Top Books Advanced Java Developers Shouldn't Miss
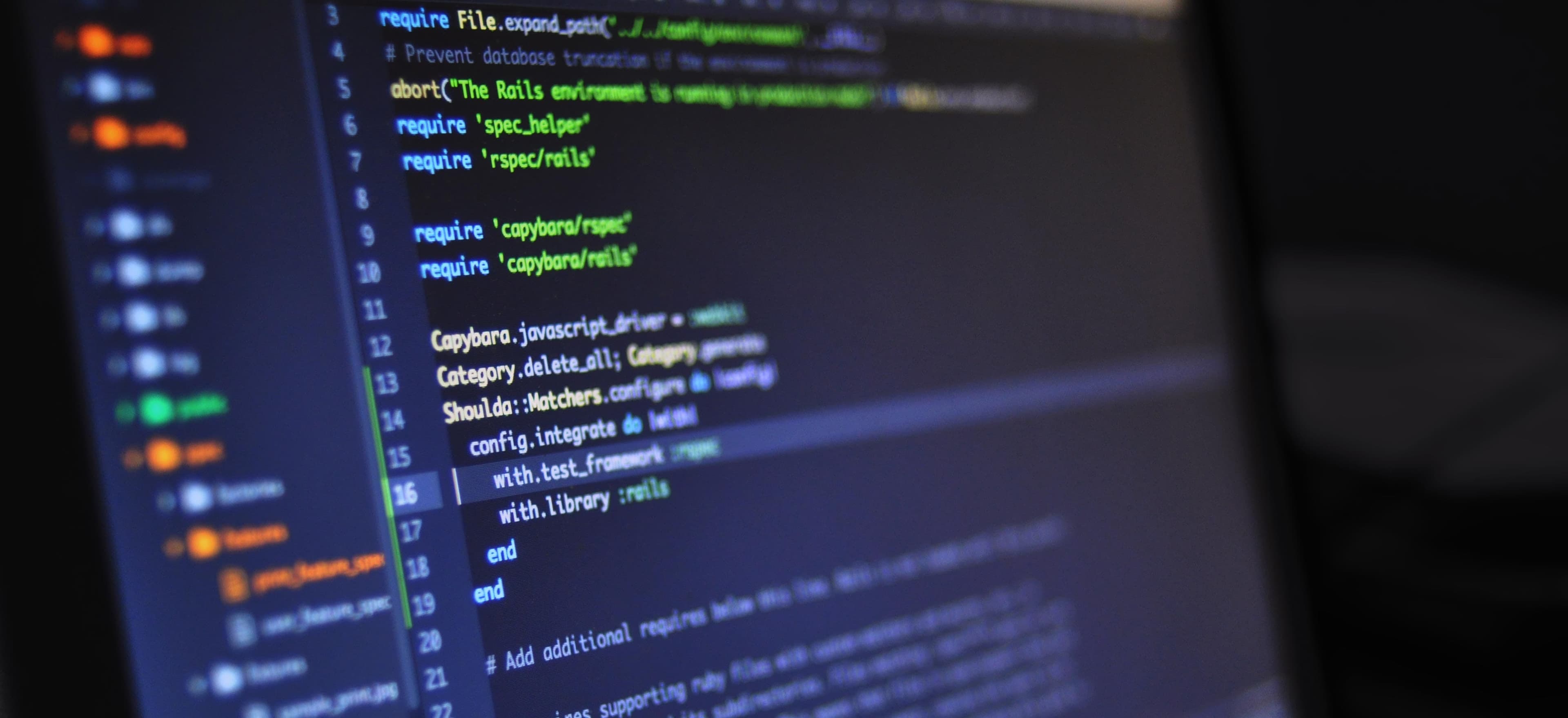
- Published on
Top Books Advanced Java Developers Shouldn't Miss
As an advanced Java developer, deepening your knowledge is both a necessity and a passion. There is always something new to learn. In this blog post, we will explore the best books that can elevate your Java expertise, covering advanced concepts, design patterns, performance tuning, and much more. Let’s dive into this reading list that promises to enhance your skills.
1. Effective Java by Joshua Bloch
Why Read It?
"Effective Java" is often considered the Bible for Java developers. Joshua Bloch, one of the architects of the Java programming language, shares insights that can help you write robust and maintainable code.
Key Takeaways:
- Best Practices: Bloch discusses 78 best practices for Java programming, some of which can significantly impact your development experience.
- Design Patterns: The book illustrates various design patterns and when to apply them.
