Mastering Java Streams: Avoiding Common Pitfalls with `contains`
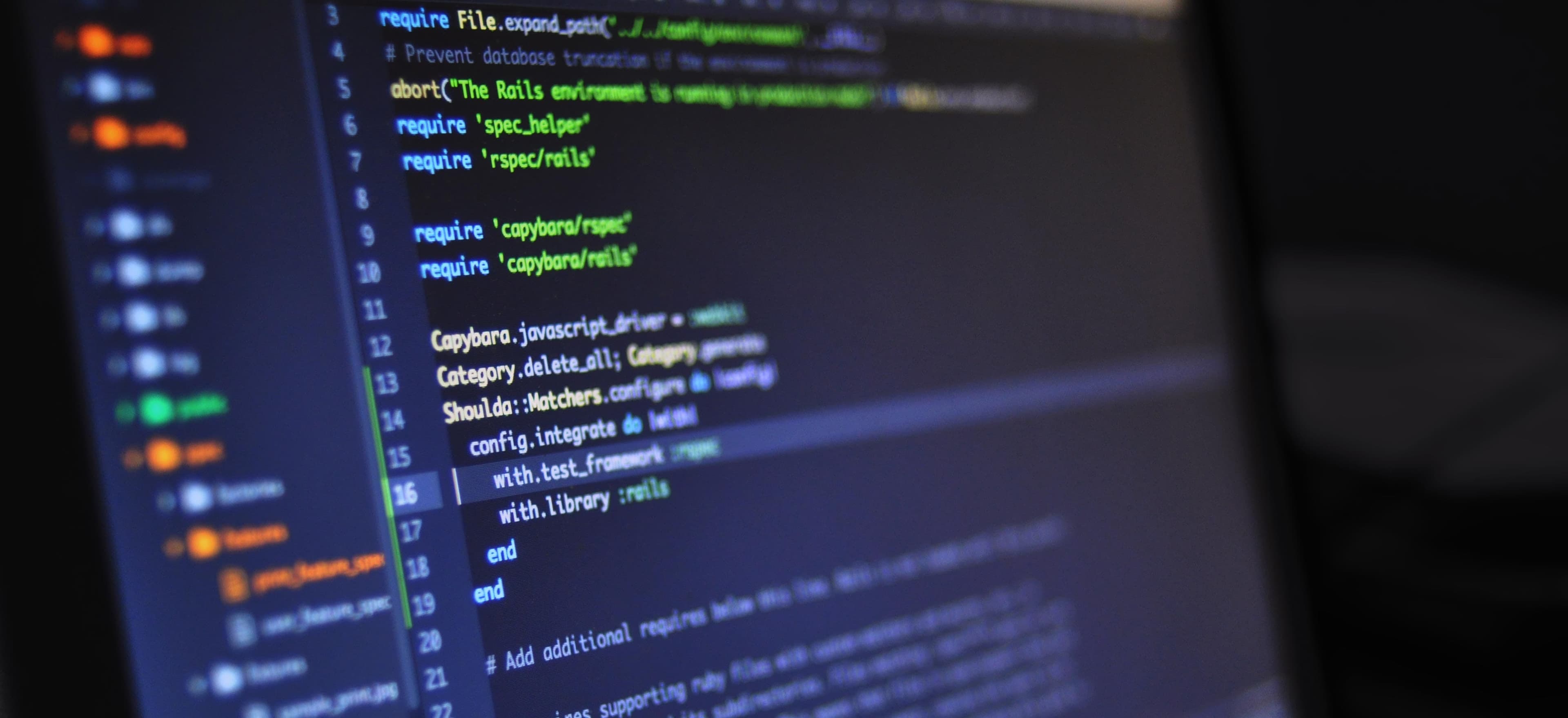
- Published on
Mastering Java Streams: Avoiding Common Pitfalls with contains
Java Streams have transformed the way we handle collections, allowing developers to write cleaner and more efficient code. However, as with any powerful feature, it's essential to understand its nuances. One of the common areas where developers can stumble is using the method contains
in conjunction with Java Streams. In this blog post, we'll explore this topic in depth and share some best practices to avoid common pitfalls.
