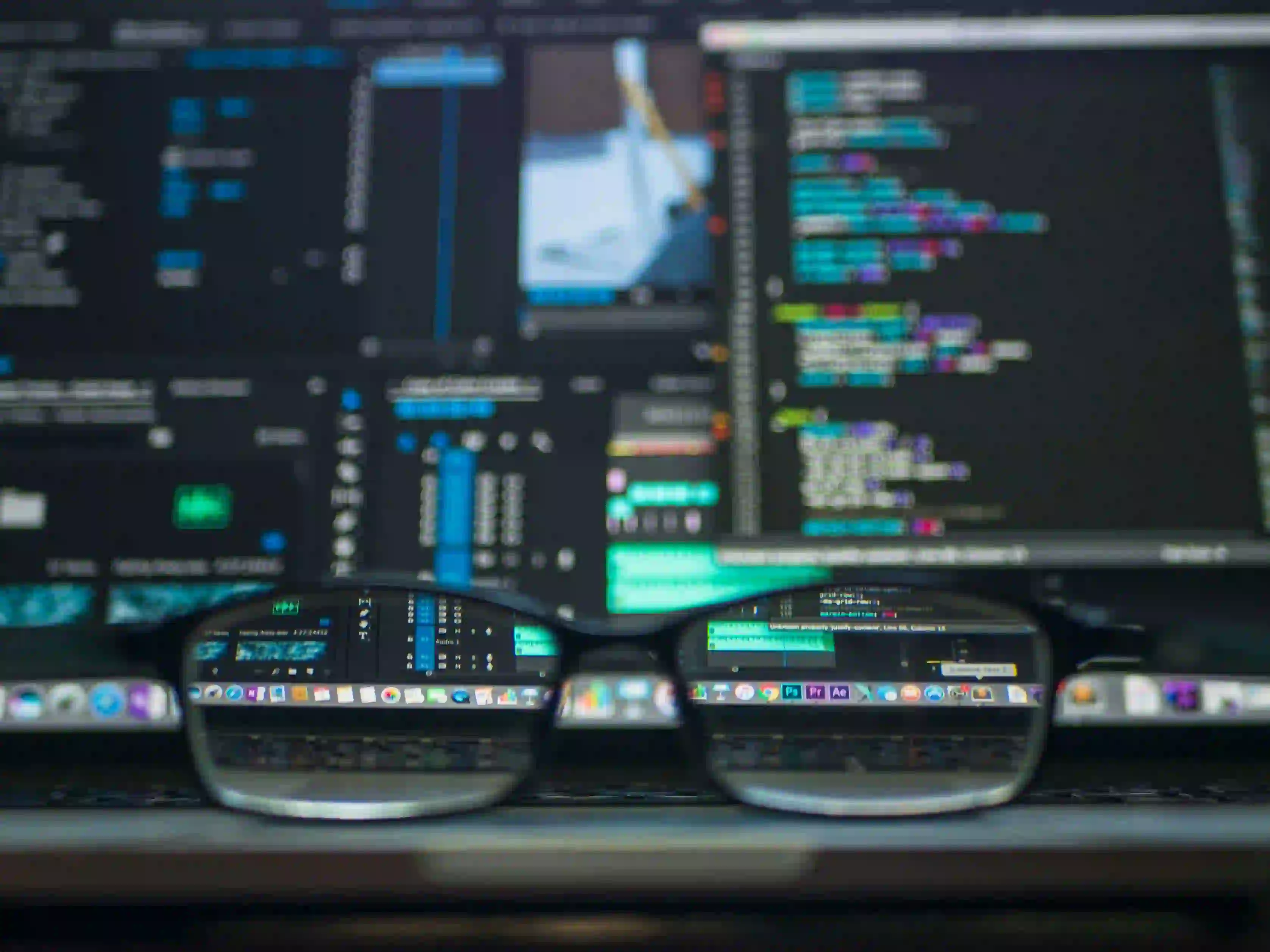
Visualizing Spring Application Startup: Key Challenges Explained
Spring is one of the most popular frameworks used for building Java applications. Known for its robust ecosystem, Spring enables developers to build scalable, maintainable, and production-ready applications. However, just like any framework, the startup processes can introduce unique challenges. This blog post aims at visualizing the Spring application startup while discussing key challenges and solutions in a concise yet informative manner.
