Mastering Custom Collectors: Avoiding Common Pitfalls in Java 8
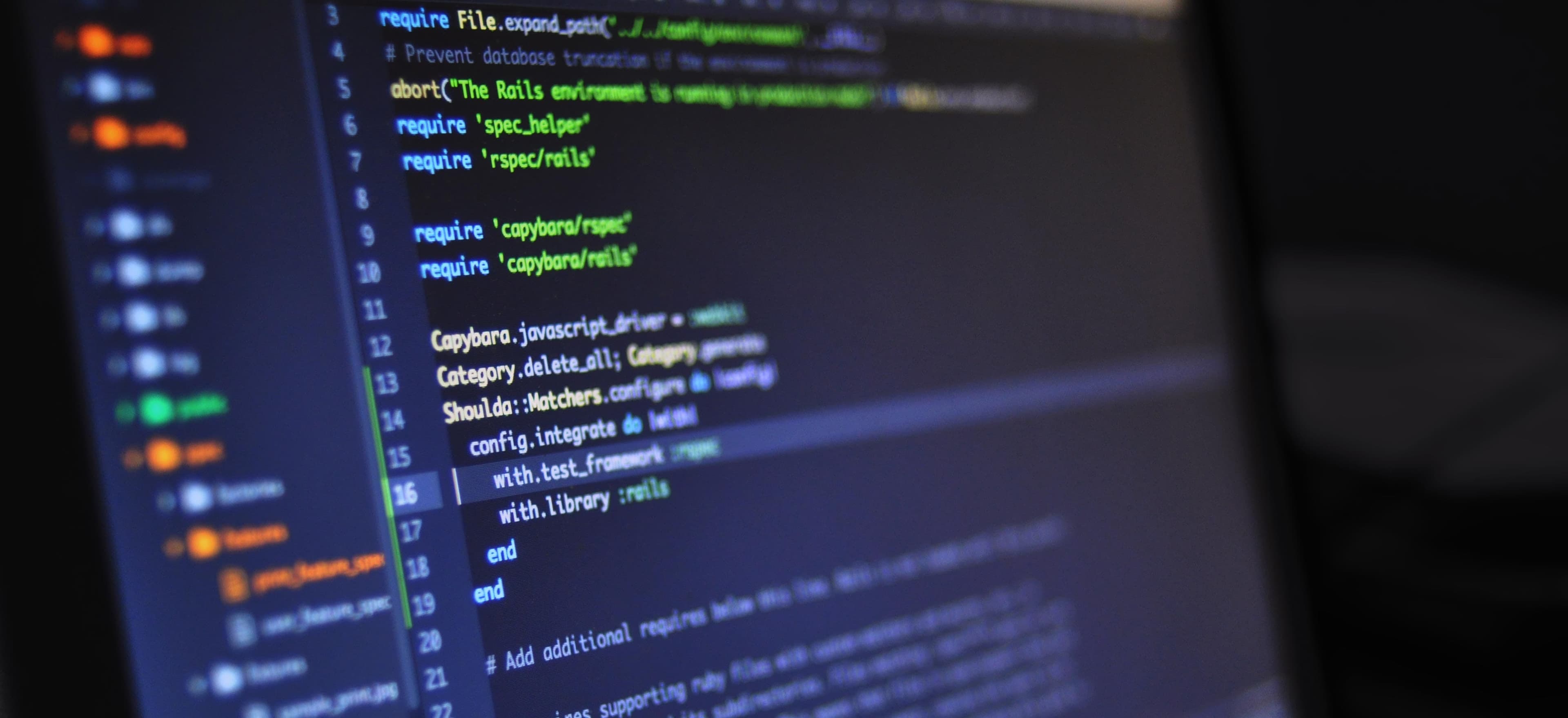
- Published on
Mastering Custom Collectors: Avoiding Common Pitfalls in Java 8
Java 8 introduced several powerful new features, among which is the Stream API. This API transformed how developers handle collections of data, allowing for a more functional programming style. One of the most intriguing capabilities within the Stream API is the ability to create custom collectors. However, with great power comes great responsibility. In this blog post, we will explore how to create effective custom collectors while avoiding common pitfalls.
