Overcoming Integration Challenges in Cloud-Native Environments
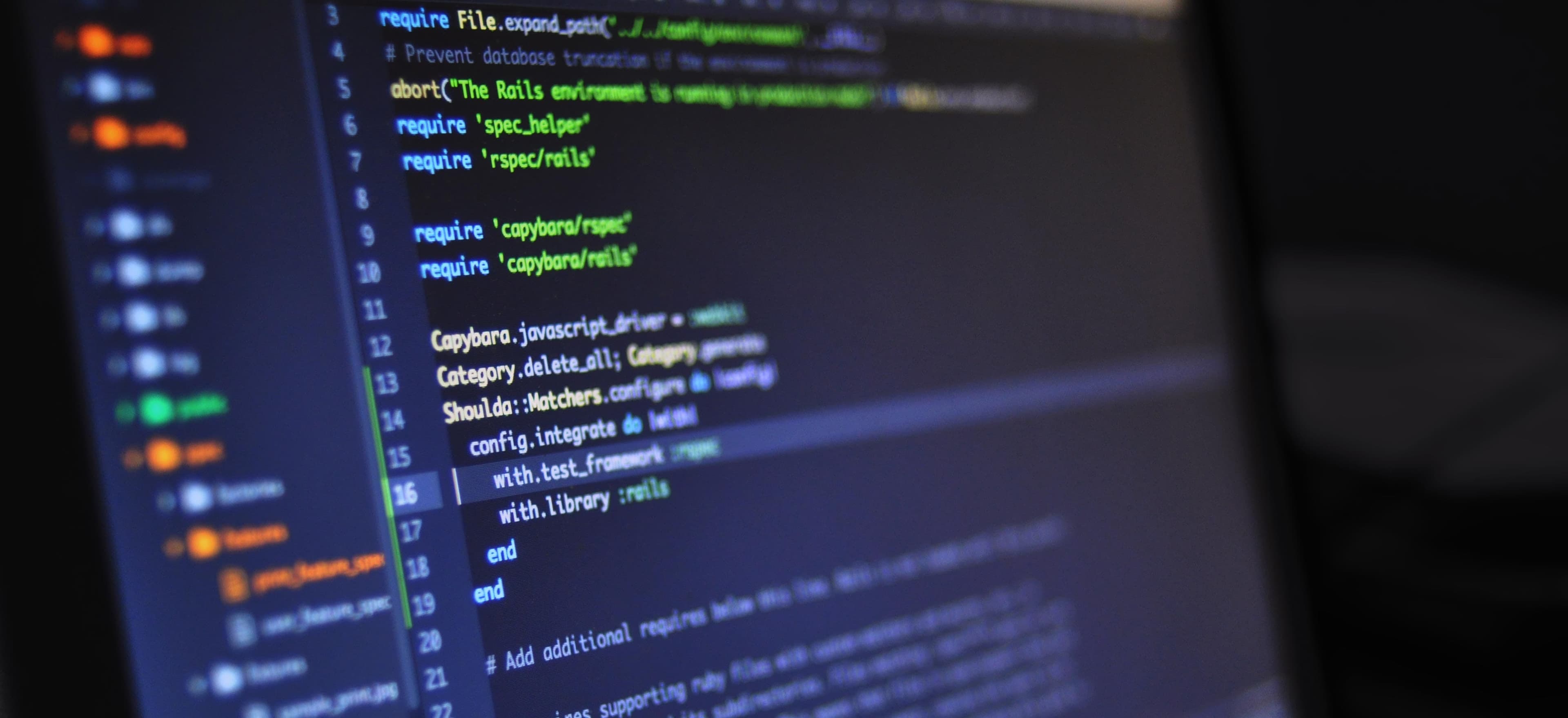
- Published on
Overcoming Integration Challenges in Cloud-Native Environments
In an era where businesses are increasingly turning to cloud-native solutions, the need for effective integration across various services and applications has never been greater. Integrating components in a cloud-native environment presents unique challenges that require innovative solutions. In this blog post, we will explore these challenges and discuss strategies for overcoming them.
Understanding Cloud-Native Architecture
Before diving into integration challenges, it’s important to understand what a cloud-native architecture entails. Cloud-native applications are designed for the cloud, leveraging microservices, containers, and dynamic orchestration technologies like Kubernetes. This architecture promotes agility, scalability, and resilience.
Key Characteristics of Cloud-Native Applications
- Microservices: Applications are designed as a suite of small services, each running independently and communicating over well-defined APIs.
- Containerization: Services are packaged in containers, providing consistency across various environments.
- Dynamic orchestration: Tools like Kubernetes manage the deployment and scaling of containerized applications.
Understanding these characteristics helps lay the foundation for discussing integration challenges.