Code Snippet:
Here's a quick example of the 'Builder Pattern' highlighted in the book for constructing complex objects:
public class NutritionFacts {
private final int servingSize; // (mL) required
private final int servings; // (per container) required
private final int calories; // (per serving) optional
private final int fat; // (g/serving) optional
public static class Builder {
// Required parameters
private final int servingSize;
private final int servings;
// Optional parameters - initialized to default values
private int calories = 0;
private int fat = 0;
public Builder(int servingSize, int servings) {
this.servingSize = servingSize;
this.servings = servings;
}
public Builder calories(int val) {
calories = val;
return this;
}
public Builder fat(int val) {
fat = val;
return this;
}
public NutritionFacts build() {
return new NutritionFacts(this);
}
}
private NutritionFacts(Builder builder) {
servingSize = builder.servingSize;
servings = builder.servings;
calories = builder.calories;
fat = builder.fat;
}
}
Why This Matters:
The Builder Pattern allows for the simplified creation of complex objects. It's particularly useful when you have immutable objects with many parameters.
2. Java Concurrency in Practice by Brian Goetz
Why Read It?
Concurrency is essential in modern software development. Brian Goetz presents an insightful exploration of building concurrent applications in Java. The book is packed with practical advice and strategies.
Key Takeaways:
- Thread Safety: Understand how to manage thread safety and the common pitfalls in concurrent programming.
- Synchronization: Get acquainted with concepts like locks, atomic variables, and concurrent collections.
Code Snippet:
A prime example demonstrating the use of synchronized methods to achieve thread safety:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
Why This Matters:
Using synchronized methods ensures that only one thread can execute increment or getCount at any time, reducing the risk of inconsistencies.
3. Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
Why Read It?
"Clean Code" illuminates the importance of writing code that is easy to understand and maintain. As advanced developers, we should always look to improve our code's readability and structure.
Key Takeaways:
- Refactoring: Learn the art of refactoring and how to identify and eliminate code smells.
- Naming Conventions: The book emphasizes proper naming and how it can make your code self-documenting.
Code Snippet:
Here’s an example of a refactoring technique to improve readability:
// Before Refactoring
public double calculate(int a, int b) {
return a * 0.20 + b * 0.80;
}
// After Refactoring
public double calculateTotalCost(int baseCost, int additionalCost) {
return baseCost * 0.20 + additionalCost * 0.80;
}
Why This Matters:
Refactoring code to have meaningful names enhances its clarity. Developers who read your code should grasp the intent without excessive context.
4. Java Performance: The Definitive Guide by Scott Oaks
Why Read It?
Performance tuning is a critical aspect of software development, and Scott Oaks provides a comprehensive guide on Java performance metrics, profiling, and optimization.
Key Takeaways:
- Garbage Collection: Understand the nuances of Java's garbage collection and how to optimize it.
- Profiling Tools: Incorporate tools like JVisualVM, JConsole, and more for effective performance tuning.
Code Snippet:
Utilizing the tuning options for the Garbage Collector could look something like this:
java -XX:+UseG1GC -Xms512m -Xmx4g -jar YourApplication.jar
Why This Matters:
Choosing the right garbage collector and configuring heap sizes can dramatically improve application performance, making your application more responsive and resource-efficient.
5. Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides
Why Read It?
Commonly referred to as the "Gang of Four" book, this classic text provides in-depth discussions on design patterns that have shaped software engineering.
Key Takeaways:
- Pattern Catalog: Comprehensive coverage of 23 design patterns and when to apply them.
- Problem-Solving: Patterns serve as tried-and-true solutions for common design problems.
Code Snippet:
Here’s an example of the Singleton Pattern:
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Why This Matters:
The Singleton Pattern restricts instantiation of a class to a single object, which is essential when control over resource usage is critical.
6. The Pragmatic Programmer by Andrew Hunt and David Thomas
Why Read It?
Though not purely focused on Java, this book is a treasure trove of wisdom for developers at any stage of their careers. It addresses broader programming philosophy and practices.
Key Takeaways:
- Outsmart Your Tools: Learn tips and tricks to maximize your coding effectiveness.
- Personal Responsibility: Encourages developers to take responsibility for their quality of code and team collaboration.
Code Snippet:
One gem from the book emphasizes the practice of Version Control:
git init
git add .
git commit -m "Initial Commit"
Why This Matters:
Version Control systems help you keep track of changes, collaborate better, and manage different versions of your code effectively.
Final Thoughts
These books are indispensable resources for advanced Java developers aiming to sharpen their skills and broaden their understanding of the language and its ecosystem. Whether you are looking to master concurrency, improve your coding practices, or learn design patterns, this reading list will serve as a strong foundation for further exploration in Java.
For more information on Java programming, check out Oracle's Official Java Documentation and consider exploring online platforms like Coursera or Udemy for courses that align with the concepts found in these books.
Happy reading and coding!
Checkout our other articles