Table of Contents
- What Are Java Streams?
- The
contains
Method Explained - Common Pitfalls When Using
contains
with Streams - Best Practices to Avoid Pitfalls
- Conclusion
What Are Java Streams?
Java Streams are a powerful abstraction introduced in Java 8. They simplify the process of manipulating and processing collections of data. Streams allow you to express complex data processing queries in a declarative way.
For example, consider the following code snippet that filters a list of integers to keep only even numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
In this code, we obtain a stream from the numbers
list, filter out odd numbers, and collect the results back into a list. The chainable method calls provide clear readability.
The contains
Method Explained
The contains
method is part of the Java Collection Framework. It checks whether a certain element exists in a collection. Its signature is as follows:
boolean contains(Object o);
This method is straightforward, but when combined with Java Streams, it can lead to less efficient and sometimes erroneous code.
For instance, if we want to check if a certain number exists in a list, we might consider:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
boolean exists = numbers.contains(3);
This works fine, but what happens when we need to combine contains
with other operations on our Stream?
Common Pitfalls When Using contains
with Streams
1. Inefficient Searches
A common pitfall is using contains
inappropriately within the context of a Stream pipeline. Instead of using contains
, which is an O(n) operation, you could use Streams to achieve better performance. Consider this example:
List<String> items = Arrays.asList("apple", "banana", "orange");
List<String> searchItem = Arrays.asList("banana", "grape");
for (String item : searchItem) {
if (items.contains(item)) {
// do something
}
}
This code checks if each item in searchItem
exists in items
. While this works, it checks contains
for each item, leading to nested iterations that can quickly degrade performance.
2. Using anyMatch
Instead of contains
A more efficient way to check for existence in conjunction with streams is using anyMatch
. This avoids creating another collection to search through.
Here’s the correct approach using anyMatch
:
boolean exists = searchItem.stream()
.anyMatch(items::contains);
In this code, we check if any item in searchItem
is also present in items
. It's more efficient since the search stops as soon as a match is found.
3. Null Pointer Exceptions
Another potential pitfall is null values in collections. Since both contains
and Streams can handle null values, a misunderstanding can lead to upstream exceptions if not properly checked.
Consider this code:
List<String> items = Arrays.asList("apple", null, "orange");
boolean containsNull = items.contains(null); // true
However, if you were to mistakenly filter a stream:
boolean containsNullStream = items.stream()
.filter(Objects::nonNull)
.anyMatch(item -> item.equals(null)); // This will always be false
Here, the filter excludes nulls, and thus any match would not be possible.
Best Practices to Avoid Pitfalls
-
Use
anyMatch
overcontains
: Instead of relying oncontains
, opt foranyMatch
for better performance and readability.boolean found = searchItems.stream() .anyMatch(item -> items.contains(item));
-
Guard Against Null Values: Always check collections for null values before operating on them. Consider using
Optional
for safer null handling.if (items != null && searchItem != null) { boolean found = searchItem.stream().anyMatch(items::contains); }
-
Utilize Sets for Fast Lookups: If you're frequently checking for the existence of elements, consider using a Set instead of a List. Sets offer O(1) average time complexity for
contains
.Set<String> itemSet = new HashSet<>(items); boolean exists = itemSet.contains("banana");
-
Readability Over Cleverness: Don’t sacrifice code readability for cleverness. Clear and straightforward code is easier to maintain.
-
Use Java Documentation: Familiarize yourself with the Java Streams Documentation for a thorough understanding of available methods and best practices.
Closing the Chapter
Java Streams offer an incredible opportunity to enhance productivity and code quality. However, it's crucial to understand the methods we employ and the implications they carry. By avoiding the common pitfalls associated with contains
and applying best practices, you can write more efficient, clear, and maintainable code. Embrace the power of Streams, and continue to learn and expand your coding skills.
For further exploration, consider reading more about stream performance here.
By keeping your code optimized while utilizing Java Streams, you'll significantly improve your overall development efficiency. Happy coding!
Checkout our other articles