How to Effectively Prioritize Bug Severity in Testing
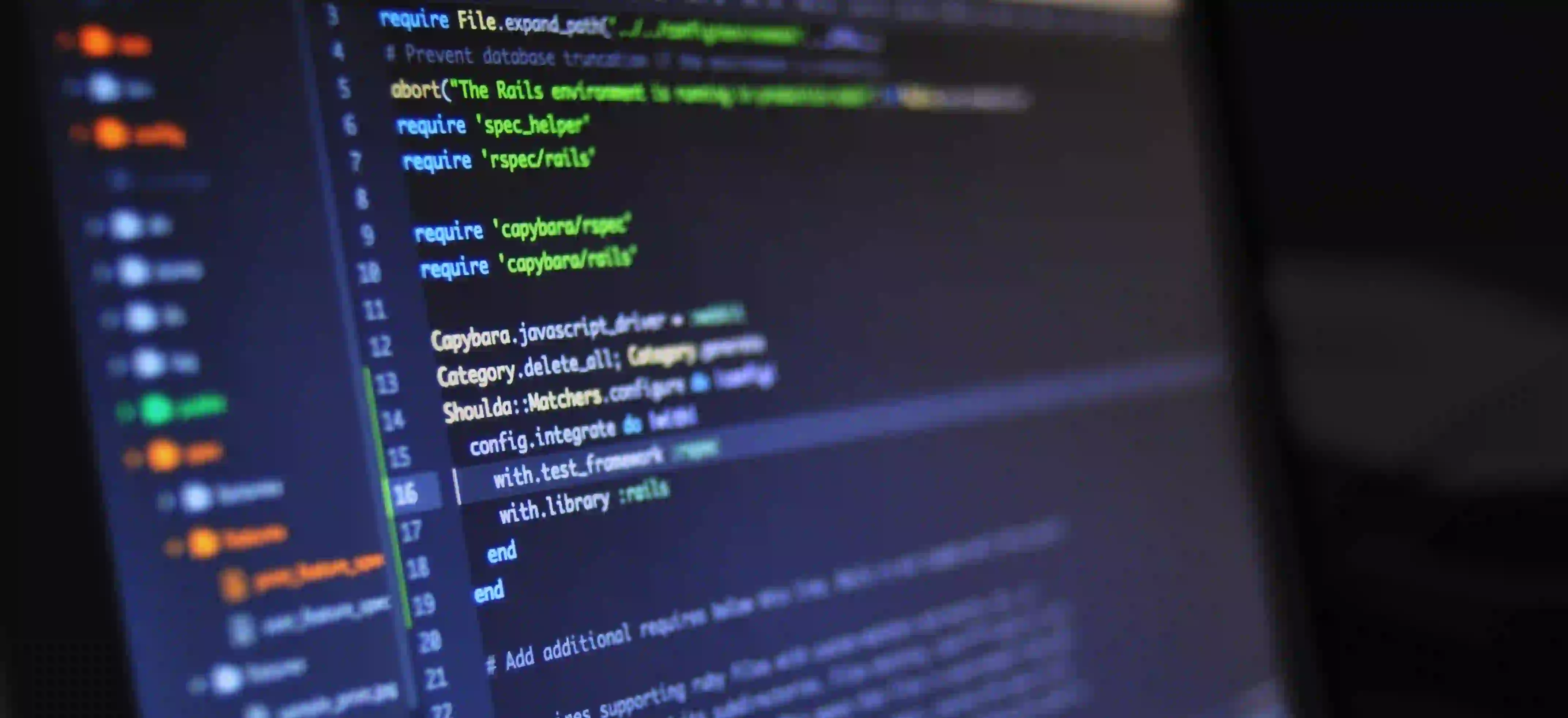
How to Effectively Prioritize Bug Severity in Testing
Bug prioritization is an essential part of the software testing process. When a bug is found, it is critical to determine its severity and determine how quickly it needs to be addressed. Without an effective prioritization system, development teams may become overwhelmed by bug reports, leading to delays in releases, reduced product quality, and wasted resources. In this blog post, we will explore how to effectively prioritize bug severity in testing and provide some best practices to make the process smoother and more effective.
Understanding Bug Severity
Before diving into prioritization, let's clarify what we mean by "bug severity." Bug severity is a classification that describes the impact of a bug on the application. It does not necessarily correlate directly with the priority of fixing the bug. For instance, a critical bug that affects a small feature may not be a top priority if it is not mission-critical for the current release.
Severity Levels
There are typically four levels of bug severity:
- Critical: The application crashes, data loss occurs, or a feature is completely unusable. These bugs need immediate attention.