Understanding Collectors
Before diving into custom collectors, let's quickly see what collectors are. With the Stream API, collectors are used to combine the elements of a stream into a single summary result. The Collectors
class offers many predefined collectors such as toList()
, toSet()
, and joining()
. Here is a basic example:
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class CollectorExample {
public static void main(String[] args) {
List<String> names = Stream.of("Alice", "Bob", "Charlie")
.collect(Collectors.toList());
System.out.println(names); // Output: [Alice, Bob, Charlie]
}
}
In this example, Collectors.toList()
takes a stream of names and accumulates them into a List.
Why Create Custom Collectors?
Custom collectors come into play when predefined collectors don't meet specific requirements. They allow for flexibility and can encapsulate complex collection logic. Here are a few situations where you might need them:
- Aggregating complex data structures (e.g., converting a list of objects into a Map).
- Custom merging strategies during parallel processing.
- Implementing specific business logic that predefined collectors cannot handle.
Let’s look into how we can implement a custom collector.
Creating a Custom Collector
Creating a custom collector involves implementing the Collector
interface. Here's a basic example of a collector that counts the occurrences of each word in a stream.
Step 1: Define the Collector
import java.util.Map;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import java.util.function.BiConsumer;
import java.util.function.Supplier;
import java.util.function.Function;
public class WordCountCollector {
public static Collector<String, ?, Map<String, Long>> toWordCount() {
return Collector.of(
// Supplier
() -> new java.util.HashMap<String, Long>(),
// Accumulator
(map, word) -> map.merge(word, 1L, Long::sum),
// Combiner
(left, right) -> {
right.forEach((word, count) -> left.merge(word, count, Long::sum));
return left;
}
);
}
}
Commentary on the Code
- Supplier: This creates a new
HashMap
to store word counts. - Accumulator: This takes each word and updates the count. It uses
merge
to add the word or increment its count. - Combiner: This combines two maps when processing streams in parallel. It ensures that word counts are aggregated correctly.
Step 2: Use the Collector
Now, let’s see how to utilize this custom collector to count words in a list.
import java.util.Arrays;
import java.util.Map;
public class CustomCollectorExample {
public static void main(String[] args) {
Map<String, Long> wordCounts = Arrays.asList("apple", "banana", "apple", "orange", "banana", "banana")
.stream()
.collect(WordCountCollector.toWordCount());
System.out.println(wordCounts); // Output: {orange=1, apple=2, banana=3}
}
}
Common Pitfalls and How to Avoid Them
While creating custom collectors is powerful, there are common pitfalls developers encounter. Here are some to watch out for.
Pitfall 1: Incorrect Thread Safety
When combining results in parallel streams, ensure that your collector’s mutable state is thread-safe. Using a concurrent collection can help mitigate state conflicts.
// Instead of HashMap, use ConcurrentHashMap
() -> new java.util.concurrent.ConcurrentHashMap<String, Long>()
Pitfall 2: Inefficient Merging
The combiner function should be efficient as it can be called multiple times during parallel processing. Avoid O(n) complexities in the combiner by ensuring you are not repeatedly iterating over the map during merges.
Pitfall 3: Ignoring Edge Cases
Make sure your custom collector correctly handles edge cases such as:
- Empty streams
- Null values (for streams of reference types)
For instance, you might want to check for null
values in your accumulator.
// Accumulator
(map, word) -> {
if (word != null) {
map.merge(word, 1L, Long::sum);
}
}
Best Practices for Creating Custom Collectors
-
Start Simple: Before implementing complex logic, try to create simple collectors to understand the framework better.
-
Test Thoroughly: Implement unit tests for both single-threaded and multi-threaded scenarios.
-
Leverage Complete Collector: Use
Collector.of
with all necessary components (supplier, accumulator, combiner) to maintain clarity and structure in your code. -
Provide Comprehensive Documentation: If others will use or maintain your collector, provide clear documentation explaining its purpose and usage.
-
Optimization: Consider optimization opportunities, particularly in memory and performance, especially when dealing with large datasets.
Final Considerations
Custom collectors in Java 8 open up a realm of possibilities for how data is aggregated and managed within your applications. However, with that flexibility comes complexity. By understanding the fundamental components of a collector, avoiding common pitfalls, and following best practices, you can harness the power of custom collectors effectively.
For more on Java 8 enhancements, consider exploring the official Java 8 documentation.
By mastering custom collectors, you not only enhance the functionality of your applications but also ensure they are more efficient and maintainable. Happy coding!
Checkout our other articles