Common Integration Challenges
While cloud-native architectures offer numerous advantages, they also introduce specific integration challenges:
- Service Discovery
- Data Consistency
- Inter-Service Communication
- Security and Compliance
- Monitoring and Logging
Let’s explore these challenges in-depth.
1. Service Discovery
In a microservices architecture, services are often instantiated dynamically. This makes it crucial to implement effective service discovery mechanisms. Without proper service discovery, components may be unable to find and communicate with each other.
Solution: Use a service discovery tool like Consul or Eureka.
Example Code Snippet
Here’s a simple example of a Spring Boot service using Eureka for service discovery.
// Application code to register with Eureka
@EnableEurekaClient
@SpringBootApplication
public class EurekaClientApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaClientApplication.class, args);
}
}
Why: The @EnableEurekaClient
annotation enables the application to register itself with the Eureka server, allowing it to be discovered by other services. This is crucial for maintaining dynamic communication in microservices.
2. Data Consistency
In a distributed system, maintaining data consistency across services is challenging. A failure in one microservice should not lead to data corruption or inconsistencies in another.
Solution: Implement patterns like Saga or two-phase commit to manage transactions across microservices.
Example Code Snippet
Here’s a brief illustration of how to implement a Saga pattern using Spring Boot:
public class BookingSaga {
private final RestaurantService restaurantService;
private final PaymentService paymentService;
public void bookFlight(FlightRequest request) {
try {
restaurantService.reserve(request.getRestaurantId());
paymentService.process(request.getPaymentId());
} catch (Exception e) {
// Implement compensation mechanism
restaurantService.cancelReservation(request.getRestaurantId());
}
}
}
Why: The Saga pattern allows each step of a business process to be compensated if subsequent steps fail. This ensures that the overall system maintains data integrity.
3. Inter-Service Communication
Microservices often need to communicate with each other. However, traditional synchronous communication can block services and lead to cascading failures.
Solution: Use asynchronous communication or messaging queues like RabbitMQ or Apache Kafka.
Example Code Snippet
Below is an example of how to send and receive messages using RabbitMQ in a Spring Boot application.
// Producer
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("exchangeName", "routingKey", message);
}
// Consumer
@RabbitListener(queues = "queueName")
public void receiveMessage(String message) {
// Process the message
}
Why: Asynchronous messaging decouples services, allowing them to operate independently. If one service fails, others can continue to function, enhancing system resilience.
4. Security and Compliance
Cloud-native environments require compliance with various regulations, including GDPR and HIPAA. Managing security in a distributed architecture can be complex.
Solution: Employ a dedicated API Gateway for managing security, traffic, and monitoring.
Example Code Snippet
Here’s how you can manage security using Spring Cloud Gateway:
// Configuration for security
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
http.authorizeExchange()
.pathMatchers("/api/**").authenticated()
.anyExchange().permitAll()
.and().oauth2Login();
return http.build();
}
Why: The API Gateway acts as the single entry point to your system, allowing for centralized authentication and security policy enforcement.
5. Monitoring and Logging
In a microservices architecture, monitoring can be challenging due to the distributed nature of services. Proper logging and monitoring help track performance and diagnose issues.
Solution: Use tools like Prometheus for monitoring and ELK Stack for centralized logging.
Example Code Snippet
Here’s a basic setup for integrating Spring Boot with ELK:
@EnableElkLogging
@SpringBootApplication
public class ElkLoggingApplication {
public static void main(String[] args) {
SpringApplication.run(ElkLoggingApplication.class, args);
}
}
Why: Centralized logging enables easier troubleshooting across multiple services, reducing the time needed to address issues.
Best Practices for Successful Integration
To effectively integrate your cloud-native applications, consider following these best practices:
- Embrace a Microservices Mindset: Adopt a domain-driven design to break down your applications into smaller services.
- Automate Everything: Use CI/CD pipelines for automating deployments and tests.
- Use API Contracts: Leverage tools like Swagger to create API contracts, ensuring that all services adhere to a defined standard.
- Implement Robust Monitoring: Set up alerts and dashboards to keep an eye on service health and performance.
The Closing Argument
Integrating components in a cloud-native environment is fraught with challenges. However, by leveraging the right tools and following best practices, organizations can create resilient, scalable systems that meet business needs. Whether it’s using service discovery tools like Eureka or adopting messaging systems like RabbitMQ, the strategies discussed here help address integration issues effectively.
For more insights on cloud-native architecture and practices, check out related articles on Cloud Native Computing Foundation, and stay updated on the latest trends in cloud computing.
By understanding and addressing these integration challenges, we can harness the full potential of cloud-native applications, leading to innovation and competitive advantage in the market.