Understanding the Spring Application Startup Process
Before we delve into the challenges, let's clarify what happens during the Spring application startup. The process can typically be broken down into several phases:
1. Bean Creation
Spring relies heavily on the concept of beans. Beans are objects that form the backbone of your application and are managed by the Spring IoC (Inversion of Control) container.
2. Dependency Injection
Once the beans are created, the next step is Dependency Injection. This feature allows for the automatic resolution of dependencies between different components.
3. Context Initialization
The application context is initialized. This is where configuration settings are read, and any additional event listeners are set up.
4. Application Startup
Finally, the application is ready to serve requests, but not without potential hiccups along the way.
Understanding these stages is vital, as it provides a context for the challenges we will discuss.
Key Challenges in Spring Application Startup
Challenge 1: Long Startup Times
One of the most common issues faced by developers is prolonged startup times. As applications grow and the number of beans increases, so does the time taken for them to be initialized.
Solution: Lazy Initialization
Switching on lazy initialization allows beans to be created only when they are needed instead of at startup. You can enable this feature simply by modifying your configuration.
@Configuration
@EnableLazy
public class AppConfig {
@Bean
public SomeService someService() {
return new SomeServiceImpl();
}
}
Why: Lazy initialization dramatically improves startup times and conserves resources, especially for larger applications where not all beans are needed immediately.
Challenge 2: Circular Dependencies
Circular dependencies occur when two or more beans require each other for initialization. This situation can either lead to application crashes or cause significant complexity in debugging.
Solution: Use Setter Injection
Instead of constructor injection, you can opt for setter injection, which can break the circular dependency chain.
public class BeanA {
private BeanB beanB;
public void setBeanB(BeanB beanB) {
this.beanB = beanB;
}
}
public class BeanB {
private BeanA beanA;
public void setBeanA(BeanA beanA) {
this.beanA = beanA;
}
}
Why: This approach significantly reduces the risk of circular dependencies by allowing the dependencies to be injected after the beans are created.
Challenge 3: Configuration Complexity
With complex applications, the configuration of beans can become convoluted. This often leads to misconfigurations that can break the application during startup.
Solution: Profile-Based Configuration
Spring profiles allow you to segregate different configurations for various environments (development, testing, production). This way, you can maintain simpler configurations while still accommodating diverse requirements.
# application-dev.yml
spring:
datasource:
url: jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1
jpa:
hibernate:
ddl-auto: update
# application-prod.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/prod_db
jpa:
hibernate:
ddl-auto: none
Why: This segmentation not only simplifies configuration but also mitigates the chances of mistakes when deploying to various environments.
Challenge 4: Monitoring Application Lifecycle
Monitoring the startup process can be a challenge as developers often work in dark. It can be hard to determine why a specific segment is taking longer than expected.
Solution: Actuator
Spring Boot's Actuator module offers ready-made endpoints that allow you to monitor and interact with your application. You can retrieve metrics specifically about the startup process.
@Bean
public WebMvcConfigurer webMvcConfigurer() {
return new WebMvcConfigurerAdapter() {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new StartupInterceptor());
}
};
}
Why: This enables you to monitor the beans being instantiated and helps identify bottlenecks in real-time.
Challenge 5: Resource Management
Inefficient resource management during startup can cause the application to consume excessive memory and CPU resources, leading to slow startup and potential crashes.
Solution: Profiling Tools
Using profiling and performance tools such as VisualVM or YourKit can help you understand memory usage and CPU consumption during startup. They can provide valuable insights into which beans consume the most resources.
import java.util.Arrays;
public class StartupController {
public void logStartupTime() {
long start = System.currentTimeMillis();
// logic to initialize beans
long end = System.currentTimeMillis();
System.out.println("Startup Time: " + (end - start) + "ms");
}
}
Why: Logging the startup time can guide you in optimizing bean initialization and help in allocating resources efficiently.
Closing the Chapter
While Spring offers powerful tools for Java development, understanding its startup process and the potential challenges can lead to a more efficient development experience. Employing strategies like lazy loading, setter injection, profile-based configurations, monitoring with Actuator, and using profiling tools can help mitigate hazards commonly associated with Spring application startup.
For those interested in diving deeper into Spring’s powerful features, consult the official Spring documentation. By addressing these challenges proactively, developers can ensure smoother deployments, improve application performance, and ultimately save themselves time and resources.
Remember, the better you visualize and understand your application startup, the easier it becomes to build robust and efficient applications with Spring! Happy coding!