- Major: Major functionality is hindered, but the application still runs. Workarounds may exist but are not optimal or user-friendly.
- Minor: These bugs do not severely impact functionality. They are annoying, but users can continue working unimpeded.
- Trivial: These are cosmetic issues or minor errors that do not affect the functionality of the application. They can often wait until the subsequent release.
Factors Influencing Bug Prioritization
Several factors influence how you prioritize bug severity:
1. Business Impact
Understanding the business impact of each bug is crucial. A bug in a mission-critical feature may be classified as a minor bug but can carry significant weight for the business's success. Assessing the bug's impact on customers, revenue, or operational efficiency can help prioritize effectively.
2. Frequency of Occurrence
If a bug is encountered frequently by users, it will naturally take higher precedence over glitches that appear infrequently. Utilize analytics to examine how often users experience the issue and prioritize accordingly.
3. User Experience
Consider the overall user experience when prioritizing bugs. Major user experience issues should be dealt with swiftly to maintain customer satisfaction and loyalty. Users encountering severe bugs are likely to abandon the product, leading to loss of revenue.
4. Regulatory Compliance
In some industries, failing to address bugs can lead to regulatory issues. If a bug affects compliance with industry regulations, it should be prioritized.
5. Reproducibility
Bugs that are easily reproducible are generally easier to fix and should be prioritized accordingly. In contrast, intermittent bugs that require extensive investigation may be deprioritized until a clearer understanding of the issue is established.
Methodology for Prioritizing Bugs
Now that we understand the factors that influence prioritization, let’s explore a step-by-step methodology.
Step 1: Triage Bugs
Triage is the first step in the prioritization process. During triage, testers and developers review each reported bug to assess severity and business impact. Assign severity levels according to the classifications described earlier. Collaboration between testers and developers can provide a rounded perspective on the bug’s impact.
Step 2: Rank Bugs
After triaging, create a list of bugs ranked by severity and business impact. This list should serve as a guide for the development team to allocate resources effectively. An example ranking matrix could look like this:
| Bug ID | Severity | Business Impact | Rank | |--------|------------|-----------------|------| | 001 | Critical | High | 1 | | 002 | Major | Medium | 2 | | 003 | Minor | Low | 3 | | 004 | Trivial | None | 4 |
Step 3: Communicate Priorities
Share the ranked list with the development team. Clear communication is essential to ensure everyone is on the same page regarding what needs immediate attention. Tools like JIRA or Trello can be tremendously helpful in managing and tracking bug priorities.
Step 4: Reassess Priorities Regularly
Bug priorities may change as new information becomes available. For instance, a bug marked as critical might have temporary workarounds, allowing the team to shift focus elsewhere. Schedule regular reassessments of the bug list to adapt to any new developments.
Example Code Snippet
To illustrate a practical bug-fixing scenario, let’s take a look at a Java program snippet that deals with user input validation—a common source of bugs.
import java.util.Scanner;
public class UserInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your age: ");
int age = validateAge(scanner);
if (age != -1) {
System.out.println("Your age is: " + age);
} else {
System.out.println("Please enter a valid age.");
}
}
public static int validateAge(Scanner scanner) {
try {
String input = scanner.nextLine();
int age = Integer.parseInt(input);
// Severity: Minor. Business impact: Low, but user experience: crucial
if (age < 0) {
System.out.println("Age cannot be negative.");
return -1; // Bug can be fixed by additional validations.
}
return age;
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter a numeric value.");
return -1; // Minor bug, can wait for the next iteration.
}
}
}
Explanation of the Code
In the above snippet, we briefly demonstrate user input validation. The code will need enhancement if:
- The user enters a negative age. This can confuse users (Minor Severity).
- The input is not numeric, leading to a crash, thus constituting a Critical issue.
Approaching these bugs from the severity perspective equips developers with insights on how to address issues strategically. Minor bugs can often wait, while critical issues necessitate immediate resources.
A Final Look
Effectively prioritizing bug severity in testing is a combination of understanding the nature of the bugs, the business impact they carry, and a structured methodology for assessment. By ranking and triaging bugs, communicating priorities, and reassessing them regularly, teams can streamline their efforts and address the critical bugs promptly.
For more information on software testing and best practices, consider visiting these resources:
- ISTQB Foundation Level Syllabus
- Software Testing Fundamentals
Utilizing a clear, organized approach to bug prioritization will not only resolve issues faster but also enhance the quality and stability of the software released, leading to increased user satisfaction and loyalty. Happy testing!